xdice 1.2.1
pip install xdice Copy PIP instructions
Released: Jan 16, 2020
The swiss knife for Dice roll : Command line, API (documented!), advanced dice notation parser, compilable patterns...etc.
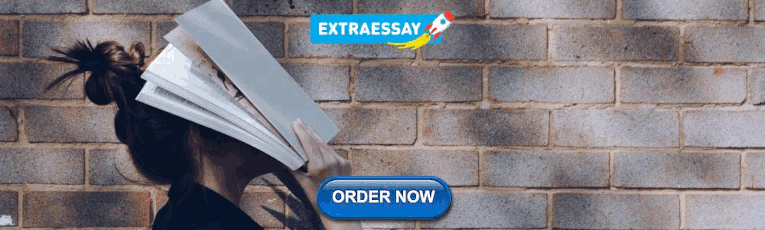
Verified details
Maintainers.
Unverified details
Github statistics.
- Open issues:
License: GNU General Public License (GPL) (GNU)
Author: Olivier Massot
Tags xdice, roll, d20, game, random, parser, dices, role, board
Requires: Python >=3.4
Classifiers
- 5 - Production/Stable
- Other Audience
- OSI Approved :: GNU General Public License (GPL)
- Python :: 3.4
- Python :: 3.5
- Python :: 3.6
- Python :: 3.7
- Python :: 3.8
- Games/Entertainment :: Board Games
- Games/Entertainment :: Multi-User Dungeons (MUD)
- Games/Entertainment :: Role-Playing
- Games/Entertainment :: Turn Based Strategy
Project description
xdice is a lightweight python library for managing dice, scores, and dice-notation patterns.
Python Versions
xdice has been tested with python 3.4+
Documentation
For more, see the Documentation
Run python roll.py [options] <expr>
CONTRIBUTION
Any opinion / contribution is welcome, please contact us.
xdice is under GNU License
Olivier Massot, 2017
Project details
Release history release notifications | rss feed.
Jan 16, 2020
Sep 28, 2017
Sep 23, 2017
Sep 21, 2017
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages .
Source Distributions
Built distribution.
Uploaded Jan 16, 2020 Python 3
Hashes for xdice-1.2.1-py3-none-any.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | ||
MD5 | ||
BLAKE2b-256 |
- português (Brasil)
Supported by
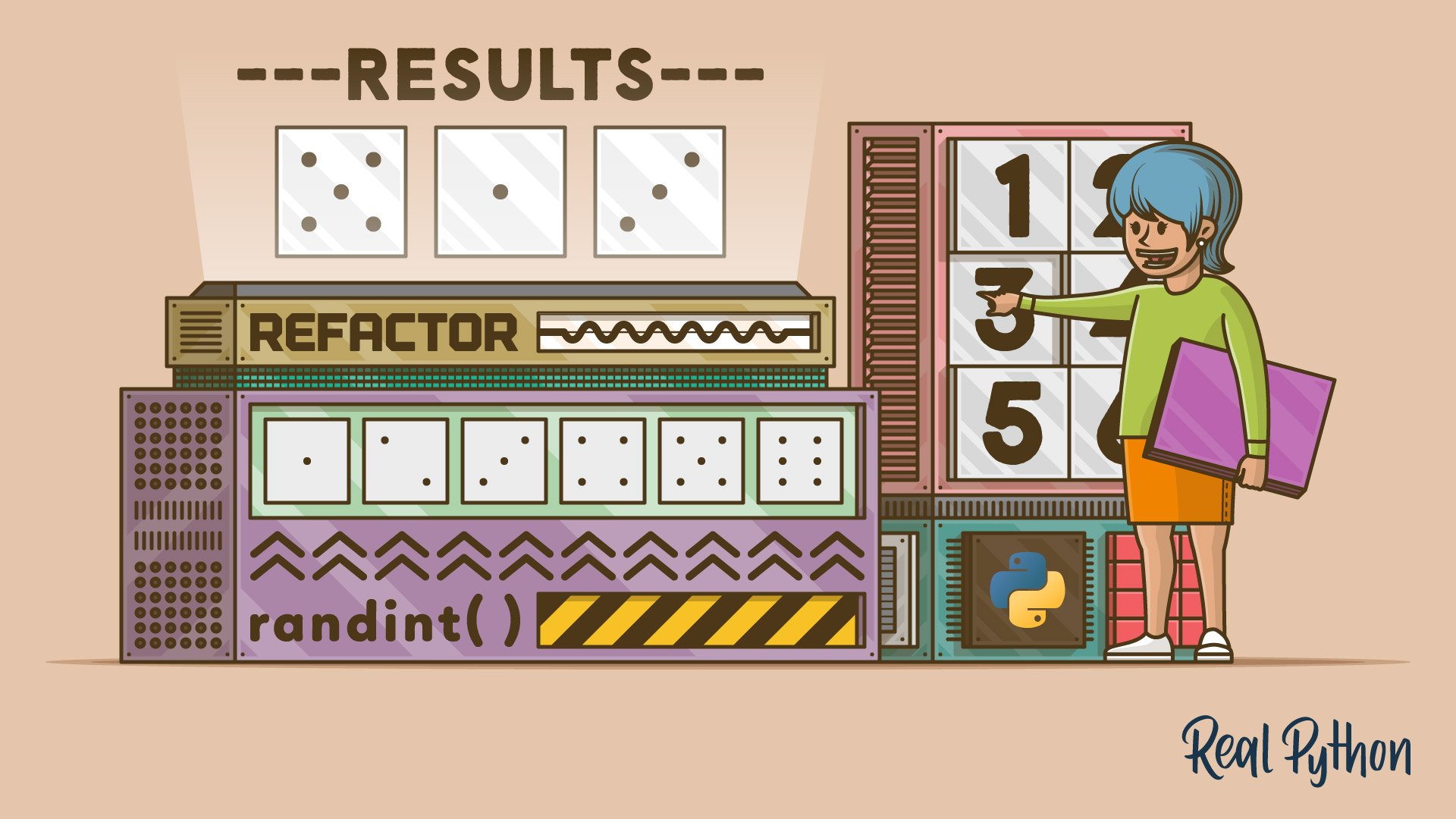
Build a Dice-Rolling Application With Python
Table of Contents
Project Overview
Prerequisites, take the user’s input at the command line, parse and validate the user’s input, try out the dice-rolling app’s tui, step 2: simulate the rolling of six-sided dice in python, set up the diagram of dice faces, generate the diagram of dice faces, finish up the app’s main code and roll the dice, step 4: refactor the code that generates the diagram of dice faces.
Building small projects, like a text-based user interface (TUI) dice-rolling application, will help you level up your Python programming skills. You’ll learn how to gather and validate the user’s input, import code from modules and packages, write functions, use for loops and conditionals, and neatly display output by using strings and the print() function.
In this project, you’ll code an application that simulates dice-rolling events. To do so, you’ll use Python’s random module.
In this tutorial, you’ll learn how to:
- Use random.randint() to simulate dice-rolling events
- Ask for the user’s input using the built-in input() function
- Parse and validate the user’s input
- Manipulate strings using methods, such as .center() and .join()
You’ll also learn the basics of how to structure, organize, document, and run your Python programs and scripts.
Click the link below to download the entire code for this dice-rolling application and follow along while you build the project yourself:
Get Source Code: Click here to get the source code you’ll use to build your Python dice-rolling app.
In this step-by-step project, you’ll build an application that runs dice-rolling simulations. The app will be able to roll up to six dice, with each die having six faces. After every roll, the application will generate an ASCII diagram of dice faces and display it on the screen. The following video demonstrates how the app works:
When you run your dice-rolling simulator app, you get a prompt asking for the number of dice you want to roll. Once you provide a valid integer from 1 to 6, inclusive, then the application simulates the rolling event and displays a diagram of dice faces on the screen.
Your dice-rolling simulator app will have a minimal yet user-friendly text-based user interface (TUI) , which will allow you to specify the number of six-sided dice that you’d like to roll. You’ll use this TUI to roll the dice at home without having to fly to Las Vegas.
Here’s a description of how the app will work internally:
Tasks to Run | Tools to Use | Code to Write |
---|---|---|
Prompt the user to choose how many six-sided dice to roll, then read the user’s input | Python’s built-in function | A call to with appropriate arguments |
Parse and validate the user’s input | String methods, comparison operators, and | A user-defined function called |
Run the dice-rolling simulation | Python’s module, specifically the function | A user-defined function called |
Generate an ASCII diagram with the resulting dice faces | Loops, , and | A user-defined function called |
Display the diagram of dice faces on the screen | Python’s built-in function | A call to with appropriate arguments |
Keeping these internal workings in mind, you’ll code three custom functions to provide the app’s main features and functionalities. These functions will define your code’s public API , which you’ll call to bring the app to life.
To organize the code of your dice-rolling simulator project, you’ll create a single file called dice.py in a directory of your choice in your file system. Go ahead and create the file to get started!
You should be comfortable with the following concepts and skills before you start building this dice-rolling simulation project:
- Ways to run scripts in Python
- Python’s import mechanism
- The basics of Python data types , mainly strings and integer numbers
- Basic data structures , especially lists
- Python variables and constants
- Python comparison operators
- Boolean values and logical expressions
- Conditional statements
- Python for loops
- The basics of input, output , and string formatting in Python
If you don’t have all of the prerequisite knowledge before starting this coding adventure, then that’s okay! You might learn more by going ahead and getting started! You can always stop and review the resources linked here if you get stuck.
Step 1: Code the TUI of Your Python Dice-Rolling App
In this step, you’ll write the required code to ask for the user’s input of how many dice they want to roll in the simulation. You’ll also code a Python function that takes the user’s input, validates it, and returns it as an integer number if the validation was successful. Otherwise, the function will ask for the user’s input again.
To download the code for this step, click the following link and navigate to the source_code_step_1/ folder:
To get your hands dirty, you can start writing the code that interacts with the user. This code will provide the app’s text-based interface and will rely on input() . This built-in function reads the user input from the command line. Its prompt argument allows you to pass a description of what type of input you need.
Fire up your favorite editor or IDE and type the following code into your dice.py file:
Your call to input() on line 5 displays a prompt asking how many dice the user wants to roll. The number must fall in the interval from 1 to 6, inclusive, as the prompt suggests.
Note: By adding the comment on line 3, you’re separating the application’s main code from the rest of the code that you’ll be adding in upcoming sections.
Likewise, the comment on line 4 reflects the specific task you’re performing at that moment. You’ll find more comments like these in other sections of this tutorial. These comments are optional, so feel free to get rid of them if you’d like.
Line 6 calls parse_input() and stores the return value in num_dice . In the following section, you’ll implement this function.
The job of parse_input() is to take the user’s input as a string, check if it’s a valid integer number, and return it as a Python int object. Go ahead and add the following to your dice.py file, right before the app’s main code:
Here’s how this code works line by line:
Line 3 defines parse_input() , which takes the input string as an argument.
Lines 4 to 9 provide the function’s docstring. Including an informative and well-formatted docstring in your functions is a best practice in Python programming because docstrings allow you to document your code .
Line 10 checks if the user input is a valid value for the number of dice to roll. The call to .strip() removes any unwanted spaces around the input string. The in operator checks if the input falls within the set of allowed numbers of dice to roll. In this case, you use a set because membership tests in this Python data structure are quite efficient.
Line 11 converts the input into an integer number and returns it to the caller.
Line 13 prints a message to the screen to alert the user of invalid input, if applicable.
Line 14 exits the app with a SystemExit exception and a status code of 1 to signal that something went wrong.
With parse_input() , you process and validate the user’s input at the command line. Validating any input that comes directly from the user or any untrusted sources is key for your application to work reliably and securely.
Note: The line numbers in the code samples in this tutorial are intended to facilitate the explanation. Most of the time, they won’t match the line numbers in your final script.
Now that you have a user-friendly TUI and a proper input validation mechanism, you need to make sure that these functionalities work correctly. That’s what you’ll do in the following section.
To try out the code that you’ve written so far, open a command-line window and run your dice.py script:
If you enter an integer number from 1 to 6, then the code doesn’t display a message. On the other hand, if the input isn’t a valid integer number or falls outside the target interval, then you get a message telling you that an integer number from 1 to 6 is required.
Up to this point, you’ve successfully written code to request and parse the user’s input at the command line. This code provides the application’s TUI, which is based on the built-in input() function. You’ve also coded a function to validate the user’s input and return it as an integer number. Now, it’s time to roll the dice!
Your dice-rolling app now provides a TUI to take the user’s input and process it. Great! To continue building the application’s main functionality, you’ll write the roll_dice() function, which will allow you to simulate a dice-rolling event. This function will take the number of dice that the user wants to roll.
Python’s random module from the standard library provides the randint() function, which generates pseudo-random integer numbers in a given interval. You’ll take advantage of this function to simulate rolling dice.
To download the code for this step, click the following link and look inside the source_code_step_2/ folder:
Here’s the code that implements roll_dice() :
In this code snippet, line 2 imports random into your current namespace . This import allows you to access the randint() function later. Here’s a breakdown of the rest of the code:
Line 6 defines roll_dice() , which takes an argument representing the number of dice to roll in a given call.
Lines 7 to 11 provide the function’s docstring .
Line 12 creates an empty list , roll_results , to store the results of the dice-rolling simulation.
Line 13 defines a for loop that iterates once for each die that the user wants to roll.
Line 14 calls randint() to generate a pseudo-random integer number from 1 to 6, inclusive. This call generates a single number on each iteration. This number represents the result of rolling a six-sided die.
Line 15 appends the current die-rolling result in roll_results .
Line 16 returns the list of dice-rolling simulation results.
To try out your newly created function, add the following lines of code to the end of your dice.py file:
In this code, line 9 calls roll_dice() with num_dice as an argument. Line 11 calls print() to show the result as a list of numbers on your screen. Each number in the list represents the result for a single die. You can remove line 11 after testing your code.
Go ahead and run your app from the command line:
The lists of results on your screen will be different because you’re generating your own pseudo-random numbers. In this example, you simulate the rolling of five and two dice, respectively. The value of each die is between 1 and 6 because you’re working with six-sided dice.
Now that you’ve written and tried out the code that simulates a dice-rolling event, it’s time to move on and provide your application with a flashy way to display these results. That’s what you’ll do in the next section.
Step 3: Generate and Display the ASCII Diagram of Dice Faces
At this point, your app already simulates the rolling of several dice and stores the results as a list of numbers. A list of numbers doesn’t look attractive from the user’s perspective, though. You need a fancier output so that your app looks professional.
In this section, you’ll write code to generate a diagram showing the faces of up to six dice. To do this, you’ll create a bit of ASCII art.
Click the link below to download the code for this step so that you can follow along with the project. You’ll find what you need in the source_code_step_3/ folder:
Your dice-rolling simulator app needs a way to show the result of rolling the dice. To this end, you’ll use an ASCII diagram of dice faces that’ll show the result of rolling the desired number of six-sided dice. For example, after rolling four dice, the diagram will look something like this:
Each die face in this diagram reflects the value that results from one iteration of the simulation. To start coding the functionality to build this diagram, you need to put together some ASCII art. Get back to your code editor and add the following:
In lines 4 to 47, you draw six dice faces using ASCII characters. You store the faces in DICE_ART , a dictionary that maps each face to its corresponding integer value.
Line 48 defines DIE_HEIGHT , which holds the number of rows a given face will occupy. In this example, each face takes up five rows. Similarly, line 49 defines DIE_WIDTH to hold the number of columns required to draw a die face. In this example, the width is 11 characters.
Finally, line 50 defines DIE_FACE_SEPARATOR , which holds a whitespace character. You’ll use all these constants to generate and display the ASCII diagram of dice faces for your application.
At this point, you’ve built the ASCII art for each die face. To put these pieces together into a final diagram representing the complete results of the dice-rolling simulation, you’ll write another custom function:
This function does the following:
Line 5 defines generate_dice_faces_diagram() with a single argument called dice_values . This argument will hold the list of dice-rolling integer values that results from calling roll_dice() .
Lines 6 to 18 provide the function’s docstring.
Line 20 creates an empty list called dice_faces to store the dice faces corresponding to the input list of dice values. These dice faces will display in the final ASCII diagram.
Line 21 defines a for loop to iterate over the dice values.
Line 22 retrieves the die face corresponding to the current die value from DICE_ART and appends it to dice_faces .
Line 25 creates an empty list to hold the rows in the final dice faces diagram.
Line 26 defines a loop that iterates over indices from 0 to DIE_HEIGHT - 1 . Each index represents the index of a given row in the dice faces diagram.
Line 27 defines row_components as an empty list to hold portions of the dice faces that will fill a given row.
Line 28 starts a nested for loop to iterate over the dice faces.
Line 29 stores each row component.
Line 30 joins the row components into a final row string, separating individual components with spaces.
Line 31 appends each row string to the list holding the rows that’ll shape the final diagram.
Line 34 creates a temporary variable to hold the width of the current dice faces diagram.
Line 35 creates a header showing the word RESULTS . To do so, it uses str.center() with the diagram’s width and the tilde symbol ( ~ ) as arguments.
Line 37 generates a string that holds the final dice faces diagram. The line feed character ( \n ) works as a row separator. The argument to .join() is a list of strings concatenating the diagram header and the strings (rows) that shape the dice faces.
Line 38 returns a ready-to-print dice faces diagram to the caller.
Wow! That was a lot! You’ll go back to this code and improve it to make it more manageable in just a bit. Before doing that, though, you’ll want to try out your application, so you need to finish writing its main code block.
With generate_dice_faces_diagram() in place, you can now finish writing the application’s main code, which will allow you to actually generate and display the diagram of dice faces on your screen. Go ahead and add the following lines of code to the end of dice.py :
Line 12 calls generate_dice_faces_diagram() with roll_results as an argument. This call builds and returns a diagram of dice faces that corresponds to the current dice-rolling results. Line 14 calls print() to display the diagram on the screen.
With this update, you can run the application again. Get back to your command line and execute the following command:
Cool! Now your dice-rolling simulator app displays a nicely formatted ASCII diagram showing the result of the simulation event. That’s neat, isn’t it?
If you go back to the implementation of generate_dice_faces_diagram() , then you’ll note that it includes a few comments that point out what the corresponding portion of code is doing:
This kind of comment often signals that your code would benefit from some refactoring . In the following section, you’ll use a popular refactoring technique that will help you clean up the code and make it more maintainable.
Your generate_dice_faces_diagram() function requires explanatory comments because it performs several operations at a time, which violates the single-responsibility principle .
Roughly stated, this principle says that every function, class, or module should do only one thing. That way, changes in a given functionality won’t break the rest of the code. As a result, you’ll end up with a more robust and maintainable code.
To download the code for this step, click the link below, then check out the source_code_step_4/ folder:
There’s a refactoring technique called extract method that can help you improve your code by extracting functionality that can work independently. For example, you can extract the code from line 20 to 22 in the previous implementation of generate_dice_faces_diagram() and place it in a non-public helper function called _get_dice_faces() :
You can call _get_dice_faces() from generate_dice_faces_diagram() to get the implied functionality. By using this technique, you can fully refactor generate_dice_faces_diagram() to satisfy the single-responsibility principle.
Note: To learn more about naming non-public functions with a leading underscore ( _ ), check out Single and Double Underscores in Python Names .
Here’s a refactored version of generate_dice_faces_diagram() that takes advantage of _get_dice_faces() and implements another helper function called _generate_dice_faces_rows() to extract the functionality from line 25 to 31:
The newly added helper functions extract functionality from the original function. Now each helper function has its own single responsibility. Helper functions also allow you to use readable and descriptive names, removing the need for explanatory comments.
Refactoring your code to get it into better shape is a great skill to have as a Python developer. To dig deeper into code refactoring, check out Refactoring Python Applications for Simplicity .
A fundamental idea behind code refactoring is that the modified code should work the same as the original code. To check this principle, go ahead and run your application again!
With that, you’ve completed your project! You’ve built a fully functional TUI application that allows you to simulate a dice-rolling event. Every time you run the application, you can simulate the rolling of up to six dice with six faces each. You’ll even get to see the resulting dice faces in a nice-looking ASCII diagram. Great job!
You’ve coded a fully functional project consisting of a text-based user interface application that simulates the rolling of six-sided dice in Python. With this project, you learned and practiced fundamental skills, such as gathering and validating the user’s input, importing code, writing functions , using loops and conditionals, and displaying nicely formatted output on-screen.
In this tutorial, you learned how to:
- Use random.randint() to simulate the rolling of dice
- Take the user’s input at the command line using the built-in input() function
- Parse and validate the user’s input using several tools and techniques
Additionally, you learned how to structure, organize, document, and run Python programs and scripts. With this knowledge, you’re better prepared to continue your coding journey with Python.
You can download the full code for this dice-rolling application by clicking the link below:
Now that you’ve finished building your dice-rolling application, you can take the project a step further by adding new functionality. Adding new features by yourself will help you continue to learn exciting new coding concepts and techniques.
Here are some ideas to take your project to the next level:
- Support for any number of dice: Modify the code so that you can roll any number of dice.
- Support for dice with different numbers of faces: Add code to support not only six-sided dice but dice with any number of sides.
The first feature will require you to modify the code that processes the user’s input for the number of dice to roll. You’ll also need to modify the code that generates and displays the diagram of dice faces. For example, you can generate a diagram that displays the dice faces in several rows to avoid cluttering your screen with cramped output.
On the other hand, supporting dice with a different number of faces will demand that you tweak the code that simulates a dice-rolling event. You’ll also need to create new ASCII art for any dice with more than six faces.
Once you’re done with these new features, you can change gears and jump into other cool projects. Here are some great next steps for you to continue learning Python and building more complex projects:
How to Build Command Line Interfaces in Python With argparse: In this step-by-step Python tutorial, you’ll learn how to take your command-line Python scripts to the next level by adding a convenient command-line interface that you can write with argparse .
Build a Python Directory Tree Generator for the Command Line: In this step-by-step project, you’ll create a Python directory tree generator application for your command line. You’ll code the command-line interface with argparse and traverse the file system using pathlib .
Build a Command-Line To-Do App With Python and Typer: In this step-by-step project, you’ll create a to-do application for your command line using Python and Typer . While you build this app, you’ll learn the basics of Typer, a modern and versatile library for building command-line interfaces.
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
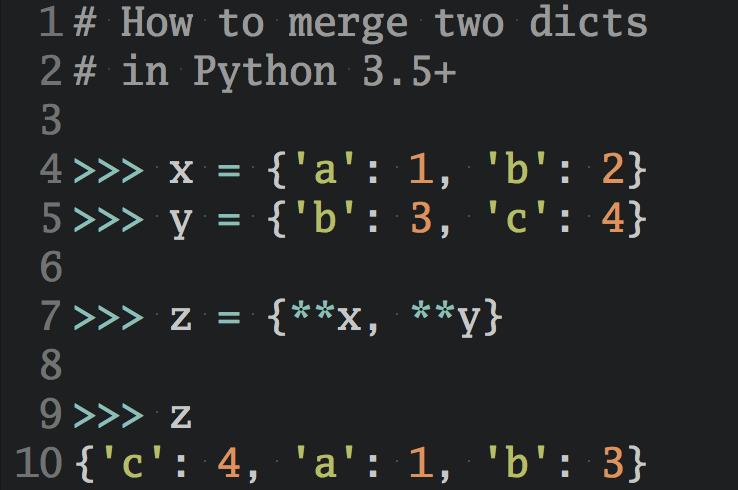
About Leodanis Pozo Ramos
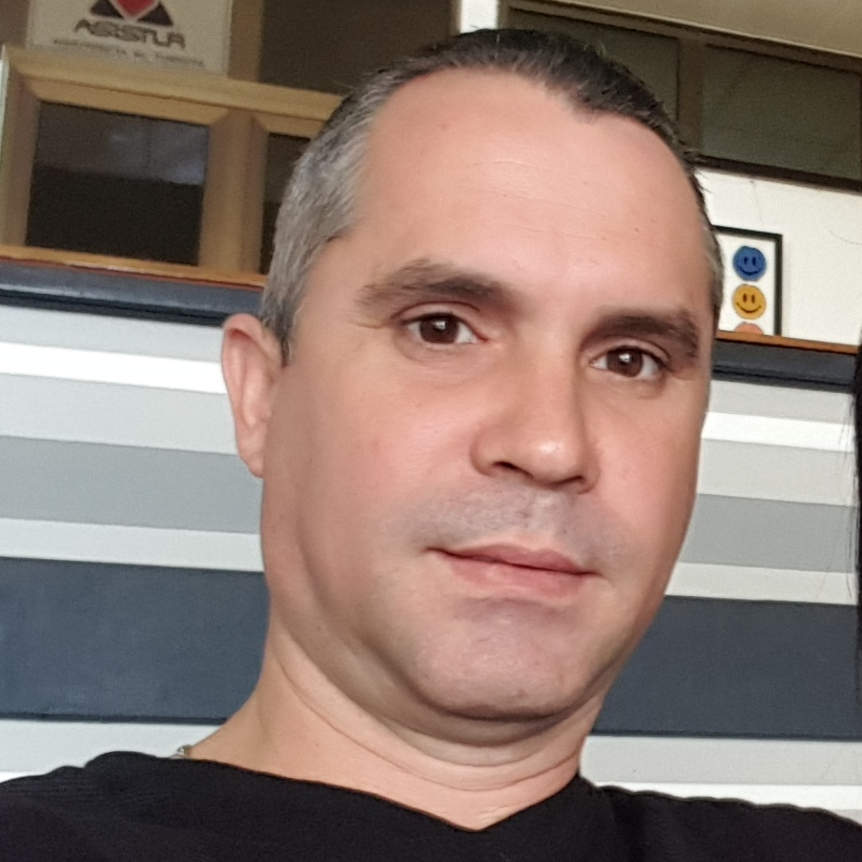
Leodanis is an industrial engineer who loves Python and software development. He's a self-taught Python developer with 6+ years of experience. He's an avid technical writer with a growing number of articles published on Real Python and other sites.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
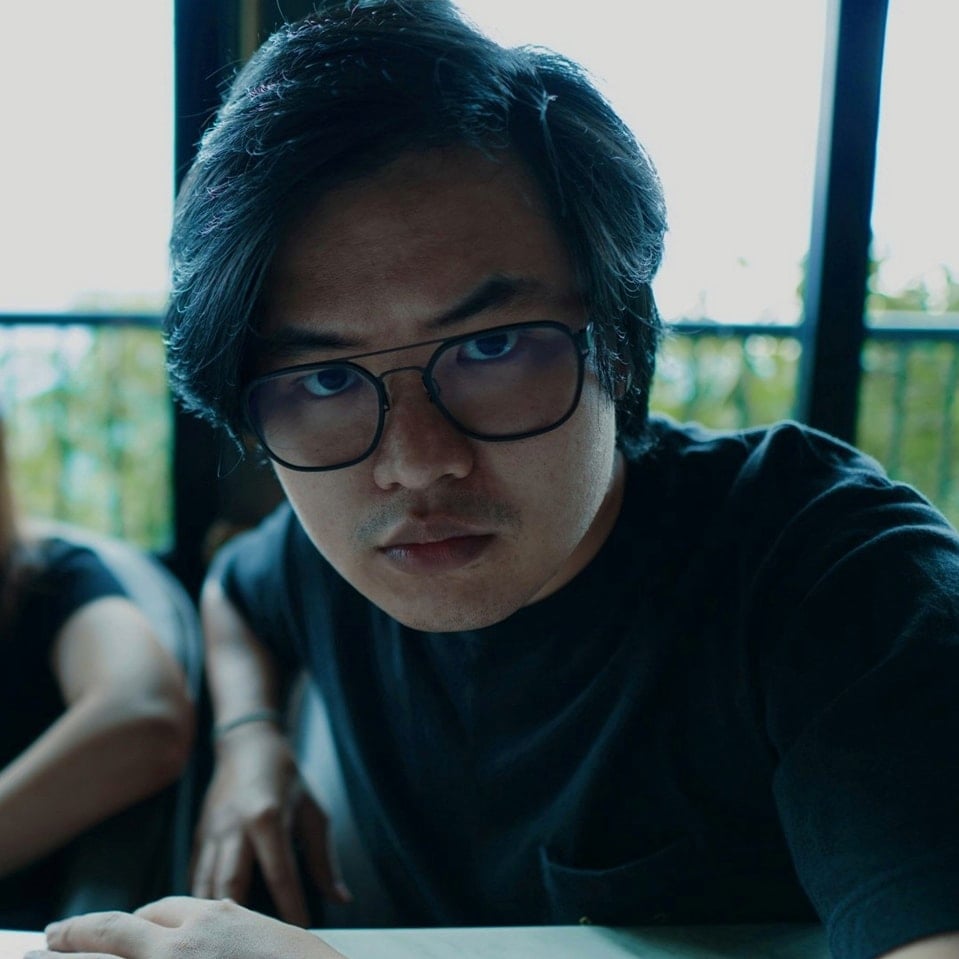
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics projects
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Build a Dice-Rolling App With Python (Source Code)
🔒 No spam. We take your privacy seriously.
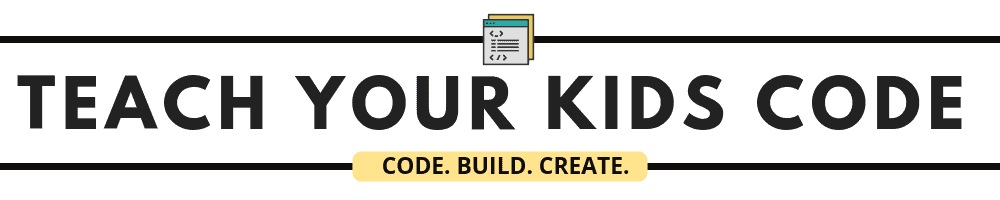
Python Dice Game Tutorial
This post may contain affiliate links. As an Amazon Associate, I earn from qualifying purchases.
Sharing is caring!
In this article, we will walk through how to create a dice game in Python from scratch! If you’re new to Python you can check our beginner tutorial !
How the Game Works
Before we start coding, let’s go over the rules of the game:
- Start with the numbers 1 – 9
- Each turn, the player rolls 2 dies and takes the sum of the numbers
- For example, if the player rolls a 7 they can either remove 7 or 4 and 3
- If the player successfully removes all of the numbers they win!
- If there are no moves that the player can make with the roll then they lose
Python Methods
Let’s first review a couple of methods that we will use in the game. Methods are functions that are built into Python that we can use on certain data structures. For example, there are string methods and list methods . The syntax for methods is a little bit different than using regular functions. Using a string method will look like this:
The data structure that the function is being applied to comes first (string), followed by a dot and then the name of the method. Finally, we end it with any arguments that the function takes in parentheses.
String Methods
One method that we will use is the string split method. The split method splits a string and returns a list with all of the split components. We can specify where we want to split with the argument. Let’s say we have a string s:
Our output will be:
The argument to the method was a string that contained a space, and we can see the string s is split on the spaces.
List Methods
There are two list methods we will use: List.append(element) and List.remove(element)
The append method adds the element you give as the argument to the end of the list:
The remove method removes the element you give as the argument:
Step 1: Importing Libraries
Python has many built-in functions . For example, len() is the built-in function that returns the length of the list. If you want to use other functions outside of what’s already in Python, you can import a library . A library contains functions that you can use once you import it!
For this game, we want to use the random library . The random library provides us with the random.randint() function. This function takes 2 numbers and randomly chooses a number in between them, and we can use this function to simulate rolling the dice. This is how we import the library:
Step 2: Rolling the Dice
Before we jump into coding the main part of the game, there are a couple of things we want to do to make it a little easier. First we need to write a few helper functions . Each helper function will do one small part of the game for us. We will use them in the final larger playGame() function.
First, we want to write a rollDice() function, which will deal with rolling two dice and summing them. We will use the random.randint() function twice, to simulate rolling two die:
We also want to print to the player the results of the roll, as well as the sum:
Finally, we just need to return the sum to use later:
Step 3: Keeping Track of Numbers
When the game begins, we start with the numbers 1-9. During each turn the player will remove some numbers, and we want to keep track of this. We will use a list to keep track of what numbers are still remaining:
We want to write the function removeNums(numList, removeList). It will take two lists as arguments: numList is the current list of remaining numbers, and removeList is a list that will hold all the numbers we want to remove.
The removeList will be generated by player input, so we need to make sure that the player gave a valid set of numbers. This function will destructively modify numList , and it will also return True if the numbers are valid and False if not.
We can start by looping through the numbers we want to remove, removeList :
Next, we want to check if the number is still in the remaining numbers, otherwise, we want to return False since that would be an invalid move.
If all of the numbers are valid, we can return True :
Step 4: Handling User Input
We will be using python’s built-in input() function to get input from the player. We will ask the player to enter what numbers they want removed separated by spaces. For example: “1 2”. The input function always returns a string, even if the user inputs numbers:
Because of this, we need some way to turn this string into a list of integers. We will write the function formatInput(inputString) that takes in the input string and returns the numbers as integers in a list.
The first thing we want to do is use the split function to split the string on the spaces:
Now we have a list with all of the numbers. However, the numbers inside the list will still be strings. We want to loop through the list and use the built in int() function to convert all the strings to ints:
Step 5: Putting it Together
Now we can start writing the main playGame() function. First thing we want to do is initialize the number list:
We want the player to continue to be able to take turns until they either win or lose, we can acomplish this by using a while True loop. This type of loop allows us to keep looping until we manually break out of the loop using a break statement. Python will exit out of the loop as soon as it hits a break statement. This is what our while loop will look like:
Next, we want to print the current numbers to the player and then roll the dice for their turn. We will use the rollDice() helper function that we wrote previously:
Next, we want to get input from the player to see which numbers they want to remove. Once we get the user input, we can use the formatInput(userInput) helper function that we wrote previously to format the input into an integer list.
We now have a list of the numbers the player wants to remove: removeList .
At this point, there’s a chance the user may have given an invalid input, where the numbers that they gave don’t add up to the number that was rolled. If the player gives an invalid input, we will end the game by putting in a break statement. We can check for this using the built-in sum() function to check that the numbers in the removeList add up to the roll:
Now we can try to remove the numbers that the player chose using the removeNums(numList, removeList) helper function that we previously wrote:
The function will return True or False depending on if the player chose numbers that were no longer in the list. We will save this value to a variable validMove . If the move is not valid, we will end the game:
There is one last thing we need to check before we allow the player to start another turn: if there are no remaining numbers, the player has won and the game is over! We can do this by checking the length of numList :
And that’s it! Now we can put everything together and play the game:
Here’s an example of the output of the game:
Congrats on making your own dice game in Python!
Python Dice Game Digital Resource
Do you want all of the material covered in this tutorial in an easy to use in classroom lesson? Grab our Python Dice Game Tutorial!

- Comprehensive Python tutorial for teachers to introduce their students to Python.
- Includes a 5-page PDF worksheet with an answer guide and a 27-slide Google Slides presentation.
- Covers how to program a Dice Game in Python.
- PDF worksheet contains exercises that gradually develop students’ programming skills.
- Google Slides presentation is engaging and visually appealing, with interactive examples and illustrations.
- Suitable for both experienced and new programming instructors.
- Provides a solid foundation in Python programming for students to build on.
- An excellent resource to teach Python in a fun and effective way.
Want all our Python Tutorials?
- The Python Ultimate Bundle is an all-in-one package that includes all the Python tutorials you need to teach your students programming and game development.
- The bundle includes tutorials on Python Basics, Python Lists, creating a story in Python, Rock Paper Scissors game, Fortune Teller game, Create Your Own Adventure game, Blackjack game, and Dice game.
- Each tutorial is engaging, fun, and easy to follow with clear instructions and real-world examples.
- The bundle includes resources such as PDF worksheets, answer guides, and Google Slides presentations to help students learn at their own pace.
- This bundle is perfect for teachers who want to provide their students with a comprehensive introduction to Python programming and game development.
Want More Python?
If you are interested in learning more, check out our advanced Python tutorials here:
- Create a Rock, Paper, Scissors game with Python
- Create a fortune-telling game with Python
- Create a Blackjack game in Python
- Create a Choose Your Own Adventure Game in Python

Nazanin Azimi is a technical writer with several years of experience in the software industry. She is currently a senior at Carnegie Mellon studying computational physics and creative writing. She has been head teaching assistant for Principles of Computing, an introductory python and computer science course at CMU. She has held several technical internships including software engineering intern at Mathworks. Outside of technical writing, Nazanin also enjoys writing and studying poetry.
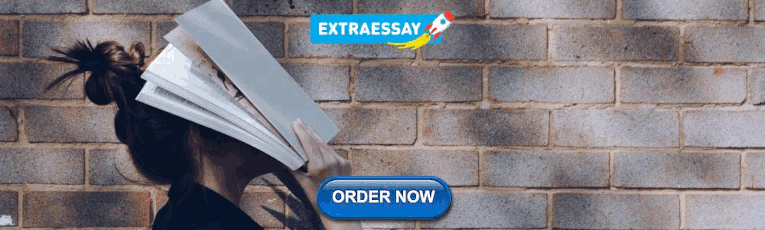
Similar Posts

Python Magic 8 Ball Fortune Teller Game
Let’s get started learning to code a Magic 8 Ball fortune teller game in Python. Today we’ll be showing you how to code this game and reviewing the important coding concepts covered. If you are…

Python For Kids
So You Want to Learn Python (for Kids!) Excellent choice! There are plenty of reasons why learning Python is rising in popularity, but for kids, Python is a great programming language with which to start…

Learn Python with Edison
Want to teach your kids to code in an actual programming language? Learn Python with Edison for an engaging and fun learning experience! If you have never heard of Python, be sure to check out…

Rock, Paper, Scissors Python Game
Are you looking to learn Python by creating a simple rock, paper, scissors game? You’ve come to the right place! In this tutorial we will show you the exact python code needed to create your…

Python Curriculum for Beginners
Python is a popular programming language that has been widely adopted in many industries, including technology, finance, and healthcare. As a result, there is a growing demand for professionals with Python skills. With Python’s intuitive…

Blackjack Python: Beginner Tutorial
In this article we will walk through how to create a blackjack game in Python from scratch! If you’re new to Python you can check our beginner tutorial! Python Blackjack Digital Resource Do you want all…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Last Updated on March 26, 2024 by Kaitlyn Siu
Instantly share code, notes, and snippets.
RyanZurrin / dice_calculator.py
- Download ZIP
- Star ( 0 ) 0 You must be signed in to star a gist
- Fork ( 0 ) 0 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save RyanZurrin/f6dff79b2d2b0b94db8492474640b815 to your computer and use it in GitHub Desktop.
#!/usr/bin/env python3 | |
import numpy | |
import nibabel | |
import argparse | |
def calculate_dice_coefficients(file1, file2): | |
input1_nii = nibabel.load(file1) | |
input2_nii = nibabel.load(file2) | |
voxel_data_1 = input1_nii.get_fdata() | |
voxel_data_2 = input2_nii.get_fdata() | |
voxel_data_1[numpy.isnan(voxel_data_1)] = 0 | |
voxel_data_2[numpy.isnan(voxel_data_2)] = 0 | |
mask_data_1 = numpy.sign(voxel_data_1) | |
mask_data_2 = numpy.sign(voxel_data_2) | |
n_1 = numpy.sum(mask_data_1) | |
n_2 = numpy.sum(mask_data_2) | |
w_1 = numpy.sum(voxel_data_1) | |
w_2 = numpy.sum(voxel_data_2) | |
mask_intersection = numpy.logical_and(mask_data_1, mask_data_2) | |
n_ = numpy.sum(mask_intersection) | |
w_1_ = numpy.sum(voxel_data_1[mask_intersection]) | |
w_2_ = numpy.sum(voxel_data_2[mask_intersection]) | |
dice_standard = 2 * n_ / (n_1 + n_2) | |
dice_weighted = (w_1_ + w_2_) / (w_1 + w_2) | |
return dice_weighted, dice_standard | |
def main(): | |
parser = argparse.ArgumentParser( | |
description="Calculate Dice Coefficients.", | |
epilog="Original logic created by Fan Zhang and converted by Ryan Zurrin" | |
) | |
parser.add_argument("file1", help="Path to the first .nii or .nii.gz file") | |
parser.add_argument( | |
"file2", help="Path to the second .nii or .nii.gz file") | |
args = parser.parse_args() | |
dice_weighted, dice_standard = calculate_dice_coefficients( | |
args.file1, args.file2) | |
print(f" ** wDice = {dice_weighted:.2f}, sDice = {dice_standard:.2f}") | |
if __name__ == "__main__": | |
main() |
Digital Technology
Technology for the 21st century and beyond, creating a dice game in python (part 1), introduction.
In this series of posts we will look at how to code a simple dice game in Python, starting with the initial concept, all the way through to the coding and adding extension features.
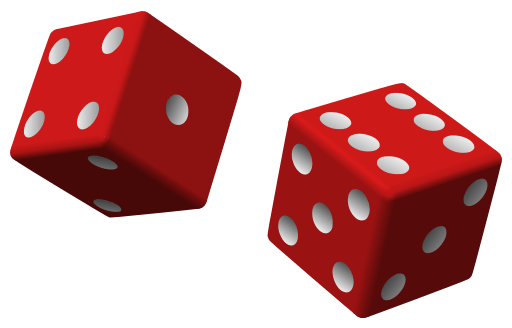
Game rules The players decide upon a winning score, this could be 500 or 1,000. Each player, in turn, throws the dice. Each player rolls and continues to roll the dice until they throw a 7. They score the sum of all the numbers thrown before the 7. Players announce their tally after each throw and once they have thrown a 7, their score is noted on the score sheet. Doubles score double the value. For example, if a player who throws two 4s making eight, their score is 16. The player reaches the pre-arranged total first is the winner.
Iteration 1
Rather than attempting to develop the entire game in one pass, we will develop the game in multiple stages using an iterative development approach . For the first iteration we will get one round of dice rolling completed for a single player.
Before writing any code we will develop a high-level design using pseudocode. This allows us to consider the key logical parts of the algorithmic solution without having to get bogged downed in the finer details of exactly how to code this in Python.
The pseudocode for our proposed first iteration is shown below. The most important part of the pseudocode is that it conveys the intended logic of the problem we are solving. For example the third and fourth lines of pseudocode indicates that a random number between 1 and 6 needs to be generated. How this is done does not matter at this stage – indeed the solution is dependent on the coding language being used, whereas pseudocode should be coding language independent.
The resulting code in Python is almost a line for line translation of the pseudocode. One noteworthy change is the addition of a Boolean-valued variable, valid , which is used to indicate whether all rolls to date are valid (do not add up to 7). The while loop will continue whilst this variable is true. When a total of 7 is rolled the value of the variable becomes false. The use of this loop termination variable is highlighted in the code below. The relationship between the remainder of the code and the pseudocode should hopefully be evident.
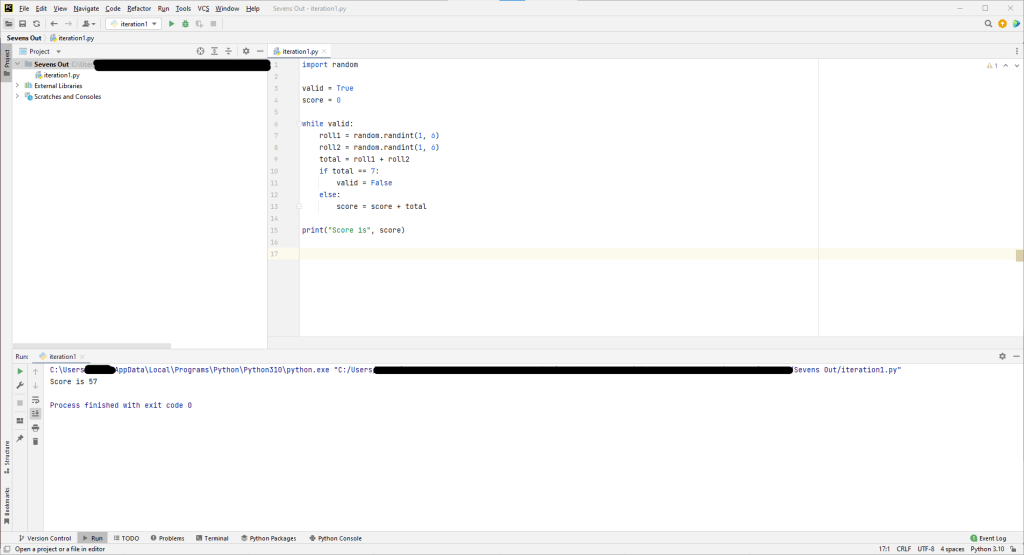
Leave a Reply Cancel reply
Login with:
Your email address will not be published. Required fields are marked *

Subscribe By Email
Get every new post delivered right to your inbox.
Your Email Leave this field blank
This form is protected by reCAPTCHA and the Google Privacy Policy and Terms of Service apply.
- About Edublogs
- Edublogs.org
- Documentation

- Get Started
Join the PyTorch developer community to contribute, learn, and get your questions answered.
Find resources and get questions answered
A place to discuss PyTorch code, issues, install, research
Discover, publish, and reuse pre-trained models
- Edit on GitHub
Dice Score ¶
Functional interface ¶.
Compute dice score from prediction scores.
preds ¶ ( Tensor ) – estimated probabilities
target ¶ ( Tensor ) – ground-truth labels
bg ¶ ( bool ) – whether to also compute dice for the background
nan_score ¶ ( float ) – score to return, if a NaN occurs during computation
no_fg_score ¶ ( float ) – score to return, if no foreground pixel was found in target
a method to reduce metric score over labels.
'elementwise_mean' : takes the mean (default)
'sum' : takes the sum
'none' or None : no reduction will be applied
Tensor containing dice score
- Functional Interface
Access comprehensive developer documentation for PyTorch
Get in-depth tutorials for beginners and advanced developers
Find development resources and get your questions answered
To analyze traffic and optimize your experience, we serve cookies on this site. By clicking or navigating, you agree to allow our usage of cookies. Read PyTorch Lightning's Privacy Policy .
- PyTorch Lightning
- TorchMetrics
- Lightning Flash
- Lightning Transformers
- Lightning Bolts
- Developer Resources
- Models (Beta)
- How it works
- Homework answers
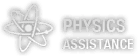
Answer to Question #225578 in Python for Binura Thiranjaya
The game is played as follows:
The game consists of 3 turns , and the aim of the game is to score as many points as possible, Each turn begins with a roll of 6 standard dice,Matching dice (2 or more of the same number) can be set aside for points , and the remaining dice can then be re-rolled in an attempt to score more points 5s Points are “amount of dice * number on dice”, e.g. rolling three 5s is worth 15 points,The player chooses whether to set matching dice aside and whether to re-roll remaining dice, If the player chooses not to re-roll the dice , the points accumulated in the turn are added to their score and the turn ends, If no dice can be put aside after a roll , the player loses the points the potential points and the turn ends – hence, the player must decide how far to push their luck with additional rolls,The game involves quite a lot of luck, but there is also a small amount of strategy in choosing whether to set matching dice aside and whether to re-roll the remaining dice.
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Given a M x N matrix, write a program to print the matrix after ordering all the elements of the mat
- 2. Two Words CombinationGiven a sentence as input, print all the unique combinations of two words in le
- 3. Max Contiguous SubarrayGiven a list of integers, write a program to identify contiguous sub-list tha
- 4. Last half of ListThis Program name is Last half of List. Write a Python program to Last half of List
- 5. First and Last Elements of ListThis Program name is First and Last Elements of List. Write a Python
- 6. List Indexing - 3This Program name is List Indexing - 3. Write a Python program to List Indexing - 3
- 7. Create and Print List -3This Program name is Create and Print List -3. Write a Python program to Cre
- Programming
- Engineering
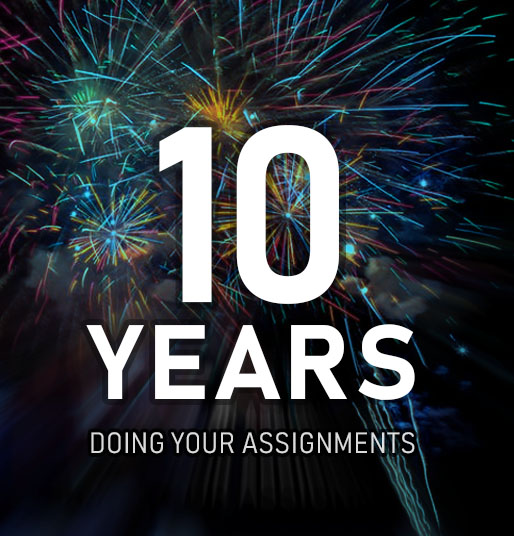
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How calculate the dice coefficient for multi-class segmentation task using Python?
I am wondering how can I calculate the dice coefficient for multi-class segmentation.
Here is the script that would calculate the dice coefficient for the binary segmentation task. How can I loop over each class and calculate the dice for each class?
Thank you in advance
- semantic-segmentation
You can use dice_score for binary classes and then use binary maps for all the classes repeatedly to get a multiclass dice score.
I'm assuming your images/segmentation maps are in the format (batch/index of image, height, width, class_map) .

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged pytorch dice semantic-segmentation or ask your own question .
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- what is wrong with my intuition for the sum of the reciprocals of primes?
- Bottom bracket: What to do about rust in cup + blemish on spindle?
- Function to find the most common numeric ordered pairings (value, count)
- They come in twos
- Will showing disinterest in stakeholder engagement affect my offer of admission?
- when translating a video game's controls into German do I assume a German keyboard?
- What is the reason why creating a veth requires root?
- ~1980 UK TV: very intelligent children recruited for secret project
- ambobus? (a morphologically peculiar adjective with a peculiar syntax here)
- What makes a new chain jump other than a worn cassette?
- Solve an integral analytically
- How can I cover all my skin (face+neck+body) while swimming outside (sea or outdoor pool) to avoid UV radiations?
- Did the United States have consent from Texas to cede a piece of land that was part of Texas?
- The complement of a properly embedded annulus in a handlebody is a handlebody
- 'best poster' and 'best talk' prizes - can we do better?
- Unexpected behaviour during implicit conversion in C
- Density matrices and locality
- Do temperature variations make trains on Mars impractical?
- How to fix a bottom bracket shell that has been cut from outside?
- Can police offer an “immune interview”?
- Is It Possible to Assign Meaningful Amplitudes to Properties in Quantum Mechanics?
- conflict of \counterwithin and cleveref package when sectioncounter is reset
- Venus’ LIP period starts today, can we save the Venusians?
- How do you "stealth" a relativistic superweapon?
Get the Reddit app
Subreddit for posting questions and asking for general advice about your python code.
I cant solve this error in my dice game assignment
Does anyone know why this wont work? I was given a computer science assignment and this is one of the many things I got wrong. I copied the last bit off off stack flow and added my own variable, its for a dice game I'm trying to program where it recognizes if the number just rolled is even and if it is it states so. Please help.
if (round2scoreP2 % 2) == 0:
print(username2"'s score for Round 2 is Even" , format(round2scoreP2))
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
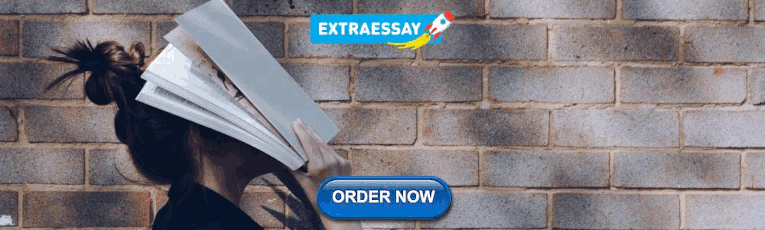
IMAGES
COMMENTS
Python. Question #332118. Dice Score: two friends are playing a dice game, the game is played with five six-sided dice. scores for each type of throws are mentioned below. three 1's = 1000 points. three 6's = 600. three 5's = 500. three 4's = 400. three 3's = 300.
Answers >. Programming & Computer Science >. Python. Question #327962. Dice Score: two friends are playing a dice game, the game is played with five six-sided dice. scores for each type of throws are mentioned below. three 1's = 1000 points. three 6's = 600. three 5's = 500.
Dice Score:two friends are playing a dice game, the game is played with five six-sided dice. scores ; 3. Number of moves:you are given a nxn square chessboard with one bishop and k number of obstacles plac; 4. Ash is now an expert in python function topic. So, he decides to teach others what he knows by makin
xdice is a lightweight python library for managing dice, scores, and dice-notation patterns. Parse almost any Dice Notation pattern: '1d6+1', 'd20', '3d%', '1d20//2 - 2* (6d6+2)', 'max (1d4+1,1d6)', '3D6L2', 'R3 (1d6+1)', '3dF'…etc. API help you to easily manipulate dices, patterns, and scores as objects. A ...
Finish Up the App's Main Code and Roll the Dice. Step 4: Refactor the Code That Generates the Diagram of Dice Faces. Conclusion. Next Steps. Remove ads. Building small projects, like a text-based user interface (TUI) dice-rolling application, will help you level up your Python programming skills.
The game is designed as such that you throw a pair of dice and get money back or loose money. Let's say we start with 5 coins. Throwing a 12 yields 1.5 coins. Throwing an 11 yields 1 coins. Throwing a 10 yields 0.5 coins. Throwing a 9,8 or 7 yields nothing. Throwing a 6,5,4,3,2 or 1 deducts 0.5 coins from your amount of coins.
Dice Game Assignment. In this game, five dice are rolled and the results shown to the player. The player can then choose which dice, if any, to re-roll. After the re-roll, the player must choose which number to score. Each number, 1-6, may be scored a single time. To record the score, add up all the values of the dice containing that number.
If you hit 1 however, all your points will be lost and your game will be over. So to add this together into a small list of components, we will roughly get something like this: Roll die. Check if ...
Step 2: Rolling the Dice. Before we jump into coding the main part of the game, there are a couple of things we want to do to make it a little easier. First we need to write a few helper functions. Each helper function will do one small part of the game for us. We will use them in the final larger playGame () function.
A Python scipt for calculating the dice scores Raw. dice_calculator.py This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters. Learn more about bidirectional Unicode characters ...
Hello again, I am currently struggling with an assignment that requires me to create a casino game. The object of the game is to roll a 7. The game starts by the user entering his starting bid, then the program rolls a random number from the two dice, if the total = 7 then the user gains + $4 to their total pot, however if they don't roll a 7 ...
Each player, in turn, throws the dice. Each player rolls and continues to roll the dice until they throw a 7. They score the sum of all the numbers thrown before the 7. Players announce their tally after each throw and once they have thrown a 7, their score is noted on the score sheet. Doubles score double the value.
Dice Score:two friends are playing a dice game, the game is played with five six-sided dice. scores ; 3. Pager:Imagine a paper with only one button. for the letter "A", you press the button one t; 4. given a matrix of M rows & N columns, you need to find the smallest number in each row & pri; 5. m rows, n columns:input:3,3output:1 2 34 5 67 8 9
About this Guided Project. By the end of this project, you will create a dice game using Python. This course will enable you to take your beginner knowledge in Python and apply that knowledge to a project and take that knowledge to the next level. This beginner tutorial will take you through creating a simple dice application with two players.
Compute dice score from prediction scores. Parameters. preds ( Tensor) - estimated probabilities. target ( Tensor) - ground-truth labels. bg ( bool) - whether to also compute dice for the background. nan_score ( float) - score to return, if a NaN occurs during computation. no_fg_score ( float) - score to return, if no foreground pixel ...
Python. Question #225578. The game is played as follows: The game consists of 3 turns, and the aim of the game is to score as many points as possible, Each turn begins with a roll of 6 standard dice,Matching dice (2 or more of the same number) can be set aside for points, and the remaining dice can then be re-rolled in an attempt to score more ...
Here are 3 alternatives for getting the Dice coefficient in Python using raw Numpy, Scipy, and Scikit-Image. Hopefully comparing these can provide some illumination on how the Dice coefficient works and how it is related to other methods...
You can use dice_score for binary classes and then use binary maps for all the classes repeatedly to get a multiclass dice score. I'm assuming your images/segmentation maps are in the format (batch/index of image, height, width, class_map).. import numpy as np import matplotlib.pyplot as plt def dice_coef(y_true, y_pred): y_true_f = y_true.flatten() y_pred_f = y_pred.flatten() intersection ...
This game needs to be in Python. Assignment Description This program will simulate part of the game of Yahtzee! This is a dice game that involves rolling five dice and scoring points based on what show up on those five dice. The players would record their scores on a score card, and then total them up, and the player with the larger total wins ...
Does anyone know why this wont work? I was given a computer science assignment and this is one of the many things I got wrong. I copied the last bit…