
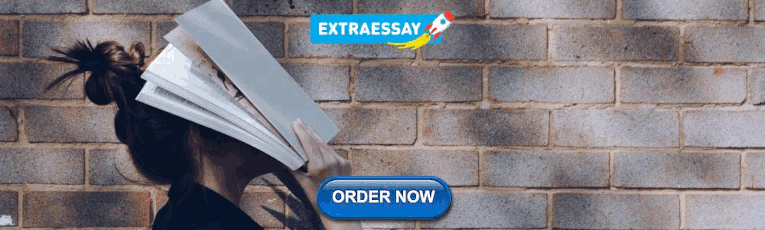
You're signed out
Sign in to ask questions, follow content, and engage with the Community
- Canvas Instructor
- Instructor Guide
How do I copy an assignment to another course?
- Subscribe to RSS Feed
- Printer Friendly Page
- Report Inappropriate Content
in Instructor Guide
Note: You can only embed guides in Canvas courses. Embedding on other sites is not supported.
Community Help
View our top guides and resources:.
To participate in the Instructure Community, you need to sign up or log in:
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
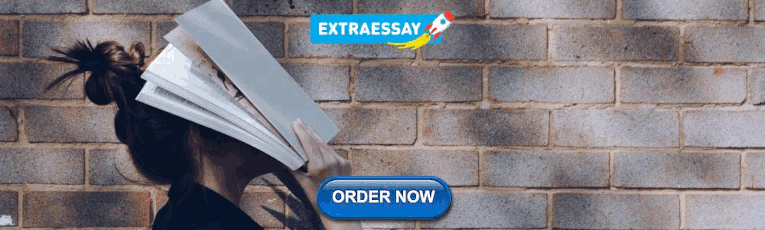
Copy Constructor vs Assignment Operator in C++
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them:
Consider the following C++ program.
Explanation: Here, t2 = t1; calls the assignment operator , same as t2.operator=(t1); and Test t3 = t1; calls the copy constructor , same as Test t3(t1);
Must Read: When is a Copy Constructor Called in C++?
Similar Reads
Improve your coding skills with practice.
What kind of Experience do you want to share?
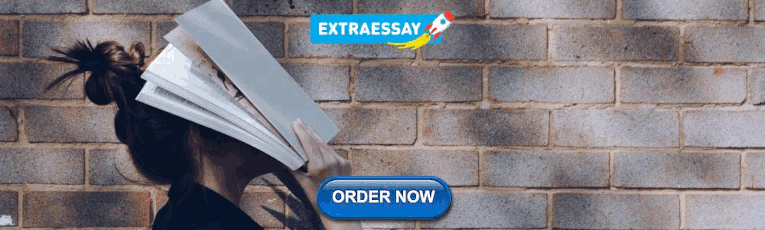
IMAGES
VIDEO