Was this page helpful?
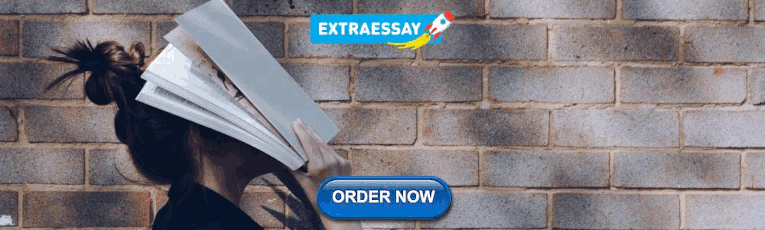
Variable Declaration
let and const are two relatively new concepts for variable declarations in JavaScript. As we mentioned earlier , let is similar to var in some respects, but allows users to avoid some of the common “gotchas” that users run into in JavaScript.
const is an augmentation of let in that it prevents re-assignment to a variable.
With TypeScript being an extension of JavaScript, the language naturally supports let and const . Here we’ll elaborate more on these new declarations and why they’re preferable to var .
If you’ve used JavaScript offhandedly, the next section might be a good way to refresh your memory. If you’re intimately familiar with all the quirks of var declarations in JavaScript, you might find it easier to skip ahead.
var declarations
Declaring a variable in JavaScript has always traditionally been done with the var keyword.
As you might’ve figured out, we just declared a variable named a with the value 10 .
We can also declare a variable inside of a function:
and we can also access those same variables within other functions:
In this above example, g captured the variable a declared in f . At any point that g gets called, the value of a will be tied to the value of a in f . Even if g is called once f is done running, it will be able to access and modify a .
Scoping rules
var declarations have some odd scoping rules for those used to other languages. Take the following example:
Some readers might do a double-take at this example. The variable x was declared within the if block , and yet we were able to access it from outside that block. That’s because var declarations are accessible anywhere within their containing function, module, namespace, or global scope - all which we’ll go over later on - regardless of the containing block. Some people call this var -scoping or function-scoping . Parameters are also function scoped.
These scoping rules can cause several types of mistakes. One problem they exacerbate is the fact that it is not an error to declare the same variable multiple times:
Maybe it was easy to spot out for some experienced JavaScript developers, but the inner for -loop will accidentally overwrite the variable i because i refers to the same function-scoped variable. As experienced developers know by now, similar sorts of bugs slip through code reviews and can be an endless source of frustration.
Variable capturing quirks
Take a quick second to guess what the output of the following snippet is:
For those unfamiliar, setTimeout will try to execute a function after a certain number of milliseconds (though waiting for anything else to stop running).
Ready? Take a look:
Many JavaScript developers are intimately familiar with this behavior, but if you’re surprised, you’re certainly not alone. Most people expect the output to be
Remember what we mentioned earlier about variable capturing? Every function expression we pass to setTimeout actually refers to the same i from the same scope.
Let’s take a minute to consider what that means. setTimeout will run a function after some number of milliseconds, but only after the for loop has stopped executing; By the time the for loop has stopped executing, the value of i is 10 . So each time the given function gets called, it will print out 10 !
A common work around is to use an IIFE - an Immediately Invoked Function Expression - to capture i at each iteration:
This odd-looking pattern is actually pretty common. The i in the parameter list actually shadows the i declared in the for loop, but since we named them the same, we didn’t have to modify the loop body too much.
let declarations
By now you’ve figured out that var has some problems, which is precisely why let statements were introduced. Apart from the keyword used, let statements are written the same way var statements are.
The key difference is not in the syntax, but in the semantics, which we’ll now dive into.
Block-scoping
When a variable is declared using let , it uses what some call lexical-scoping or block-scoping . Unlike variables declared with var whose scopes leak out to their containing function, block-scoped variables are not visible outside of their nearest containing block or for -loop.
Here, we have two local variables a and b . a ’s scope is limited to the body of f while b ’s scope is limited to the containing if statement’s block.
Variables declared in a catch clause also have similar scoping rules.
Another property of block-scoped variables is that they can’t be read or written to before they’re actually declared. While these variables are “present” throughout their scope, all points up until their declaration are part of their temporal dead zone . This is just a sophisticated way of saying you can’t access them before the let statement, and luckily TypeScript will let you know that.
Something to note is that you can still capture a block-scoped variable before it’s declared. The only catch is that it’s illegal to call that function before the declaration. If targeting ES2015, a modern runtime will throw an error; however, right now TypeScript is permissive and won’t report this as an error.
For more information on temporal dead zones, see relevant content on the Mozilla Developer Network .
Re-declarations and Shadowing
With var declarations, we mentioned that it didn’t matter how many times you declared your variables; you just got one.
In the above example, all declarations of x actually refer to the same x , and this is perfectly valid. This often ends up being a source of bugs. Thankfully, let declarations are not as forgiving.
The variables don’t necessarily need to both be block-scoped for TypeScript to tell us that there’s a problem.
That’s not to say that a block-scoped variable can never be declared with a function-scoped variable. The block-scoped variable just needs to be declared within a distinctly different block.
The act of introducing a new name in a more nested scope is called shadowing . It is a bit of a double-edged sword in that it can introduce certain bugs on its own in the event of accidental shadowing, while also preventing certain bugs. For instance, imagine we had written our earlier sumMatrix function using let variables.
This version of the loop will actually perform the summation correctly because the inner loop’s i shadows i from the outer loop.
Shadowing should usually be avoided in the interest of writing clearer code. While there are some scenarios where it may be fitting to take advantage of it, you should use your best judgement.
Block-scoped variable capturing
When we first touched on the idea of variable capturing with var declaration, we briefly went into how variables act once captured. To give a better intuition of this, each time a scope is run, it creates an “environment” of variables. That environment and its captured variables can exist even after everything within its scope has finished executing.
Because we’ve captured city from within its environment, we’re still able to access it despite the fact that the if block finished executing.
Recall that with our earlier setTimeout example, we ended up needing to use an IIFE to capture the state of a variable for every iteration of the for loop. In effect, what we were doing was creating a new variable environment for our captured variables. That was a bit of a pain, but luckily, you’ll never have to do that again in TypeScript.
let declarations have drastically different behavior when declared as part of a loop. Rather than just introducing a new environment to the loop itself, these declarations sort of create a new scope per iteration . Since this is what we were doing anyway with our IIFE, we can change our old setTimeout example to just use a let declaration.
and as expected, this will print out
const declarations
const declarations are another way of declaring variables.
They are like let declarations but, as their name implies, their value cannot be changed once they are bound. In other words, they have the same scoping rules as let , but you can’t re-assign to them.
This should not be confused with the idea that the values they refer to are immutable .
Unless you take specific measures to avoid it, the internal state of a const variable is still modifiable. Fortunately, TypeScript allows you to specify that members of an object are readonly . The chapter on Interfaces has the details.
let vs. const
Given that we have two types of declarations with similar scoping semantics, it’s natural to find ourselves asking which one to use. Like most broad questions, the answer is: it depends.
Applying the principle of least privilege , all declarations other than those you plan to modify should use const . The rationale is that if a variable didn’t need to get written to, others working on the same codebase shouldn’t automatically be able to write to the object, and will need to consider whether they really need to reassign to the variable. Using const also makes code more predictable when reasoning about flow of data.
Use your best judgement, and if applicable, consult the matter with the rest of your team.
The majority of this handbook uses let declarations.
Destructuring
Another ECMAScript 2015 feature that TypeScript has is destructuring. For a complete reference, see the article on the Mozilla Developer Network . In this section, we’ll give a short overview.
Array destructuring
The simplest form of destructuring is array destructuring assignment:
This creates two new variables named first and second . This is equivalent to using indexing, but is much more convenient:
Destructuring works with already-declared variables as well:
And with parameters to a function:
You can create a variable for the remaining items in a list using the syntax ... :
Of course, since this is JavaScript, you can just ignore trailing elements you don’t care about:
Or other elements:
Tuple destructuring
Tuples may be destructured like arrays; the destructuring variables get the types of the corresponding tuple elements:
It’s an error to destructure a tuple beyond the range of its elements:
As with arrays, you can destructure the rest of the tuple with ... , to get a shorter tuple:
Or ignore trailing elements, or other elements:
Object destructuring
You can also destructure objects:
This creates new variables a and b from o.a and o.b . Notice that you can skip c if you don’t need it.
Like array destructuring, you can have assignment without declaration:
Notice that we had to surround this statement with parentheses. JavaScript normally parses a { as the start of block.
You can create a variable for the remaining items in an object using the syntax ... :
Property renaming
You can also give different names to properties:
Here the syntax starts to get confusing. You can read a: newName1 as ” a as newName1 ”. The direction is left-to-right, as if you had written:
Confusingly, the colon here does not indicate the type. The type, if you specify it, still needs to be written after the entire destructuring:
Default values
Default values let you specify a default value in case a property is undefined:
In this example the b? indicates that b is optional, so it may be undefined . keepWholeObject now has a variable for wholeObject as well as the properties a and b , even if b is undefined.
Function declarations
Destructuring also works in function declarations. For simple cases this is straightforward:
But specifying defaults is more common for parameters, and getting defaults right with destructuring can be tricky. First of all, you need to remember to put the pattern before the default value.
The snippet above is an example of type inference, explained earlier in the handbook.
Then, you need to remember to give a default for optional properties on the destructured property instead of the main initializer. Remember that C was defined with b optional:
Use destructuring with care. As the previous example demonstrates, anything but the simplest destructuring expression is confusing. This is especially true with deeply nested destructuring, which gets really hard to understand even without piling on renaming, default values, and type annotations. Try to keep destructuring expressions small and simple. You can always write the assignments that destructuring would generate yourself.
The spread operator is the opposite of destructuring. It allows you to spread an array into another array, or an object into another object. For example:
This gives bothPlus the value [0, 1, 2, 3, 4, 5] . Spreading creates a shallow copy of first and second . They are not changed by the spread.
You can also spread objects:
Now search is { food: "rich", price: "$$", ambiance: "noisy" } . Object spreading is more complex than array spreading. Like array spreading, it proceeds from left-to-right, but the result is still an object. This means that properties that come later in the spread object overwrite properties that come earlier. So if we modify the previous example to spread at the end:
Then the food property in defaults overwrites food: "rich" , which is not what we want in this case.
Object spread also has a couple of other surprising limits. First, it only includes an objects’ own, enumerable properties . Basically, that means you lose methods when you spread instances of an object:
Second, the TypeScript compiler doesn’t allow spreads of type parameters from generic functions. That feature is expected in future versions of the language.
using declarations
using declarations are an upcoming feature for JavaScript that are part of the Stage 3 Explicit Resource Management proposal. A using declaration is much like a const declaration, except that it couples the lifetime of the value bound to the declaration with the scope of the variable.
When control exits the block containing a using declaration, the [Symbol.dispose]() method of the declared value is executed, which allows that value to perform cleanup:
At runtime, this has an effect roughly equivalent to the following:
using declarations are extremely useful for avoiding memory leaks when working with JavaScript objects that hold on to native references like file handles
or scoped operations like tracing
Unlike var , let , and const , using declarations do not support destructuring.
null and undefined
It’s important to note that the value can be null or undefined , in which case nothing is disposed at the end of the block:
which is roughly equivalent to:
This allows you to conditionally acquire resources when declaring a using declaration without the need for complex branching or repetition.
Defining a disposable resource
You can indicate the classes or objects you produce are disposable by implementing the Disposable interface:
await using declarations
Some resources or operations may have cleanup that needs to be performed asynchronously. To accommodate this, the Explicit Resource Management proposal also introduces the await using declaration:
An await using declaration invokes, and awaits , its value’s [Symbol.asyncDispose]() method as control leaves the containing block. This allows for asynchronous cleanup, such as a database transaction performing a rollback or commit, or a file stream flushing any pending writes to storage before it is closed.
As with await , await using can only be used in an async function or method, or at the top level of a module.
Defining an asynchronously disposable resource
Just as using relies on objects that are Disposable , an await using relies on objects that are AsyncDisposable :
await using vs await
The await keyword that is part of the await using declaration only indicates that the disposal of the resource is await -ed. It does not await the value itself:
await using and return
It’s important to note that there is a small caveat with this behavior if you are using an await using declaration in an async function that returns a Promise without first await -ing it:
Because the returned promise isn’t await -ed, it’s possible that the JavaScript runtime may report an unhandled rejection since execution pauses while await -ing the asynchronous disposal of x , without having subscribed to the returned promise. This is not a problem that is unique to await using , however, as this can also occur in an async function that uses try..finally :
To avoid this situation, it is recommended that you await your return value if it may be a Promise :
using and await using in for and for..of statements
Both using and await using can be used in a for statement:
In this case, the lifetime of x is scoped to the entire for statement and is only disposed when control leaves the loop due to break , return , throw , or when the loop condition is false.
In addition to for statements, both declarations can also be used in for..of statements:
Here, x is disposed at the end of each iteration of the loop , and is then reinitialized with the next value. This is especially useful when consuming resources produced one at a time by a generator.
using and await using in older runtimes
using and await using declarations can be used when targeting older ECMAScript editions as long as you are using a compatible polyfill for Symbol.dispose / Symbol.asyncDispose , such as the one provided by default in recent editions of NodeJS.
Nightly Builds
How to use a nightly build of TypeScript
The TypeScript docs are an open source project. Help us improve these pages by sending a Pull Request ❤
Last updated: Aug 15, 2024
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Destructuring assignment
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax but uses it on the left-hand side of the assignment instead. It defines which values to unpack from the sourced variable.
Similarly, you can destructure objects on the left-hand side of the assignment.
This capability is similar to features present in languages such as Perl and Python.
For features specific to array or object destructuring, refer to the individual examples below.
Binding and assignment
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern , with slightly different syntaxes.
In binding patterns, the pattern starts with a declaration keyword ( var , let , or const ). Then, each individual property must either be bound to a variable or further destructured.
All variables share the same declaration, so if you want some variables to be re-assignable but others to be read-only, you may have to destructure twice — once with let , once with const .
In many other syntaxes where the language binds a variable for you, you can use a binding destructuring pattern. These include:
- The looping variable of for...in for...of , and for await...of loops;
- Function parameters;
- The catch binding variable.
In assignment patterns, the pattern does not start with a keyword. Each destructured property is assigned to a target of assignment — which may either be declared beforehand with var or let , or is a property of another object — in general, anything that can appear on the left-hand side of an assignment expression.
Note: The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{ a, b } = { a: 1, b: 2 } is not valid stand-alone syntax, as the { a, b } on the left-hand side is considered a block and not an object literal according to the rules of expression statements . However, ({ a, b } = { a: 1, b: 2 }) is valid, as is const { a, b } = { a: 1, b: 2 } .
If your coding style does not include trailing semicolons, the ( ... ) expression needs to be preceded by a semicolon, or it may be used to execute a function on the previous line.
Note that the equivalent binding pattern of the code above is not valid syntax:
You can only use assignment patterns as the left-hand side of the assignment operator. You cannot use them with compound assignment operators such as += or *= .
Default value
Each destructured property can have a default value . The default value is used when the property is not present, or has value undefined . It is not used if the property has value null .
The default value can be any expression. It will only be evaluated when necessary.
Rest property
You can end a destructuring pattern with a rest property ...rest . This pattern will store all remaining properties of the object or array into a new object or array.
The rest property must be the last in the pattern, and must not have a trailing comma.
Array destructuring
Basic variable assignment, destructuring with more elements than the source.
In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N , only the first N variables are assigned values. The values of the remaining variables will be undefined.
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Using a binding pattern as the rest property
The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after collecting the rest elements, so you cannot access any properties present on the original iterable in this way.
These binding patterns can even be nested, as long as each rest property is the last in the list.
On the other hand, object destructuring can only have an identifier as the rest property.
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Using array destructuring on any iterable
Array destructuring calls the iterable protocol of the right-hand side. Therefore, any iterable, not necessarily arrays, can be destructured.
Non-iterables cannot be destructured as arrays.
Iterables are only iterated until all bindings are assigned.
The rest binding is eagerly evaluated and creates a new array, instead of using the old iterable.
Object destructuring
Basic assignment, assigning to new variable names.
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo .
Assigning to new variable names and providing default values
A property can be both
- Unpacked from an object and assigned to a variable with a different name.
- Assigned a default value in case the unpacked value is undefined .
Unpacking properties from objects passed as a function parameter
Objects passed into function parameters can also be unpacked into variables, which may then be accessed within the function body. As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property.
Consider this object, which contains information about a user.
Here we show how to unpack a property of the passed object into a variable with the same name. The parameter value { id } indicates that the id property of the object passed to the function should be unpacked into a variable with the same name, which can then be used within the function.
You can define the name of the unpacked variable. Here we unpack the property named displayName , and rename it to dname for use within the function body.
Nested objects can also be unpacked. The example below shows the property fullname.firstName being unpacked into a variable called name .
Setting a function parameter's default value
Default values can be specified using = , and will be used as variable values if a specified property does not exist in the passed object.
Below we show a function where the default size is 'big' , default co-ordinates are x: 0, y: 0 and default radius is 25.
In the function signature for drawChart above, the destructured left-hand side has a default value of an empty object = {} .
You could have also written the function without that default. However, if you leave out that default value, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can call drawChart() without supplying any parameters. Otherwise, you need to at least supply an empty object literal.
For more information, see Default parameters > Destructured parameter with default value assignment .
Nested object and array destructuring
For of iteration and destructuring, computed object property names and destructuring.
Computed property names, like on object literals , can be used with destructuring.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifier that is valid.
Destructuring primitive values
Object destructuring is almost equivalent to property accessing . This means if you try to destruct a primitive value, the value will get wrapped into the corresponding wrapper object and the property is accessed on the wrapper object.
Same as accessing properties, destructuring null or undefined throws a TypeError .
This happens even when the pattern is empty.
Combined array and object destructuring
Array and object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
The prototype chain is looked up when the object is deconstructed
When deconstructing an object, if a property is not accessed in itself, it will continue to look up along the prototype chain.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators
- ES6 in Depth: Destructuring on hacks.mozilla.org (2015)
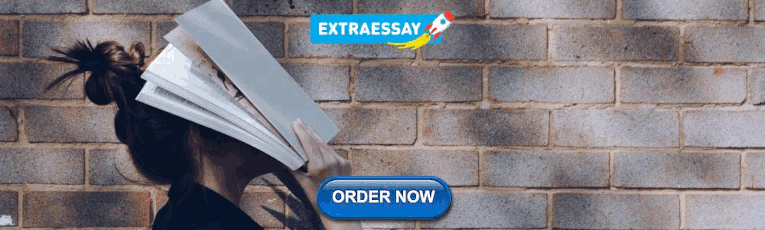
Destructuring
TypeScript supports the following forms of Destructuring (literally named after de-structuring i.e. breaking up the structure):
Object Destructuring
Array destructuring.
It is easy to think of destructuring as an inverse of structuring . The method of structuring in JavaScript is the object literal:
Without the awesome structuring support built into JavaScript, creating new objects on the fly would indeed be very cumbersome. Destructuring brings the same level of convenience to getting data out of a structure.
Destructuring is useful because it allows you to do in a single line, what would otherwise require multiple lines. Consider the following case:
Here in the absence of destructuring you would have to pick off x,y,width,height one by one from rect .
To assign an extracted variable to a new variable name you can do the following:
Additionally you can get deep data out of a structure using destructuring. This is shown in the following example:
Object Destructuring with rest
You can pick up any number of elements from an object and get an object of the remaining elements using object destructuring with rest.
A common use case is also to ignore certain properties. For example:
A common programming question: "How to swap two variables without using a third one?". The TypeScript solution:
Note that array destructuring is effectively the compiler doing the [0], [1], ... and so on for you. There is no guarantee that these values will exist.
Array Destructuring with rest
You can pick up any number of elements from an array and get an array of the remaining elements using array destructuring with rest.
Array Destructuring with ignores
You can ignore any index by simply leaving its location empty i.e. , , in the left hand side of the assignment. For example:
JS Generation
The JavaScript generation for non ES6 targets simply involves creating temporary variables, just like you would have to do yourself without native language support for destructuring e.g.
Destructuring can make your code more readable and maintainable by reducing the line count and making the intent clear. Array destructuring can allow you to use arrays as though they were tuples.
Last updated 4 years ago
Popular Articles
- Typescript In Data Science: Career Prospects (Jun 24, 2024)
- Typescript In Iot Applications: Job Roles (Jun 24, 2024)
- Typescript In Cloud Computing: Career Growth (Jun 24, 2024)
- Typescript In Game Development: Job Opportunities (Jun 24, 2024)
- Typescript In Mobile App Development: Career Prospects (Jun 24, 2024)
TypeScript Destructuring
Switch to English
Table of Contents
Introduction
Destructuring arrays, destructuring objects, advanced destructuring, tips and tricks.
- Basic Array Destructuring
- Ignoring Values
- Basic Object Destructuring
- Renaming Variables
- Default Values
- Nested Destructuring
- Error-Prone Cases
- Using Destructuring in Function Parameters
Object destructuring with types in TypeScript
Object destructuring is a powerful feature of JavaScript and TypeScript that can help you write cleaner and more expressive code. This syntax allows you to extract properties from an object and assign them to variables or parameters.
You can use object destructuring to create variables, assign default values, rename properties, or omit some properties.
In TypeScript, you can also specify the type of the object or the properties that you are destructuring. There are two ways to do this:
- You can use a type annotation after the destructuring pattern, like this:
This tells TypeScript that the object you are destructuring has two properties, name and age, and they are both of type string and number respectively.
- You can use an interface or a type alias to define the shape of the object, and then use it as a type annotation, like this:
This tells TypeScript that the object you are destructuring conforms to the Person interface, which has two properties, name and age, of type string and number respectively.
Using an interface or a type alias can be more convenient and reusable than writing the type annotation inline, especially if you have complex or nested objects.
You can also use object destructuring in function parameters, which can make your code more concise and readable. For example, instead of writing a function like this:
You can write it like this:
This way, you don’t have to repeat the person parameter inside the function body, and you can directly access the name and age properties.
You might also like
Destructuring Object parameters in TypeScript functions
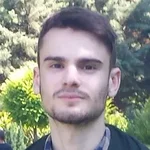
Last updated: Feb 27, 2024 Reading time · 3 min
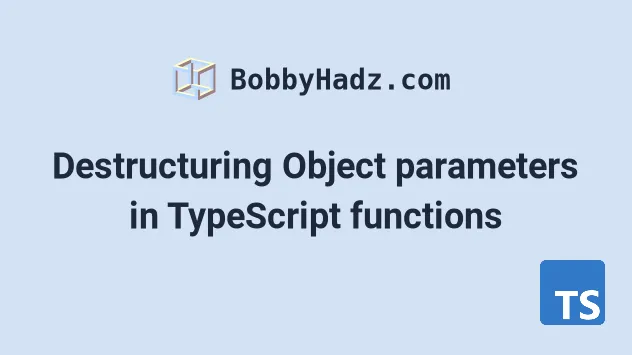
# Destructuring Object parameters in TypeScript functions
When destructuring object parameters in a function, separate the destructured parameters and the type for the specific properties with a colon
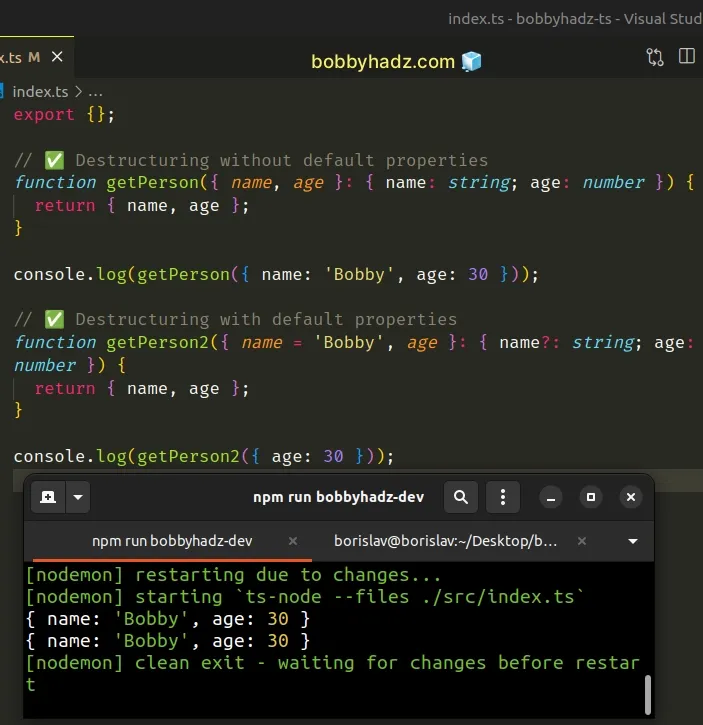
Unfortunately, when destructuring parameters in TypeScript functions, we have to repeat the properties when typing them.
The first example shows how to destructure the name and age properties of an object parameter.
We didn't provide default values for the properties in the first example and both of the properties are required because they weren't marked as optional using a question mark.
# Destructuring Object parameters with default values
The second example shows how to destructure two properties from an object and set a default value for one of the properties.
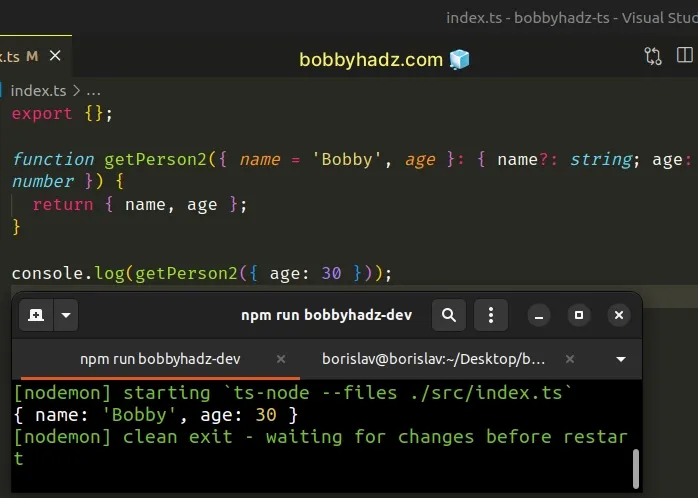
We set a default value for the name property when destructuring it. Note that we used a question mark to mark the property as optional.
If you have default values for all of the object's properties, set a default value for the entire object instead.
This is better than setting a default value for each property when destructuring because you'd still have to pass an empty object to the function.
Even though we provided default values for all of the object's properties when destructuring, we are still required to pass an empty object because the object itself is required.
# Using a Type Alias or an Interface
If your function declaration gets too busy, use a type alias .
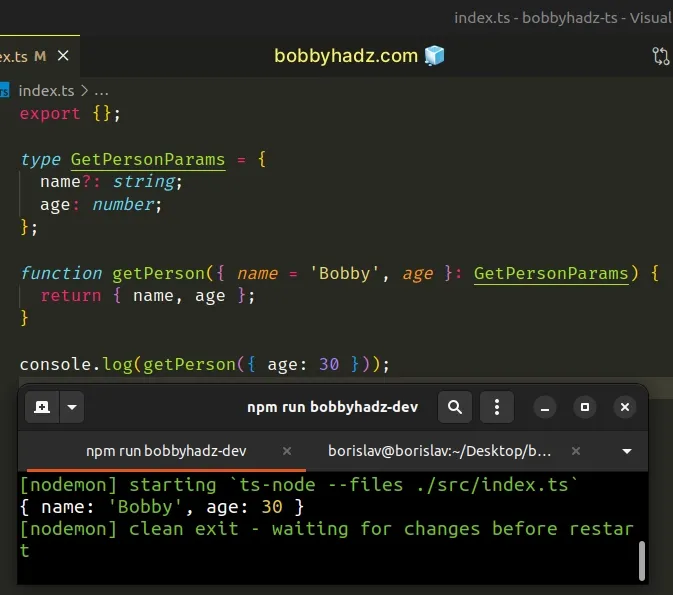
This is a bit easier to read, as we can clearly see that the name property has a default value and the function expects an object of type GetPersonParams .
# Using destructuring inside of a function's body
Alternatively, you can use destructuring inside of the function's body.
Which approach you pick is a matter of personal preference. You can set default values when destructuring inside of the function's body as well.
If you need to pass a function as a parameter, check out the following article .
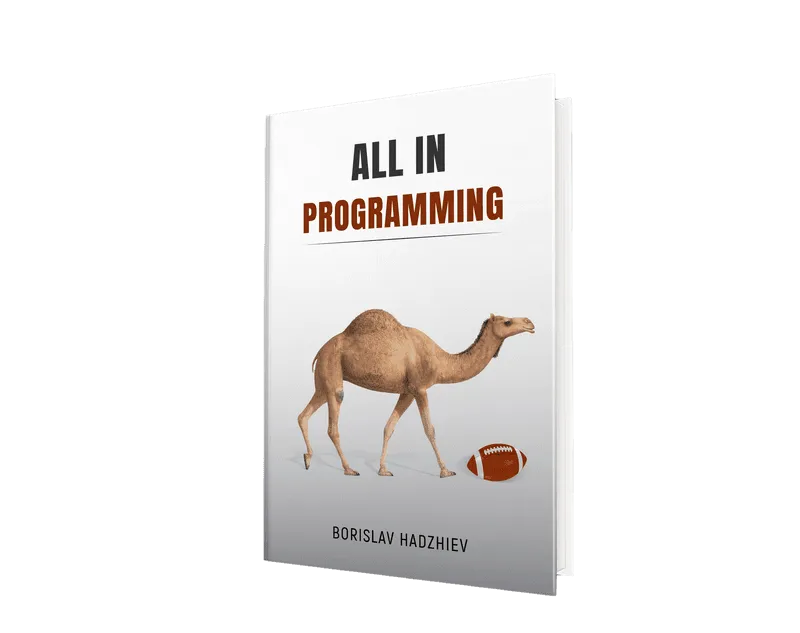
Borislav Hadzhiev
Web Developer
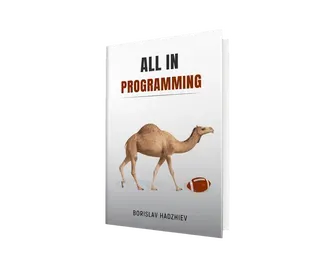
Copyright © 2024 Borislav Hadzhiev

DEV Community

Posted on Apr 16, 2023
Finding the Balance: When to Use Object Destructuring and When to Avoid It
One of the powerful features of both JavaScript and TypeScript is object destructuring, which allows developers to extract properties from objects and assign them to variables in a concise and readable manner. This feature has proven particularly useful for handling function parameters in TypeScript, as it works seamlessly with the language's typing system. In this blog post, we will explore the benefits of using object destructuring for function parameters in TypeScript, and discuss situations where it might not be the best choice.
Let's go through the benefits of object destructuring for Function Parameters in TypeScript
Improved readability: Object destructuring can make your TypeScript code more readable, especially when dealing with functions that have multiple parameters. Enclosing parameters within an object can make it easier for others to understand their purpose and values.
Named parameters: Unlike Python and Swift Javascript has no built-in support for named parameters. Destructuring provides a way to use it though, reducing confusion when dealing with multiple parameters. This makes the code self-documenting, and it also prevents the risk of passing arguments in the wrong order.
Optional parameters and default values: With destructuring, you can easily provide optional parameters and set default values. You can simply omit properties in the object that you don't want to pass, and the function can set default values for those properties. This is more flexible than having to provide undefined or some other placeholder value for unused parameters. TypeScript's typing system further enhances this capability, allowing you to define optional properties with the ? notation.
Extensibility: Using object destructuring can make your functions more extensible. You can add new properties to the object without changing the function signature, and existing calls to the function will still work. TypeScript's typing system ensures that new properties are correctly typed and adhered to.
Strong typing and IntelliSense: TypeScript's type-checking capabilities, combined with object destructuring, provide strong typing for function parameters. This helps you catch potential type-related issues early in the development process, and it also enables better autocompletion and IntelliSense in code editors, making it easier to work with function parameters.
When to Use Multiple Parameters Instead of Object Destructuring
Despite the benefits of object destructuring, there are cases where using multiple parameters is more appropriate:
Functions with only a few mandatory parameters: If a function has just one or two mandatory parameters, and the order of parameters is easy to understand, using multiple parameters can be more straightforward and less verbose.
Simple callback functions: When using simple callback functions, like array iterators or event listeners, where the signature is well-defined and there are only a few parameters, using multiple parameters is usually more appropriate.
Mathematical or utility functions: In some cases, like mathematical functions or utility functions, the parameters are well-understood and have a specific order. In these cases, using multiple parameters can be more appropriate.
Let's talk about performance overhead
While the benefits of object destructuring are plentiful, it is important to consider the potential performance implications. Object destructuring can introduce a slight performance overhead as it involves creating a new object and accessing its properties. In most scenarios, this overhead is negligible and should not be a concern. However, in high-traffic backends or performance-critical applications, such as real-time data processing systems or high-frequency trading platforms, the cumulative impact of this overhead might become significant. In such cases, it is crucial to thoroughly evaluate the trade-offs between the benefits of object destructuring and the potential performance impact before making a decision.
Object destructuring is a powerful feature in TypeScript that can make your code more readable, flexible, and extensible, particularly when it comes to handling function parameters. TypeScript's strong typing capabilities, combined with object destructuring, provide robust support for optional parameters and improved code quality. However, it is important to recognize situations where using multiple parameters may be more appropriate, such as simple functions, callback functions, or mathematical/utility functions. Ultimately, the choice depends on your
Top comments (0)
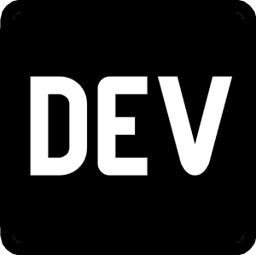
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
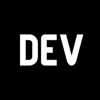
Congrats to the Frontend Challenge: Recreation Edition Winners!
dev.to staff - Aug 6

The Perils of Callback Hell: Navigating the Pyramid of Doom in JavaScript
Eze Onyekachukwu - Jul 9

Build a Grammarly Alternative Using ToolJet and OpenAI in 10 Minutes📝
Karan Rathod - Aug 7

How to Handle RTK-Query Request Cancellations in React
Rahul Kumar - Aug 1
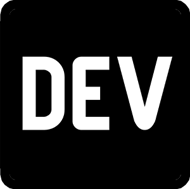
We're a place where coders share, stay up-to-date and grow their careers.

Typing Destructured Object Parameters in TypeScript
In TypeScript, you can add a type annotation to each formal parameter of a function using a colon and the desired type, like this:
That way, your code doesn't compile when you attempt to call the function with an argument of an incompatible type, such as number or boolean . Easy enough.
Let's now look at a function declaration that makes use of destructuring assignment with an object parameter, a feature that was introduced as part of ECMAScript 2015. The toJSON function accepts a value of any type that should be stringified as JSON. It additionally accepts a settings parameter that allows the caller to provide configuration options via properties:
The type of the value parameter is explicitly given as any , but what type does the pretty property have? We haven't explicitly specified a type, so it's implicitly typed as any . Of course, we want it to be a boolean, so let's add a type annotation:
However, that doesn't work. The TypeScript compiler complains that it can't find the name pretty that is used within the function body. This is because boolean is not a type annotation in this case, but the name of the local variable that the value of the pretty property gets assigned to. Again, this is part of the specification of how object destructuring works.
Because TypeScript is a superset of JavaScript, every valid JavaScript file is a valid TypeScript file (set aside type errors, that is). Therefore, TypeScript can't simply change the meaning of the destructuring expression { pretty: boolean } . It looks like a type annotation, but it's not.
# Typing Immediately Destructured Parameters
Of course, TypeScript offers a way to provide an explicit type annotation. It's a little verbose, yet (if you think about it) consistent:
You're not directly typing the pretty property, but the settings object it belongs to, which is the actual parameter passed to the toJSON function. If you now try to compile the above TypeScript code, the compiler doesn't complain anymore and emits the following JavaScript function:
# Providing Default Values
To call the above toJSON function, both the value and the settings parameter have to be passed. However, it might be reasonable to use default settings if they aren't explicitly specified. Assuming that pretty should be true by default, we'd like to be able to call the function in the following various ways:
The function call #1 already works because all parameters are specified. In order to enable function call #2, we have to mark the pretty property as optional by appending a question mark to the property name within the type annotation. Additionally, the pretty property gets a default value of true if it's not specified by the caller:
Finally, function call #3 is made possible by providing a default value of {} for the destructuring pattern of the settings object. If no settings object is passed at all, the empty object literal {} is being destructured. Because it doesn't specify a value for the pretty property, its fallback value true is returned:
Here's what the TypeScript compiler emits when targeting "ES5" :
When targeting "ES6" , only the type information is removed:
# Extracting a Type for the Settings Parameter
With multiple properties, the inline type annotation gets unwieldy quickly, which is why it might a good idea to create an interface for the configuration object:
You can now type the settings parameter using the new interface type:
TypeScript: Destructuring Objects with Types
Learn the simple yet effective way to destructure objects with type annotations in typescript. this post breaks down the common pitfalls and provides a clear guide to using types with object destructuring, making your typescript code cleaner and more reliable..
Published Jan 31, 2024
Recently, I ran into a little issue while using TypeScript in a project. I was trying to pull out some values from an object, something I’ve done a bunch of times. But this time, I wanted to make sure those values were the right type.
Here’s what I started with:
I thought I could just slap the types right in there like this:
Turns out, that wasn’t right. TypeScript thought I was trying to rename title to string and pages to number , which wasn’t what I wanted.
The right way to do it is to put the types after the whole thing:
But then I found an even cooler way. If I make a type or interface first, like this:
I can keep it super simple when I pull out the values:
This little adventure showed me that even when you think you know something, there’s always a twist or a new trick to learn, especially with TypeScript.
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy

Home » Introduction and Basics » Typescript object destructuring results in property assignment expected
Typescript object destructuring results in property assignment expected
Introduction.
Typescript is a popular programming language that adds static typing to JavaScript. It provides developers with the ability to catch errors during development and improve code quality. However, like any programming language, Typescript has its own set of challenges and issues that developers may encounter. One common issue is the “Typescript object destructuring results in property assignment expected” error. In this article, we will explore this error and provide solutions with examples.
The “Typescript object destructuring results in property assignment expected” error occurs when you try to destructure an object but forget to provide a default value for the property being destructured. Let’s take a look at an example:
In this example, we are trying to destructure the “person” object and assign the value of the “name” property to a variable called “name”. However, since we did not provide a default value for the “name” property, Typescript throws the “Typescript object destructuring results in property assignment expected” error.
To solve this error, we need to provide a default value for the property being destructured. We can do this by using the assignment operator (=) and specifying a default value after the property name. Let’s modify our previous example to include a default value:
In this modified example, we have provided a default value of an empty string (“”) for the “name” property. Now, even if the “name” property is not present in the “person” object, the destructuring assignment will not throw an error.
The “Typescript object destructuring results in property assignment expected” error can be easily solved by providing a default value for the property being destructured. By doing so, we ensure that the destructuring assignment does not throw an error even if the property is not present in the object. This helps in writing more robust and error-free Typescript code.
- No Comments
- assignment , destructuring , expected , object , property , results , typescript
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Not stay with the doubts.

- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
typescript how to do object destructured assignment
I have some typescript code which looks like this:
typescript compiler is giving me error:
Now I am fairly new to typescript but it seems to me like the compiler is telling me: "you can't assign 'payload' which is an object type to the destructured variables which are of type number and array."
If that is true then how am I supposed to do a destructured assignment in typescript?

- 1 Try ={...payload} on the right side of your assignment statement. – julianobrasil Commented Apr 30, 2019 at 2:39
- What exactly are you trying to achieve? Are you trying to extract the properties count and malfunctions ? – wentjun Commented Apr 30, 2019 at 2:41
- @jpavel - that doesn't work - typescript doesn't seem to allow object rest params – HelloWorld Commented Apr 30, 2019 at 3:17
I figured it out - need to perform the destructure in the parameter:
- You don't have to "perform the destructure in the parameter", you just have to have the type for the param. The problem was object can't be destructured, because it's a vague type. So transform( payload: { count: number, malfunctions: Array<object> } ) with your original code would work. – Aaron Beall Commented Apr 30, 2019 at 3:22
- well yes I could do that too but then I'd still have to destructure the object later or access the properties on the object via dot notation right? – HelloWorld Commented Apr 30, 2019 at 3:42
- You would destructure like const { count = 0, malfunctions = [] } = payload -- again the issue was the use of object – Aaron Beall Commented Apr 30, 2019 at 3:59
- when you say 'const { count = 0, malfunctions = [] } = payload' - thats what i mean by ' I'd still have to destructure the object later' – HelloWorld Commented Apr 30, 2019 at 4:17
- Yep, you can destructure in the param assignment or later... but in any case the original error had nothing to do with that. – Aaron Beall Commented Apr 30, 2019 at 6:38
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged angular typescript or ask your own question .
- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- Did the Space Shuttle weigh itself before deorbit?
- How to Handle a Discovery after a Masters Completes
- What was Bertrand Russell's take on the resolution of Russell's paradox?
- I submitted a paper and later realised one reference was missing, although I had written the authors in the body text. What could happen?
- What is the airspeed record for a helicopter flying backwards?
- How do *Trinitarians* explain why Jesus didn't mention the Holy Spirit in Matthew 24:36 regarding his return?
- Is it illegal to allow (verbally) someone to do action that may kill them?
- Why HIMEM was implemented as a DOS driver and not a TSR
- Does Gimp do batch processing?
- A study on the speed of gravity
- Should I pay off my mortgage if the cash is available?
- Can two different points can be connected by multiple adiabatic curves?
- Get the first character of a string or macro
- Why are extremally disconnected spaces so hard to give examples of?
- Rock paper scissor game in Javascript
- Has the application of a law ever being appealed anywhere due to the lawmakers not knowing what they were voting/ruling?
- In "Take [action]. When you do, [effect]", is the action a cost or an instruction?
- Is an invalid date considered the same as a NULL value?
- What role does the lower bound play in the statement of Savitch's Theorem?
- Do space stations have anything that big spacecraft (such as the Space Shuttle and SpaceX Starship) don't have?
- Do "Whenever X becomes the target of a spell" abilities get triggered by counterspell?
- How to use the `=short-text` method in conjunction with a key-value list
- Inaccurate group pace
- How can I cross an overpass in "Street View" without being dropped to the roadway below?
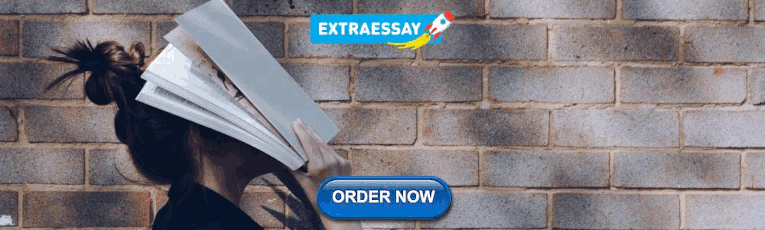
IMAGES
VIDEO
COMMENTS
Destructuring. Another ECMAScript 2015 feature that TypeScript has is destructuring. For a complete reference, see the article on the Mozilla Developer Network. In this section, we'll give a short overview. Array destructuring. The simplest form of destructuring is array destructuring assignment:
Destructuring with more elements than the source. In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N, only the first N variables are assigned values. The values of the remaining variables will be ...
The method of structuring in JavaScript is the object literal: var foo = { bar: { bas: 123 } }; Without the awesome structuring support built into JavaScript, creating new objects on the fly would indeed be very cumbersome. Destructuring brings the same level of convenience to getting data out of a structure.
In this article, we will be exploring the concept of Destructuring in TypeScript. Destructuring is a feature that allows you to extract multiple items from an array or object and assign them to their own variables. This feature is incredibly useful in simplifying your code and increasing readability. By the end of this article, you will have a ...
This is especially true with deeply nested destructuring, which gets really hard to understand even without piling on renaming, default values, and type annotations. Try to keep destructuring expressions small and simple. You can always write the assignments that destructuring would generate yourself. Destructuring function parameters
TypeScript is then able to understand that the function takes an object argument that is of the shape of the Person interface and destructuring occurs as you would expect it to in ES6. This ...
To work around this, we have the destructuring assignment introduced in ES6: The same code using destructuring. That's a lot more concise. And notice how we're setting the value for id . When ...
Object destructuring with types in TypeScript. Object destructuring is a powerful feature of JavaScript and TypeScript that can help you write cleaner and more expressive code. This syntax allows you to extract properties from an object and assign them to variables or parameters. You can use object destructuring to create variables, assign ...
The code for this article is available on GitHub. Unfortunately, when destructuring parameters in TypeScript functions, we have to repeat the properties when typing them. The first example shows how to destructure the name and age properties of an object parameter. index.ts. function getPerson({ name, age }: { name: string; age: number ...
As TypeScript developers, we occasionally face perplexing issues with object destructuring assignments. Here, we dissect these problems and offer practical solutions. A common scenario involves…
The cool part about TypeScript is that we can define types for certain variables. However, there is a scenario that might prove a bit difficult. And this is destructuring an object. Let's take the following example: const user = { firstname: 'Chris', lastname: 'Bongers', age: 32}; const { firstname, age} = user;
Object destructuring is a powerful feature in TypeScript that can make your code more readable, flexible, and extensible, particularly when it comes to handling function parameters. TypeScript's strong typing capabilities, combined with object destructuring, provide robust support for optional parameters and improved code quality.
Therefore, TypeScript can't simply change the meaning of the destructuring expression { pretty: boolean }. It looks like a type annotation, but it's not. #Typing Immediately Destructured Parameters. Of course, TypeScript offers a way to provide an explicit type annotation. It's a little verbose, yet (if you think about it) consistent:
Learn the simple yet effective way to destructure objects with type annotations in TypeScript. This post breaks down the common pitfalls and provides a clear guide to using types with object destructuring, making your TypeScript code cleaner and more reliable. Recently, I ran into a little issue while using TypeScript in a project.
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
Introduction Typescript is a popular programming language that adds static typing to JavaScript. It provides developers with the ability to catch errors during development and improve code quality. However, like any programming language, Typescript has its own set of challenges and issues that developers may encounter. One common issue is the "Typescript object destructuring results […]
return Object.getOwnPropertyNames(this).map(key => ({[key]: this[key]})); You can use Object.assign (es5+), as suggested by Frank, or Object.defineProperty to initialize the member fields in the constructor. The latter gives the option to make the properties writable or assign getters and/or setters.
Destructuring assignment in Typescript for global variables. 9. Using Object Destructuring to Assign Class Members. 20. Destructuring assignment in Typescript. 5. How to safely destructure a JavaScript object in TypeScript. 6. JavaScript Destructure and assign to new object. 0.