React-Portfolio
Unit 20 react homework: react portfolio.
Now that you’ve worked with React and have multiple projects to share, you’ll be updating your portfolio and other materials to build toward being employer competitive. Creating a portfolio using React will help set you apart from other developers whose portfolios do not use some of the latest technologies.
If you are opting out of career services, this is still a required assignment . Part of being a web developer means being a part of a community. Having a place to share your projects is necessary if you’re applying for jobs, but is still critical on your journey as a developer.
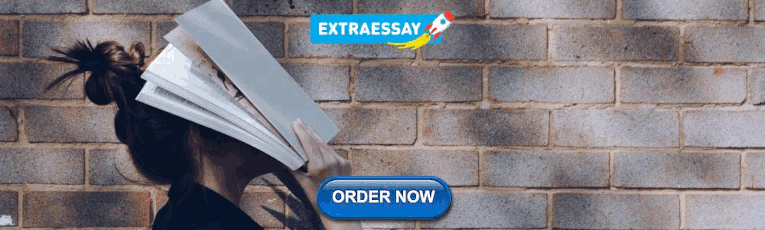
Requirements
Updated portfolio featuring 6 total projects
A Header component that appears on multiple pages
A single Project component that will be used multiple times on a single page
Navigation with React Router, dynamic rendering, or another third part router
A Footer component that appears on multiple pages
Update GitHub profile with pinned repositories featuring those same projects
Requirement | Weight |
---|---|
Portoflio | 90% |
GitHub Profile | 10% |
Instructions
Updated linkedin profile, updated portfolio.
Your updated site should still have all of the content it previously had:
Links to your GitHub profile & LinkedIn page as well as your email address and phone number
A link to a PDF of your resume with updated projects
A list of projects. For each project, make sure you have the following:
Project title
Link to the deployed version
Link to the GitHub repository
GIF or screenshot of the deployed application
As with the previous portfolio homework, “good” design is subjective. Your site should look “polished.” Here are a few guidelines on what that means:
Mobile-first design
Choose a color palette for your site so it doesn’t just look like the default bootstrap theme or an unstyled HTML site. You may we
Make sure the font size is large enough to read, and that the colors don’t cause eye strain.
If you want to go above and beyond, try using animations and react component libraries. Note that this will not affect your grade, but it may impact how potentials employers gauge your knowledge.
Additionally, this new portfolio should be created using React.
At a minimum, your portfolio should include the following:
Make sure to update your LinkedIn Profile with the new skills you’ve acquired since the last time it was updated.
© 2019 Trilogy Education Services, a 2U, Inc. brand. All Rights Reserved.
Create a portfolio using React and GitHub Student Developer Pack
In this blog we will be creating a Portfolio website using React and the tools provided by the GitHub Student Developer Pack.
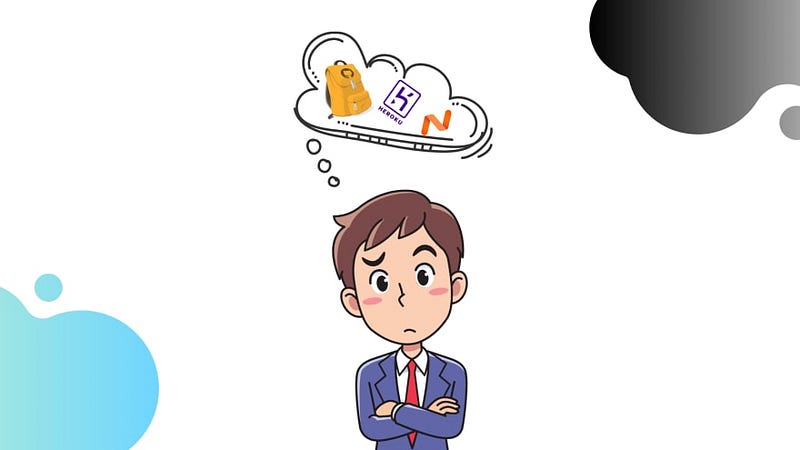
What is the GitHub Student Developer Pack? Never heard of it.
Real-world tools such as cloud hosting services, domain names, specific software can be expensive for students. That’s why GitHub created the Student Developer Pack in partnership with other tech companies to give students free access to the best developer tools in one place.
That’s great. What all is included in the Student Developer Pack?
Currently there are 23 services and tools included in the pack which can be used by a student. Some of the services are, 50$ Digital Ocean credits, a free Heroku Hobby Dev Dyno for two years, a free .me domain name, emailing via SendGrid with higher limits, private continuous integration builds through Travis CI and many more.
Awesome. How can I apply for it?
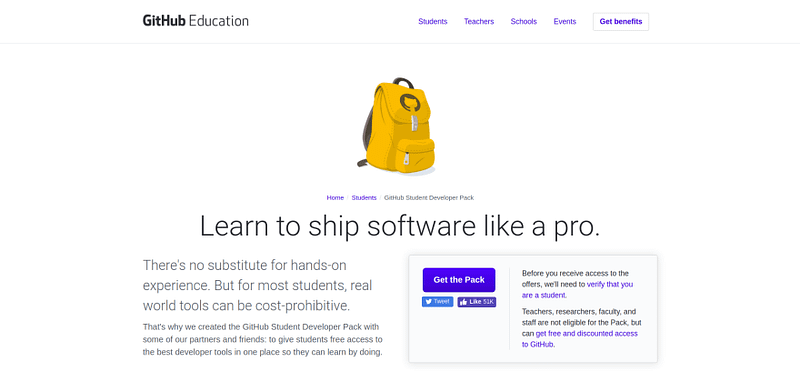
To apply for the GitHub Student Developer Pack, you need to be a current student. If you are not a student, then you aren’t eligible for the pack. Apply for the student developer pack at https://education.github.com/pack . Click on get the pack and follow the onscreen requests. If you do not have a school-issued email address that ends in .edu, you will also need a valid school ID, or other proof of enrollment like a picture of your schedule, which will be reviewed by the GitHub team. It takes 24 to 48 hours for the request to get reviewed.
A summary about what’s coming next
In this blog, we will be creating a Portfolio website using React and the tools provided by the GitHub Student Developer Pack. For this, we will be using the free Heroku dyno from the Pack and free Namecheap domain. We will also use GitHub to push our code and deploy it to GitHub pages.
Getting Started
Before we dive deep into the coding part, we will first install the required tools. I will be using the yarn package manager. You can find instructions for installing yarn at https://yarnpkg.com/lang/en/docs/install/
Install and create a react app named portfolio using the following command
2. Heroku CLI
Execute the following command to install Heroku CLI on Ubuntu 16+ OS.
Instruction for all other OS can be found at https://devcenter.heroku.com/articles/heroku-cli
Make sure to login to Heroku CLI using heroku login command.
3. Install gh-pages and fontawesome package using
Coding The Portfolio
We will be using Bootstrap 4’s resume template to build our portfolio. The template can be found here. https://github.com/BlackrockDigital/startbootstrap-resume
Copying jquery and bootstrap
Create directories css and js inside public folder and copy the following files to it from the downloaded template.
1. bootstrap.min.css
2. resume.min.css
3. bootstrap.bundle.min.js
4. jquery.easing.min.js
5. jquery.min.js
6. resume.min.js
Linking the added dependencies
Open the index.html inside the public directory and link the copied css and js as follows:
For Javascript
Adding template to React Component based structure
The Bootstrap resume template needs to be split into components. Create a directory Components in the src directory where all the components will reside. We will split it into the following 7 Components:
1. Sidebar.js
2. Landing.js
3. Experience.js
4. Education.js
5. Skills.js
6. Interests.js
7. Awards.js
Using json as a user data store
Create a json file inside the src directory with the name profileData.json . This file will hold the portfolio data for the user. The structure of json file is as follows:
Each key of the json is named for the component of the data that is going to be used.
Modifying App.js
App.js is the main file that imports all the other components and defines the structure of the website.
First, we import all the created components and the user’s data from the json created. In the constructor, we set the state for each component with its respective data from the json. This data from the state will be passed to components as props . All the components are then arranged per the Bootstrap’s template.
Creating Sidebar.js
The Sidebar component consists of the code for the sidebar of the website. This is the complete nav tag from the template. Before copying the code from the template, make sure to make it jsx compliant. class should be renamed to className or you can use this https://magic.reactjs.net/htmltojsx.htm to convert your HTML to JSX.
In the constructor of every component, the data from the props will be assigned to a variable through which it will be added to its relevant position in the jsx .
For the Sidebar component, this is done as this.sidebarData = props.sidebarData . It is done in a similar way for all the other components.
Replace all the hardcoded names and fields with the data from the JSON. To do this, reference your JSON data through the variable to which the props passed to the component are assigned. The syntax to reference a variable in JSX is referencing the variable within curly braces. So the first name of the user in the sidebar can be accessed as {this.sidebarData.firstName} . All other data fields can be accessed and replaced by the data from the JSON in a similar way.
Find Sidebar.js on GitHub at this link.
Creating Landing.js
Landing.js is created in a similar way. The first section with id about is the needed landing section HTML. First the data from the props are loaded in the constructor and added on to the jsx of the component. Find Landing.js on GitHub at this link.
Creating Skills.js
Skills of a user in the JSON data is a list of dictionaries. To add this to the jsx the loaded data from the props is looped over using a map and the data is inserted in the jsx .
where this.skills is the skills data of a user loaded from profileData.json
In a similar way that other components are created. Run the server using yarn start to see your portfolio at http://localhost:3000 in your browser.
Using GitHub Student Developer Pack
Using Namecheap to get free **.me** domain
Go to https://education.github.com/pack/offers and look for Namecheap. Get a free domain name by connecting your GitHub account on Namecheap.
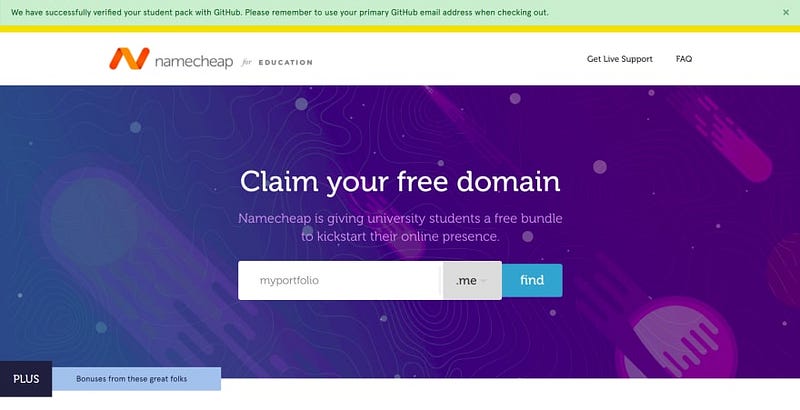
Authorize Namecheap and then find your free domain. After getting your domain, go to your domains list and click on manage to manage your domain.
Click on Advanced DNS tab and find Host Records . Click Add new Record button to add new records. Add the following records:
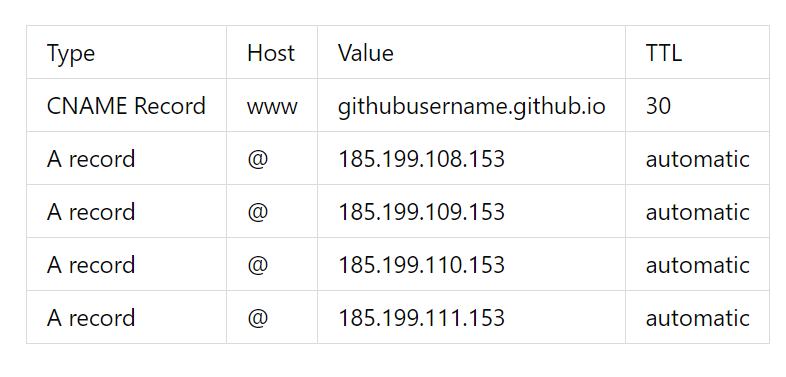
Replace githubusername.github.io with your actual GitHub username. After this, your domain is ready to be used with GitHub Pages.
Using GitHub to host your portfolio using GitHub pages.
In the root directory of your project, initialize a git repository as follows
git init . Head over to GitHub and create an empty repository with the name githubusername.github.io , here replace githubusername with your actual GitHub username. Copy the git link for your repo and add it to your local git repo as follows
git remote add origin <git link>
Create a file with name CNAME in the root of your directory and add your namecheap domain name to it, in the format yournamecheapdomain.me and save it.
Add 2 scripts predeploy and deploy to your scripts in package.json as follows.
Both the scripts should be inside the scripts key of package.json . Also add a homepage key to package.json and set it to http://yournamecheapdomain.me/
Run yarn run deploy to push your code to gh-pages branch and host it from it. Head over to http://yournamecheapdomain.me/ to see your hosted portfolio.
Push your code to master by doing the following steps:
Hosting your portfolio on Heroku
With the GitHub Student Developer Pack, you get a free Hobby Dev Dyno for Heroku. Headover to Heroku on https://education.github.com/pack/offers and connect your GitHub account with Heroku.
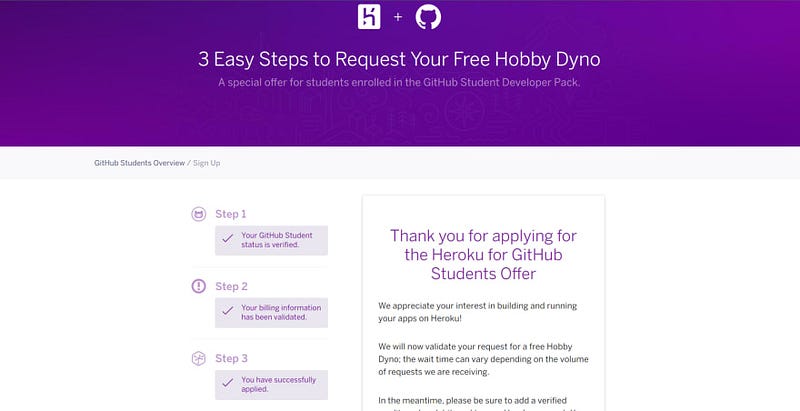
The process involves a manual verification by the Heroku team and may take a day or two to get approved. After the request gets approved, the app is ready to be deployed on your Heroku account with a Hobby Dev Dyno.
To deploy your app, make sure to login to Heroku CLI. Also, add a new object inside package.json as follows:
You can get your node version by running node -v and npm version by running npm -v in your terminal.
Create a Heroku app by executing heroku create appname where app name is your choice of app name for your portfolio. Commit your code if you haven’t yet and do git push heroku master . Wait for Heroku to complete the deployment.
Run heroku open to open your app hosted on Heroku.
That’s it, it’s as simple as that.
The complete source code for the app is on GitHub at DimuthuKasunWP/GitHub-Education-Portfolio

How to create your portfolio website using React.js

Dhruv Barochiya
We’ve moved to freeCodeCamp.org/news
After my friends canceled our weekend plans 😒, I was looking for something to kill time and finally ended up with a plan to create a portfolio website after going through my long pending list of ‘ Wish-To-Do ’ things.
Many hours of searching for technologies and templates later, I ended up creating this website using React.js and deploying it using Github pages. You can find the code for the website here (It’s called a ‘ web-app ’ technically, but for this article, I will be referring to it as a ‘website’ … I hope that’s ok 😜).
What you will learn
- Some basic concepts of React.js
- Create React-app from HTML website
- Deploy your portfolio website using ‘Github pages’
Some concepts you need to know before we start ..
Note — feel free to skip this part if you are already familiar with basic concepts of React.js and React Components. Note — these points will provide a very basic idea of the React world. I highly recommend you to study more about React from the documentation and get hands-on with the help of freeCodeCamp .
What is React.js >
For now, it is enough to know that React.js is the JavaScript library used for building UI components. It was created by the engineers of Facebook and nowadays, it is rocking the JavaScript world..
What is a React Component >
React lets you define components as a class or a function. You can provide optional inputs to the components called ‘ props ’.
Components let you split up the UI into independent sections like header, footer, and body. Each component will work independently so any individual component can be rendered independently into the ReactDOM without affecting the whole page.
It also comes with ‘lifecycle methods ’ which let you define pieces of code you want to execute according to the state of the component like mounting, rendering, updating and un-mounting.
React components come with their own trade-offs. For example, we can reuse a component by exporting it to other components, but sometimes it gets confusing to handle multiple components talking and triggering renders for each other.
this is how a component would look like!
What is GitHub Pages >
With GitHub Pages, you can easily deploy your site using GitHub for free and without the need to set up any infrastructure. They have provided modules so that you don’t have to worry about many things. If you stick around till the end you’ll see that it works like MAGIC!
Before you go ahead make sure to:
Decide what content you want to put up on your website.
Go through your latest resume once (if you don’t have one then create one now and postpone this project until next weekend 😛). It will help you to have a clear idea about what kind of information you want to put on your portfolio website.
Find inspiration
Browse through the hundreds of free portfolio website templates on the web, see how and what you can use from them — take out a pen and paper and sketch out a rough diagram to get an idea of what your website will look like. I will be using this template to demonstrate.
Gather some amazing pictures of yourself
It’s obvious that you don’t want to look bad on your own portfolio website. So dig into your archives of photos to find the perfect photos for your website.
Tune into your favourite playlist
Legend has it that good things come only with good music… and you surely don’t want to miss out any great things.
Let’s jump into the building part
In the following sections, I will describe steps to building the portfolio app but you don’t have to follow the same code I use. Focus on learning the concept and show some creativity! Further reading has been divided into three sections.
- Setting up the React-app
- Breaking down the HTML page into React components
- Deploying your app onto Github pages
Setting up React-app
We will be using create-react-ap p — a module provided by Facebook — which helps us to create React.js apps with ease and without worrying about build tools.
- Go to the console and run npm install create-react-app to install this module via npm (make sure that you have installed npm before using it — follow this link for more info).
- Now run npm create-react-app ${project-name} which will fetch build scripts and create a file-structure which will look like this.
Create a components folder under the src directory. This is where we will store our components in the future.
- Copy all the images, fonts, HTML and CSS files from the HTML template you decided to work with into the public folder.
Now your project directory should look like this.
- Run the npm install command which will install dependent modules under node_module directory.
- If you’ve got it right up until now, then running the npm start command will start the React app on the localhost . Go to https://localhost:3000 and you should be able to see the starter page of the React-app.
Breaking-down the HTML page into React components..
Remember the component folder which we created under src directory in the previous step, now we will break down the HTML template page into components and combine these components to make our React-app.
- First, you need to identify which components you can create from the monolithic HTML file — like header, footer and contact me. You need to be a little creative here!!
- Look for tags like section/div which aren’t nested into some other section/div . These should contain code about that particular section of the page which is independent of other sections. Try looking into my GitHub Repo to get a better idea about this one. Hint: Use the ‘ inspect element ’ tool to play around with the code and take notice of the effect of changes within the browser.
- These pieces of HTML code will be used in the render() method of the component. The render() method will return this code whenever a component gets rendered into the ReactDOM. Take a look at the code blocks given below for reference.
Hint: If things are getting confusing on the react side — try focusing on the concept of ‘how to identify wanna be components from the HTML codebase’. After getting comfortable with React, implementation will be a piece of cake.
Did you notice that there are some changes in the HTML code? class became className . These changes are required because React doesn’t support HTML 😅 — they have come up with their own HTML-like JS syntax which is called JSX . So, we need to change some parts of the HTML code to make it JSX.
I bumped into this HTML to JSX converter during this project, which converts HTML code into JSX for you 😯. I highly recommend using this rather than changing your code manually.
After some time, you should come up with some different components. Now the EndGame is near!! Combine these different components under one App.js component (YES!! You can render one component from another component!) and your portfolio app will be ready.
Notice in the above code that we need to first import the components in order to use them in the render() section. And we can use the components just by adding <component-name></component-name> or just <component-name/> tag in the render method.
- Run npm start from your terminal and you should be able to see the changes reflected in the website. You don’t need to run this command again if you have made more changes in the code, it will be reflected automatically when you save those changes. You can do some lightning fast development thanks to the hot reload feature .
- Play around with the HTML and CSS to change the content according to your resume and make your portfolio even cooler by changing the content, trying out different fonts, changing the colours and adding photos of your choice.
Deploy React-app to Github pages
Okay, so you survived until this point… take a moment to appreciate your hard work. But you still need to complete your deployment so that you can share your cool work with your friends who ditched those weekend plans.
- First, you need to install the npm library of Github pages. To install, run this command npm install gh-pages on your terminal.
Now, you need to make the following changes in your manifest.json file:
- Add the homepage field — value will be in the following format — https://{github_id}.github.io/{github_repo}
- Add predeploy and deploy fields under scripts
Now your manifest.json should look like this:
Now go to the terminal, run npm run deploy and wait for the magic!! Your app will be deployed after the deployment scripts execute successfully. Verify whether your app has deployed or not by visiting the link you provided in the homepage field.
Caution: Please be careful when deploying anything onto the web. Perform safety checks like removing internal links, passwords, or anything that you don’t want to be there in the hands of smart people out there.
Homework for you
Congratulations! You have finally created and deployed your portfolio app. If you are interested, then you can add these features to your website
- Blog feature: create your own blog using Node.js and a NoSQL database like MongoDB and merge it into this portfolio website.
- Gallery: add a section to the page where you can show the screenplay of the recent photos from your social media websites.
- Twitter Feed: add a section showing recent tweets by you.
- Random Quote: add a section showing some random motivational quotes.
If you implement any of these features, share your work with me. I would be more than happy to help 😃 ( if I can 🐵)
Have your project? — Share it
I am trying to build a community of developers where people can share their ideas, knowledge, work with others and find other people with similar ideology to build things together.
- Gitter channel: https://gitter.im/weekend-devs/community
- Github Organization: https://github.com/weekend-developers
Wrapping up
I would like to take a moment to acknowledge the work of the people who gave me the inspiration and knowledge to complete this article.
- Quincy Larson, Sahat Yalkabov & community: For creating freeCodeCamp — the platform where you can learn and gain knowledge about almost everything related to web technologies; using hands-on tutorials and all without paying fees. 😍
- Colorlib: for providing state of the art templates which were a huge inspiration for my portfolio website. 😸
- Daniel Lo Nigro & community: for creating HTML to JSX Compiler, which turned out to be handy while converting HTML blocks into JSX code. 😋
- My dearest friends: who helped me in correcting my mistakes.
- YOU: for sticking around, I hope you had a productive time. Keep exploring and building amazing things!
Some rights reserved
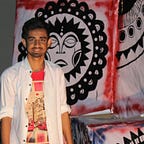
Written by Dhruv Barochiya
Just another curious random guy who happens to enjoy a writing a bit, you can find more stuff here: https://dhruvbarochiya.com
Text to speech
ReactPortfolio
React portfolio, multiple pages with dark mode, a portfolio for developers.
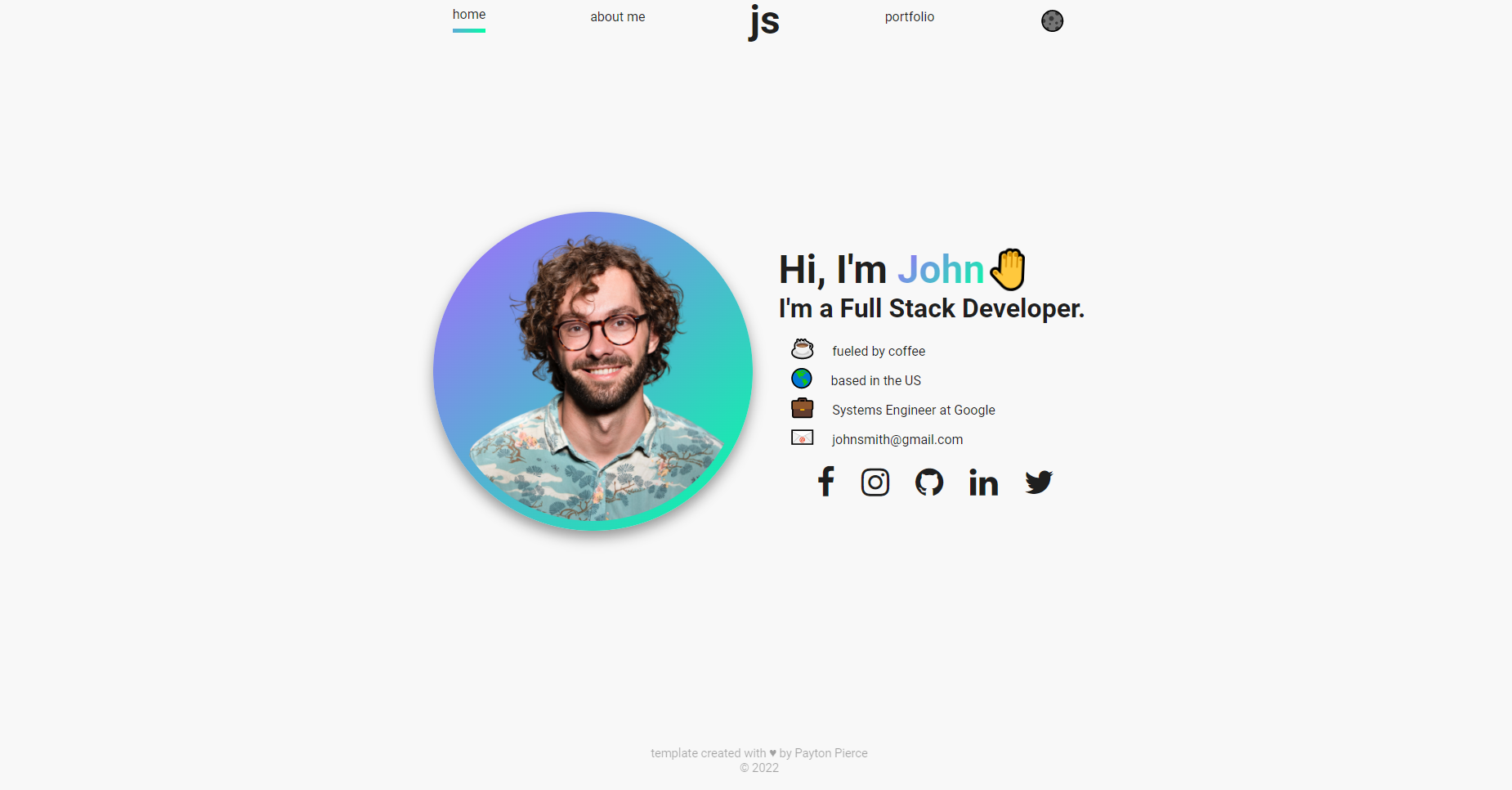
🌑 Dark Mode Toggler
📖 Multi-Page Layout
📱 Fully Responsive
🎨 Modern Design
💡 Perfect Lighthouse Score
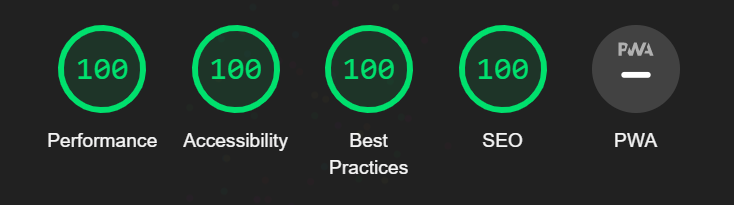
getting started
Prerequisites.
- have Git installed on your machine
- have Node.js installed on your machine
- basic familiarity with your machine’s command line
- basic understanding of JSON data outline (arrays of objects basically)
- fork the repository and clone locally
- run npm install to install dependencies
- once installation is complete, run npm run start to get your local copy running in the browser.
template instructions
1. replace the images.
- open the project in your file explorer, and navigate to src/img . there, you will see a bunch of images. these need to be replaced with your own images.
- add an image of yourself with the background removed, and cropped into a square. i know this seems picky, but the template is set up in a way that this is how it will look best :) I recommend using https://remove.bg to remove the background from your image. Once you add it to this img folder, rename it to self.png (delete the old self.png so yours replaces it)
- if background removal is not an option for you, any image you use will automatically be made into a circle. for this reason, square images without too tall of an aspect-ratio work best.
- example with background removed:
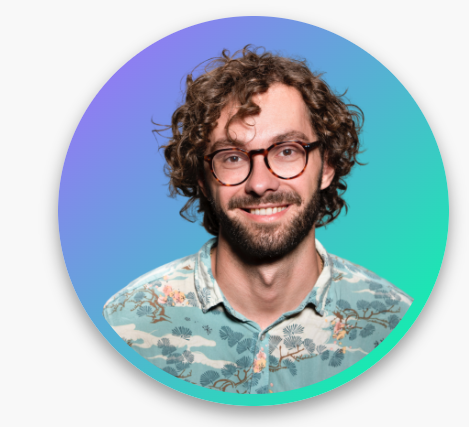
- example with background not removed:
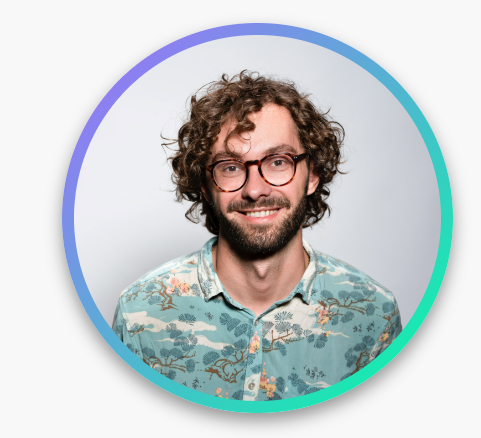
- add to the img folder up to 5 screenshots of projects you have completed to be added to your portfolio page. this can either be a regular screenshot, or you can create mockups like what are on the default template. I created these in Canva – if you would like help with creating them for your own projects, feel free to message me on Twitter and I will happily help you make them :)
- to make life easier later, name these files mock1.png , mock2.png , etc.
2. edit Info.js
Next, open the project in your code editor. Navigate to src/info/Info.js . There are some instructions written in the comments there, but I will go over it all here as well:
Color Scheme
near the top of the file, you will find a line that looks like this:
There are the colors being used to determine the “accents” throughout the site - the circle behind your self portrait, the color of your name, the labels on the about page, and the underline for the navigation.
You can pick any colors you like for this, just play around with it and make sure to check the colors against both light mode and dark mode to make sure it looks good on both :)
Your Information
going further down the Info.js file, you can start replacing the default information with your own information, such as first name, last name, initials, position, etc. Some of the values in this file should remain untouched – be sure to read the comments to see what to edit.
if you’re not sure how to get emojis for the miniBio and hobbies, on Mac you should be able to access the emoji keyboard with ctrl+cmd+space, and on Windows with windows+period(‘.’)
Otherwise, use Emojipedia to find and copy-paste what you like.
For the ‘socials’ section, be sure to replace the links with your own social profile links.
For the ‘portfolio’ section, update the titles to whatever you want. The live link should be a link to the live demo of your project. The source link should be a link to the repository where the code of the project is hosted , such as GitHub.
Note: If you have more than 5 projects, you will need to add more objects to the portfolio array. Just copy from the opening bracket to the comma of one object and continue pasting as many as you need. If you need help with this, feel free to reach out.
And that’s it! If you save the file and check your live server, you should see that the information has been updated and the portfolio has been customized.
Now, you can commit and push your changes to your forked repository, and deploy however you like.
And you’re done!
If you have any questions or issues with the setup process, feel free to contact me by any of the means below:
[email protected] |
Or, use this repo and post an “issue” :)
All that I ask is that you please credit me for the template :) If you’d like to remove the credit from the bottom of the site, go for it, just link my profile somewhere in your ReadMe when you deploy, or wherever.
If you enjoyed this template and want to support my ability to make more of these, as well as creating video tutorials about React projects, writing articles about helpful developer tools, or you just want to be nice, feel free to support me on Ko-Fi !
If you have any suggestions for how to improve this template, the ReadMe guide, etc. please don’t hesitate to reach out – I will always happily accept constructive feedback!
examples of people using this template!
24 Best Free, Open Source React Portfolio Page Templates 2024
React portfolio page.
Creating a standout portfolio website is essential for showcasing your work and skills as a developer. With the help of React portfolio templates, you can easily create a dynamic and responsive portfolio that leaves a lasting impression on visitors.
Below, we've curated a list of the top 24 free and open-source React portfolio page templates available in 2024.
Each of these templates offers unique features and designs, allowing you to customize your portfolio website to suit your style and preferences.
Whether you're a developer, designer, or creative professional, these React portfolio templates will help you create a stunning online presence to showcase your work effectively.
1.open-react-template
open-react-template is a free React / Next.js landing page template meticulously crafted with Tailwind CSS. Ideal for developers and makers seeking to create polished landing pages swiftly, Open is perfect for showcasing open source projects, SaaS products, online services, and more. Elevate your project's online presence with Open's professional design and seamless integration with React and Next.js.
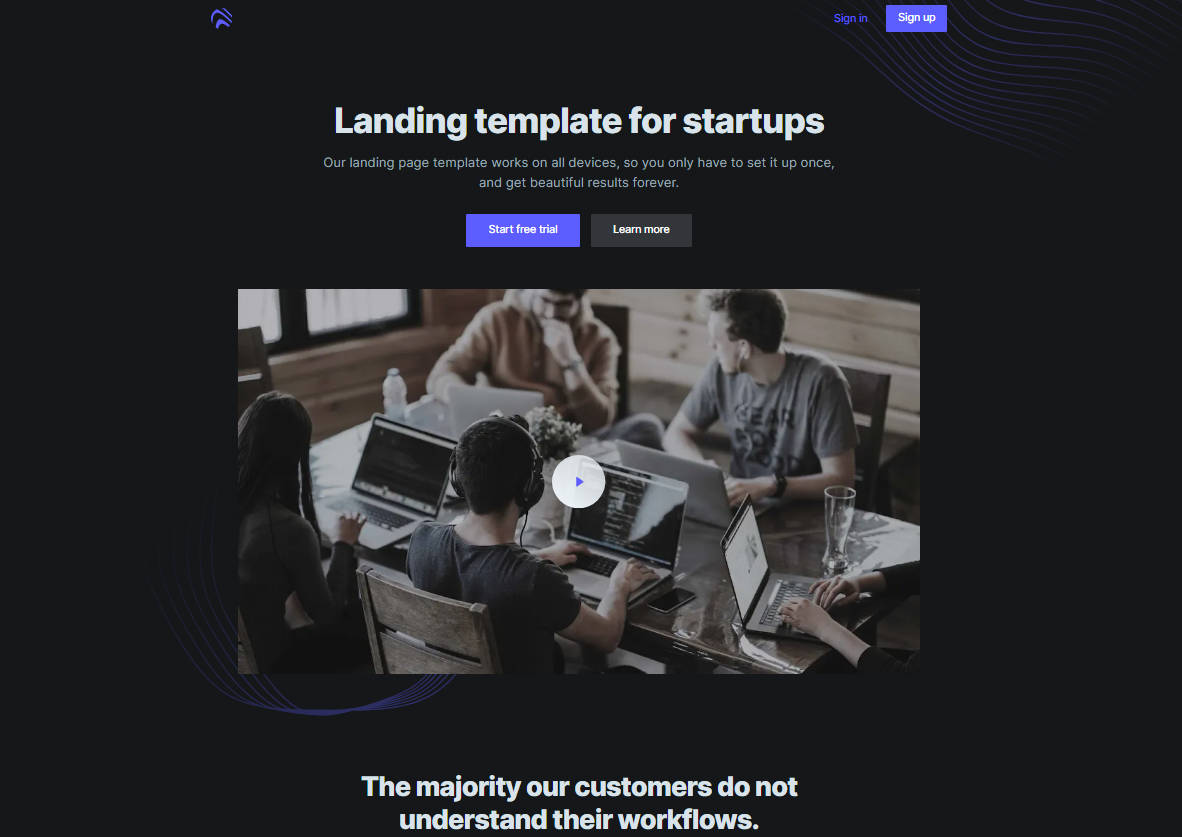
2.soumyajit
Soumyajit Behera's portfolio template, Built with React.js, Node.js, and Express.js, this stunning website showcases the versatility of modern web development technologies. With its multi-page layout and fully responsive design, Portfolio Website - v2.0 ensures that your work shines on any device. Styled with React-Bootstrap and CSS for easy customization.
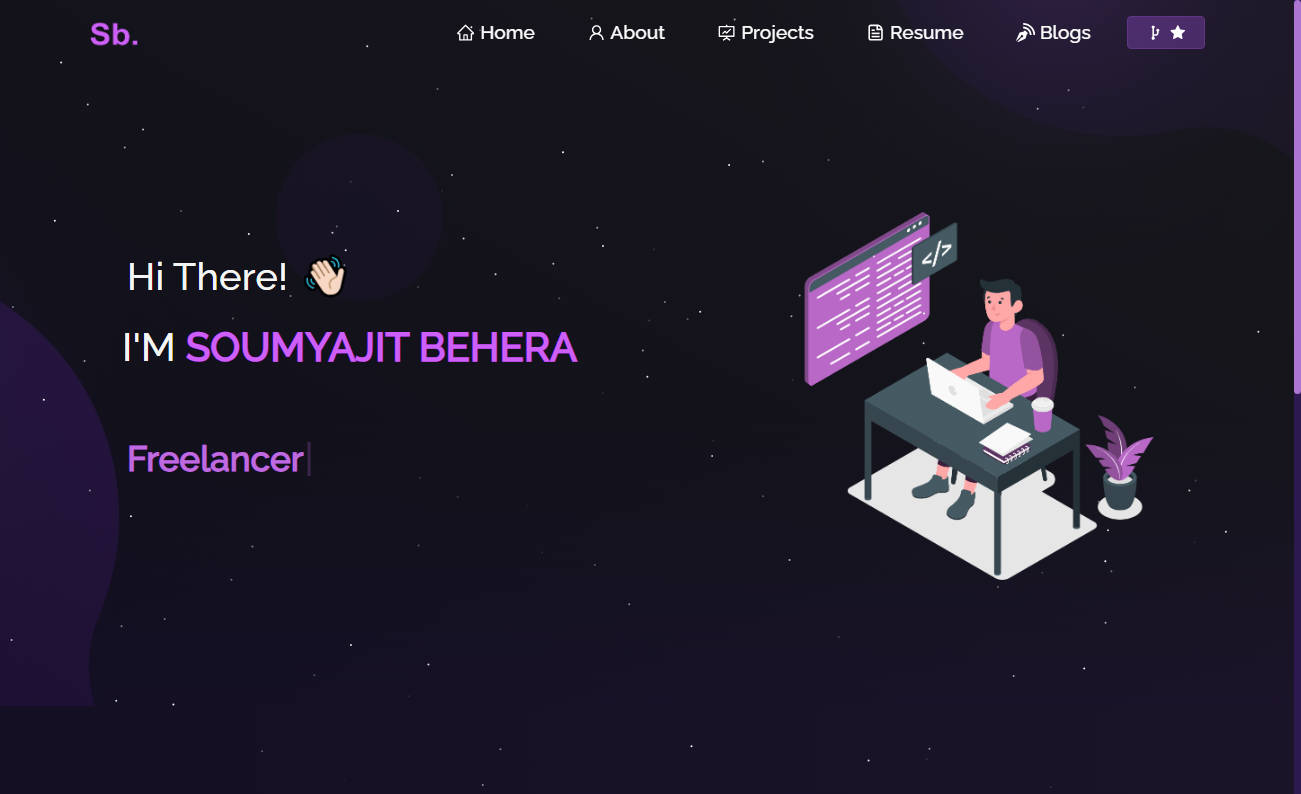
Discover simplicity and elegance with Chetan React Portfolio Template! Crafted by Chetan Verma, this modern portfolio template is powered by Next.js and TailwindCSS, offering a clean and sleek design ideal for showcasing your skills and projects. With its responsive layout, your portfolio will look stunning on any device, ensuring a seamless browsing experience. Enjoy easy customization of your details through a user-friendly GUI and leverage features like Blog CRUD and Dark Mode to elevate your portfolio presentation.
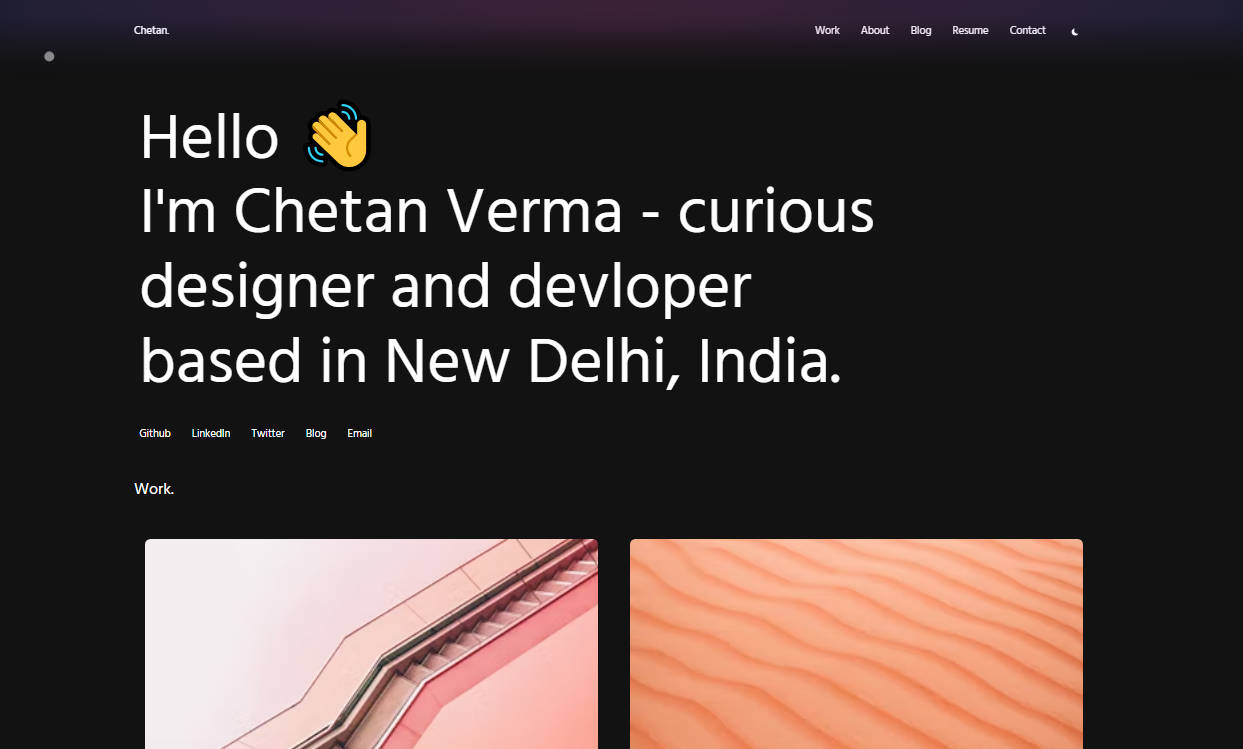
4.Simple Portfolio Template
Elevate your portfolio presentation with Ubaida Mutlaq's Simple Portfolio Template! Designed for developers and designers, this template harnesses the capabilities of React.js and Bootstrap to provide a straightforward yet visually appealing portfolio solution. With its fully responsive design and multi-page layout, your work will shine across all devices. Enjoy features like a contact form with EmailJs and the convenience of editing content from one centralized location.
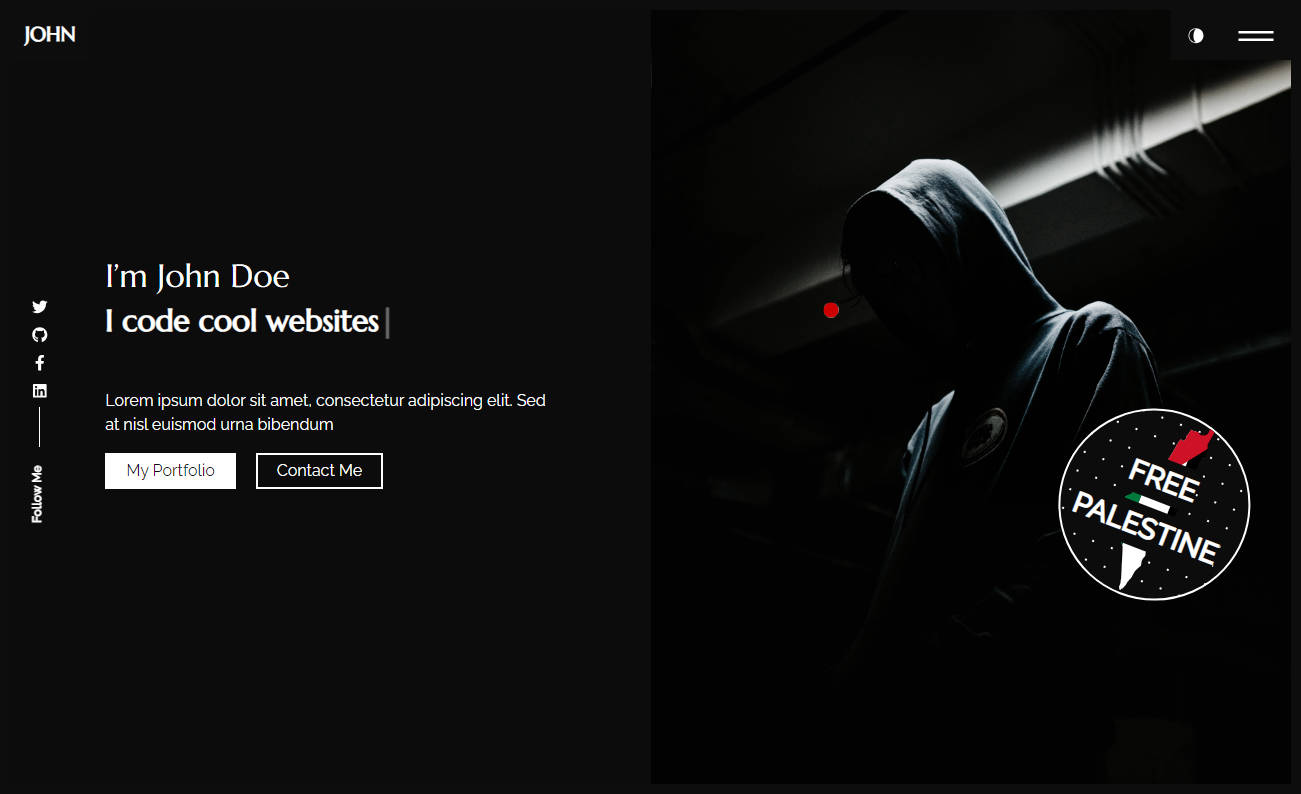
5.GitProfile
Unlock the potential of your GitHub profile with GitProfile! This powerful portfolio builder empowers you to create a stunning and personalized portfolio site within minutes, regardless of your coding experience. By simply providing your GitHub username, GitProfile automatically generates a professional portfolio showcasing your projects and contributions. With seamless deployment to GitHub Pages, your portfolio becomes instantly accessible to the world.
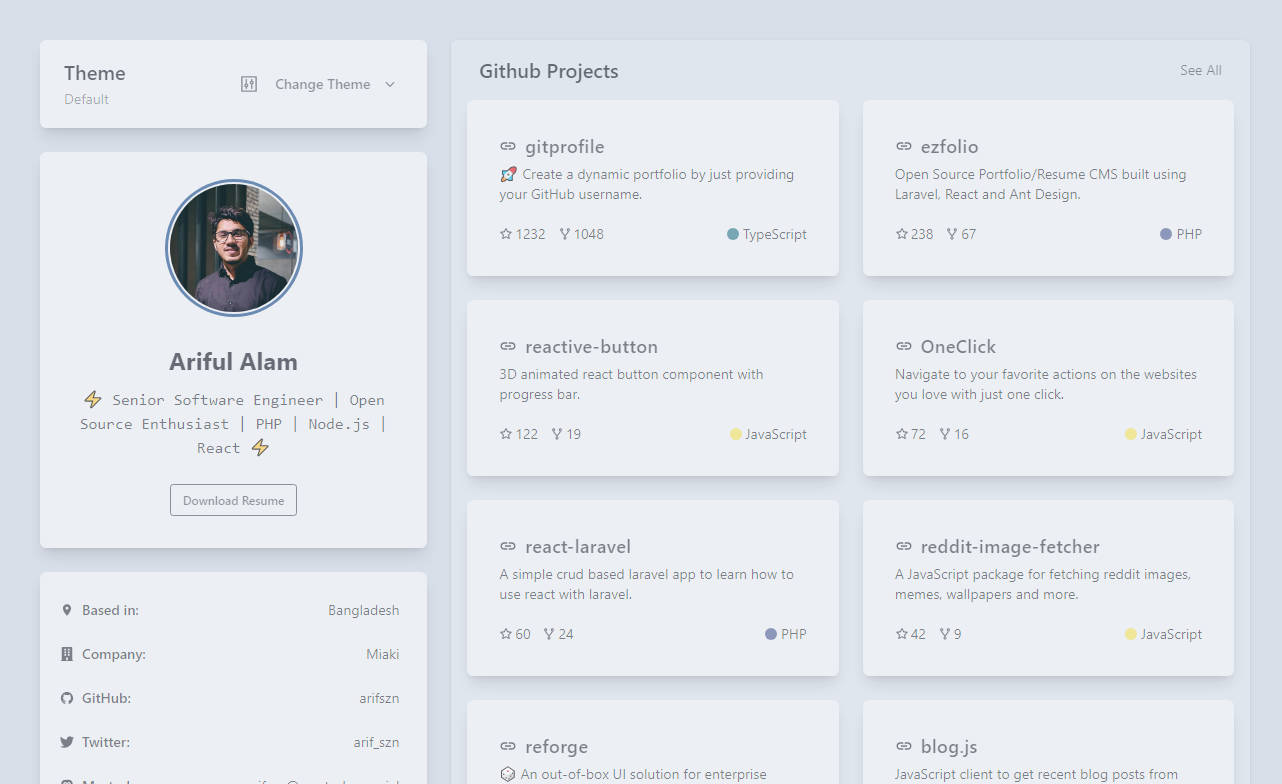
6.React Portfolio 2
Explore the latest in web development with React Portfolio 2! This project, built with React.js and Tailwind CSS, offers a comprehensive learning experience covering essential topics such as React.js hooks, folder structure, Tailwind CSS, Swiper.js, React Icons, React packages like React-Modal, Email.js, and AOS scroll animation.
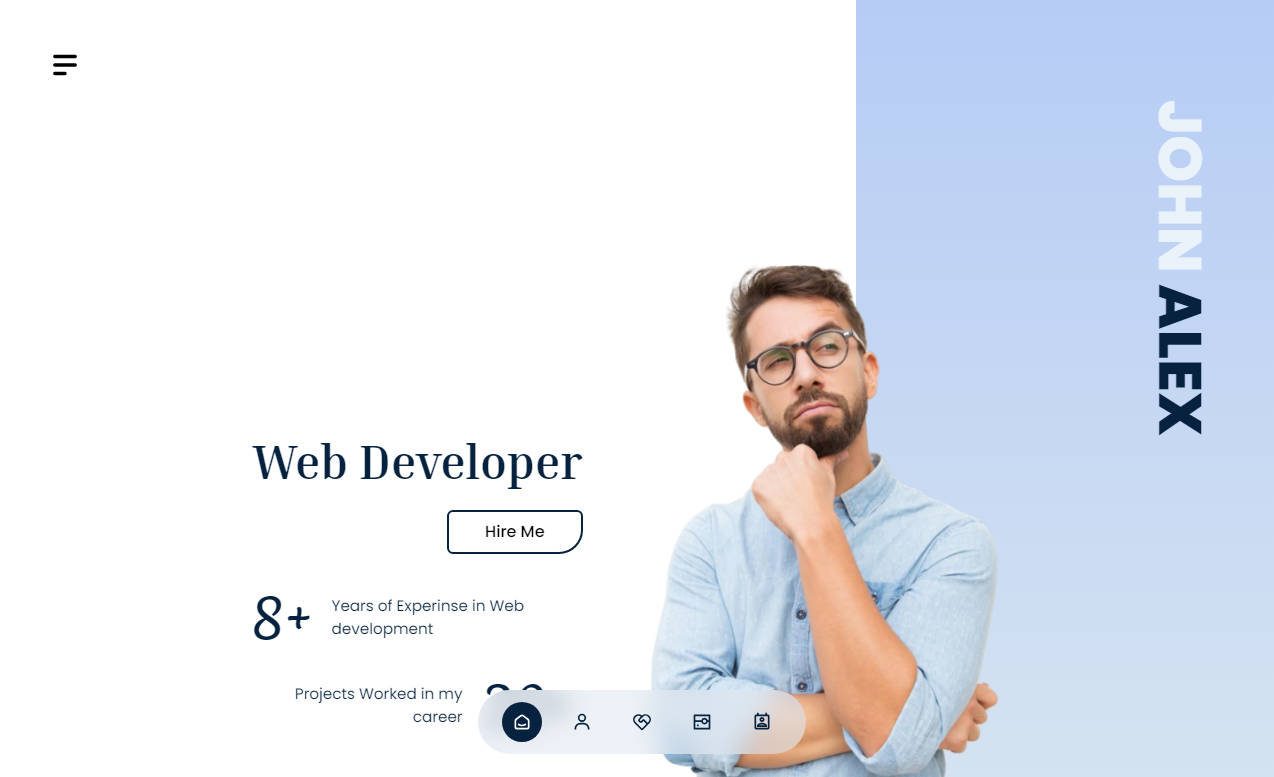
7.Cleanfolio
Elevate your portfolio presentation with Cleanfolio, a sleek and minimalist template crafted by Raj Shekhar. Powered by React.js, Cleanfolio puts your work front and center with its clean design. Whether viewed on a desktop or mobile device, Cleanfolio's responsive layout ensures your portfolio maintains its polished appearance across all screens. Showcase your projects effortlessly and let your work speak for itself with Cleanfolio.
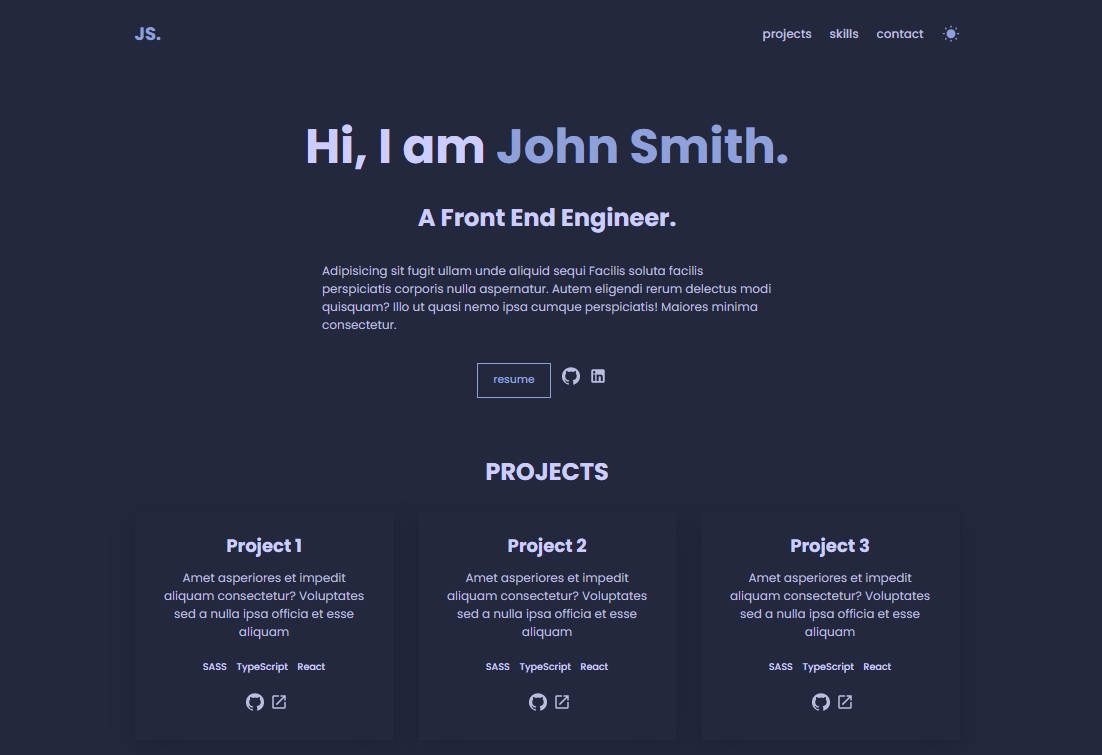
8.shaq portfolio
Unleash the potential of your portfolio with Shaquille Ndunda's creation! Originally designed for personal use, this portfolio template is now available as an open-source resource on GitHub. Utilizing React.js, Three.js, Framer Motion, and TailwindCSS, this template showcases skills, experience, and accomplishments in a visually appealing and professional manner. Customize and modify the theme and components to reflect your personal style and branding preferences. Explore, adapt, and create your own standout portfolio with this versatile template.
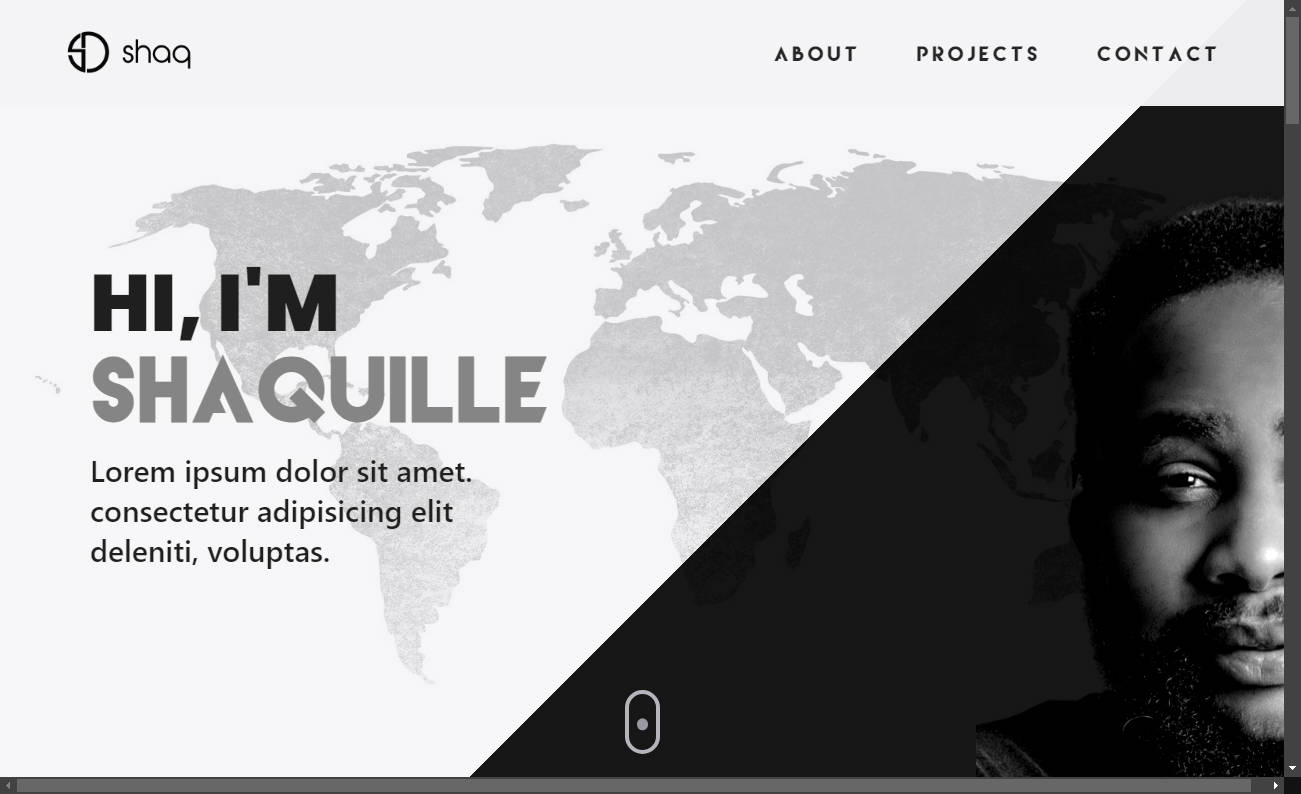
9.developer-portfolio
Introducing Hanzla's Software Developer Portfolio Template! Built with Next.js, axios, and Reactstrap, this template is tailored for software developers seeking to showcase their skills and projects effectively. With its responsive design, your portfolio will shine on any device, ensuring a lasting impression on potential clients and employers. Explore the versatility of this template and elevate your portfolio presentation as a software developer.
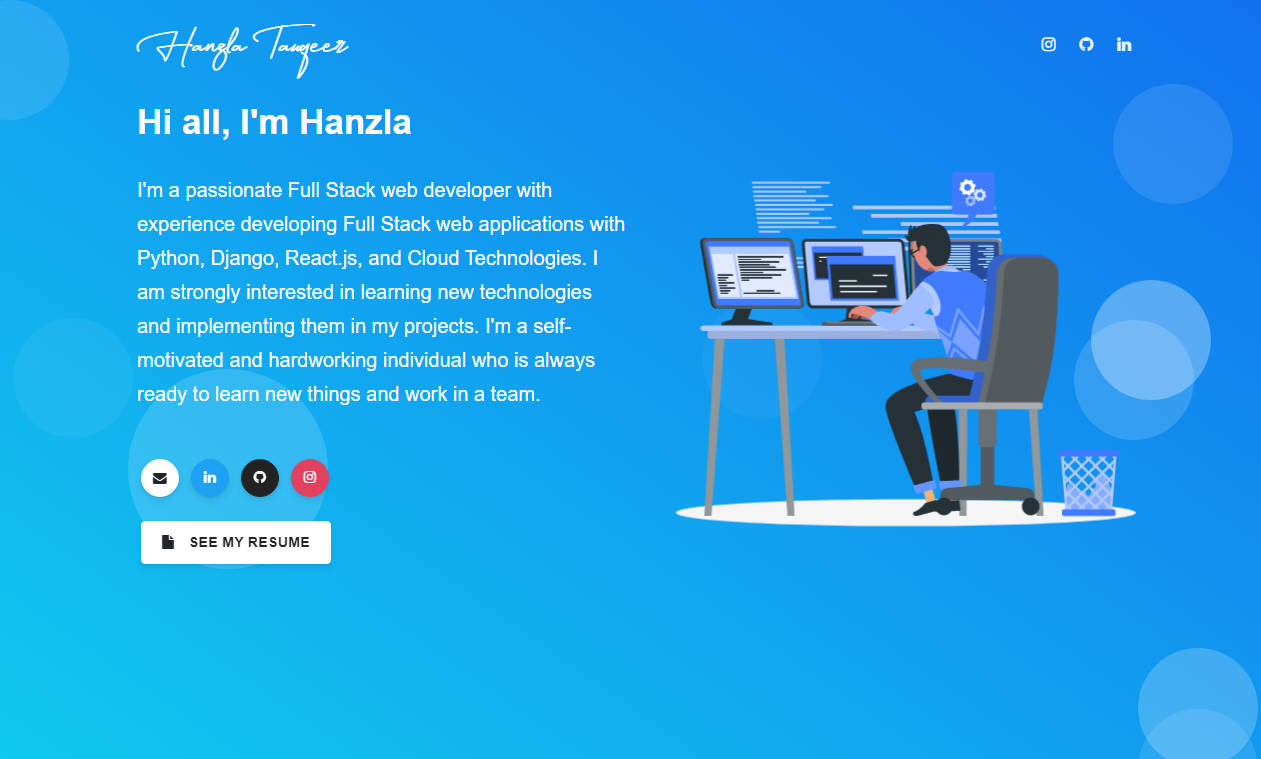
10.react-portfolio-final
Elevate your portfolio presentation with CodeBucks' Portfolio template! Powered by React JS and enhanced with framer-motion for stunning transitions and animations, this template offers a versatile solution for creating an impressive portfolio quickly. With its responsive design, your portfolio will captivate viewers across all devices, leaving a lasting impression.
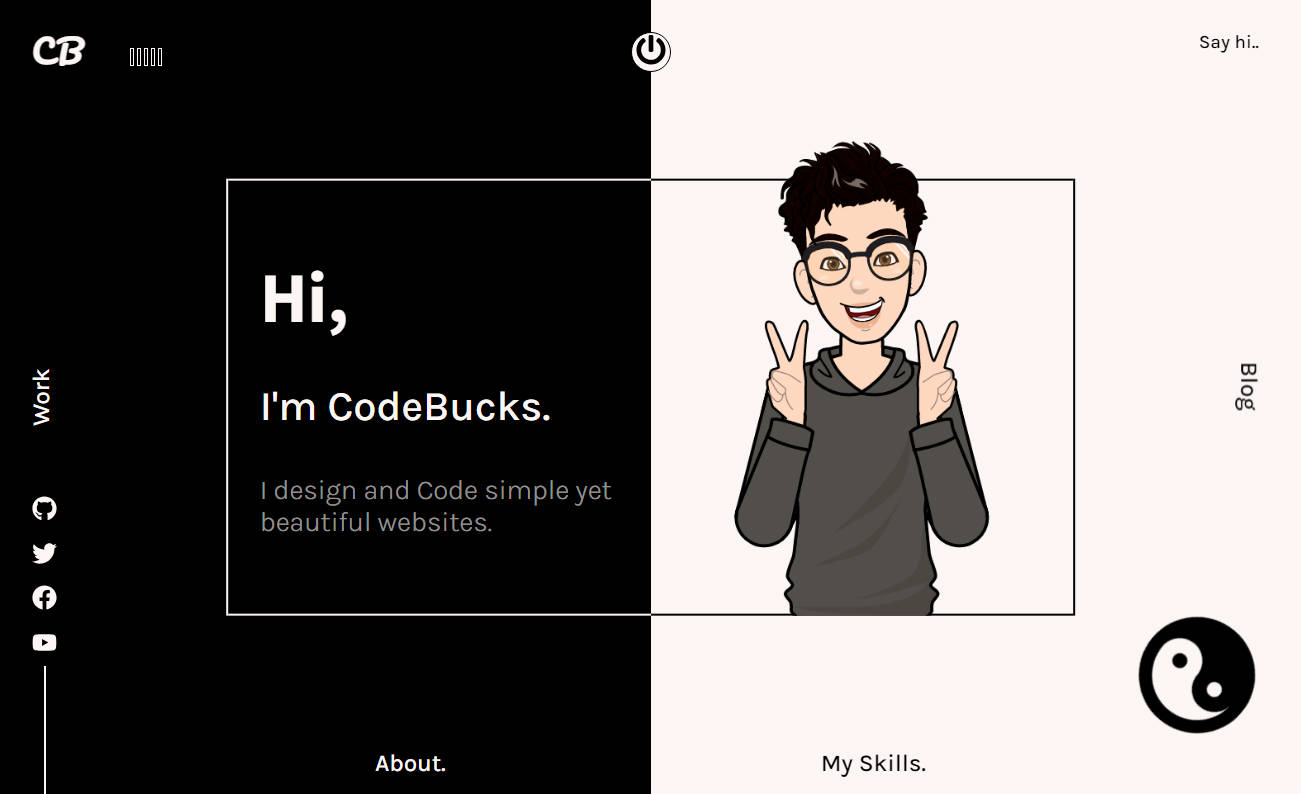
11.Prasad Chavan's Personal Portfolio
Prasad Chavan's Personal Portfolio and Blog template, Crafted with a focus on responsiveness and functionality, this template offers sections for showcasing personal information, resume, projects, and contact details. Leveraging the power of Next.js for dynamic routing and SEO optimization, this template ensures your portfolio and blog rank high on search engines. With a technology stack including React JS, Next JS, MarkDown, HTML/CSS/JS, Tailwind CSS, and Node JS, this template is your go-to choice for building a standout personal portfolio and blog.
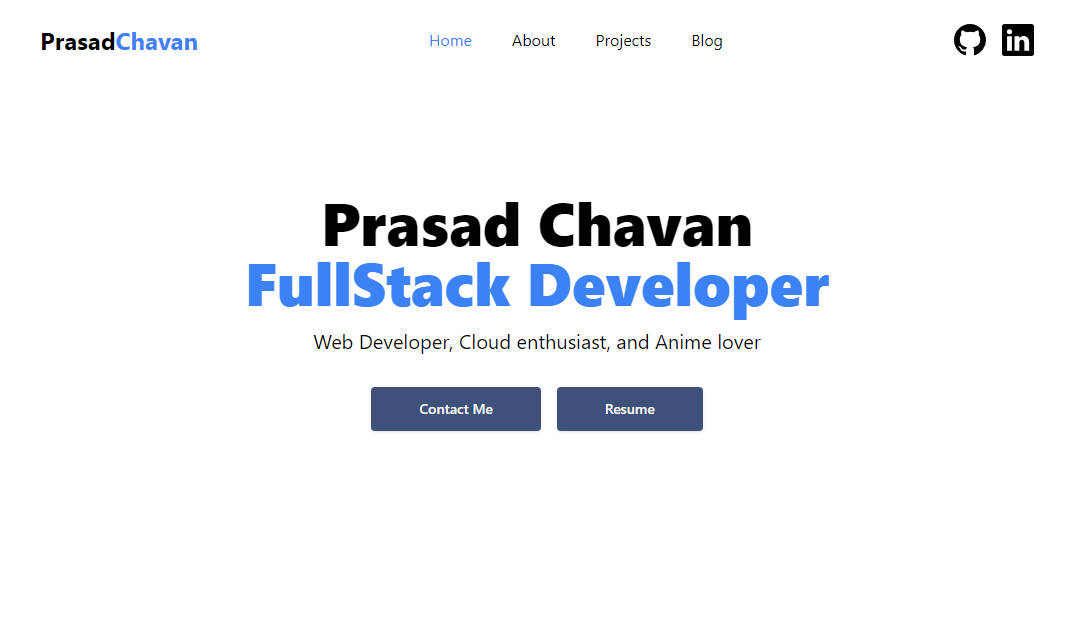
12.seek's portfolio v5
Explore the fifth iteration of seek's portfolio website! Built with Next.js, React, and Tailwind CSS, this iteration showcases a simplistic glass-morphism-like design, offering a modern and visually appealing user experience. seek's goal was to experiment with design concepts while deepening their knowledge of React and Next.js.
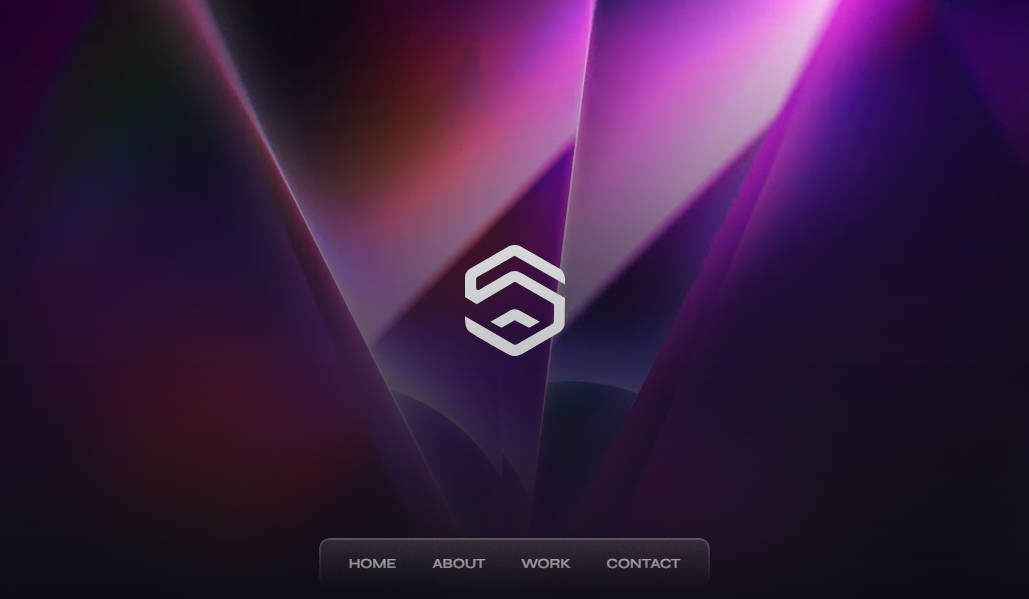
13.portable-portfolio
Elevate your portfolio presentation with the Portfolio template using React.js and Chakra UI! This versatile template empowers you to swiftly create and customize your portfolio website using markdown files. With the power of React.js and the sleek design elements of Chakra UI, your portfolio will stand out with a modern and professional look. Showcase your projects, skills, and achievements effortlessly with this customizable portfolio template.
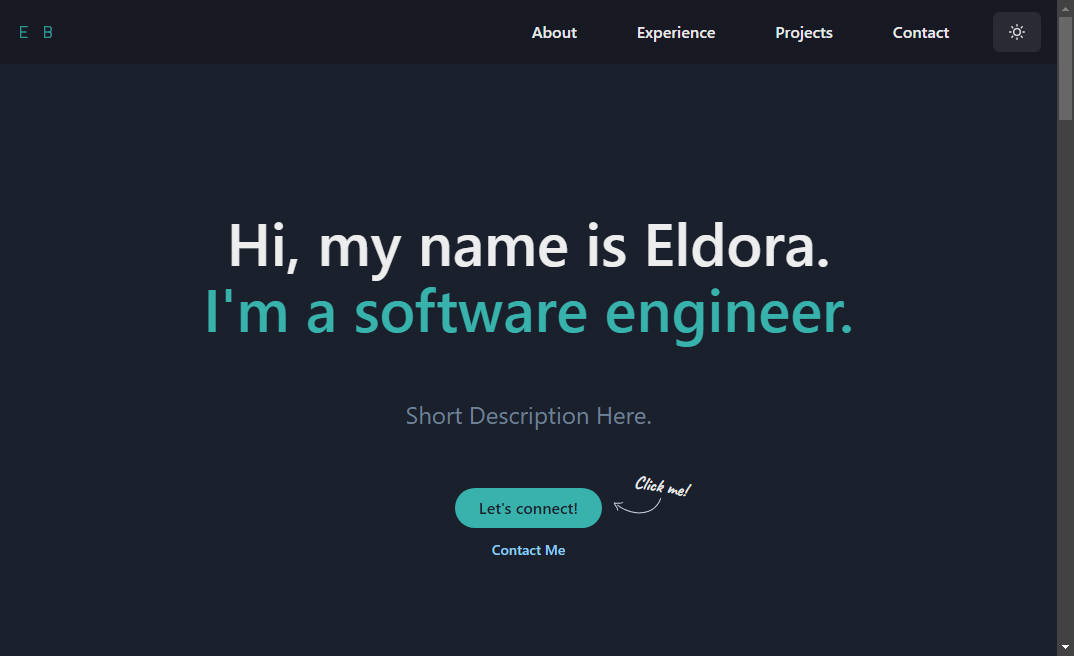
14.playground-macos
Immerse yourself in Xiaohan Zou's Playground-macOS, a unique portfolio website that simulates macOS's GUI. Developed with React and UnoCSS, this innovative project offers a captivating user experience reminiscent of macOS. Explore Xiaohan Zou's portfolio and projects within a familiar environment, providing both functionality and aesthetic appeal.
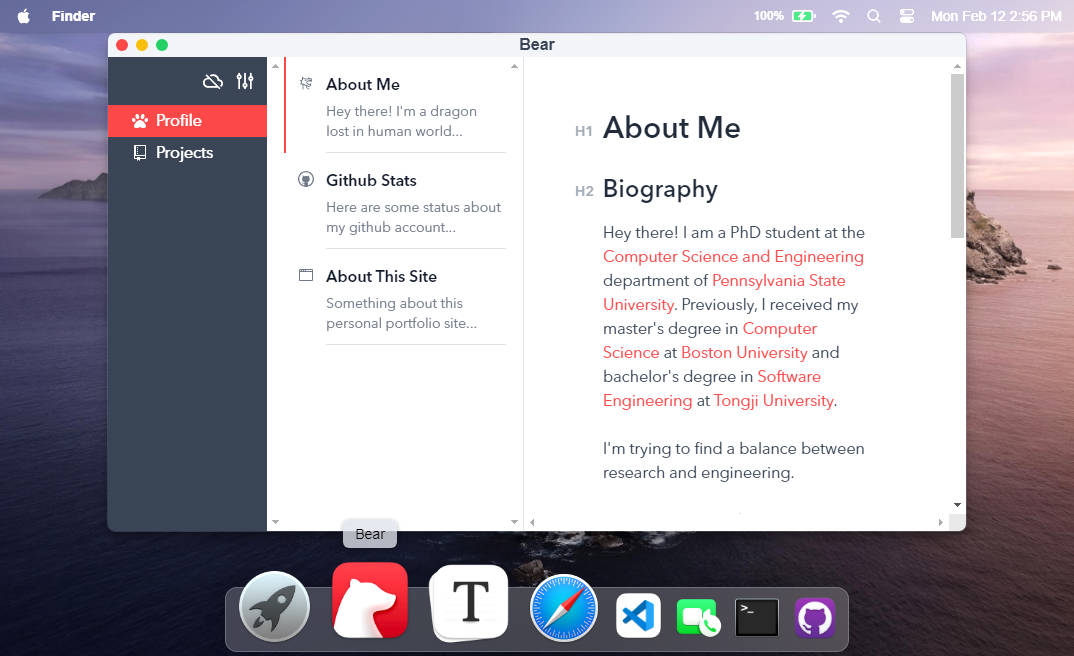
15.react-portfolio
Unlock your potential with Mark Christian Tan's portfolio template! Designed for fellow developers, this clean and customizable ReactJS template offers a sleek platform to showcase your skills and projects. Built using React and bootstrapped with Create React App, it's enhanced with the stylish components of Material-UI. Whether you're a seasoned developer or just starting out, this template, deployed on Vercel, provides an ideal canvas to present your work to the world.
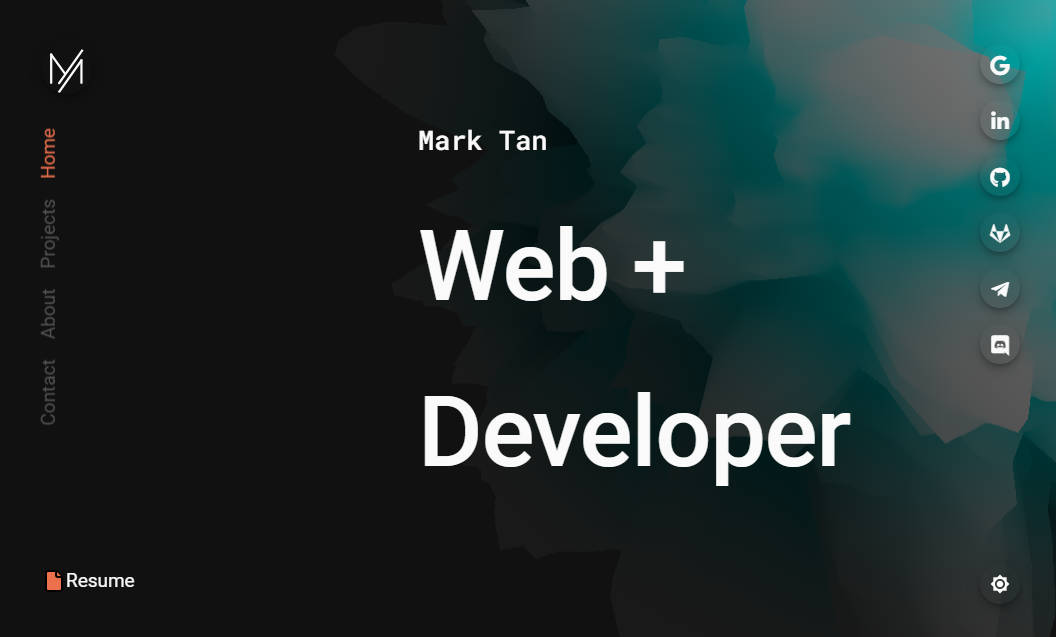
16.react-tailwindcss-portfolio
This sleek and versatile starter theme offers a plethora of features, including React v18 with React Router v6, Tailwind CSS v3, Context API for State Management, and Framer Motion transitions & animations. With reusable components, dynamic forms, and a responsive design, this template provides everything you need to create a stunning portfolio website.
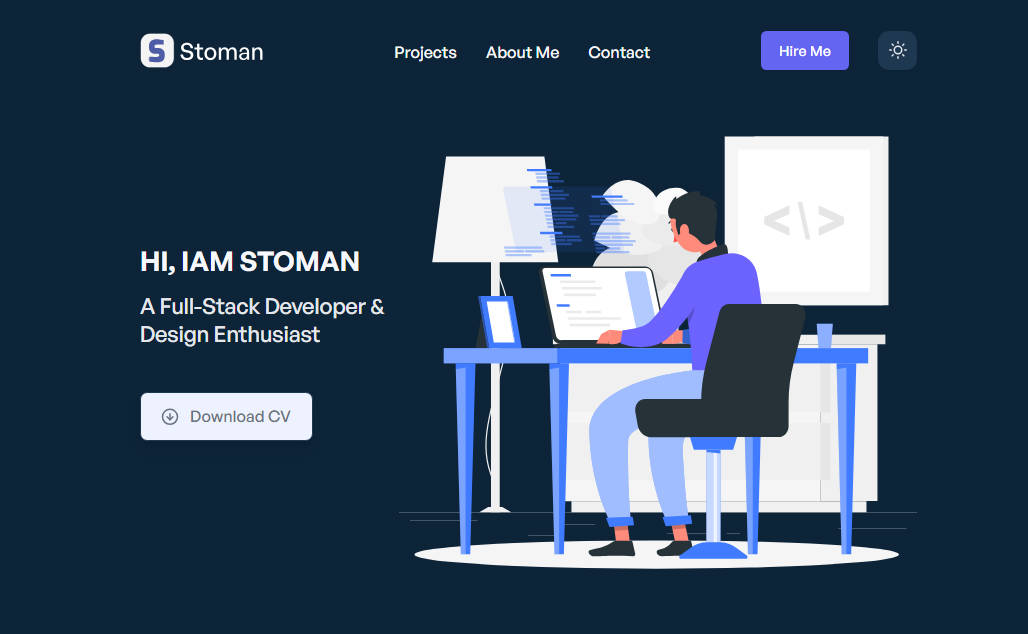
17.Rahul Vijay portfolio
This visually stunning website, entirely crafted by Rahul Vijay, showcases his talents in web development. With multiple pages enabled by React Router v6, users can easily navigate through various aspects of Rahul's skills and projects. The website's fully responsive design ensures a seamless experience across all devices, while its modern design, enhanced with animations, captivates visitors and leaves a lasting impression.
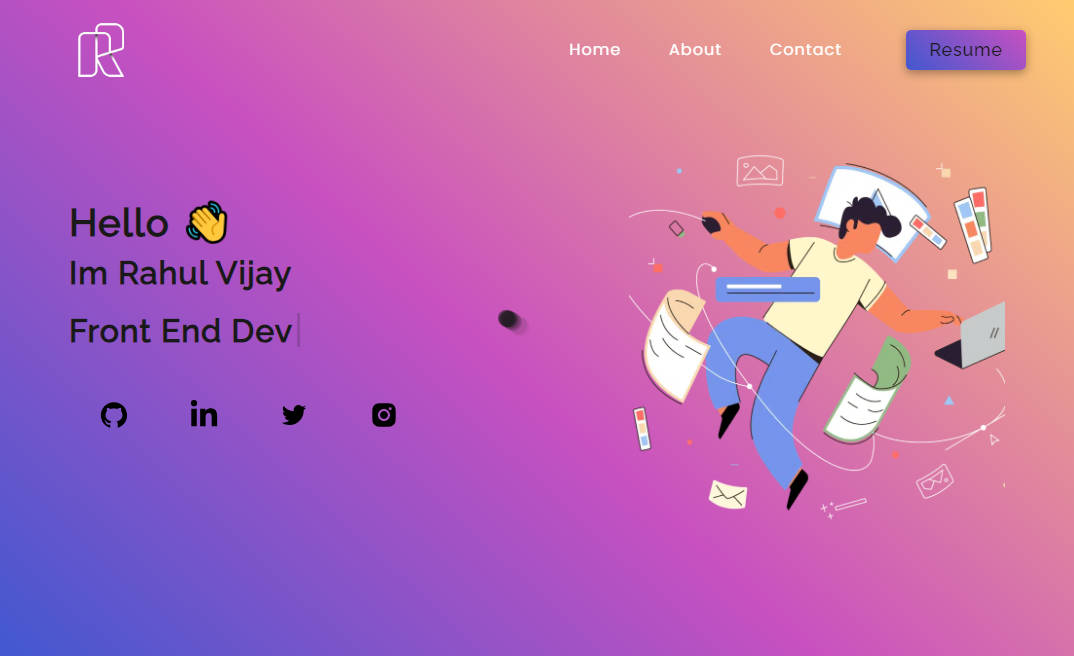
18.3D Portfolio
Elevate your portfolio presentation with 3D Portfolio, a meticulously crafted website built with React.js and Three.js. This fully functional and visually stunning portfolio showcases your work in a dynamic and immersive 3D environment. With its responsive design, 3D Portfolio ensures a seamless experience across all devices, allowing your projects to shine on desktops, tablets, and smartphones alike.
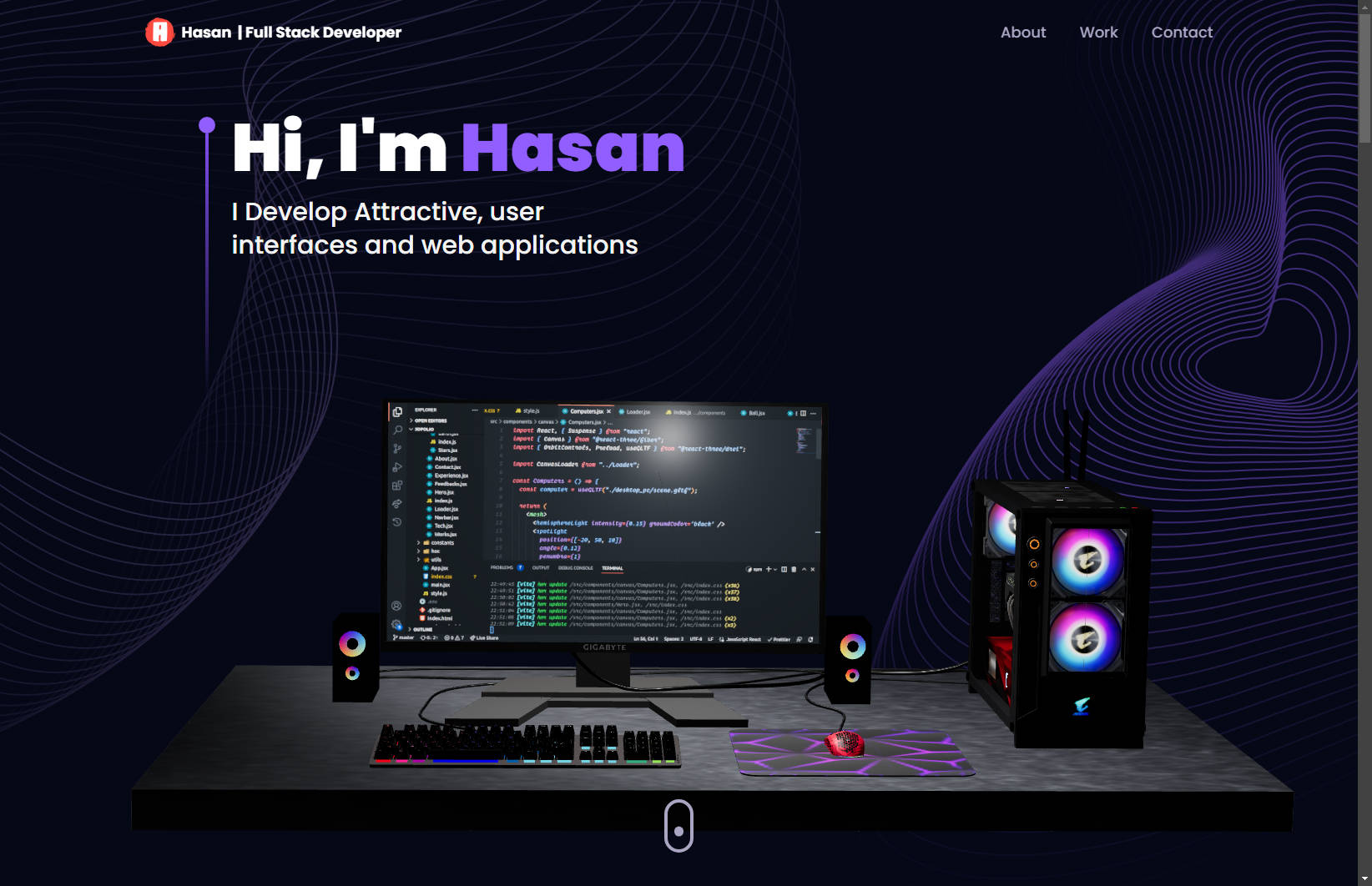
19.Developer Portfolio
Developer Portfolio is a versatile web template designed specifically for developers to showcase their skills and projects. Built with Next.js, this template offers a modern and customizable platform for developers to present themselves professionally. Whether you're a seasoned developer or just starting out, Developer Portfolio provides the tools you need to create an impressive online presence.
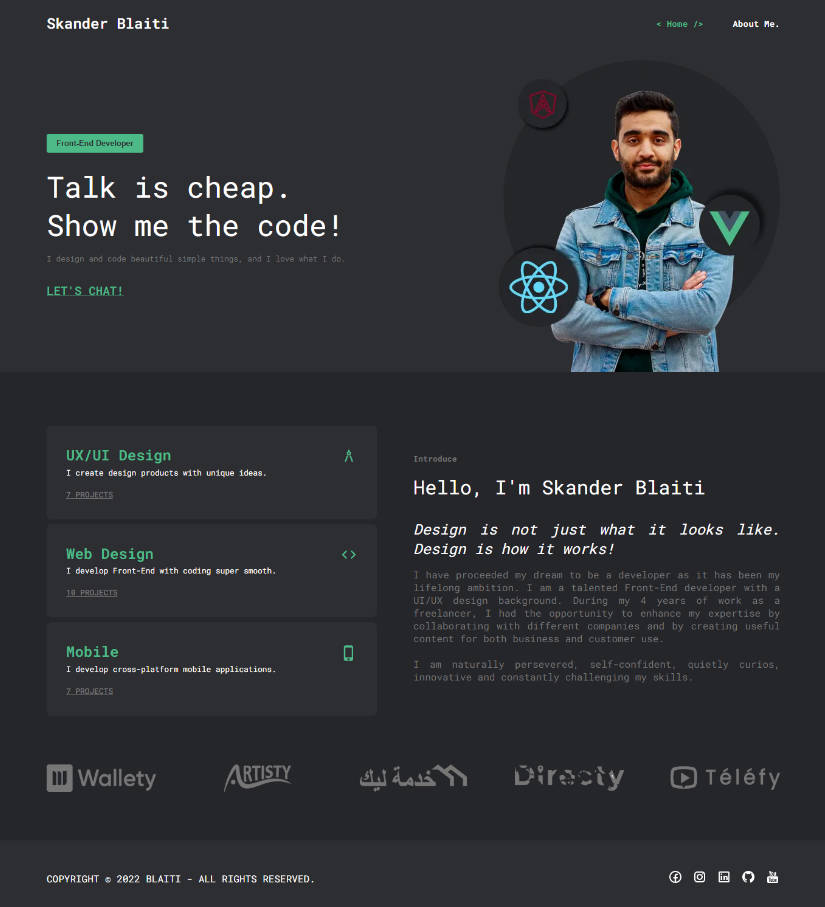
20.braydentw.io portfolio
Crafted with React, Next.js, and TailwindCSS, this portfolio showcases Brayden's skills and projects in a sleek and modern design. Explore Brayden's work for inspiration and discover the possibilities of web development with React, Next.js, and TailwindCSS.
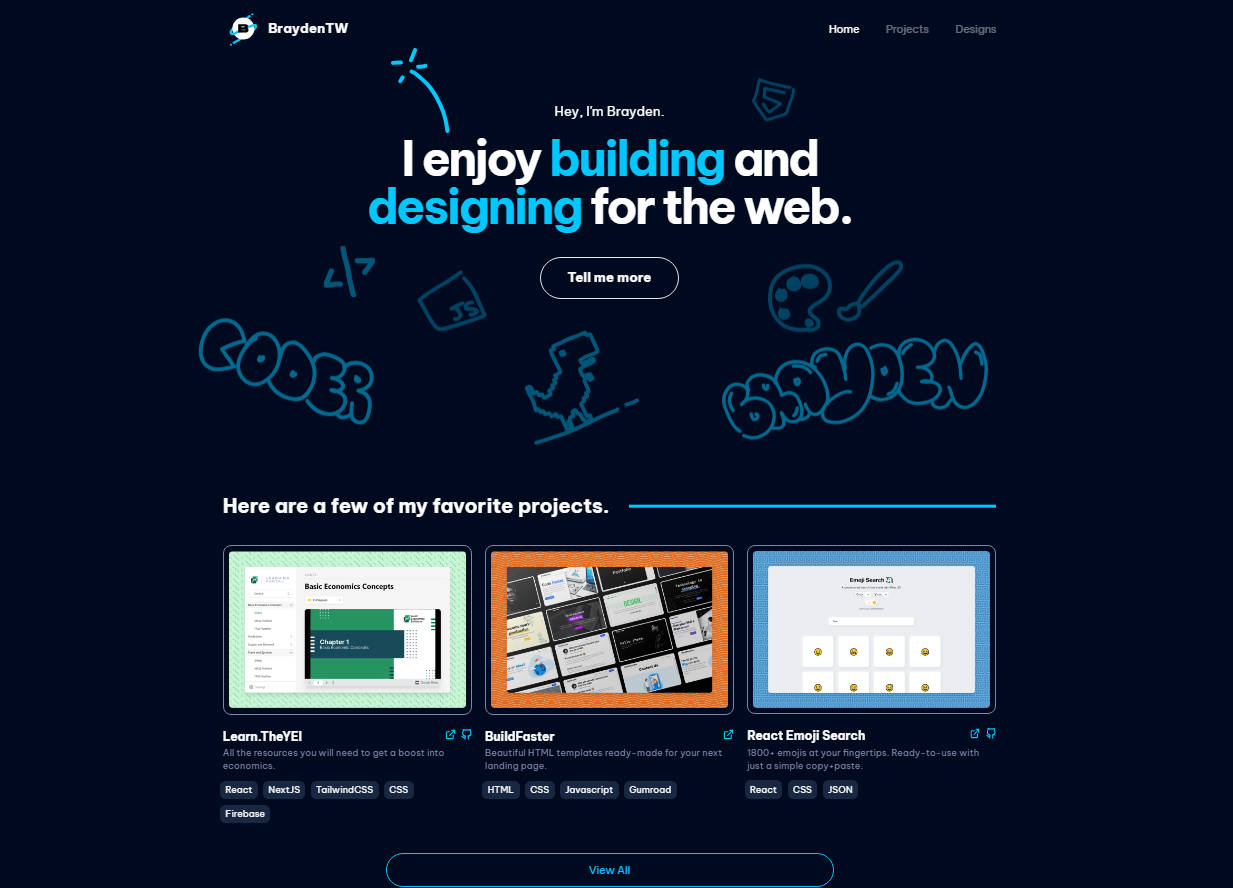
21.Sudip's portfolio
Sudip Banerjee's personal portfolio website, a showcase of his skills and projects built with React. Dive into Sudip's world and discover his passion for web development through his sleek and intuitive design. Sudip's personal portfolio serves as a testament to his expertise and creativity in the field of React development.
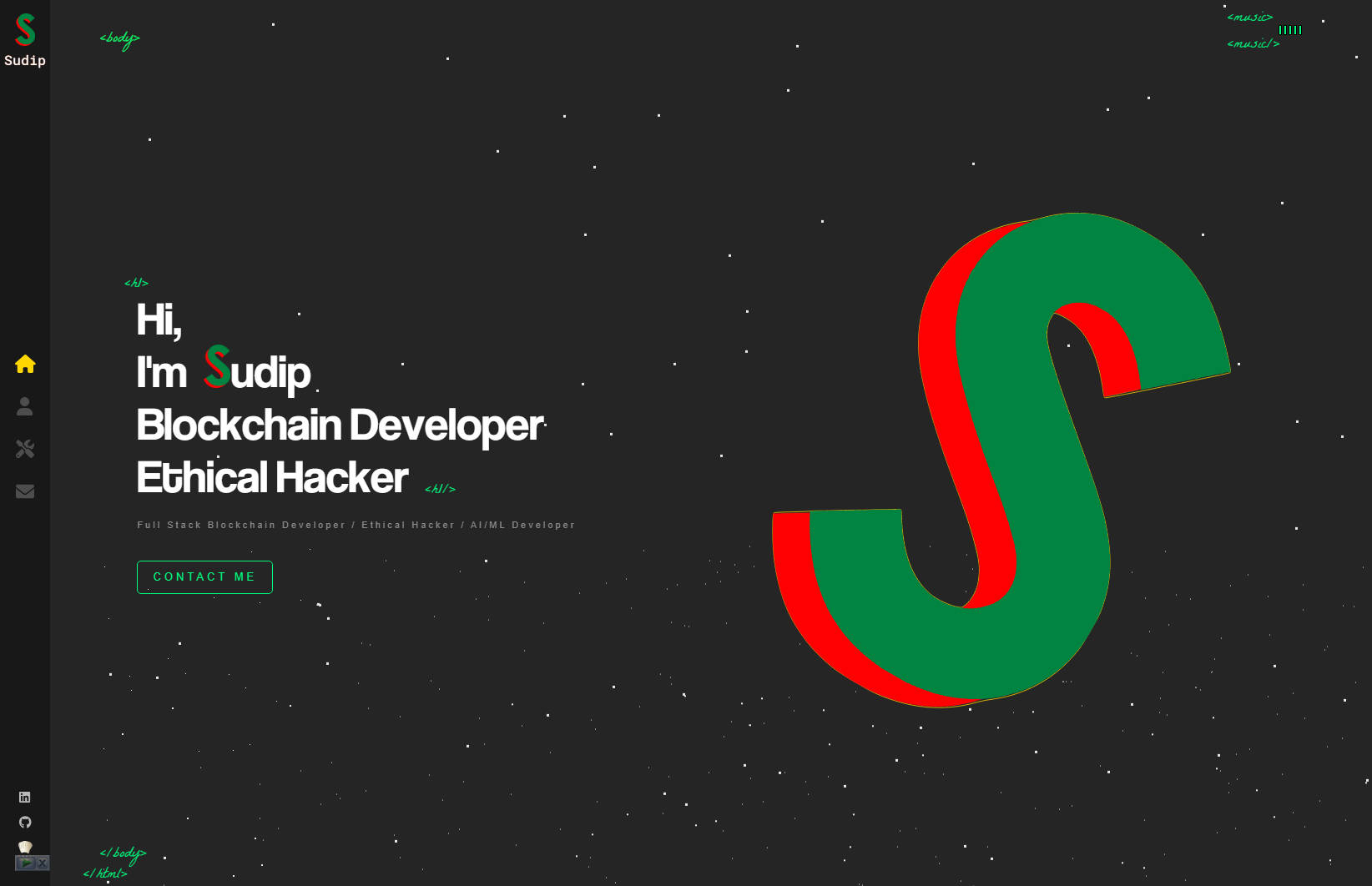
22.Developer Portfolio
Developer Portfolio is a fantastic tool for anyone looking to create a professional portfolio with ease. With its user-friendly interface and multiple themes to choose from, you can quickly customize your portfolio to reflect your unique style and showcase your skills and projects effectively. Whether you're a developer or a freelancer, Developer Portfolio provides the perfect platform to highlight your achievements and attract potential clients or employers.
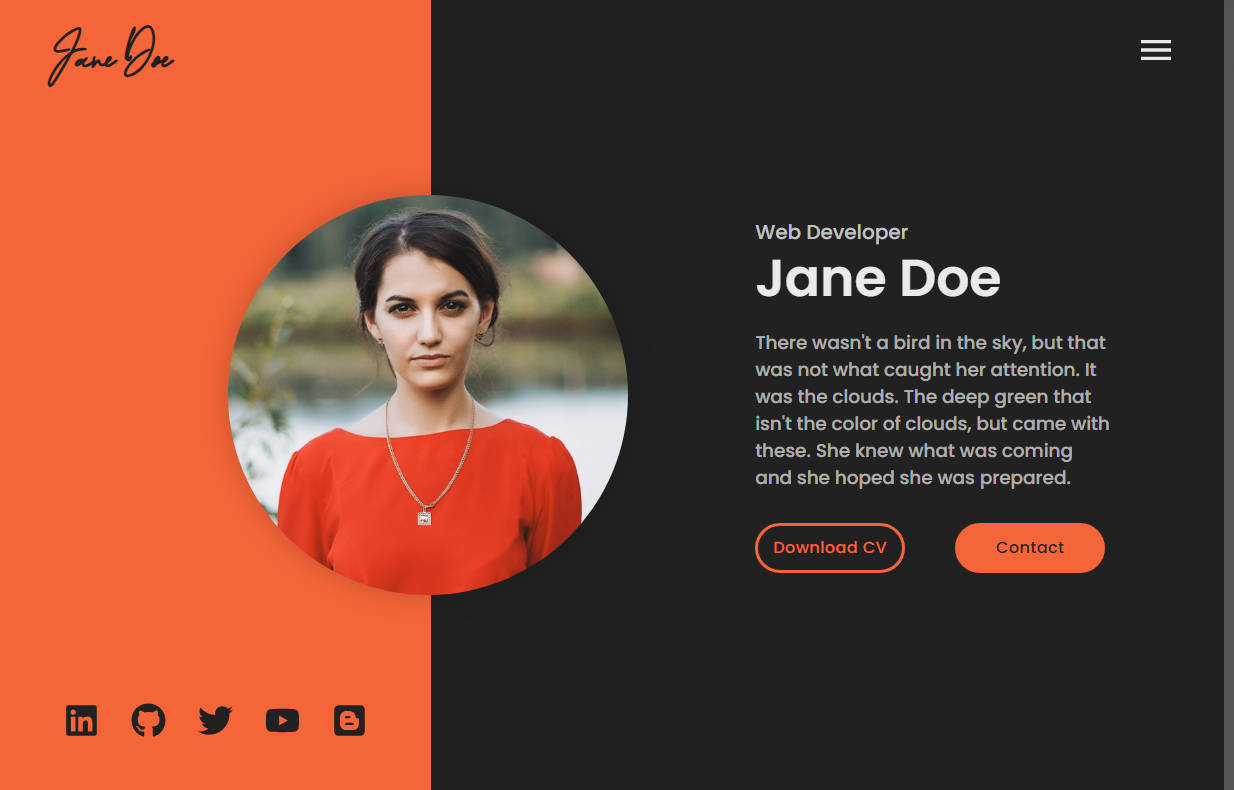
23.vscode-portfolio
vscode-portfolio is a developer portfolio website inspired by Visual Studio Code and built with Next.js. Deployed on Vercel, this sleek and stylish portfolio showcases your skills and projects in a familiar environment for developers. With its clean design and intuitive navigation, vscode-portfolio offers a professional platform to showcase your work and attract potential clients or employers.
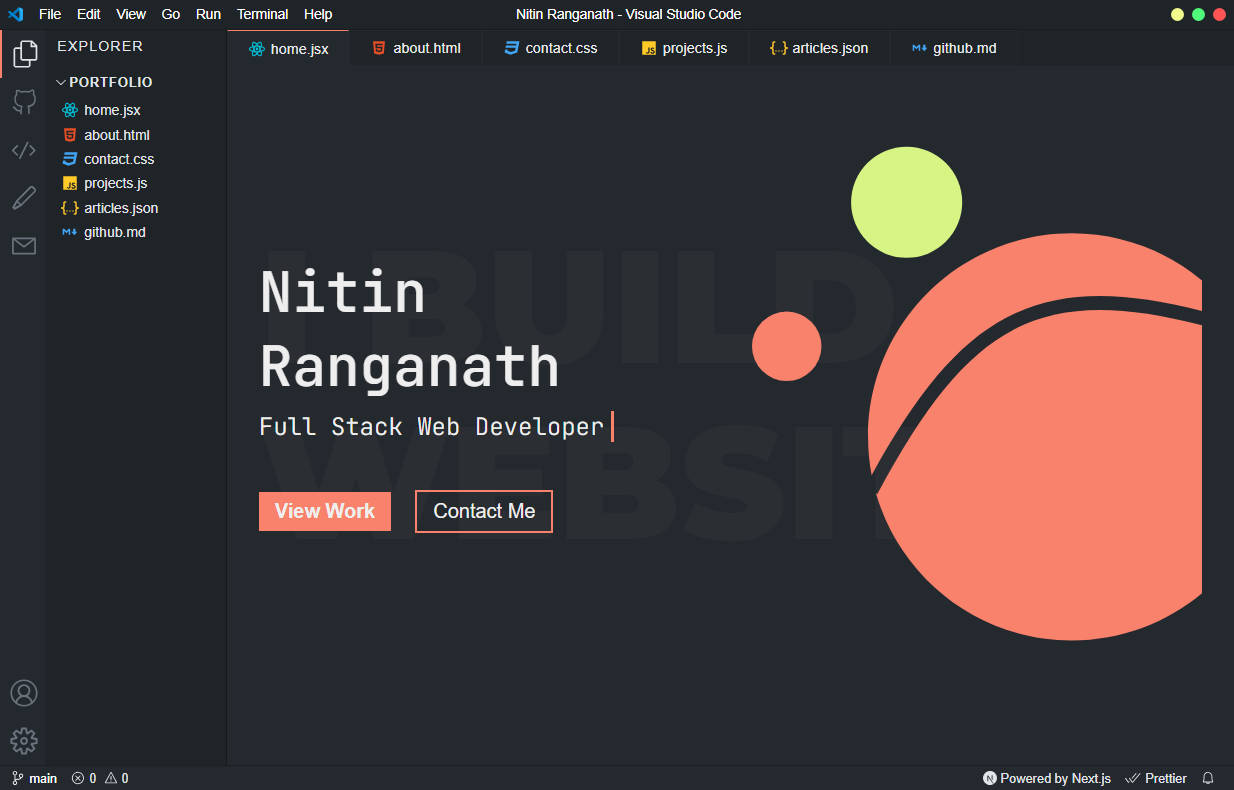
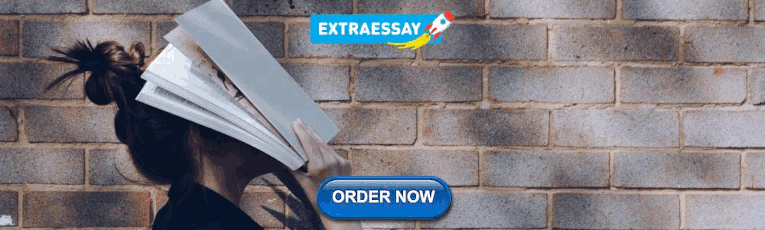
24.Vipul Jha's Personal Portfolio
Vipul Jha's Personal Portfolio is a sleek and modern template built with React and Next.js. Tailored for those who prefer Next.js for their web development needs, this template offers a clean and responsive platform to showcase your work and skills. With its simple yet elegant design, your personal portfolio will captivate visitors and leave a lasting impression.
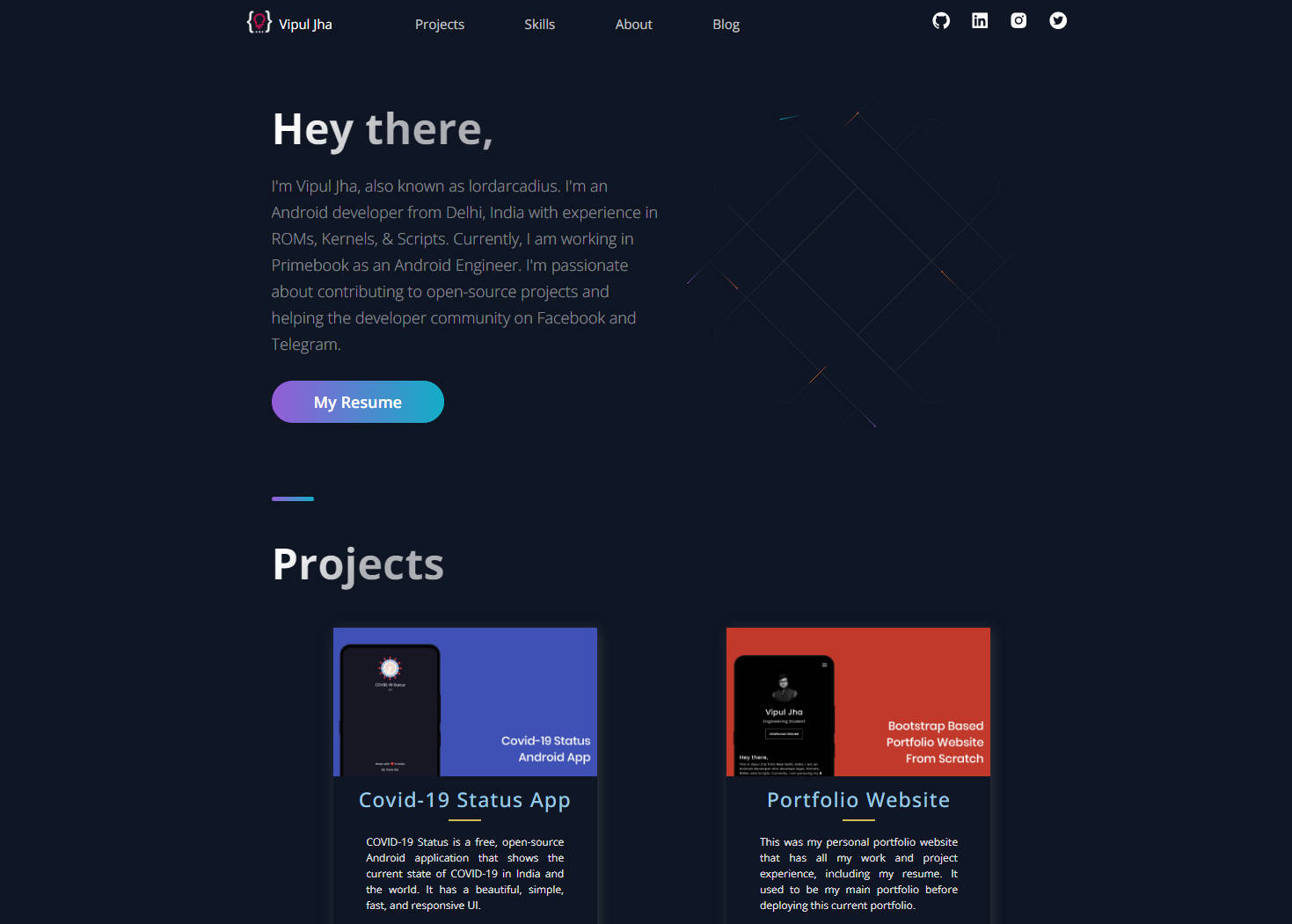
A simple ecommerce demo project developed with react, typescript & tailwind
A simple emojis todo app built with next.js, you might also like..., unded - a meticulously crafted creative agency & portfolio next.js template, superprops: trending landing page collections with next.js, 38 best react portfolio templates in 2023, subscribe to react.js examples.
Get the latest posts delivered right to your inbox
How to Build a Portfolio Website with React

Today you're going to create one of the most important apps you can build for yourself: your developer portfolio.
Every React developer or web developer in general needs to be able to show off what they can do to any potential client or employer.
That's exactly what we're going to be building right now, with the help of a number of industry standard tools, including React, Tailwind CSS, and Netlify.
Let's get started!
What Will the Portfolio Look Like?
This is the final version of the portfolio you will be building.
It will feature information about yourself, what projects you have made, what skills you've used in making those projects, and will include a contact form for clients or employers to reach out to you.
What Tools Will We Be Using?
- We will use React to create the app's user interface. It will allow us to compose each part of our landing page through reusable components and extend our app if we want to add additional features, such as a blog.
- To style our application, we will use Tailwind CSS. To give our app a professional appearance, Tailwind will allow us to easily apply multiple styles through combining classnames on our React elements.
- For pushing our app to the web, we will use the free service Netlify. It will serve our project on a custom domain to users very quickly with the help of a CDN (content delivery network).
How to Get Started
You can download the starting files for our project here .
When you grab the code, all you will have to do is drag your (unzipped) project folder into your code editor and run the command:
And you're good to go!
What Tools Do I Need to Build my Portfolio?
To go through the entire process of creating our app from start to deployment, you will need to have the following:
- Node.js installed on your computer. You can download it at nodejs.org.
- Git installed on your computer. You can download it at git-scm.com.
- I would recommend using VS Code as your code editor. You can download it at code.visualstudio.com.
- A free Netlify account at netlify.com.
- A free GitHub account at github.com.
How to Build the Portfolio Structure
The benefit of using React is that we could expand our app to as many pages as we like, very simply, and add a lot of additional content.
However, since we're just working with one page, we can within our app component figure out the different components that we need very quickly. We will have a Navbar on top with all of the links to jump to different sections of our portfolio.
After that, we will include an about section, a section for our projects, testimonials, and finally our contact form.
This quick planning allows us to figure out what our components should be named and in what order. We can go ahead and add all of them to our App.js file (in src):
How to Create our Components
Now that we have all these components listed out we need to go ahead and create them.
Within our source (src) folder, we're going to create a folder called components with all of the files that we need:
Then we will create the basic structure of each React component and export it from that file with export default :
And finally make sure to import it back in App.js:
Note that there should be six components in total.
Intro to Tailwind CSS
Once that's done, we can start working with Tailwind CSS, in order to start to give our app a basic appearance.
The benefit of using Tailwind CSS is that we don't have to write any styles manually in a CSS stylesheet. All we have to do is combine multiple classes to create the appearance that we want.
For example, to give our portfolio a dark background with gray text applied to all of our child components, you can add the following classes to our main element:
How to Build the About Component
Let's start on our first section, the about section. This will consist of a basic introduction to ourselves and what skills we specialize in.
It's also going to include some links to the contact form as well as our past projects. Since these links will be to different parts of the same page, we can use the hashes: "/#projects" and "/#contact".
To make these links work and to be able to jump to each section, we will set the id attribute of the projects section to "projects" and those of the contact section to "contact".
For the image on the righthand side of the section, I am using an svg file from the public folder, coding.svg.
This image serves merely as a temporary placeholder. I would highly recommend using an actual image of yourself.
How to Build the Projects Component
Our projects section will consist of a section element with an id of "projects". This will feature a gallery of all the projects that we've built, which will include images.
It'll have the title of the project, along with the technologies we use to make it, and a link to it (if it is deployed).
Note that we are also going to use the library @heroicons/react in order to be able to write some SVG icons as React components.
We are importing an array of projects from a data.js file in the same folder. There we are exporting an array of objects which each include an individual project's data:
How to Build the Skills Component
Let's fill out the section for all the skills and technologies that we know.
This will consist of a simple list of all of the major tools that we're familiar with and can use in our employers or clients projects.
Once again, we are going to import an array from our data folder. But this array consists of number of strings which represent each of the skills that we know such as JavaScript, React, and Node:
How to Build the Testimonials Component
In the Testimonials component, we are going to list a couple of testimonials maybe from past clients or people who are familiar with our work.
These are going to consist of a couple of cards that feature the testimonial itself as well as who it's from and the company that this person is from.
We are also importing a testimonials array with a number of objects that feature the quote, image, name, and company.
How to Build the Contact Component
At the end of our landing page, we're going to include our contact form to allow potential employers to reach out to us.
This form will have 3 inputs: a name, email, and message input.
To receive these form submissions, we will use the tool Netlify Forms to very easily take care of saving those messages.
How to Embed a Google Maps Location
To the left of the form we will include a Google Maps embedded Google map of where we are located.
We can do so with the help of an online tool: embed-map.com. All you have to do is just enter your location and hit "Generate HTML code".
In the code we are given, don't copy all of the code, just the src attribute from the iframe element. We will replace that value with the default src value we have for our iframe.

To send over any submitted form data to Netlify, Netlify Forms needs to recognize a form as static HTML. Because our React app is controlled by JavaScript and doesn't consist of plain HTML, we need to add a hidden form to our index.html file in the public folder.
We need to hide this form, because it doesn't need to be seen by the user, just Netlify.
We'll give it the attribute of hidden as well as a name attribute that matches the JSX form in Contact.js. We also need to give it the netlify attribute so that Netlify Forms recognizes it. Finally, we need to include all of the same inputs as our JSX form: for name, email, message.
How to Submit the Contact Form
Once that's done, we'll head back to Contact.js. We're going to use JavaScript in order to submit this form.
First of all, we're going to create some dedicated state for each of the values that are typed in the form for name, email, and message:
We will store what the user types in to each of the inputs in state with the help of the onChange handler.
To handle submission of the form, we will add the onSubmit prop to it. The function that will be called, handleSubmit , will make a post request to the endpoint "/" with all of our form data.
We will set the headers of the request to indicate that we are sending over form data. For the request body, we will include the form name as well as all of the form data from the name , email , and message state variables.
As you can see above, we are encoding the form data with a special encode function that you see here.
If the message is sent correctly, we will display an alert that says "Message sent". Otherwise if there is an error, we are going to alert the user of that error.
How to Build the Navbar Component
The last step is to build out our Navbar component.
We want this navbar to stick to the top of our app on large devices and not be sticky on mobile devices.
Additionally, we want to include links to each of our relevant sections for our project skills testimonials and our contact form:
How does this stick to top of the page on a larger device? With the help of the class md:sticky on our header element.
This class means that it will have the style rule position: sticky; applied starting on a medium-sized breakpoint (768px).
How to Deploy Your Portfolio
Now to make our portfolio live, we need to push our application to GitHub.
If you're not familiar with Git and GitHub, I would take a little while just to learn how to push your code to your GitHub account for the first time. It's an essential skill for any developer to know.
Once you're familiar with this process, we can first create a new Github repository. After that, we will run git add . , git commit -m "Deploy" , create our git remote, and git push -u origin master .
Once our project is on GitHub, we can head over to Netlify and select the option "Choose Site from Git". Then we will choose GitHub for our continuous deployment, and pick the GitHub repository to which we just pushed our code.
After that, our project will be automatically deployed to the web!
What's Next
Congratulations! You now have a portfolio app live on the web that shows off all of your projects and skills to potential employers.
The next step to take would be to set up a custom domain, preferably with your name (i.e. reedbarger.com ). Since Netlify includes a DNS you can easily set up a custom domain with them.
Look into maybe adding a blog to your React app to show off even more of your developer knowledge to potential employers.
Make your personal portfolio an expression of yourself and what you are passionate about as a developer and you'll have success!
Become a Professional React Developer
React is hard. You shouldn't have to figure it out yourself.
I've put everything I know about React into a single course, to help you reach your goals in record time:
Introducing: The React Bootcamp
It’s the one course I wish I had when I started learning React.
Click below to try the React Bootcamp for yourself:

Full stack developer sharing everything I know.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
gh-react-portfolio-template
A react portfolio template for github.
A performant, accessible, progressive React portfolio template that uses the GitHub REST API .
Add your GitHub username once and all of your info will automatically be updated. Deploy to GitHub pages in a few simple steps.
Google PageSpeed Insights
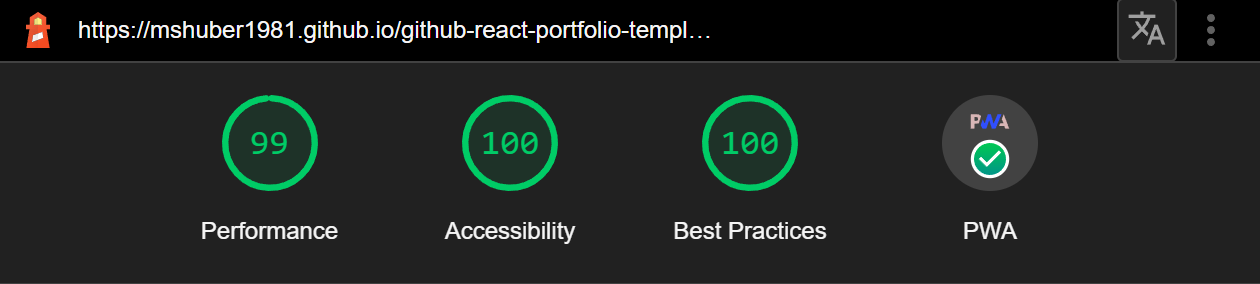
Light And Dark Themes
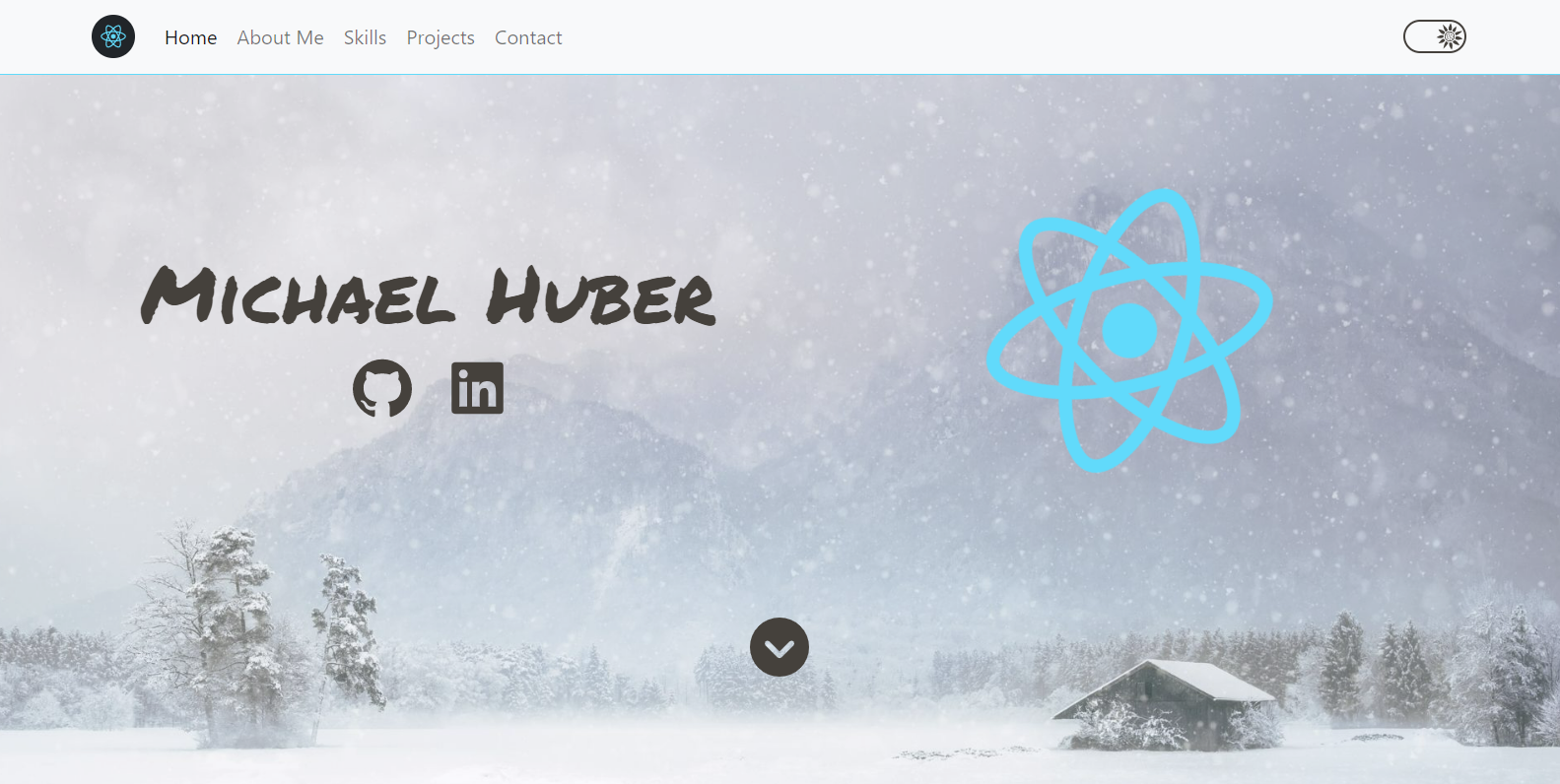
Getting Started
- Create a repository from this template
- Clone your repository
- Make sure Node is installed
Open your project and install the dependencies
- Navigate to the src directory and open data.js
Add your GitHub username ( data.js lines 14-18)
Start the development server to view the results
Updating the Projects section
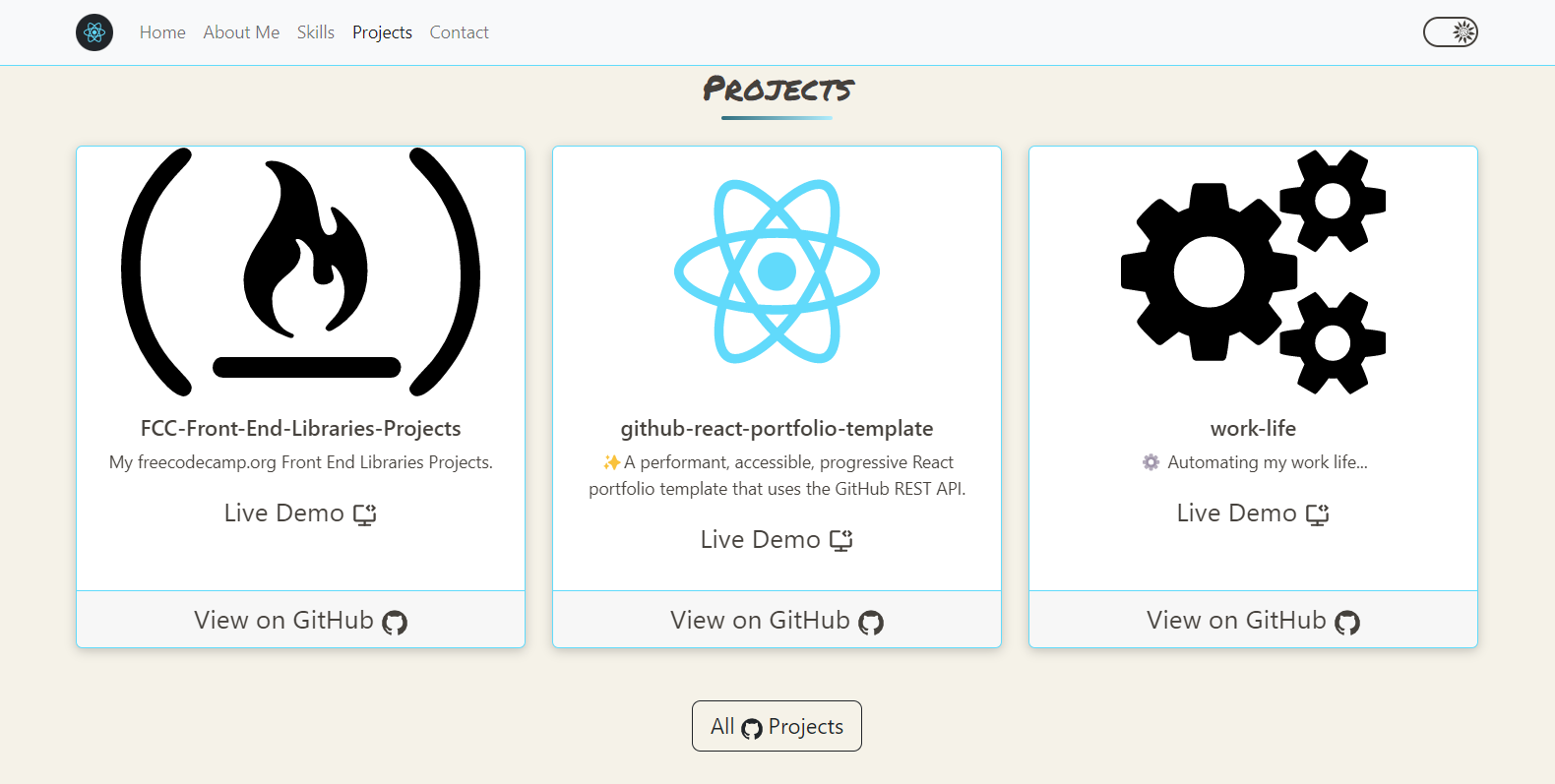
Follow the instructions to update the filteredProjects array ( data.js lines 85-89)
Import the projects images you want to use ( data.js lines 4-5) or the default image will be applied
Follow the instructions to update the projectData array ( data.js lines 91-97)
Updating the Contact section
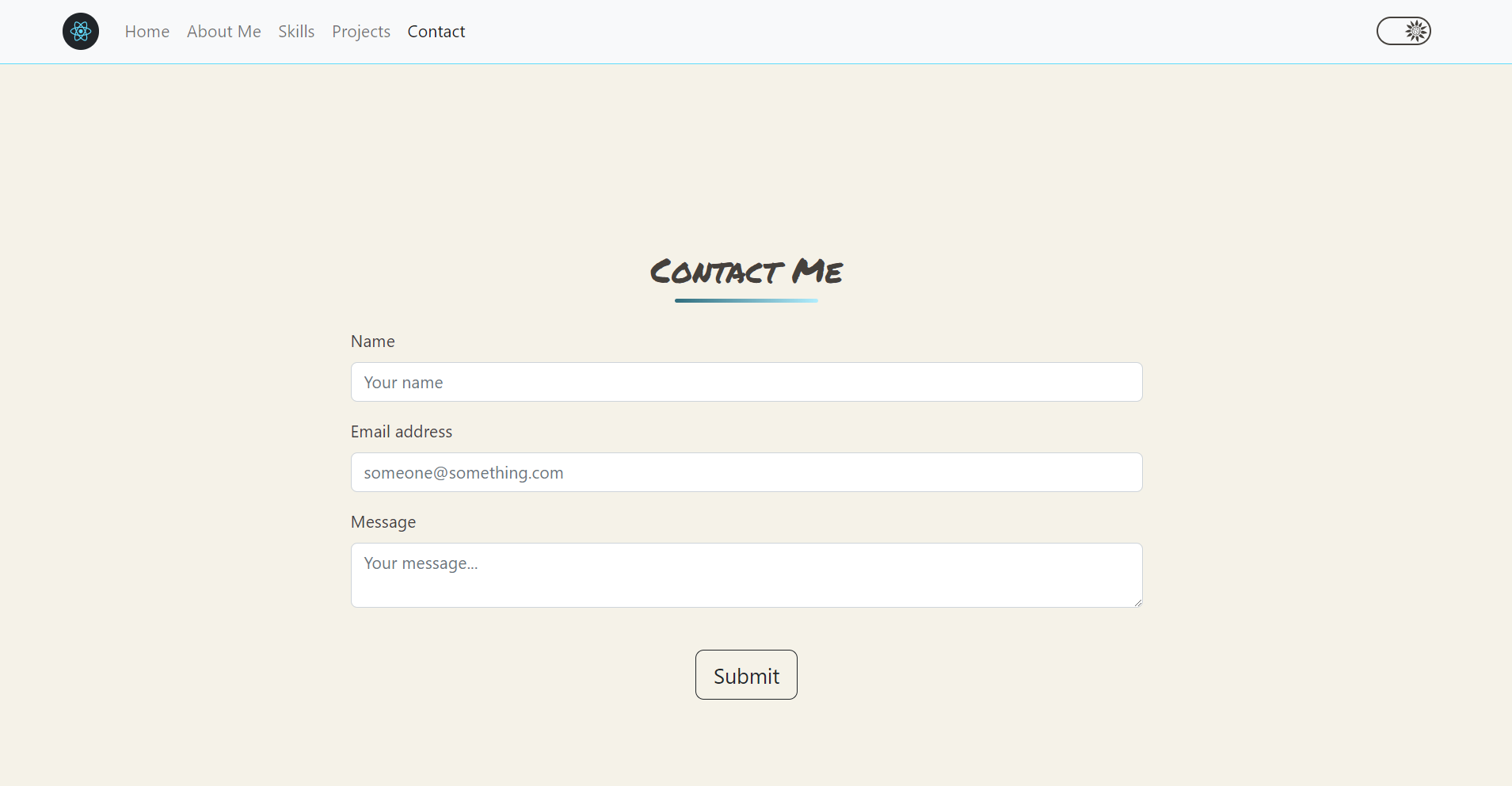
The contact form uses Formspree , create an account and add your endpoint URL ( data.js lines 99-104)
A helpful guide for Create React App deployments with GitHub Pages can be found here .
Update the homepage value ( package.json line 3)
Run the deploy command
Customization Options
Checkout the Wiki for additional customization options:
- Updating the Hero images
- Add a custom Blog icon
- Updating the About Me section
- Updating the Skills section
- Add a link to your resume
Back to top :top:

DEV Community

Posted on Oct 9, 2020 • Updated on Oct 27, 2020
Creating a Portfolio Website Using GitHub Pages and React: Part 1
photo by @joannakosinska
Part 2 on how to get started with your GitHub Pages and React app is available here . Part 3 on how to use a custom domain name is available here .
As a recent graduate from the Flatiron School Software Engineering program, I've just begun my job search and have been trying to grow my online presence. One important aspect of this has been creating my own portfolio website. In this post, I'll be discussing why a portfolio is essential for any new software developer and why GitHub Pages and React are great tools for creating it.
In future posts, I'll be going step by step through the process of creating a portfolio website using GitHub Pages and React, so stay tuned!
Click here to see the website I've created using GitHub Pages and React.
Why Create a Portfolio Website?
If you're a developer, it's likely that you already have a variety of platforms where you talk about your projects and experience. Why then bother with an additional portfolio?
1. It gives you more flexibility than other platforms
While LinkedIn and GitHub are fantastic tools for showing potential employers what skills you have and what projects you've worked on, you can't always personalise your page in the way that you'd like to. Having your own portfolio website allows you to show exactly what you want to show in the way that you want to show it. It's like having a resume but, instead of trying to fit everything onto one page, you have the space to show as much as you'd like and to draw attention to anything you think is important.
2. It allows you to show what you can do
Personally, I would love the opportunity to become a front-end developer and many of those roles ask for languages and libraries such as JavaScript and React.js. By building my website using React, I'm able to actively show potential employers what I can do with those tools and hopefully impress them with aspects I wouldn't be able to show as effectively on my resume.
3. It keeps you coding
As any fellow bootcamp grad knows, completing an intensive program is tough and it can be really tempting to take a break from coding. While it's definitely a good idea to take a couple of days off every now and then, you should never stop coding altogether. It can seem hard to find time to code, especially given how much you have to do when beginning your job search, for instance trying to put your resume together, getting those project demo videos recorded, and developing your network. For me, creating my portfolio website was a great opportunity to feel as though I was working on my job search while still keeping my coding skills sharp. It also gives you the opportunity to learn new things, shows employers that you're still working on projects, and keeps your GitHub contributions in the green.
4. It's fun!
For me, one of the most enjoyable aspects of creating my portfolio website was being able to make something in exactly the way I wanted to. While there were certain things that I knew I had to include, I had free rein over the look and functionality of my portfolio. It was nice coming from the bootcamp world of rules and regulations with regards to projects and instead being able to make something that looked and worked exactly how I wanted it to.

photo by @richygreat
Why Use GitHub Pages?
GitHub Pages allows you to turn a GitHub repository into a website. GitHub looks for web content on the master or GitHub Pages branch tied to your personal URL (more on this below) and builds your site for you in a matter of seconds. There are many options out there in terms of how to get your website online, so why choose GitHub Pages?
1. It's easy to use
As I'll cover in the next post, GitHub Pages is incredibly easy to use. Starting out on my job search, I wanted to get my site up and running as fast as possible, without having to worry about setting up a database or configuring a server. Because I already had a GitHub account which I used on a regular basis, I was familiar with the GitHub interface and flow, so the process of setting up a GitHub Pages site wasn't too much of a leap. Even if you don't have a GitHub account or don't feel very comfortable with how GitHub works, I'd still recommend using GitHub Pages. GitHub is essential for any developer to know how to use well, so this is the perfect opportunity to get familiar with it.
2. It's free
Yes, 100% free, making it the perfect option for your first website, especially if you're in the process of looking for a job. As I'll talk about below, you might choose to invest in a custom domain name, but it's not necessary if you're happy with the standard version.
3. You can add your own custom domain name
When you create your site, it will initially be available at http(s)://.github.io (for instance, http://iona-b.github.io ). While this domain name might suit your purposes, you may want something a little more personalised. You can purchase a different domain name at a number of sites at an affordable price and make your GitHub Pages site available there.
4. It's open source
GitHub is fantastic because it actually allows other users to see what your code looks like (as long as you're working in a public repository). That means that you can show people the interesting ways in which you've solved particular problems, provide examples to other users, and generally contribute to the coding community.
5. You can make changes and redeploy whenever you like
Once you've deployed your website, it's really simple to make updates and redeploy. If you've ever used GitHub before you'll be familiar with pushing changes and it just takes one extra step to redeploy your website.

photo by @sapegin
Why Use React?
There are different options when it comes to building your website with GitHub Pages and React is by no means required. For instance, you may want to use GitHub's recommended static site generator, Jekyll to build your website. Why then did I choose to use React?
1. It's a great way to improve your React.js skills
I personally love using React, so choosing to utilise it for my portfolio website wasn't a hard decision. If you already have experience working with React, this should be a fairly intuitive process. Depending on what you want to show, you can make it as straightforward or complex as you like and it's a great way to continue building on your skills. If you've never worked with React before, this could be a great opportunity to learn something new. There are so many fantastic React.js tutorials out there and it's easy to keep your site simple while you develop your skills. Once you become more comfortable with it, you can always add more features.
2. You gain access to many amazing libraries
Working with React gives you access to a variety of different libraries which allow you to further personalise your app. Want to add videos? There's a library for that. Want to add CSS specifically geared towards React? There's a library for that. Want to create a game using Unity and have it in your React app? Yep, there's also a library for that.
3. There's a really strong React.js community
No matter what question you have when it comes to working with React, somebody will have answered it somewhere. React is supported by Facebook and Instagram engineering teams, as well as dedicated experts, and there is a wealth of documentation, Stack Overflow discussions, Dev and Medium blog posts, and other resources to help you along in your React journey.
In future posts, I'll be working through exactly how I built my website using GitHub Pages and React. See you then!
- " About GitHub Pages ", GitHub Docs, Accessed October 8, 2020
- " Setting up a GitHub Pages site with Jekyll ", GitHubDocs, Accessed October 8, 2020
- " What is GitHub Pages ", GitHub Pages, Accessed October 8, 2020
Top comments (4)
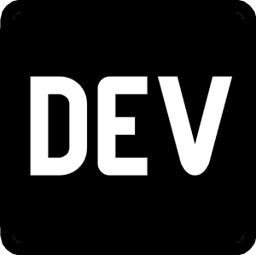
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Tampa, Florida
- Joined Jan 8, 2021
Great tips, thanks! I am about to begin my final quarter in Kenzie Academy's SE Program and one of our instructors linked to this article in a Slack channel. I have been kind of spinning my wheels as I figure out how to start creating things and putting them out into the world. The GitHub Pages and React information really helps me to start to make a mental model of how to begin. Thanks again and I hope you found a sweet job! Your portfolio site looks amazing!

- Location Mountain View, CA
- Education Flatiron School
- Work Agfa HealthCare
- Joined Jun 11, 2020
Thanks, DeQuan! I really appreciate your comments. Let me know if you have any questions!

- Joined May 9, 2020
Très bonne travail ( i notice u speaks french :P, lang section), i am Fan of blue background tho xd
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Introducing Dev Encyclopedia: A "Wikipedia", but for developers
Buzzpy 💡 - Aug 13

The Never Ending Battle Against Software Complexity
Everaldo Junior - Jul 26
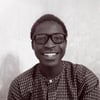
Rich Text Trimming Algorithm
Abdulmumin yaqeen - Jul 26

Understand API Requests in JavaScript: A 2024 Guide to Fetching Data
Vishal Yadav - Aug 15
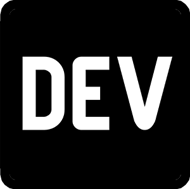
We're a place where coders share, stay up-to-date and grow their careers.
React-Portfolio
React portfolio, description, user history.
- As a visitor, I want to learn about the person behind the portfolio and their skills and experience.
- As a visitor, I want to see a collection of the developer’s projects and learn more about each project.
- As a visitor, I want to easily navigate through the different sections of the portfolio.
- As a visitor, I want to have a way to contact the developer for potential collaborations or inquiries.
- As a visitor, I want to have access to the developer’s resume for further information.
Technologies Used
- React.js: A JavaScript library for building user interfaces, used to create the portfolio’s components and manage the application’s state.
- HTML5: Markup language used for structuring the content of the portfolio.
- CSS3: Stylesheet language used for enhancing the visual appearance and layout of the portfolio.
- JavaScript: Programming language used to add interactivity and functionality to the portfolio.
- Bootstrap: CSS framework used for responsive and mobile-first web design.
- FontAwesome: Library of icons used to enhance the visual representation of social media links and other elements.
Installation
- Update the content in the App.js file to customize the portfolio sections, project details, and contact form.
- Replace the placeholder images in the img folder with your project screenshots or images.
- Customize the styling and layout in the App.css file and other CSS files as needed.
Contributing
This project is licensed under the MIT License.
Acknowledgements
React Bootstrap FontAwesome
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Romlion/React-Portfolio
Folders and files.
Name | Name | |||
---|---|---|---|---|
2 Commits | ||||
Repository files navigation
React-portfolio.
- JavaScript 42.4%
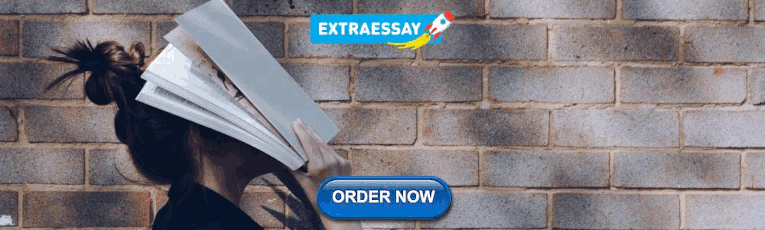
IMAGES
COMMENTS
To associate your repository with the react-portfolio topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
Additionally, this new portfolio should be created using React. At a minimum, your portfolio should include the following: A Header component that appears on multiple pages. A single Project component that will be used multiple times on a single page. Navigation with React Router, dynamic rendering, or another third part router
Builds the app for production to the build folder. It correctly bundles React in production mode and optimizes the build for the best performance. The build is minified and the filenames include the hashes. Your app is ready to be deployed! See the section about deployment for more information.
React-Portfolio. Unit 20 React Homework: React Portfolio. Now that you've worked with React and have multiple projects to share, you'll be updating your portfolio and other materials to build toward being employer competitive. Creating a portfolio using React will help set you apart from other developers whose portfolios do not use some of ...
Using GitHub to host your portfolio using GitHub pages. In the root directory of your project, initialize a git repository as follows. git init. Head over to GitHub and create an empty repository with the name githubusername.github.io, here replace githubusername with your actual GitHub username. Copy the git link for your repo and add it to ...
Step 2: Setup GitHub Repository. Setting up the repository is pretty straight forward. Make sure you flag the repository as public since it'll be needed for hosting. Follow this link for setup. Now link it to the React project that we set up locally. Using terminal, cd into the project and enter: git init.
Go to the console and run npm install create-react-app to install this module via npm (make sure that you have installed npm before using it — follow this link for more info). Now run npm create-react-app ${project-name} which will fetch build scripts and create a file-structure which will look like this. my-portfolio-app.
Deploy your portfolio website using 'Github pages' ... Deploy React-app to Github pages. Okay, so you survived until this point… take a moment to appreciate your hard work. ... Homework for ...
Set Up Form / Contact Submission. Then, create a (free) Formspree Account to collect and manage your contact submissions. Create a .env file and paste in REACT_APP_FORM_ID="not-a-real-form-id". Then, replace not-a-real-form-id with the form id listed in your Formspree account (the last part of your form's endpoint: https://formspree.io/f/not-a ...
For the 'portfolio' section, update the titles to whatever you want. The live link should be a link to the live demo of your project. The source link should be a link to the repository where the code of the project is hosted, such as GitHub. Note: If you have more than 5 projects, you will need to add more objects to the portfolio array.
Step 2: Creating Your GitHub Repository. Once you've set up your environment, the next step is to create the repo which will contain your website files. After logging into your GitHub account, click the button to create a new repository. In order for GitHub Pages to work correctly, you'll need to name this repo using the following format ...
2.soumyajit. Soumyajit Behera's portfolio template, Built with React.js, Node.js, and Express.js, this stunning website showcases the versatility of modern web development technologies. With its multi-page layout and fully responsive design, Portfolio Website - v2.0 ensures that your work shines on any device.
First of all, we're going to create some dedicated state for each of the values that are typed in the form for name, email, and message: const [name, setName] = React.useState(""); const [email, setEmail] = React.useState(""); const [message, setMessage] = React.useState(""); We will store what the user types in to each of the inputs in state ...
Click on Advanced DNS tab and find Host Records. Click Add new Record button to add new records. Add the following records: Replace githubusername.github.io with your actual GitHub username. After this, your domain is ready to be used with GitHub Pages. Using GitHub to host your portfolio using GitHub pages.
gh-react-portfolio-template. A React Portfolio Template for GitHub. A performant, accessible, progressive React portfolio template that uses the GitHub REST API. Add your GitHub username once and all of your info will automatically be updated. Deploy to GitHub pages in a few simple steps.
Add this topic to your repo. To associate your repository with the react-portfolio topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
In this post, I'll be discussing why a portfolio is essential for any new software developer and why GitHub Pages and React are great tools for creating it. In future posts, I'll be going step by step through the process of creating a portfolio website using GitHub Pages and React, so stay tuned!
React.js: A JavaScript library for building user interfaces, used to create the portfolio's components and manage the application's state. HTML5: Markup language used for structuring the content of the portfolio. CSS3: Stylesheet language used for enhancing the visual appearance and layout of the portfolio.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Packages. Host and manage packages
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.