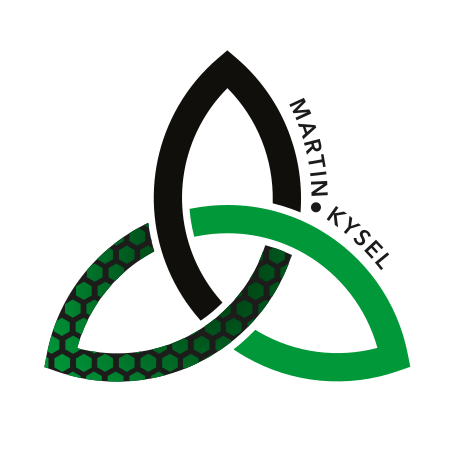
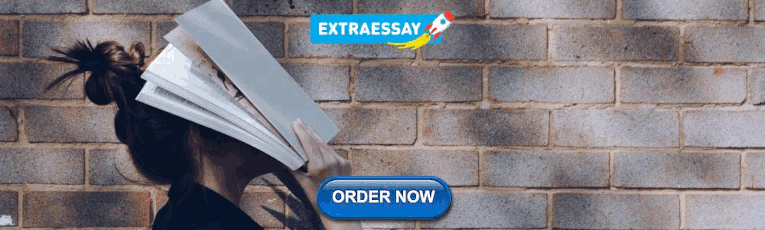
Martin Kysel
Coding Challenges and More
HackerRank 'Lily's Homework' Solution
Martin Kysel · September 21, 2018
Short Problem Definition:
Whenever George asks Lily to hang out, she’s busy doing homework. George wants to help her finish it faster, but he’s in over his head! Can you help George understand Lily’s homework so she can hang out with him?
Consider an array of m distinct integers, arr = [a[0], a[1], …, a[n-1]]. George can swap any two elements of the array any number of times. An array is if the sum of | a[i] - a[i-1] among 0 < i < n is minimal. |
Lily’s Homework
Complexity:
time complexity is O(N\*log(N))
space complexity is O(N)
Let us rephrase the problem to a sorting problem: Find the number of swaps to make the array sorted. We need to consider both ascending and descending arrays. The solution assumes no duplicates.
Share: Twitter , Facebook
To learn more about solving Coding Challenges in Python, I recommend these courses: Educative.io Python Algorithms , Educative.io Python Coding Interview .
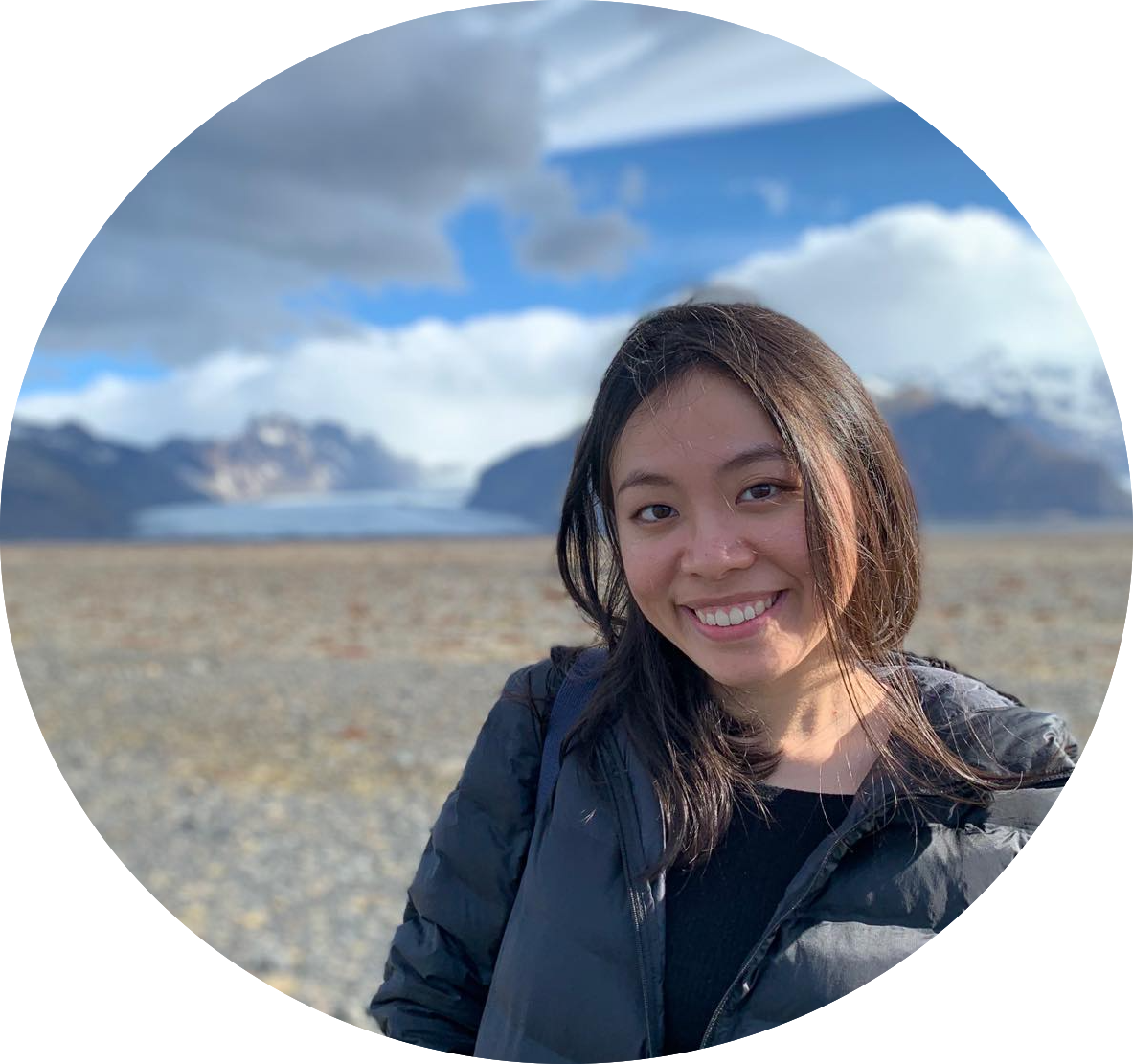
Solutions to HackerRank Coding Challenges in Python
One of the best ways to absorb and retain new concepts that I have learnt about coding in Python is through documentation! As part of my journey to future-proof my data career and also to improve my proficiency in the python language, I have spent some of my free time to solve coding challenges on HackerRank . In this blog post, I shared the solutions to some of the practice challenges which I have completed and successfully passed the code in the compiler on HackerRank . Do click on Read More or the title of this blog post for the Python 3 solutions which I have created.
Arithmetic Operators
Print function, write a function, python introduction (easy) questions.
Given an integer, n , perform the following conditional actions:
- If n is odd, print Weird
- If n is even and in the inclusive range of 2 to 5 , print Not Weird
- If n is even and in the inclusive range of 6 to 20 , print Weird
- If n is even and greater than 20 , print Not Weird
Input Format: A single line containing a positive integer, n .
Constraints:
- 1 <= n <= 100
Output Format: Print Weird if the number is weird. Otherwise, print Not Weird .
The provided code stub reads two integers from STDIN, a and b . Add code to print three lines where:
- The first line contains the sum of the two numbers.
- The second line contains the difference of the two numbers (first - second).
- The third line contains the product of the two numbers.
Print the following:
Input Format: The first line contains the first integer, a . The second line contains the second integer, b .
Output Format: Print the three lines as explained above.
Sample Input 0
Sample Output 0
The provided code stub reads two integers from STDIN, a and b . Add logic to print two lines. The first line should contain the result of integer division, a//b . The second line should contain the result of float division, a/b .
No rounding or formatting is necessary.
- The result of the integer division 3//5 = 0 .
- The result of the float division is 3/5 = 0.6 .
Input Format
The first line contains the first integer, a . The second line contains the second integer, b .
Output Format
Print the two lines as described above.
The provided code stub reads and integer, n , from STDIN. For all non-negative integers i < n , print i**2 .
The list of non-negative integers that are less than n = 3 is [0,1,2] . Print the square of each number on a separate line.
Input Format: The first and only line contains the integer, n .
Constraints: 1 <= n <= 20
Output Format: Print n lines, one corresponding to each i .
The included code stub will read an integer, n , from STDIN.
Without using any string methods, try to print the following: 123 . . . n
Note that “…” represents the consecutive values in between.
Example n=5
Print the string 12345 .
Input Format: The first line contains an integer n .
Constraints: 1 <= n <= 150
Output Format: Print the list of integers from 1 through n as a string, without spaces.
Python Introduction (Medium) Questions
An extra day is added to the calendar almost every four years as February 29, and the day is called a leap day. It corrects the calendar for the fact that our planet takes approximately 365.25 days to orbit the sun. A leap year contains a leap day.
In the Gregorian calendar, three conditions are used to identify leap years:
- The year can be evenly divided by 100, it is NOT a leap year, unless:
- The year is also evenly divisible by 400. Then it is a leap year. This means that in the Gregorian calendar, the years 2000 and 2400 are leap years, while 1800, 1900, 2100, 2200, 2300 and 2500 are NOT leap years.
Given a year, determine whether it is a leap year. If it is a leap year, return the Boolean True , otherwise return False .
Note that the code stub provided reads from STDIN and passes arguments to the is_leap function. It is only necessary to complete the is_leap function.
Input Format: Read year , the year to test.
Constraints
1900 <= year <= 10 ** 5
Output Format: The function must return a Boolean value (True/False). Output is handled by the provided code stub.
Explanation 0
1990 is not a multiple of 4 hence it’s not a leap year.
Coding Made Simple
Array Mathematics in Python | HackerRank Solution
Hello coders, today we are going to solve Array Mathematics HackerRank Solution in Python .
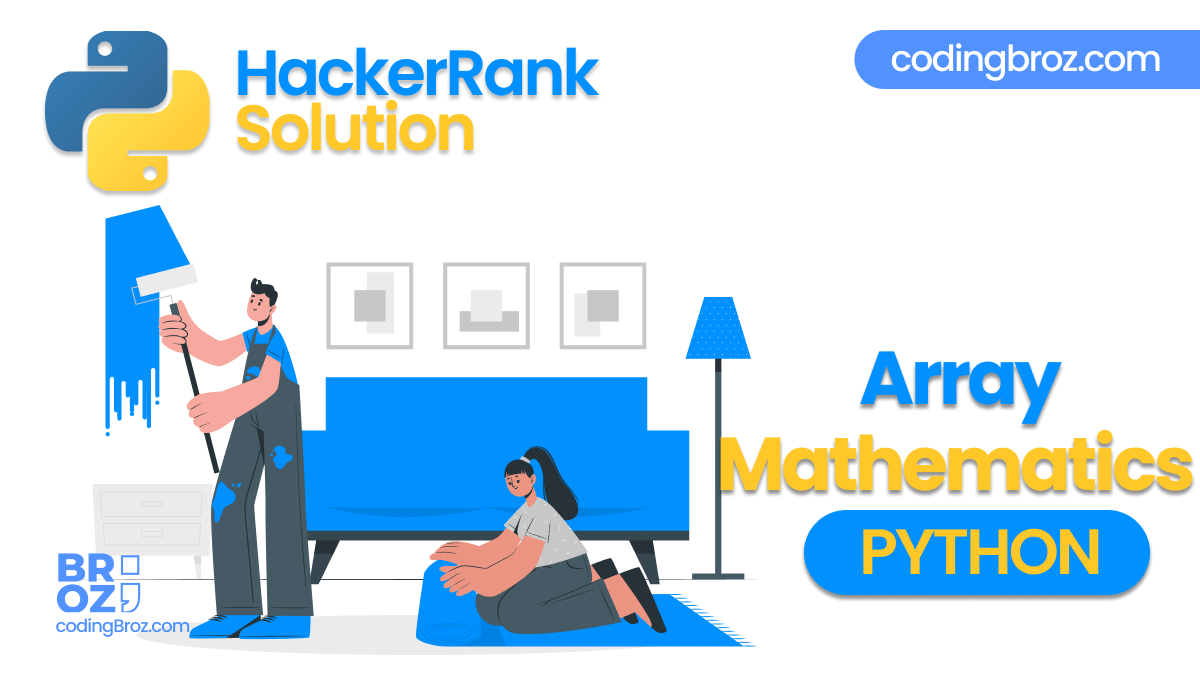
Basic mathematical functions operate element-wise on arrays. They are available both as operator overloads and as functions in the NumPy module.
You are given two integer arrays, A and B of dimensions N X M . Your task is to perform the following operations:
- Add ( A + B )
- Subtract ( A – B )
- Multiply ( A * B )
- Integer Division ( A / B )
- Mod ( A % B )
- Power ( A ** B )
Note There is a method numpy.floor_divide() that works like numpy.divide() except it performs a floor division.
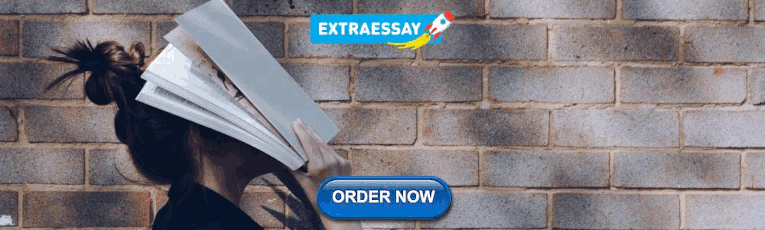
Input Format
The first line contains two space separated integers, N and M . The next N lines contains M space separated integers of array . The following N lines contains M space separated integers of array B .
Output Format
Print the result of each operation in the given order under Task .
Sample Input
Sample Output
Use // for division in Python 3.
Solution – Array Mathematics in Python
Disclaimer: The above Problem ( Array Mathematics ) is generated by Hacker Rank but the Solution is Provided by CodingBroz . This tutorial is only for Educational and Learning Purpose.
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
drawing-book.py
Latest commit, file metadata and controls.
Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.19%
Python if-else easy python (basic) max score: 10 success rate: 89.63%, arithmetic operators easy python (basic) max score: 10 success rate: 97.35%, python: division easy python (basic) max score: 10 success rate: 98.67%, loops easy python (basic) max score: 10 success rate: 98.07%, write a function medium python (basic) max score: 10 success rate: 90.28%, print function easy python (basic) max score: 20 success rate: 97.30%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
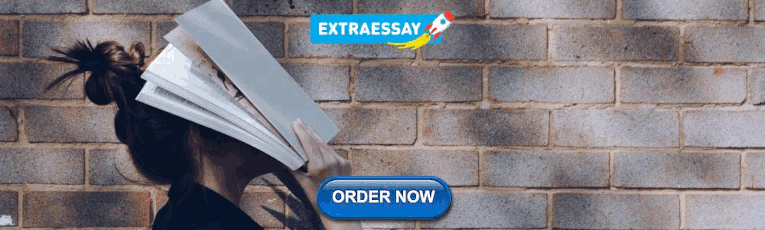
COMMENTS
In this post, we will solve Lily's Homework HackerRank Solution. This problem (Lily's Homework) is a part of HackerRank Problem Solving series. ... Python import math import os import random import re import sys # # Complete the 'lilysHomework' function below. # # The function is expected to return an INTEGER.
Function Description Complete the lilysHomework function in the editor below. lilysHomework has the following parameter (s): int arr [n]: an integer array Returns int: the minimum number of swaps required Input Format The first line contains a single integer, n, the number of elements in arr.
Lily's Homework. Complexity: time complexity is O(N\*log(N)) space complexity is O(N) Execution: Let us rephrase the problem to a sorting problem: Find the number of swaps to make the array sorted. We need to consider both ascending and descending arrays. The solution assumes no duplicates. Solution:
Solutions to Problems from the Hackerrank Math 🧮 Domain. - anishLearnsToCode/hackerrank-math
Function Description. Complete the lilysHomework function in the editor below. lilysHomework has the following parameter (s): int arr [n]: an integer array. Returns. int: the minimum number of swaps required. Input Format. The first line contains a single integer, , the number of elements in . The second line contains space-separated integers, .
A collection of solutions to competitive programming exercises on HackerRank. - kilian-hu/hackerrank-solutions
Mean, Var and Std - Hacker Rank Solution. Dot and Cross - Hacker Rank Solution. Inner and Outer - Hacker Rank Solution. Polynomials - Hacker Rank Solution. Linear Algebra - Hacker Rank Solution. Disclaimer: The above Python Problems are generated by Hacker Rank but the Solutions are Provided by CodingBroz.
Easy Python (Basic) Max Score: 10 Success Rate: 89.63%. Solve Challenge. ... Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... Math. Itertools. Collections. Date and Time. Errors and Exceptions. Classes. Built-Ins. Python Functionals.
In this blog post, I shared the solutions to some of the practice challenges which I have completed and successfully passed the code in the compiler on HackerRank. Do click on Read More or the title of this blog post for the Python 3 solutions which I have created. Python Introduction (Easy) Questions. If-Else; Arithmetic Operators; Division; Loops
Hello coders, today we are going to solve Array Mathematics HackerRank Solution in Python. Table of Contents. Objective; Task; Input Format; Output Format; Solution - Array Mathematics in Python; Objective. Basic mathematical functions operate element-wise on arrays.
Students have been assigned a series of math problems - LeetCode Discuss. Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
1. Create an object named "pair" that contains the value of the element and its primary index position. 2. Get all the pair in a list, sort that list with customized comparator. 3. Traverse through the pair list, using the graph theory to identify circles consisting the group of element need to be swapped. 4. Specify the number of swaps.
This Solution set is authored by myself when I was solving some of Mathematics problems, It is not a complete set - A work in Progress. If you have a code with better time complexity feel free to give a PR and also feel free to contribute.
This video contains solution to HackerRank "Triangle Quest 2" problem. But remember...before looking at the solution you need to try the problem once for bui...
#tarun'sCodehub #Python #hackerranksolutions #codingtutorial In this video we have see that how to take input and arithmetic operatorsPython tutorials Play...
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Mathematics. Find the Point. Easy Problem Solving (Basic) Max Score: 5 Success Rate: 88.49%. Solve Challenge. Maximum Draws. ... Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. We use cookies to ensure you have the best browsing experience on our website. ...
This problem has been solved! You'll get a detailed solution from a subject matter expert that helps you learn core concepts. See Answer See Answer See Answer done loading
Code your solution in our custom editor or code in your own environment and upload your solution as a file. 4 of 6; Test your code You can compile your code and test it for errors and accuracy before submitting. 5 of 6; Submit to see results When you're ready, submit your solution! Remember, you can go back and refine your code anytime. 6 of 6
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
drawing-book.py. Cannot retrieve latest commit at this time. History. Code. Blame. 34 lines (25 loc) · 591 Bytes. #!/bin/python3 import math import os import random import re import sys # # Complete the 'pageCount' function below. # # The function is expected to return an INTEGER.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... Easy Python (Basic) Max Score: 10 Success Rate: 96.57%. Solve Challenge. ... Math. Itertools. Collections. Date and Time. Errors and Exceptions. Classes. Built-Ins. Python Functionals. Regex and Parsing. XML.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... Easy Python (Basic) Max Score: 10 Success Rate: 89.64%. Solve Challenge. Arithmetic Operators. ... Math. Itertools. Collections. Date and Time. Errors and Exceptions. Classes. Built-Ins. Python Functionals.