
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
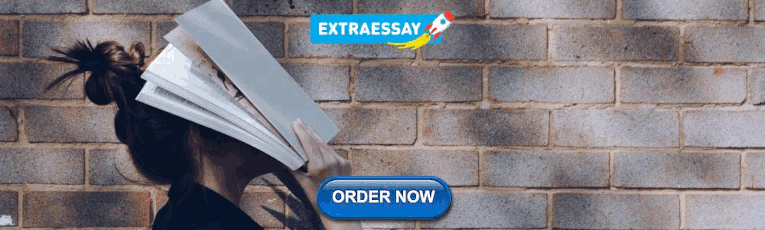
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- DSA Tutorial
- Data Structures
- Linked List
- Dynamic Programming
- Binary Tree
- Binary Search Tree
- Divide & Conquer
- Mathematical
- Backtracking
- Branch and Bound
- Pattern Searching
Constraint Satisfaction Problems (CSP) in Artificial Intelligence
Finding a solution that meets a set of constraints is the goal of constraint satisfaction problems (CSPs), a type of AI issue. Finding values for a group of variables that fulfill a set of restrictions or rules is the aim of constraint satisfaction problems. For tasks including resource allocation, planning, scheduling, and decision-making, CSPs are frequently employed in AI.
There are mainly three basic components in the constraint satisfaction problem:
Variables: The things that need to be determined are variables. Variables in a CSP are the objects that must have values assigned to them in order to satisfy a particular set of constraints. Boolean, integer, and categorical variables are just a few examples of the various types of variables, for instance, could stand in for the many puzzle cells that need to be filled with numbers in a sudoku puzzle.
Domains: The range of potential values that a variable can have is represented by domains. Depending on the issue, a domain may be finite or limitless. For instance, in Sudoku, the set of numbers from 1 to 9 can serve as the domain of a variable representing a problem cell.
Constraints: The guidelines that control how variables relate to one another are known as constraints. Constraints in a CSP define the ranges of possible values for variables. Unary constraints, binary constraints, and higher-order constraints are only a few examples of the various sorts of constraints. For instance, in a sudoku problem, the restrictions might be that each row, column, and 3×3 box can only have one instance of each number from 1 to 9.
Constraint Satisfaction Problems (CSP) representation:
- The finite set of variables V 1 , V 2 , V 3 ……………..V n .
- Non-empty domain for every single variable D 1 , D 2 , D 3 …………..D n .
- e.g., V 1 ≠ V 2
- Example: <(V 1 , V 2 ), V 1 not equal to V 2 >
- Scope = set of variables that participate in constraint.
- There might be a clear list of permitted combinations. Perhaps a relation that is abstract and that allows for membership testing and listing.
Constraint Satisfaction Problems (CSP) algorithms:
- The backtracking algorithm is a depth-first search algorithm that methodically investigates the search space of potential solutions up until a solution is discovered that satisfies all the restrictions. The method begins by choosing a variable and giving it a value before repeatedly attempting to give values to the other variables. The method returns to the prior variable and tries a different value if at any time a variable cannot be given a value that fulfills the requirements. Once all assignments have been tried or a solution that satisfies all constraints has been discovered, the algorithm ends.
- The forward-checking algorithm is a variation of the backtracking algorithm that condenses the search space using a type of local consistency. For each unassigned variable, the method keeps a list of remaining values and applies local constraints to eliminate inconsistent values from these sets. The algorithm examines a variable’s neighbors after it is given a value to see whether any of its remaining values become inconsistent and removes them from the sets if they do. The algorithm goes backward if, after forward checking, a variable has no more values.
- Algorithms for propagating constraints are a class that uses local consistency and inference to condense the search space. These algorithms operate by propagating restrictions between variables and removing inconsistent values from the variable domains using the information obtained.
Implementations code for Constraint Satisfaction Problems (CSP):
Implement constraint satisfaction problems algorithms with code, define the problem .
Here we are solving Sudoku Puzzle with Constraint Satisfaction Problems algorithms
Define Variables for the Constraint Satisfaction Problem
Define the domains for constraint satisfaction problem, define the constraint for constraint satisfaction problem, find the solution to the above sudaku problem, full code :, real-world constraint satisfaction problems (csp):.
- Scheduling: A fundamental CSP problem is how to efficiently and effectively schedule resources like personnel, equipment, and facilities. The constraints in this domain specify the availability and capacity of each resource, whereas the variables indicate the time slots or resources.
- Vehicle routing: Another example of a CSP problem is the issue of minimizing travel time or distance by optimizing a fleet of vehicles’ routes. In this domain, the constraints specify each vehicle’s capacity, delivery locations, and time windows, while the variables indicate the routes taken by the vehicles.
- Assignment: Another typical CSP issue is how to optimally assign assignments or jobs to humans or machines. In this field, the variables stand in for the tasks, while the constraints specify the knowledge, capacity, and workload of each person or machine.
- Sudoku: The well-known puzzle game Sudoku can be modeled as a CSP problem, where the variables stand in for the grid’s cells and the constraints specify the game’s rules, such as prohibiting the repetition of the same number in a row, column, or area.
- Constraint-based image segmentation: The segmentation of an image into areas with various qualities (such as color, texture, or shape) can be treated as a CSP issue in computer vision, where the variables represent the regions and the constraints specify how similar or unlike neighboring regions are to one another.
Constraint Satisfaction Problems (CSP) benefits:
- conventional representation patterns
- generic successor and goal functions
- Standard heuristics (no domain-specific expertise).
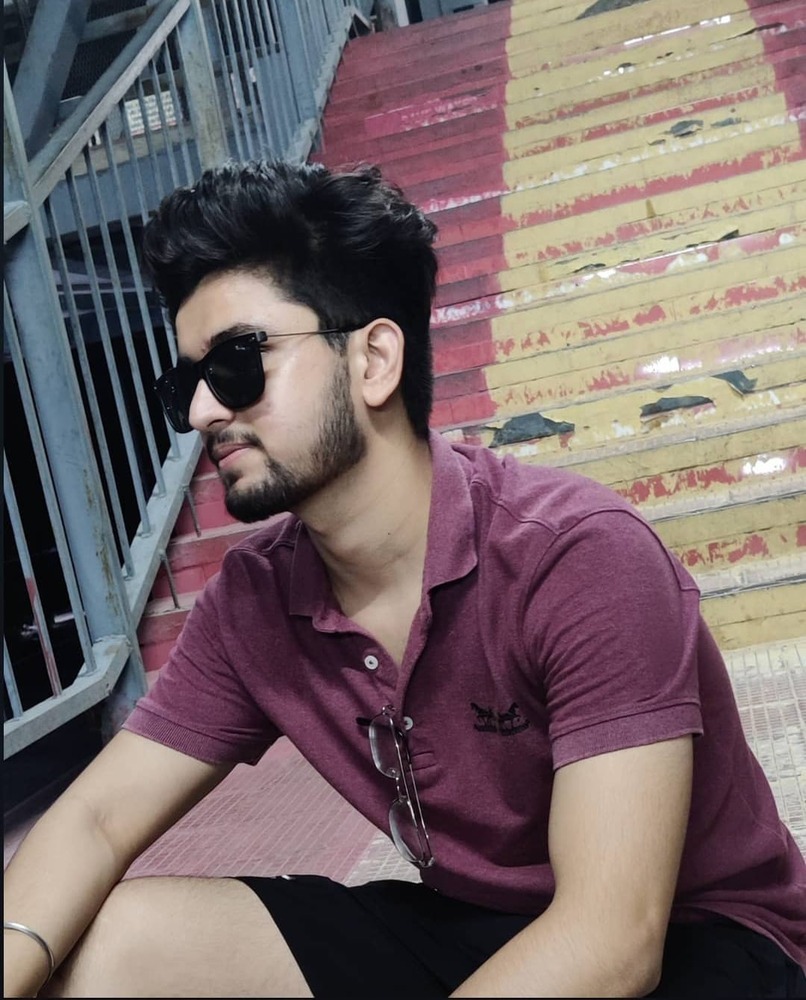
Please Login to comment...
Similar reads.
Improve your Coding Skills with Practice

What kind of Experience do you want to share?
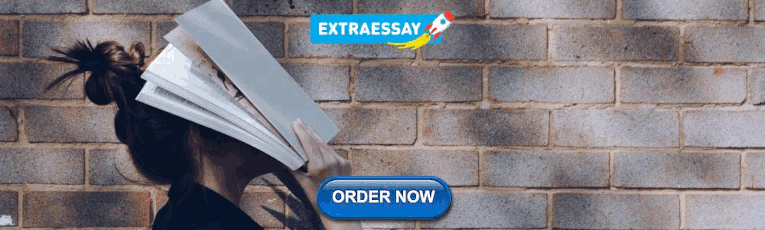
IMAGES
COMMENTS
Our DAA Tutorial includes all topics of algorithm, asymptotic analysis, algorithm control structure, recurrence, master method, recursion tree method, simple sorting algorithm, bubble sort, selection sort, insertion sort, divide and conquer, binary search, merge sort, counting sort, lower bound theory etc.
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Branch and bound is one of the techniques used for problem solving. It is similar to the backtracking since it also uses the state space tree. It is used for solving the optimization problems and minimization problems. If we have given a maximization problem then we can convert it using the Branch and bound technique by simply converting the ...
how to solve job assignment problem using branch and bound method Analysis and design of algorithms programs 31 subscribers Subscribed 2 294 views 3 years ago
In this video, we discuss the introduction of an Assignment problem and the mathematical representation of the Assignment problem.Link For Complete Playlist ...
Introduction: Time complexity is a critical concept in computer science and plays a vital role in the design and analysis of efficient algorithms and data structures. It allows us to measure the amount of time an algorithm or data structure takes to execute, which is crucial for understanding its efficiency and scalability.
Explained how job assignment problem is solved using Branch and Bound technique by prof. Pankaja Patil ...more
The quadratic assignment problem ( QAP) is one of the fundamental combinatorial optimization problems in the branch of optimization or operations research in mathematics, from the category of the facilities location problems first introduced by Koopmans and Beckmann.
Channel Allocation Problem in Computer Network. Channel allocation is a process in which a single channel is divided and allotted to multiple users in order to carry user specific tasks. There are user's quantity may vary every time the process takes place. If there are N number of users and channel is divided into N equal-sized sub channels ...
The transportation problem is commonly approached through simplex methods, and the assignment problem is addressed using specific algorithms like the Hungarian method. In this article, we will learn the difference between transportation problems and assignment problems with the help of examples.
Learn how to solve the job sequencing problem, a classic optimization problem in computer science, with examples and algorithms on GeeksforGeeks.
Java is a popular programming language that is used to develop a wide variety of applications. One of the best ways to learn Java is to practice writing programs. Many resources are available online and in libraries to help you find Java practice programs. When practising Java programs, it is important to focus on understanding the concepts ...
After reading this article you will learn about:- 1. Meaning of Assignment Problem 2. Definition of Assignment Problem 3. Mathematical Formulation 4. Hungarian Method 5. Variations. Meaning of Assignment Problem: An assignment problem is a particular case of transportation problem where the objective is to assign a number of resources to an equal number of activities so as to minimise total ...
Quadratic Assignment Problem Example | DAA | lec-38 Er Sahil ka Gyan 26.5K subscribers Subscribed 201 19K views 3 years ago RAJASTHAN #quadraticassignmentproblem #quadratic #assignmentproblem ...more
Learn Java programming with hundreds of exercises, practice problems and solutions on w3resource.com, a comprehensive online tutorial platform.
Java Inheritance Programming : Exercises, Practice, Solution - Improve your Java inheritance skills with these exercises with solutions. Learn how to create subclasses that override methods, add new methods, and prevent certain actions. An editor is available to practice and execute the code.
Java Programs or Java programming tutorial with examples of fibonacci series, armstrong number, prime number, palindrome number, factorial number, bubble sort, selection sort, insertion sort, swapping numbers etc.
Job Sequencing with Deadline - Job scheduling algorithm is applied to schedule the jobs on a single processor to maximize the profits.
Learn and practice Java collection with exercises, solutions, and examples. Explore different types of collections, methods, and operations.
Transportation problem is a special kind of Linear Programming Problem (LPP) in which goods are transported from a set of sources to a set of destinations subject to the supply and demand of the sources and destination respectively such that the total cost of transportation is minimized. It is also sometimes called as Hitchcock problem. Types ...
Merge Sort with Introduction, Asymptotic Analysis, Array, Pointer, Structure, Singly Linked List, Doubly Linked List, Circular Linked List, Binary Search, Linear ...
Data Structures | DS Tutorial with Introduction, Asymptotic Analysis, Array, Pointer, Structure, Singly Linked List, Doubly Linked List, Circular Linked List, Binary ...
Finding a solution that meets a set of constraints is the goal of constraint satisfaction problems (CSPs), a type of AI issue. Finding values for a group of variables that fulfill a set of restrictions or rules is the aim of constraint satisfaction problems. For tasks including resource allocation, planning, scheduling, and decision-making, CSPs are frequently employed in AI.