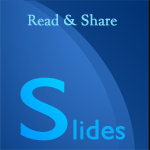
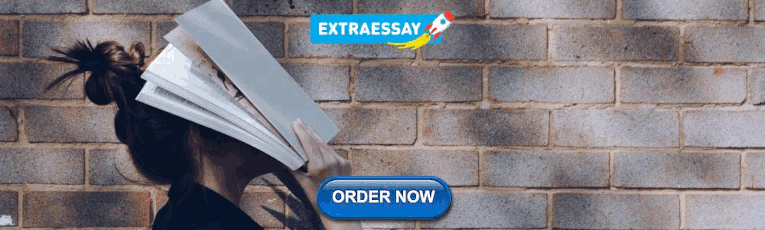
HTML BASICS Slides Presentation
Click to access all Slides..
This slide presentation shows basics of HTML.
HTML and XHTML are the foundation of all web development. HTML is used as the graphical user interface in client-side programs written in JavaScript. Server-side languages like PHP and Java also receive data from web pages and use HTML as the output mechanism. The emerging Ajax technologies likewise use HTML and XHTML as their visual engine. HTML was once a very loosely-defined language with very little standardization, but as it has become more important, the need for standards has become more apparent. Regardless of whether you choose to write HTML or XHTML, understanding the current standards will help you provide a solid foundation that will simplify all your other web coding. Fortunately HTML and XHTML are actually simpler than they used to be, because much of the functionality has moved to CSS.
Common Elements
Every page (HTML or XHTML shares certain elements in common.) All are essentially plain text files, with the .html extension. HTML files should not be created with a word processor, but in some type of editor that creates plain text. Every page has a large container (HTML or XHTML) and two major subcontainers, the head and the body. The head area contains information useful behind the scenes, such as CSS formatting instructions and JavaScript code. The body contains the part of the page that is visible to the user.
Tags and Attributes
An HTML document is based on the notion of tags. A tag is a piece of text inside angle brackets (<>). Tags typically have a beginning and an end, and usually contain some sort of text inside them. For example, a paragraph is normally denoted like this:
The <p> indicates the beginning of a paragraph. Text is then placed inside the tag, and the end of the paragraph is denoted by an end tag, which is similar to the start tag but with a slash (</p>.) It is common to indent content in a multi-line tag, but it is also legal to place tags on the same line:
Tags are sometimes enhanced by attributes, which are name value pairs that modify the tag. For example, the tag (used to embed an image into a page) usually includes the following attributes:
The src attribute describes where the image file can be found, and the alt attribute describes alternate text that is displayed if the image is unavailable.
Nested tags
Tags can be (and frequently are) nested inside each other. Tags cannot overlap, so <a><b></a></b> is not legal, but <a><b></b></a> is fine.
HTML VS XHTML
HTML has been around for some time. While it has done its job admirably, that job has expanded far more than anybody expected. Early HTML had very limited layout support. Browser manufacturers added many competing standards and web developers came up with clever workarounds, but the result is a lack of standards and frustration for web developers. The latest web standards (XHTML and the emerging HTML 5.0 standard) go back to the original purpose of HTML: to describe the structure of the data only, and leave all formatting to CSS (Please see the DZone CSS Refcard Series). XHTML is nothing more than HTML code conforming to the stricter standards of XML. The same style guidelines are appropriate whether you write in HTML or XHTML (but they tend to be enforced in XHTML):
Most of the requirements of XHTML turn out to be good practice whether you write HTML or XHTML. I recommend using XHTML strict so you can validate your code and know it follows the strictest standards.
XHTML has a number of flavors. The strict type is recommended, as it is the most up-to-date standard which will produce the most predictable results. You can also use a transitional type (which allows deprecated HTML tags) and a frameset type, which allows you to add frames. For most applications, the strict type is preferred.
HTML Template
The following code can be copied and pasted to form the foundation of a basic web page:
The structure of your web pages is critical to the success of programs based on those pages, so use a validating tool to ensure you haven't missed anything
Validating Tool | Description |
WC3 | The most commonly used validator is online at http://validator.w3.org this free tool checks your page against the doctype you specify and ensures you are following the standards. This acts as a 'spell-checker' for your code and warns you if you made an error like forgetting to close a tag. |
HTML Tidy | There's an outstanding free tool called HTML tidy which not only checks your pages for validity, but also fixes most errors automatically. Download this tool at http://tidy.sourceforge.net/ or (better) use the HTML validator extension to build tidy into your browser. |
HTML Validator extension | The extension mechanism of Firefox makes it a critical tool for web developers. The HTML Validator extension is an invaluable tool. It automatically checks any page you view in your browser against both the w3 validation engine and tidy. It can instantly find errors, and repair them on the spot with tidy. With this free extension available at http://users.skynet. be/mgueury/mozilla/ , there's no good reason not to validate your code. |
USEFUL OPEN SOURCE TOOLS
Some of the best tools for web development are available through the open source community at no cost at all. Consider these application as part of your HTML toolkit:
Open Source Tool | Description |
Aptana | http://www.aptana.com/ This free programmer's editor (based on Eclipse) is a full-blown IDE customized for HTML / XHTML, CSS, JavaScript, and Ajax. It offers code completion, syntax highlighting, and FTP support within the editor. |
Web Developer Toolbar | https://www.addons.mozilla.org/en-US/firefox/addon/60 This Firefox extension adds numerous debugging and web development tools to your browser. |
Firebug | https://addons.mozilla.org/en-US/firefox/addon/1843 is an add-on that adds full debugging capabilities to the browser. The firebug lite version even works with IE. |
PAGE STRUCTURE ELEMENTS
The following elements are part of every web page.
Element | Description |
<html></html> | Surrounds the entire page |
<head></head> | Contains header information (metadata, CSS styles, JavaScript code) |
<title></title> | Holds the page title normally displayed in the title bar and used in search results |
<body></body> | Contains the main body text. All parts of the page normally visible are in the body |
KEY STRUCTURAL ELEMENTS
Most pages contain the following key structural elements:
Element | Name | Description |
<h1> </h1> | Heading 1 | Reserved fo strongest emphasis |
<h2> </h2> | Heading 2 | Secondary level heading. Headings go down to level 6, but <h1> through <h3> are most common |
<p> </p> | Paragraph | Most of the body of a page should be enclosed in paragraphs |
<div> </div> | Division | Similar to a paragraph, but normally marks a section of a page. Divs usually contain paragraphs |
LISTS AND DATA
Web pages frequently incorporate structured data so HTML includes several useful list and table tag
Element | Name | Description |
<ul></ul> | Unordered list | Normally these lists feature bullets (but that can be changed with CSS) |
<ol></ol> | Ordered list | These usually are numbered, but this can be changed with CSS |
<li></li> | List item | Used to describe a list item in an unordered list or an ordered list |
<dl></dl> | Definition list | Used for lists with name-value pairs |
<dt></dt> | Definition term | The name in a name-value pair. Used in definition lists |
<dd></dd> | Definition description | The value (or definition) of a name, value pair |
<table></table> | Table | Defines beginning and end of a table |
<tr></tr> | Table row | Defines a table row. A table normally consists of several <tr> pairs (one per row) |
<td></td> | Table data | Indicates data in a table cell. <td> tags occur within <tr> (which occur within <table>) |
<th></th> | Table heading | Indicates a table cell to be treated as a heading with special formatting |
Standard List Types
HTML supports three primary list types. Ordered lists and unordered lists are the primary list types. By default, ordered lists use numeric identifiers, and unordered lists use bullets.
However, you can use the list-style-type CSS attribute to change the list marker to one of several types.
Lists can be nested inside each other
Definition lists
The special definition list is used for name / value pairs. The definition term (dt) is a word or phrase that is used as the list marker, and the definition data is normally a paragraph:
Use of tables
Tables were used in the past to overcome the page-layout shortcomings of HTML. That use is now deprecated in favor of CSS-based layout. Use tables only as they were intended, to display tabular data.
A table mainly consists of a series of table rows (tr.) Each table row consists of a number of table data (td) elements. The table heading (th) element can be used to indicate a table cell should be marked as a heading.
The rowspan and colspan attributes can be used to make a cell span more than one row or column.
Each row of a table should have the same number of columns, and each column should have the same number of rows. Use of the span attribute may require adjustment to other rows or columns.
LINKS AND IMAGES
Links and images are both used to incorporate external resources into a page. Both are reliant on URIs (Universal Resource Indicators), commonly referred to as URLs or addresses.
<a> (anchor) The anchor tag is used to provide the basic web link:
In this example, http://www.example.com is the site to be visited. The text "link to example.com" will be highlighted as a link.
absolute and relative references
<link>
The link tag is used primarily to pull in external CSS files:
<img>
The img tag is used in to attach an image. Valid formats are .jpg, .png, and .gif. An image should always be accompanied by an alt attribute describing the contents of the image.
Image formatting attributes (height, width, and align) are deprecated in favour of CSS.
SPECIALTY MARKUP
HTML / XHTML includes several specialty tags. These are used to describe special purpose text. They have default styling, but of course the styles can be modified with CSS.
<quote>
The quote tag is intended to display a single line quote:
Quote is an inline tag. If you need a block level quote, use <blockquote>.
<pre>
The <pre> tag is used for pre-formatted text. It is sometimes used for code listings or ASCII art because it preserves carriage returns. Pre-formatted text is usually displayed in a fixed-width font.
<code>
The code format is used to manage pre-formatted text, especially code listings. It is very similar to pre.
<blockquote>
This tag is used to mark multi-line quotes. Frequently it is set off with special fonts and indentation through CSS. It is a block-level tag.
<span>
The span tag is a vanilla inline tag. It has no particular formatting of its own. It is intended to be used with a class or ID when you want to apply style to an inline chunk of code.
The em tag is used for standard emphasis. By default, <em> italicizes text, but you can use CSS to make any other type of emphasis you wish.
<strong>
This tag represents strong emphasis. By default, it is bold, but you can modify the formatting with CSS.
Forms are the standard user input mechanism in HTML / XHTML. You will need another language like JavaScript or PHP to read the contents of the form elements and act upon them.
Form Structure
A number of tags are used to describe the structure of the form. Begin by looking over a basic form:
The <form></form> pair describes the form. In XHTML strict, you must indicate the form's action property. This is typically the server-side program that will read the form. If there is no such program, you can set the action to null ("") The method attribute is used to determine whether the data is sent through the get or post mechanism.
Most form elements are inline tags, and must be encased in a block element. The fieldset is designed exactly for this purpose. Its default appearance draws a box around the form. You can have multiple fieldsets inside a single form.
You can add a legend inside a fieldset. This describes the purpose of the fieldset.
A label is a special inline element that describes a particular field. A label can be paired with an input element by putting that element's ID in the label's for attribute.
The input element is a general purpose inline element. It is meant to be used inside a form, and it is the basis for several types of more specific input. The subtype is indicated by the type attribute. Input elements usually include an id attribute (used for CSS and JavaScript identification) and / or a name attribute (used in server-side programming.) The same element can have both a name and an id.
This element allows a single line of text input:
Passwords display just like textboxes, except rather than showing the text as it is typed, an asterisk appears for each letter. Note that the data is not encoded in any meaningful way. Typing text into a password field is still entirely unsecure.
Radio Button
Radio buttons are used in a group. Only one element of a radio group can be selected at a time. Give all members of a radio group the same name value to indicate they are part of a group.
Attaching a label to a radio button means the user can activate the button by clicking on the corresponding label. For best results, use the selected attribute to force one radio button to be the default.
Checkboxes are much like radio buttons, but they are independent. Like radio buttons, they can be associated with a label.
Hidden fields hold data that is not visible to the user (although it is still visible in the code) It is primarily used to preserve state in server-side programs.
Note that the data is still not protected in any meaningful way.
Buttons are used to signal user input. Buttons can be created through the input tag:
This will create a button with the caption "launch the missiles." When the button is clicked, the page will attempt to run a JavaScript function called "launchMissiles()" Standard buttons are usually used with JavaScript code on the client. The same button can also be created with this alternate format:
This second form is preferred because buttons often require different CSS styles than other input elements. This second form also allows an <img> tag to be placed inside the button, making the image act as the button.
The reset button automatically resets all elements in its form to their default values. It doesn't require any other attributes.
Select / option
Drop-down lists can be created through the select / option mechanism. The select tag creates the overall structure, which is populated by option elements.
The select has an id (for client-side code) or name (for serverside code) identifier. It contains a number of options. Each option has a value which will be returned to the program. The text between <option> and </option> is the value displayed to the user. In some cases (as in this example) the value displayed to the user is not the same as the value used by programs.
Multiple Selections
You can also create a multi-line selection with the select and option tags:
DEPRECATED FORMATTING TAGS
Certain tags common in older forms of HTML are no longer recommended as CSS provides much better alternatives.
The font tag was used to set font color, family (typeface) and size. Numerous CSS attributes replace this capability with much more flexible alternatives. See the CSS refcard for details.
I (italics)
HTML code should indicate the level of emphasis rather than the particular stylistic implications. Italicizing should be done through CSS. The <em> tag represents emphasized text. It produces italic output unless the style is changed to something else. The <i> tag is no longer necessary and is not recommended. Add font-style: italic to the style of any element that should be italicized.
Like italics, boldfacing is considered a style consideration. Use the <strong> tag to denote any text that should be strongly emphasized. By default, this will result in boldfacing the enclosed text. You can add bold emphasis to any style with the font-weight: bold attribute in CSS.
DEPRECATED TECHNIQUES
In addition to the deprecated tags, there are also techniques which were once common in HTML that are no longer recommended.
Frames have been used as a layout mechanism and as a technique for keeping one part of the page static while dynamically loading other parts of the page in separate frames. Use of frames has proven to cause major usability problems. Layout is better handled through CSS techniques, and dynamic page generation is frequently performed through server-side manipulation or AJAX.
Table-based design
Before CSS became widespread, HTML did not have adequate page formatting support. Clever designers used tables to provide an adequate form of page layout. CSS provides a much more flexible and powerful form of layout than tables, and keeps the HTML code largely separated from the styling markup.
HTML ENTITIES
Sometimes you need to display a special character in a web page. HTML has a set of special characters for exactly this purpose. Each of these entities begins with the ampersand(&) followed by a code and a semicolon.
Character | Name | Code | Note |
---|---|---|---|
Non-breaking space | Adds white space | ||
< | Used to display HTML code or mathematics | ||
> | Greater than | > | Used to display HTML code or mathematics |
& | Ampersand | & | If you're not displaying an entity but really want the & symbol |
© | Copyright | © | Copyright symbol |
® | Registered trademark | ® | Registered trademark |
HTML 5 / CSS3 PREVIEW
New technologies are on the horizon. Firefox 3.5 now has support for significant new HTML 5 features, and CSS 3 is not far behind. While the following should still be considered experimental, they are likely to become very important tools in the next few years. Firefox 3.5, Safari 4 (and a few other recent browsers) support the following new features:
Audio and video tags
Finally the browsers have direct support for audio and video without plugin technology. These tags work much like the img tag.
The HTML 5 standard currently supports Ogg Theora video, Ogg Vorbis audio, and wav audio. The Ogg formats are opensource alternatives to proprietary formats, and plenty of free tools convert from more standard video formats to Ogg. The autoplay option causes the element to play automatically. The controls element places controls directly into the page.
The code between the beginning and ending tag will execute if the browser cannot process the audio or video tag. You can place alternate code here for embedding alternate versions (Flash, for example)
The canvas tag offers a region of the page that can be drawn upon (usually with Javascript.) This creates the possibility of real interactive graphics without requiring plugins like Flash.
This is actually a CSS improvement, but it's much needed. It allows you to define a font-face in CSS and include a ttf font file from the server. You can then use this font face in your ordinary CSS and use the downloaded font. If this becomes a standard, we will finally have access to reliable downloadable fonts on the web, which will usher in web typography at long last.
Follow us on Facebook and Twitter for latest update.
https://www.w3resource.com/slides/basics-of-html-5.php
- Weekly Trends and Language Statistics
Unsupported browser
This site was designed for modern browsers and tested with Internet Explorer version 10 and later.
It may not look or work correctly on your browser.
How to Create Presentation Slides With HTML and CSS

As I sifted through the various pieces of software that are designed for creating presentation slides, it occurred to me: why learn yet another program, when I can instead use the tools that I'm already familiar with?
We can easily create beautiful and interactive presentations with HTML, CSS, and JavaScript, the three basic web technologies. In this tutorial, we'll use modern HTML5 markup to structure our slides, we'll use CSS to style the slides and add some effects, and we'll use JavaScript to trigger these effects and reorganize the slides based on click events.
This tutorial is perfect for those of you new to HTML5, CSS, and JavaScript, who are looking to learn something new by building. After this you could even learn to build an HTML5 slide deck or a dynamic HTML with JavaScript PPT . The sky is the limit.
Wondering how to create an HTML slideshow? Here's the final preview of the presentation HTML tutorial slides we're going to build:
Have you checked out HTML tutorial slides? It's a good example of HTML PPT slides for download.
Let's begin.
1. Create the Directory Structure
Before we get started, let's go ahead and create our folder structure; it should be fairly simple. We'll need:
- css/style.css
- js/scripts.js
This is a simple base template. Your files remain blank for the time being. We'll fix that shortly.
2. Create the Starter Markup
Let's begin by creating the base markup for our presentation page. Paste the following snippet into your index.html file.
lang="en"> | |
charset="UTF-8"> | |
name="viewport" content="width=device-width, initial-scale=1.0"> | |
http-equiv="X-UA-Compatible" content="ie=edge"> | |
Document</title> | |
rel="stylesheet" href="css/style.css"> | |
rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css" integrity="sha512-9usAa10IRO0HhonpyAIVpjrylPvoDwiPUiKdWk5t3PyolY1cOd4DSE0Ga+ri4AuTroPR5aQvXU9xC6qOPnzFeg==" crossorigin="anonymous" referrerpolicy="no-referrer" /> | |
class="container" | |
div id="presentation-area"> | |
src="js/index.js" type="text/javascript"></script> | |
From the base markup, you can tell that we are importing Font Awesome Icons, our stylesheet ( style.css ), and our JavaScript ( index.js ).
Now we'll add the HTML markup for the actual slides inside the <div> wrapper:
class="presentation"> | |
class="slide show"> | |
class="heading"> | |
class="content grid center"> | |
class="title"> | |
/> All You Need To Know | |
class="slide"> | |
class="heading"> | |
class="content grid center"> | |
class="title"> | |
class="sub-title"> | |
Lecture No. 1</p> | |
My Email Address</p> | |
href="">[email protected]</a></p> | |
We have seven slides in total, and each slide is composed of the heading section and the content section.
Only one slide will be shown at a time. This functionality is handled by the .show class, which will be implemented later on in our stylesheet. Using JavaScript, later on, we'll dynamically add the .show class to the active slide on the page.
Below the slides, we'll add the markup for our slide's counter and tracker:
id="presentation-area"> | |
class="counter"> | |
Later on, we'll use JavaScript to update the text content as the user navigates through the slides.
Finally, we'll add the slide navigator just below the counter:
id="presentation-area"> | |
class="navigation"> | |
id="full-screen" class="btn-screen show"> | |
class="fas fa-expand"></i> | |
id="small-screen" class="btn-screen"> | |
class="fas fa-compress"></i> | |
id="left-btn" class="btn"> | |
class="fas fa-solid fa-caret-left"></i> | |
id="right-btn" class="btn"> | |
class="fa-solid fa-caret-right"></i> | |
This section consists of four buttons responsible for navigating left and right and switching between full-screen mode and small-screen mode. Again, we'll use the class .show to regulate which button appears at a time.
That'll be all for the HTML part. Now, let's move over to styling.
3. Make It Pretty
Our next step takes place within our stylesheet. We'll be focusing on both aesthetics and functionality here. To make each slide translate from left to right, we'll need to target the class .show with a stylesheet to show the element.
Here's the complete stylesheet for our project:
{ | |
: 0; | |
: 0; | |
: border-box; | |
: sans-serif; | |
: all 0.5s ease; | |
{ | |
: 100vw; | |
: 100vh; | |
: flex; | |
: center; | |
: center; | |
{ | |
: 2rem; | |
li, | |
{ | |
: 1.2em; | |
{ | |
: #212121; | |
: 100%; | |
: 100%; | |
: relative; | |
: flex; | |
: center; | |
: center; | |
{ | |
: 1000px; | |
: 500px; | |
: relative; | |
: purple; | |
.presentation { | |
: 100%; | |
: 100%; | |
: hidden; | |
: #ffffff; | |
: relative; | |
.counter { | |
: absolute; | |
: -30px; | |
: 0; | |
: #b6b6b6; | |
.navigation { | |
: absolute; | |
: -45px; | |
: 0; | |
.full-screen { | |
: 100%; | |
: 100%; | |
: hidden; | |
.full-screen .counter { | |
: 15px; | |
: 15px; | |
.full-screen .navigation { | |
: 15px; | |
: 15px; | |
.full-screen .navigation .btn:hover { | |
: #201e1e; | |
: #ffffff; | |
.full-screen .navigation .btn-screen:hover { | |
: #201e1e; | |
button { | |
: 30px; | |
: 30px; | |
: none; | |
: none; | |
: 0.5rem; | |
: 1.5rem; | |
: 30px; | |
: center; | |
: pointer; | |
.btn { | |
: #464646; | |
: #ffffff; | |
: 0.25rem; | |
: 0; | |
: scale(0); | |
.btn.show { | |
: 1; | |
: scale(1); | |
: visible; | |
.btn-screen { | |
: transparent; | |
: #b6b6b6; | |
: hidden; | |
{ | |
: 1; | |
: scale(1); | |
: visible; | |
{ | |
: #ffffff; | |
: 0px 10px 30px rgba(0, 0, 0, 0.1); | |
.content { | |
: 2em; | |
: 100%; | |
: calc(100% - 100px); | |
: 11; | |
.content.grid { | |
: grid; | |
.content.grid.center { | |
: center; | |
: center; | |
: center; | |
.title { | |
: 3em; | |
: purple; | |
.sub-title { | |
: 2.5em; | |
: purple; | |
p { | |
: 1.25em; | |
: 1rem; | |
.slide { | |
: absolute; | |
: 0; | |
: 0; | |
: 100%; | |
: 100%; | |
: #ffffff; | |
: 0; | |
: scale(0); | |
: none; | |
{ | |
: 1; | |
: scale(1); | |
: visible; | |
.heading { | |
: 2rem; | |
: purple; | |
: 2em; | |
: bold; | |
: #ffffff; | |
4. Enable Slide Navigation
Whenever we click on the left or right icon, we want the next slide or previous slide to appear. We also want to be able to toggle between full-screen mode and small-screen mode.
Furthermore, we want the slide's counter to display the accurate slide number on every slide. All these features will be enabled with JavaScript.
Inside js/index.js , we'll begin by storing references to the presentation wrapper, the slides, and the active slide:
slidesParentDiv = document.querySelector('.slides'); | |
slides = document.querySelectorAll('.slide'); | |
currentSlide = document.querySelector('.slide.show'); |
Next, we'll store references to the slide counter and both of the slide navigators (left and right icons):
slideCounter = document.querySelector('.counter'); | |
leftBtn = document.querySelector('#left-btn'); | |
rightBtn = document.querySelector('#right-btn'); |
Then store references to the whole presentation container and both button icons for going into full screen and small screen mode:
presentationArea = document.querySelector('#presentation-area'); | |
fullScreenBtn = document.querySelector('#full-screen'); | |
smallScreenBtn = document.querySelector('#small-screen'); |
Now that we're done with the references, we'll initialize some variables with default values:
screenStatus = 0; | |
currentSlideNo = 1 | |
totalSides = 0; |
screenStatus represents the screen orientation. 0 represents a full screen mode, and 1 represents a small screen mode.
currentSlideNo represents the current slide number, which as expected is the first slide. totalSlides is initialized with 0, but this will be replaced by the actual number of our slides.
Moving the Presentation to the Next and Previous Slides
Next, we'll add click event listeners to the left button, right button, full screen button, and small screen button:
.addEventListener('click', moveToLeftSlide); | |
.addEventListener('click', moveToRightSlide); | |
.addEventListener('click', fullScreenMode); | |
.addEventListener('click', smallScreenMode); |
We bind corresponding functions that will run when the click event is triggered on the corresponding element.
Here are the two functions responsible for changing the slide:
moveToLeftSlide() { | |
tempSlide = currentSlide; | |
= currentSlide.previousElementSibling; | |
.classList.remove('show'); | |
.classList.add('show'); | |
moveToRightSlide() { | |
tempSlide = currentSlide; | |
= currentSlide.nextElementSibling; | |
.classList.remove('show'); | |
.classList.add('show'); | |
In the function moveToLeftSlide , we basically access the previous sibling element (i.e. the previous slide), remove the .show class on the current slide, and add it to that sibling. This will move the presentation to the previous slide.
We do the exact opposite of this in the function moveToRightSlide . Because nextElementSibling is the opposite of previousElementSibling , we'll be getting the next sibling instead.
Code for Showing the Presentation in Full Screen and Small Screen
Recall that we also added click event listeners to the full screen and small screen icons. Here's the function responsible for toggling full-screen mode:
fullScreenMode() { | |
.classList.add('full-screen'); | |
.classList.remove('show'); | |
.classList.add('show'); | |
= 1; | |
smallScreenMode() { | |
.classList.remove('full-screen'); | |
.classList.add('show'); | |
.classList.remove('show'); | |
= 0; | |
Recall that presentationArea refers to the element that wraps the whole presentation. By adding the class full-screen to this element, we trigger the CSS that will expand it to take up the whole screen.
Since we're now in full-screen mode, we need to show the icon for reverting back to the small screen by adding the class .show to it. Finally, we update the variable screenStatus to 1.
For the smallScreenMode function, we do the opposite—we remove the class full-screen , show the expand button icon, and update screenStatus .
Hiding the Left and Right Icons on the First and Last Slides
Now, we need to invent a way to hide the left and right buttons when we're on the first slide and last slide respectively.
We'll use the following two functions to achieve this:
hideLeftButton() { | |
(currentSlideNo == 1) { | |
.classList.remove('show'); | |
else { | |
.classList.add('show'); | |
hideRightButton() { | |
(currentSlideNo === totalSides) { | |
.classList.remove('show'); | |
else { | |
.classList.add('show'); | |
Both these functions perform a very simple task: they check for the current slide number and hide the left and right buttons when the presentation is pointing to the first and last slide respectively.
Updating and Displaying the Slide Number
Because we're making use of the variable currentSlideNo to hide or show the left and right button icons, we need a way to update it as the user navigates through the slides. We also need to display to the user what slide they are currently viewing.
We'll create a function getCurrentSlideNo to update the current slide number:
getCurrentSlideNo() { | |
counter = 0; | |
.forEach((slide, i) => { | |
++ | |
(slide.classList.contains('show')){ | |
= counter; | |
We start the counter at 0, and for each slide on the page, we increment the counter. We assign the active counter (i.e. with the class .show ) to the currentSlideNo variable.
With that in place, we create another function that inserts some text into the slide counter:
setSlideNo() { | |
.innerText = `${currentSlideNo} of ${totalSides}` | |
So if we were on the second slide, for example, the slide's counter would read: "2 of 6".
Putting Everything Together
To ensure that all of these functions run in harmony, we'll run them in a newly created init function that we'll execute at the start of the script, just below the references:
(); | |
init() { | |
(); | |
= slides.length | |
(); | |
(); | |
(); | |
We must also run init() at the bottom of both the moveToLeftSlide and moveToRightSlide functions:
moveToLeftSlide() { | |
(); | |
moveToRightSlide() { | |
(); | |
This will ensure that the init function runs every time the user navigates left or right in the presentation.
Wrapping Up
I hope this tutorial helped you understand basic web development better. Here we built a presentation slideshow from scratch using HTML, CSS, and JavaScript. It's a great way to get into creating dynamic HTML with JavaScript PPT
With this project, you should have learned some basic HTML, CSS, and JavaScript syntax to help you with web development.

This is a PHP TEST
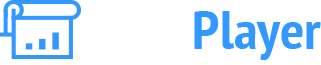
- My presentations
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
Introduction to HTML and CSS
Published by Helen Higgins Modified over 9 years ago
Similar presentations
Presentation on theme: "Introduction to HTML and CSS"— Presentation transcript:
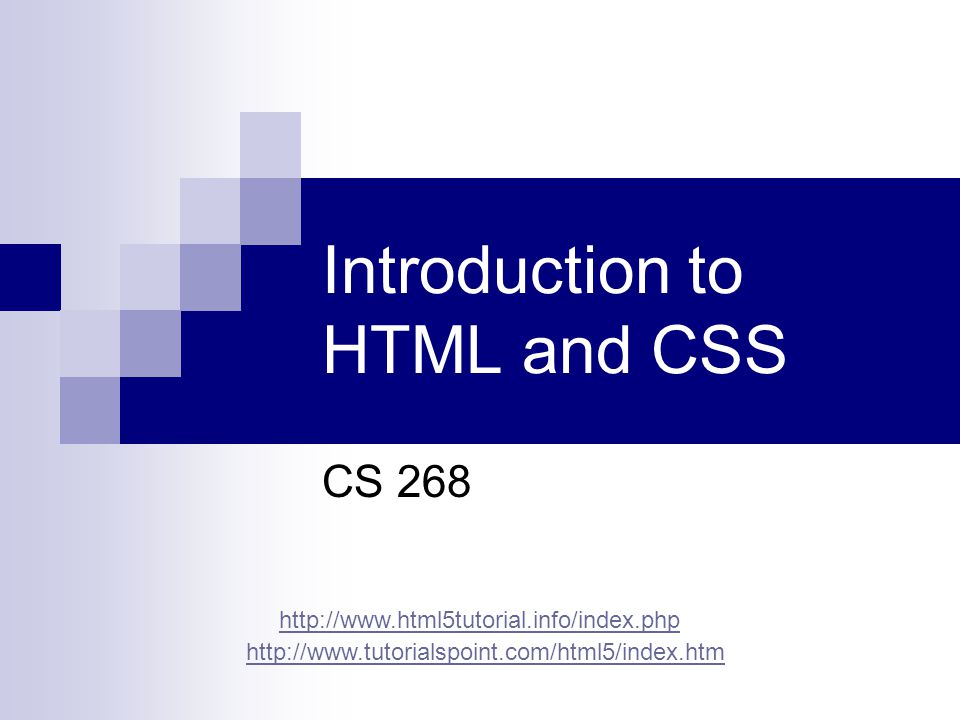
HTML Basics Customizing your site using the basics of HTML.
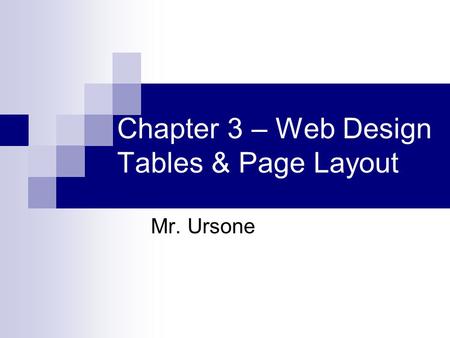
Chapter 3 – Web Design Tables & Page Layout
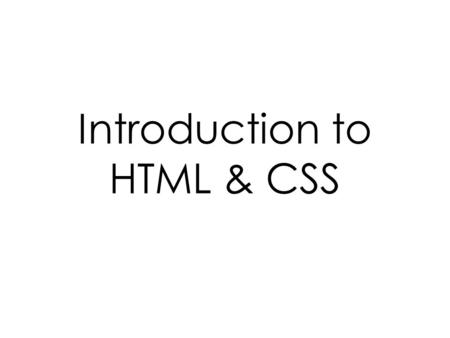
Introduction to HTML & CSS
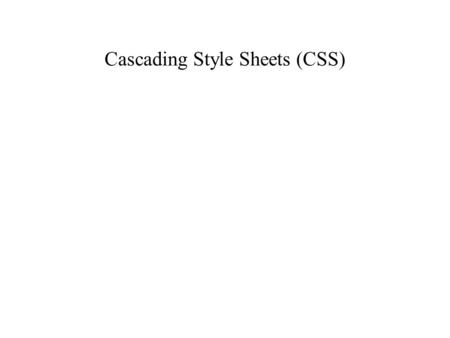
Cascading Style Sheets (CSS). Cascading Style Sheets With the explosive growth of the World Wide Web, designers and programmers quickly explored and reached.
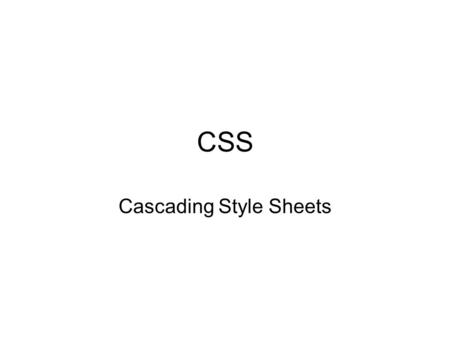
CSS Cascading Style Sheets. Objectives Using Inline Styles Working with Selectors Using Embedded Styles Using an External Style Sheet Applying a Style.
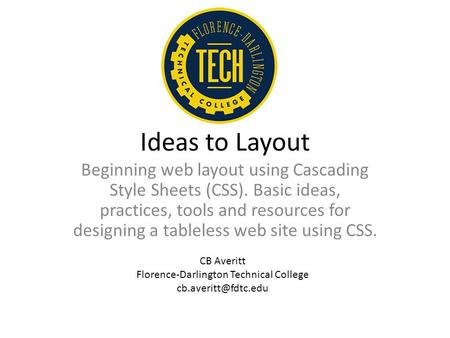
Ideas to Layout Beginning web layout using Cascading Style Sheets (CSS). Basic ideas, practices, tools and resources for designing a tableless web site.
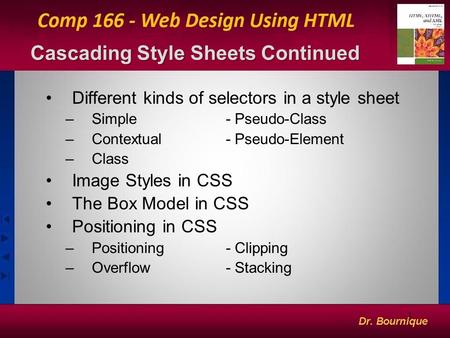
1 Cascading Style Sheets Continued Different kinds of selectors in a style sheet –Simple- Pseudo-Class –Contextual- Pseudo-Element –Class Image Styles.
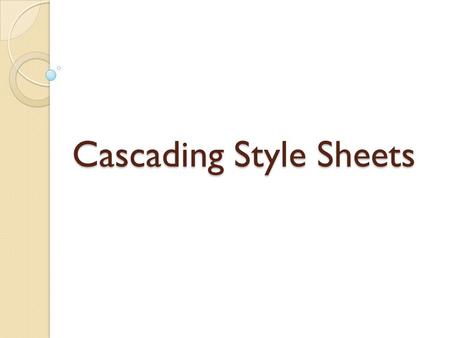
Cascading Style Sheets
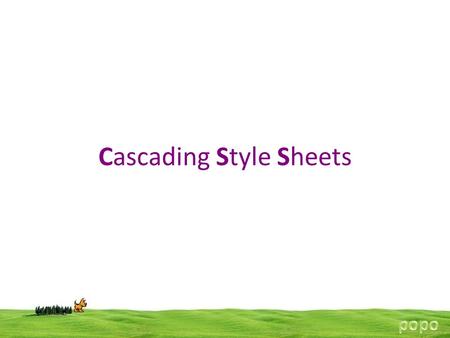
Cascading Style Sheets. CSS stands for Cascading Style Sheets and is a simple styling language which allows attaching style to HTML elements. CSS is a.
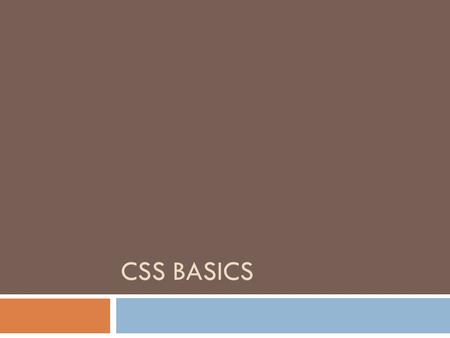
CSS BASICS. CSS Rules Components of a CSS Rule Selector: Part of the rule that targets an element to be styled Declaration: Two or more parts: a.
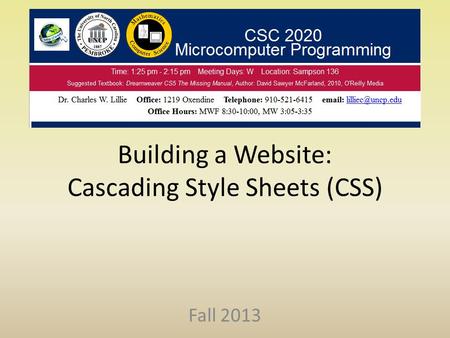
Building a Website: Cascading Style Sheets (CSS) Fall 2013.
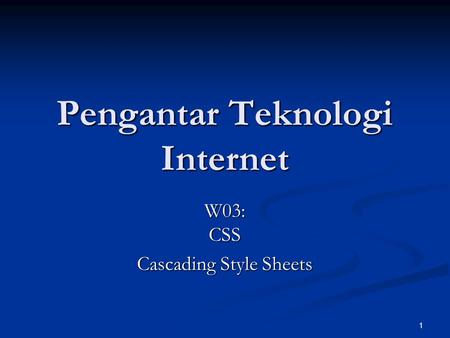
1 Pengantar Teknologi Internet W03: CSS Cascading Style Sheets.
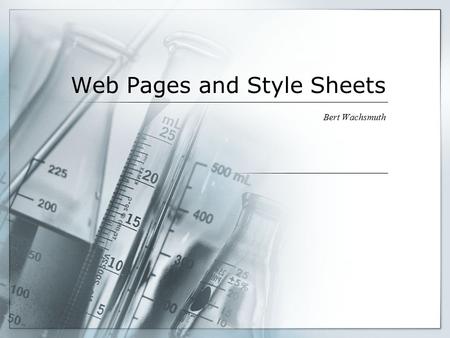
Web Pages and Style Sheets Bert Wachsmuth. HTML versus XHTML XHTML is a stricter version of HTML: HTML + stricter rules = XHTML. XHTML Rule violations:
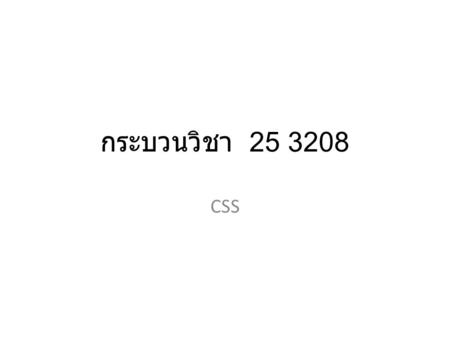
กระบวนวิชา CSS. What is CSS? CSS stands for Cascading Style Sheets Styles define how to display HTML elements Styles were added to HTML 4.0 to.
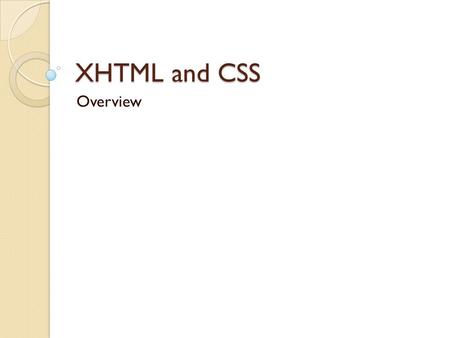
XHTML and CSS Overview. Hypertext Markup Language A set of markup tags and associated syntax rules Unlike a programming language, you cannot describe.
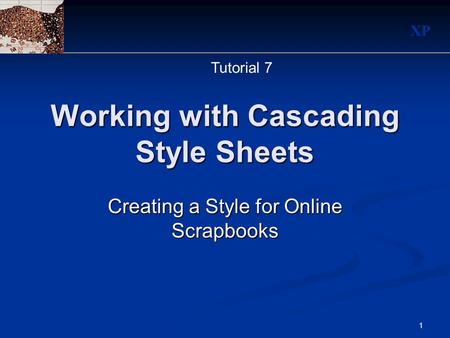
XP 1 Working with Cascading Style Sheets Creating a Style for Online Scrapbooks Tutorial 7.
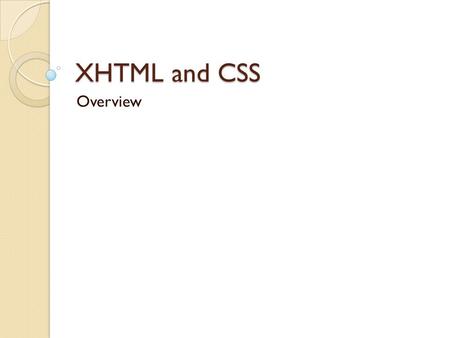
Cascading Style Sheet (CSS) Instructor: Dr. Fang (Daisy) Tang
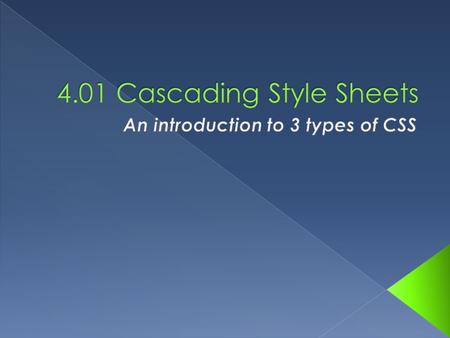
4.01 Cascading Style Sheets
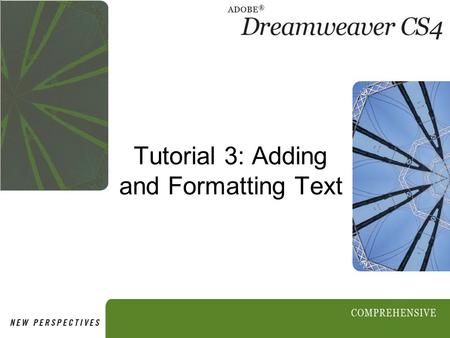
Tutorial 3: Adding and Formatting Text. 2 Objectives Session 3.1 Type text into a page Copy text from a document and paste it into a page Check for spelling.
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
Create beautiful stories
WebSlides makes HTML presentations easy. Just the essentials and using lovely CSS.
WebSlides 1.5.0 Github
Why WebSlides?
Good karma & Productivity.
An opportunity to engage.
WebSlides is about good karma. This is about telling the story, and sharing it in a beautiful way. HTML and CSS as narrative elements.
Work better, faster.
Designers, marketers, and journalists can now focus on the content. Simply choose a demo and customize it in minutes.
WebSlides is really easy
Each parent <section> in the #webslides element is an individual slide.
Code is clean and scalable. It uses intuitive markup with popular naming conventions. There's no need to overuse classes or nesting. Making an HTML presentation has never been so fast .
→ Simple Navigation
Slide counter, 40 + beautiful components, vertical rhythm, 500 + svg icons, webslides demos.
Contribute on Github . View all ›
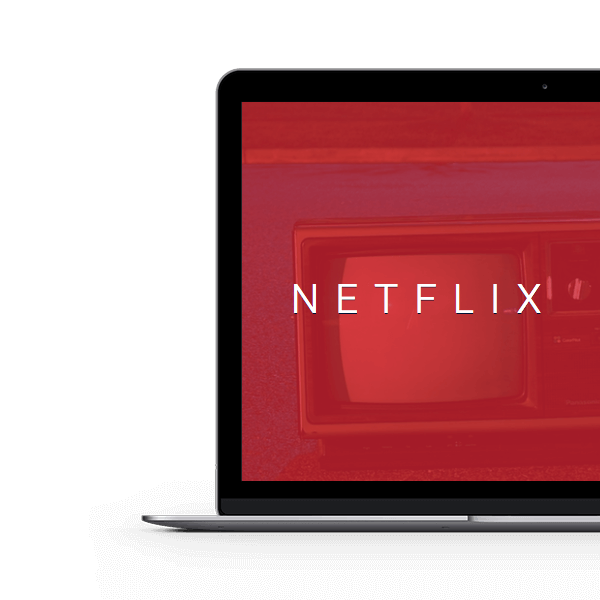
If you need help, here's just some tutorials. Just a basic knowledge of HTML is required:
- Components · Classes .
- WebSlides on Codepen .
- WebSlides Media: images, videos...
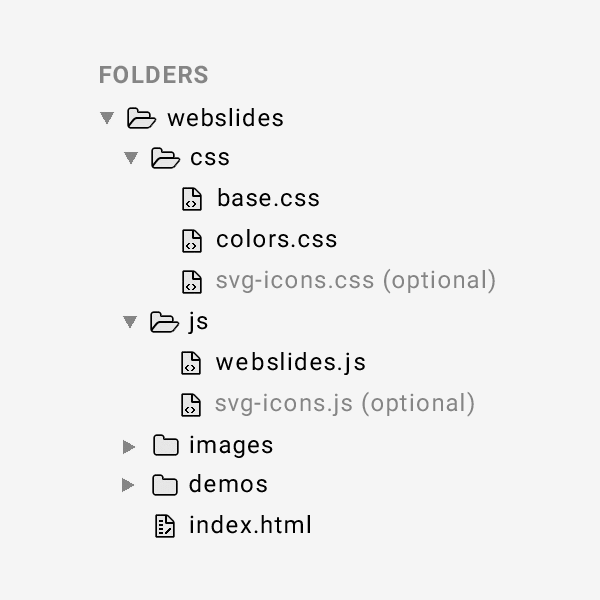
Built to expand
The best way to inspire with your content is to connect on a personal level:
- Background images: Unsplash .
- CSS animations: Animate.css .
- Longforms: Animate on scroll .
Ready to Start?
Create your own stories instantly. 120+ premium slides ready to use.
Free Download Pay what you want.
People share content that makes them feel inspired. WebSlides is a very effective way to engage young audiences, customers, and teams.
@jlantunez , @ant_laguna , and @luissacristan .
- Request PPT
- Privacy Policy
HTML Basics PPT
- Share on Facebook
- Share on Twitter
- Share on Google+
- Share on Reddit
- Share on Pinterest
- Share on Linkedin
- Share on Tumblr
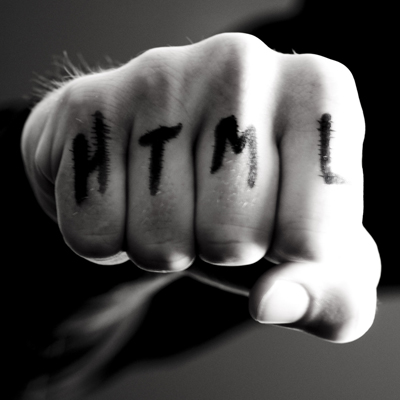
HTML (abbreviated as HyperText Markup Language) is a computer language used to develop web pages with the help of various tags and attributes. The major advantage is that it is easy to learn and code even for a newbie. Almost every web browsers and websites use HTML for creating web contents. So, it is necessary for everyone to know about HTML and its capabilities.
If you are new to HTML, have a look at the below HTML Basics PPT:
Download (PPTX, 1.2MB)
- More By Yogi
- More In Computer
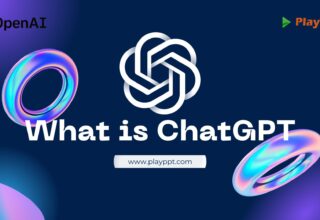
What is ChatGPT PPT
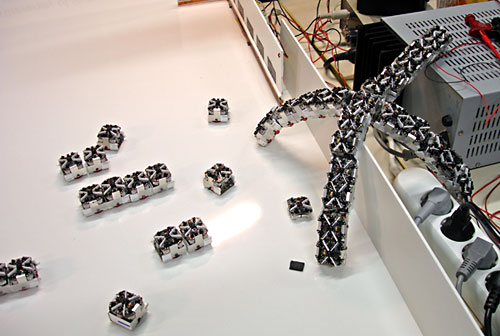
Swarm Robotics PPT
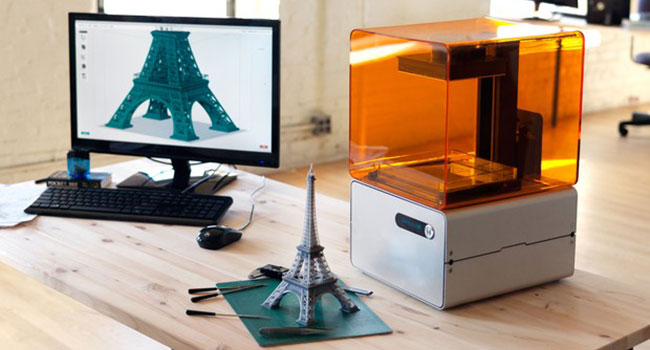
3D Printer PPT
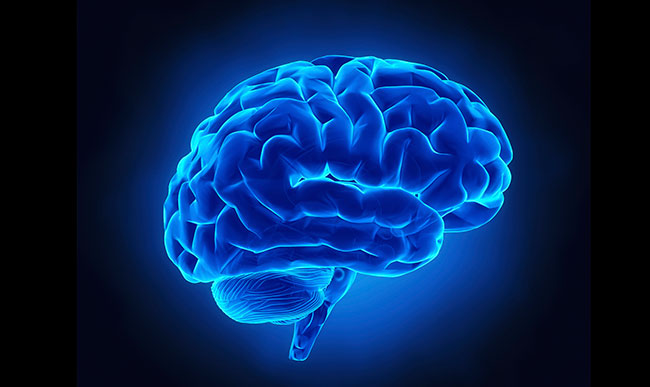
Blue Brain PPT
ChatGPT is a conversational language model developed by OpenAI. It is based on the GPT (Ge…
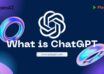
Bio Mechatronic Hand PPT
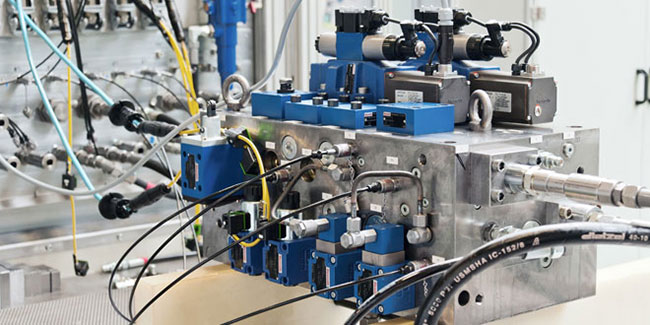
Hydraulics PPT
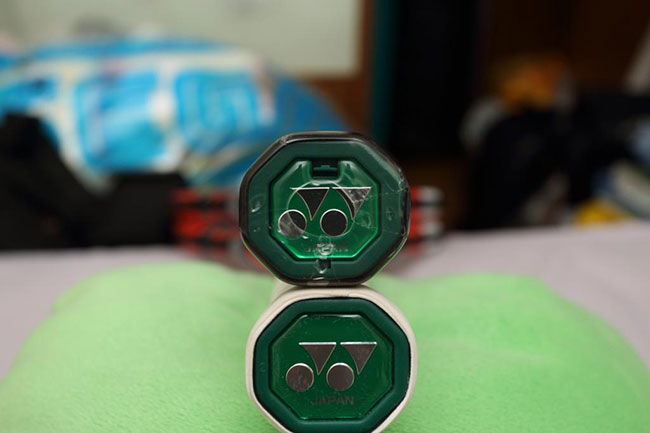
Smart Sensors PPT
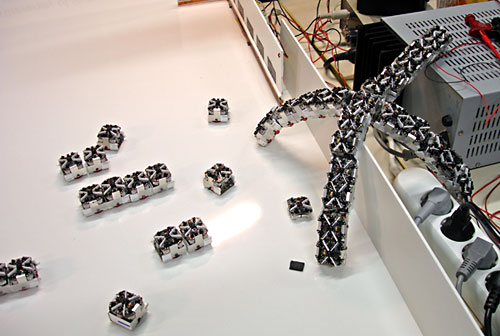

How to Create Beautiful HTML & CSS Presentations with WebSlides
Share this article
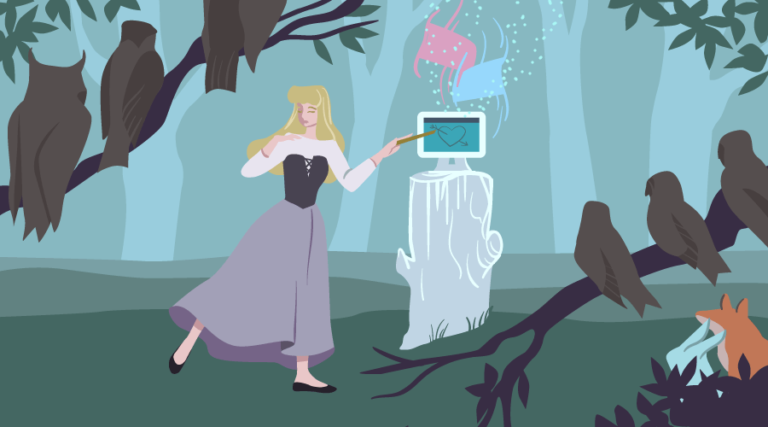
Getting Started with WebSlides
Create a web presentation with webslides.
- Frequently Asked Questions (FAQs) about Creating Beautiful HTML & CSS Presentations with WebSlides
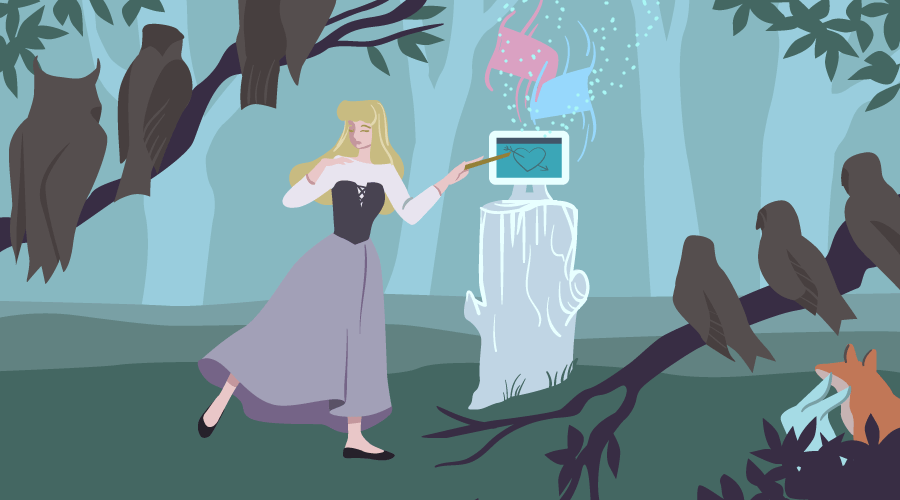
This article was peer reviewed by Ralph Mason , Giulio Mainardi , and Mikhail Romanov . Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
Presentations are one of the best ways to serve information to an audience. The format is short and sharp, made up of small, digestible chunks, which makes any topic under discussion engaging and easier to understand. A presentation can contain all kinds of data, represented by many different elements, such as tables, charts, diagrams, illustrations, images, videos, sounds, maps, lists, etc, all of which lends great flexibility to this medium of expression.
Particularly on the web, presentations come in handy on many occasions, and there are loads of tools at your disposal to create some nifty ones. Today, I’ll introduce you to WebSlides — a small and compact library with a nice set of ready-to-use components, which you can leverage to build well-crafted and attractive web presentations:
WebSlides “is about telling the story, and sharing it in a beautiful way.”
In fact, one of WebSlides’ main benefits is that you can share your story beautifully and in a variety of different ways. With one and the same architecture — 40+ components with semantic classes, and clean and scalable code — you can create portfolios, landings, longforms, interviews, etc.
Besides, you can also extend WebSlides’ functionality by combining it with third-party services and tools such as Unsplash , Animate.css , Animate On Scroll , and so on.
WebSlides is easy to learn and fun to use. Let’s see it in action now.
To get started, first download WebSlides . Then, in the root folder, create a new folder and call it presentation . Inside the newly created presentation folder, create a new file and call it index.html . Now, enter the following code, which contains the needed references to the WebSlides’ files (make sure the filepaths correspond to the folder structure in your setup):
In this section you’re going to create a short, but complete presentation, which explains why SVG is the future of web graphics. Note: If you are interested in SVG, please check my articles: SVG 101: What is SVG? and How to Optimize and Export SVGs in Adobe Illustrator .
You’ll be working step by step on each slide. Let’s get started with the first one.
The first slide is pretty simple. It contains only one sentence:
Each parent <section> inside <article id="webslides"> creates an individual slide. Here, you’ve used two classes from WebSlides’ arsenal, i.e., bg-gradient-r and aligncenter , to apply a radial gradient background and to align the slide content to the center respectively.
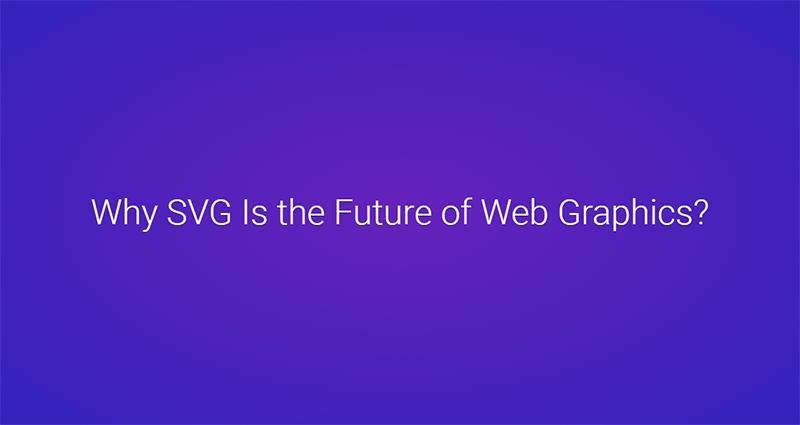
The second slide explains what SVG is:
The code above uses the content-left and content-right classes to separate the content into two columns. Also, in order to make the above classes work, you need to wrap all content by using the wrap class. On the left side, the code uses text-subtitle to make the text all caps, and text-intro to increase the font size. The right side consists of an illustrative image.
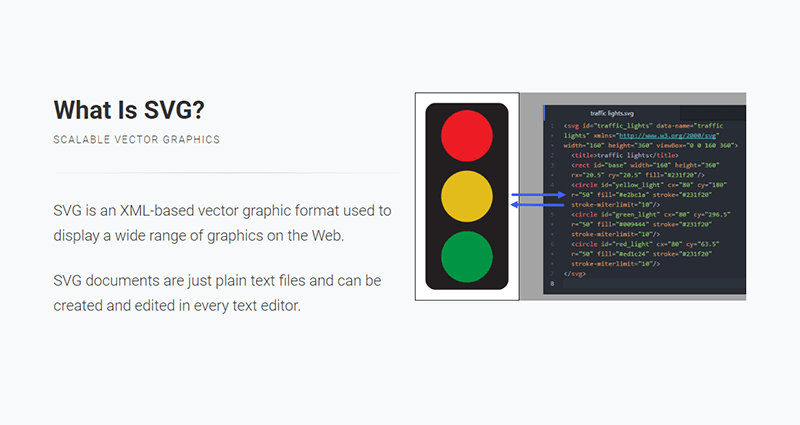
The next slide uses the grid component to create two columns:
The snippet above shows how to use the grid and column classes to create a grid with two columns. In the first column the style attribute aligns the text to the left (Note how the aligncenter class on the <section> element cascades through to its .column child element, which causes all text inside the slide to be center aligned). In the second column, the browser class makes the illustrative image look like a screenshot.
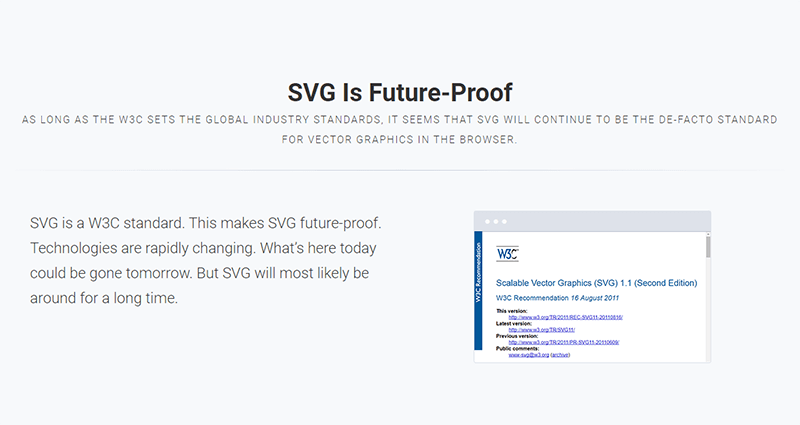
In the fourth slide, use the grid component again to split the content into two columns:
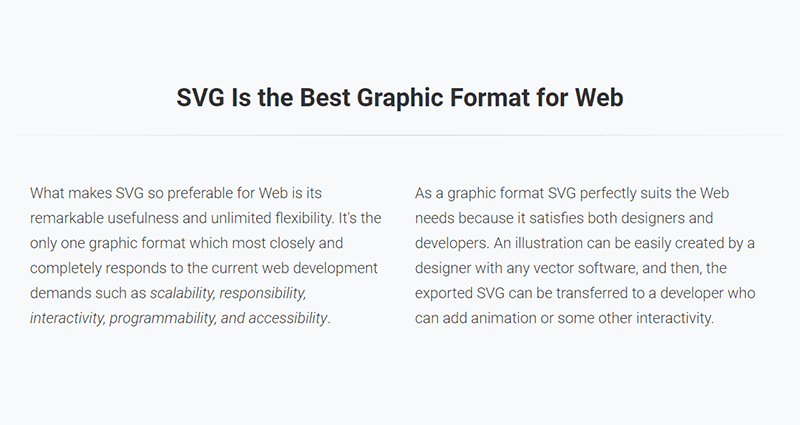
In this slide, place half of the content to the left and the other half to the right using the content-left and content-right classes respectively:
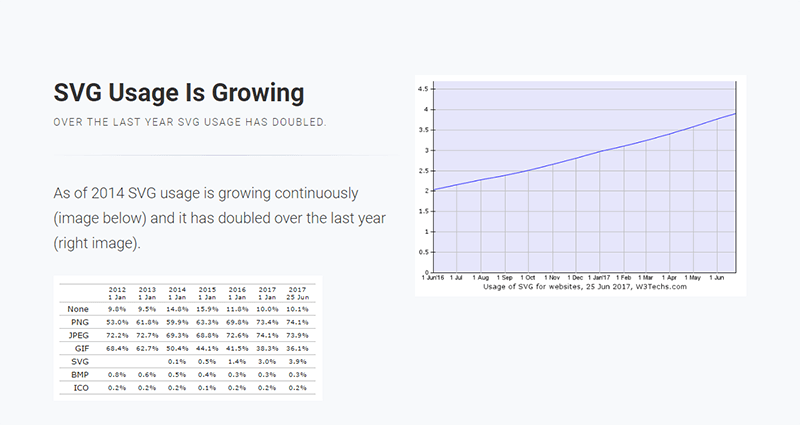
In this slide, use the background class to embed an image as a background with the Unsplash service . Put the headline on light, transparent background by using the bg-trans-light class. The text’s color appears white, because the slide uses a black background with the bg-black class, therefore the default color is inversed, i.e., white on black rather than black on white. Also, for the text to be visible in front of the image, wrap it with <div class="wrap"> :
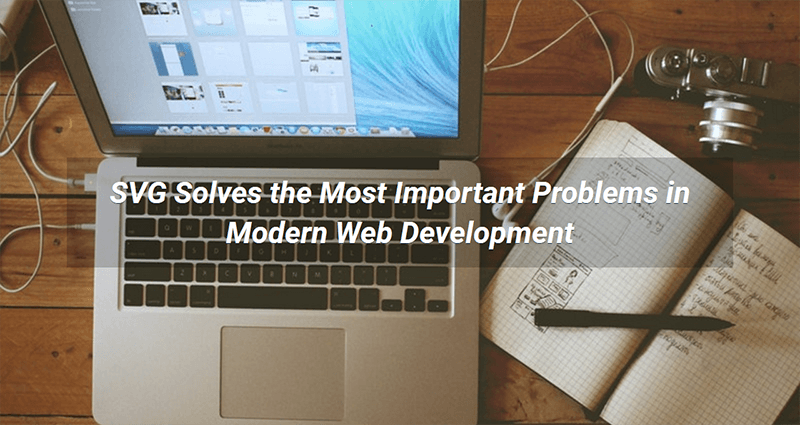
In this slide, put the explanation text on the left and the illustrative image on the right at 40% of its default size (with the alignright and size-40 classes on the <img> element). For this and the next three slides, use slideInRight , which is one of WebSlides’ built-in CSS animations:
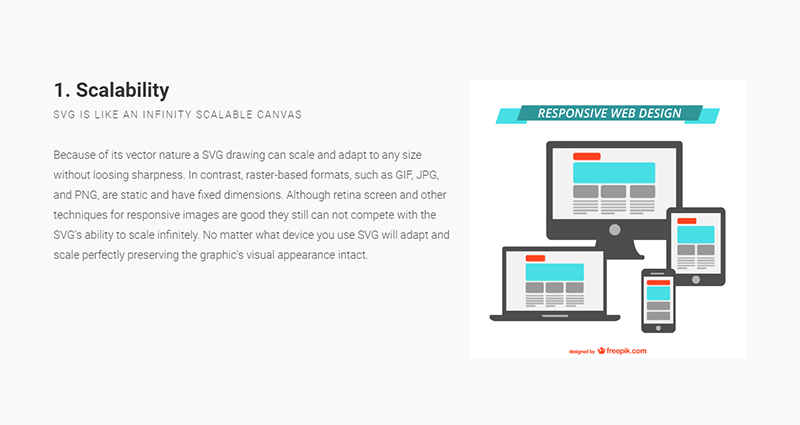
Do a similar thing here:
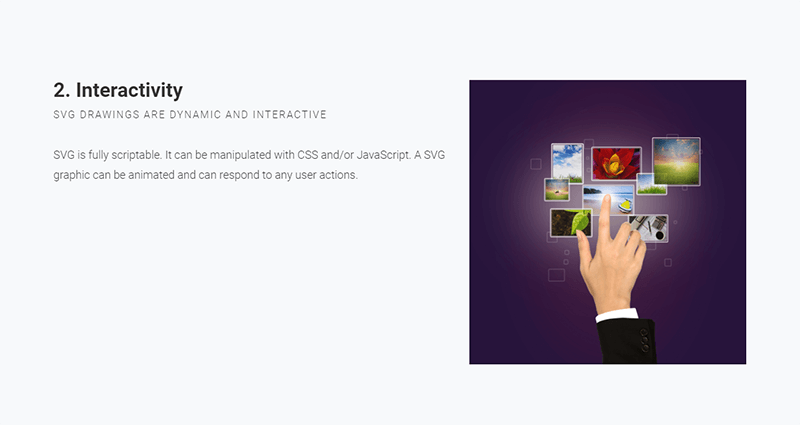
This slide also uses a similar structure:
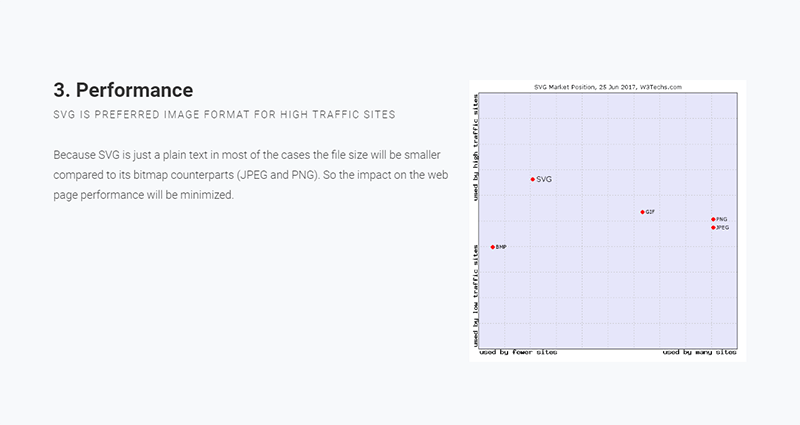
Here, divide the content into left and right again. In the second <p> tag, use the inline style attribute to adjust the font-size and line-height properties. Doing so will override the text-intro class styles that get applied to the element by default. On the right side, use <div class="wrap size-80"> to create a container for the SVG code example:
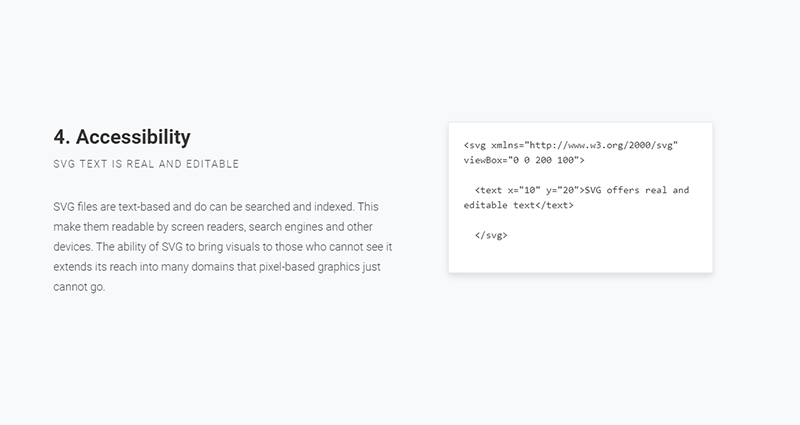
Here, leverage some of the classes you’ve already used to illustrate browser support for SVG:
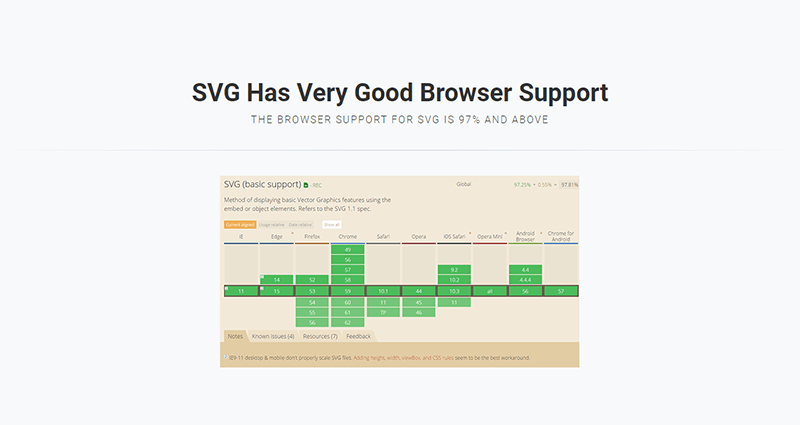
In this slide, show some of the use cases for SVG in the form of an image gallery. To this end, use an unordered list with the flexblock and gallery classes. Each item in the gallery is marked up with a li tag:
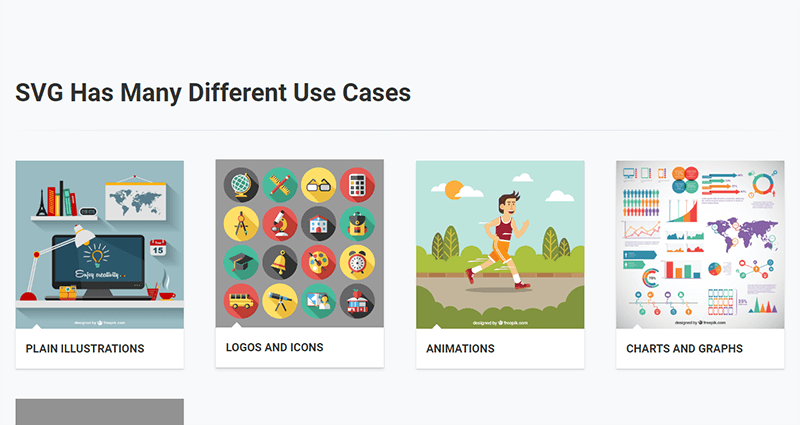
This section shows a typical SVG workflow, so you need to use the flexblock and steps classes, which show the content as a sequence of steps. Again, each step is placed inside a li tag:
For each step after the first one, you need to add the process-step-# class. This adds a triangle pointing to the next step.
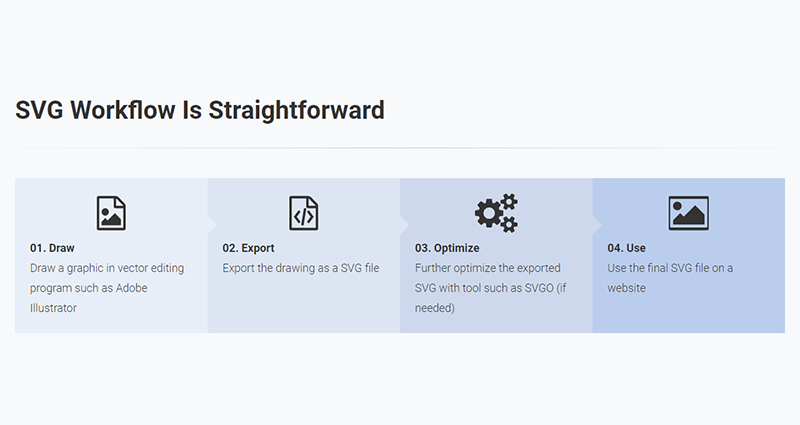
In the last slide, use another one of WebSlides’ built-in CSS animations, i.e., zoomIn :
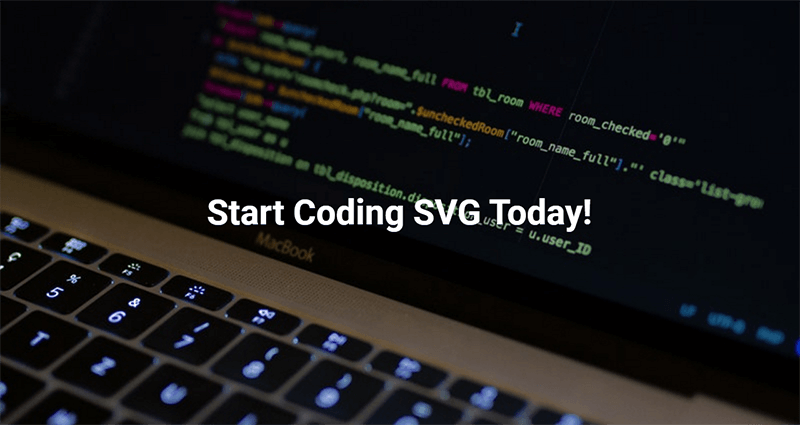
Congratulations! You’re done. You can see the final outcome here:
See the Pen HTML and CSS Presentation Demo with WebSlides by SitePoint ( @SitePoint ) on CodePen .
Et voilà! You have just created a beautiful, fully functional and responsive web presentation. But this is just the tip of the iceberg, there’s a lot more you can quickly create with WebSlides and many other WebSlides features which I didn’t cover in this short tutorial.
To learn more, explore the WebSlides Components and CSS architecture documentation , or start customizing the demos already available to you in the downloadable folder.
Then, focus on your content and let WebSlides do its job.
Frequently Asked Questions (FAQs) about Creating Beautiful HTML & CSS Presentations with WebSlides
How can i customize the design of my webslides presentation.
WebSlides allows you to customize your presentation to suit your style and needs. You can change the color scheme, fonts, and layout by modifying the CSS file. If you’re familiar with CSS, you can easily tweak the styles to create a unique look. If you’re not, there are plenty of online resources and tutorials that can help you learn. Remember, the key to a great presentation is not only the content but also the design. A well-designed presentation can help keep your audience engaged and make your content more memorable.
Can I add multimedia elements to my WebSlides presentation?
How can i share my webslides presentation with others.
Once you’ve created your WebSlides presentation, you can share it with others by hosting it on a web server. You can use a free hosting service like GitHub Pages, or you can use your own web server if you have one. Once your presentation is hosted, you can share the URL with anyone you want to view your presentation. They’ll be able to view your presentation in their web browser without needing to install any special software.
Can I use WebSlides for commercial projects?
Yes, WebSlides is free to use for both personal and commercial projects. You can use it to create presentations for your business, for your clients, or for any other commercial purpose. However, please note that while WebSlides itself is free, some of the images and fonts used in the templates may be subject to copyright and may require a license for commercial use.
How can I add interactive elements to my WebSlides presentation?
You can add interactive elements to your WebSlides presentation by using JavaScript. For example, you can add buttons that the user can click to navigate to different slides, or you can add forms that the user can fill out. This can be done by adding the appropriate HTML and JavaScript code to your slides. If you’re not familiar with JavaScript, there are plenty of online resources and tutorials that can help you learn.
Can I use WebSlides offline?
Yes, you can use WebSlides offline. Once you’ve downloaded the WebSlides files, you can create and view your presentations offline. However, please note that some features may not work offline, such as loading external images or fonts. To ensure that all features work correctly, it’s recommended to host your presentation on a web server.
How can I add transitions and animations to my WebSlides presentation?
You can add transitions and animations to your WebSlides presentation by using CSS. CSS allows you to control the appearance and behavior of elements on your slides, including transitions and animations. For example, you can use the transition property to animate the change of a property from one value to another, or you can use the animation property to create more complex animations.
Can I use WebSlides on mobile devices?
Yes, WebSlides is designed to be responsive and works well on both desktop and mobile devices. However, please note that due to the smaller screen size, some elements may not display as intended on mobile devices. It’s recommended to test your presentation on different devices to ensure that it looks and works well on all platforms.
How can I add navigation controls to my WebSlides presentation?
You can add navigation controls to your WebSlides presentation by using the built-in navigation options. You can add arrows to navigate between slides, or you can add a slide counter to show the current slide number and the total number of slides. This can be done by adding the appropriate HTML and CSS code to your slides.
Can I use WebSlides with other web development tools?
Yes, you can use WebSlides with other web development tools. For example, you can use it with a text editor to write your HTML and CSS code, or you can use it with a version control system like Git to manage your project files. You can also use it with a build tool like Gulp or Grunt to automate tasks like minifying your code or compiling your CSS.
I am a web developer/designer from Bulgaria. My favorite web technologies include SVG, HTML, CSS, Tailwind, JavaScript, Node, Vue, and React. When I'm not programming the Web, I love to program my own reality ;)
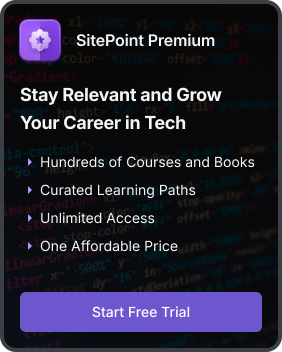

- Preferences
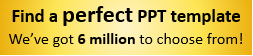
HTML5 Tutorial for Beginners - PowerPoint PPT Presentation

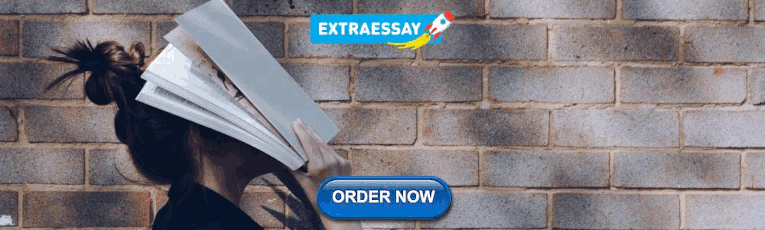
HTML5 Tutorial for Beginners
In this tutorial we provide all the details of all 40 above html tags with examples. html5 is just a new version of html and with it you get some new features which make it easier. these features are layout clearer, header, footer and all. so just visit here to get full tutorial now. – powerpoint ppt presentation.
- Here are few features of HTML5
- New parsing rules which are not based on SGML but are oriented towards flexible parsing and compatibility.
- Support use of inline Scalar Vector Graphics (SVG) and Mathematical Markup Language (MathML) in text/html.
- Many new elements are includes article, aside, audio, canvas, command, data list, details, embed, fig caption, figure, footer, header, nav, output, progress, rp, rt, ruby, section, source, summary, time, video and wbr and many more
- There are new available types of form controls include dates and times, email, url, search, number, range, tel and color.
- Also New available attributes of charset on meta and async on script.
- Worldwide/ Global attributes that can be applied for every element that include id, tabindex, hidden, data- or customer data attributes.
- In HTML 5 allows you to play a video and audio file.
- HTML 5 allows you to draw on a canvas.
- It is facilitates you to design better forms and build web applications that work offline.
- With HTML 5 provides you advance features for that you would normally have to write JavaScript to do.
- Main important reason to use HTML 5 is, we believe it is not going anywhere. It will be here to serve for a long time according to W3C recommendation.
- List of HTML 5 Tags
- ltarticlegt
- ltdetailsgt
- ltfigcaptiongt
- ltsectiongt
- ltsummarygt
PowerShow.com is a leading presentation sharing website. It has millions of presentations already uploaded and available with 1,000s more being uploaded by its users every day. Whatever your area of interest, here you’ll be able to find and view presentations you’ll love and possibly download. And, best of all, it is completely free and easy to use.
You might even have a presentation you’d like to share with others. If so, just upload it to PowerShow.com. We’ll convert it to an HTML5 slideshow that includes all the media types you’ve already added: audio, video, music, pictures, animations and transition effects. Then you can share it with your target audience as well as PowerShow.com’s millions of monthly visitors. And, again, it’s all free.
About the Developers
PowerShow.com is brought to you by CrystalGraphics , the award-winning developer and market-leading publisher of rich-media enhancement products for presentations. Our product offerings include millions of PowerPoint templates, diagrams, animated 3D characters and more.
- [email protected]
Bootstraphunter
Free and Premium Bootstrap Templates and Themes
How to Create Presentation Slides with HTML and CSS
- March 15, 2022
As I sifted through the various pieces of software that are designed for creating presentation slides, it occurred to me: why learn yet another program, when I can instead use the tools that I’m already familiar with?
We can easily create beautiful and interactive presentations with HTML, CSS and JavaScript, the three basic web technologies. In this tutorial, we’ll use modern HTML5 markup to structure our slides, we’ll use CSS to style the slides and add some effects, and we’ll use JavaScript to trigger these effects and reorganize the slides based on click events.
This tutorial is perfect for those of you new to HTML5, CSS and JavaScript, who are looking to learn something new by building.
Here’s the final preview of the presentation slide we’re going to build:
You can also find the complete source code in the GitHub repo .
Let’s begin.
Table of Contents
1. Create the Directory Structure
Before we get started, let’s go ahead and create our folder structure; it should be fairly simple. We’ll need:
index.html css/style.css js/scripts.js
This is a simple base template. Your files remain blank for the time being. We’ll fill that shortly.
2. Create the Starter Markup
Let’s begin by creating the base markup for our presentation page. Paste the following snippet into your index.html file.
<!DOCTYPE html> <html lang=”en”> <head> <meta charset=”UTF-8″> <meta name=”viewport” content=”width=device-width, initial-scale=1.0″> <meta http-equiv=”X-UA-Compatible” content=”ie=edge”> <title>Document</title> <link rel=”stylesheet” href=”css/style.css”>
<!– Font Awesome Icon CDN –> <link rel=”stylesheet” href=”https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css” integrity=”sha512-9usAa10IRO0HhonpyAIVpjrylPvoDwiPUiKdWk5t3PyolY1cOd4DSE0Ga+ri4AuTroPR5aQvXU9xC6qOPnzFeg==” crossorigin=”anonymous” referrerpolicy=”no-referrer” /> </head> <body> <div class=”container” <div id=”presentation-area”> <!– slides go here –> </div> </div> <script src=”js/index.js” type=”text/javascript”></script> </body> </html>
From the base markup, you can tell that we are importing Font Awesome Icons, our stylesheet ( style.css ) and our JavaScript ( index.js ).
Now we’ll add the HTML markup for the actual slides inside the <div> wrapper:
<section class=”presentation”>
<!– Slide 1 –> <div class=”slide show”> <div class=”heading”> Presentation on C# </div> <div class=”content grid center”> <h3 class=”title”> What is C# ? <br /> All You Need To Know </h3> </div> </div>
<!– Slide 1 –> <div class=”slide”> <div class=”heading”> Overview </div> <div class=”content grid center”> <h3 class=”title”> Introduction to C+ </h3> <p class=”sub-title”> Basic and Advanced Concepts </p> <p>Lecture No. 1</p> <p>My Email Address</p> <p><a href=””> [email protected] </a></p> </div> </div>
<!– Add 5 more slides here –> </section>
We have seven slides in total, and each slide is comprised of the heading section and the content section.
Only one slide will be shown at a time. This functionality is handled by the .show class which will be implemented later on in our stylesheet.
Using JavaScript, later on, we’ll dynamically add the .show class to the active slide on the page.
Below the slides, we’ll add the markup for our slide’s counter and tracker:
<div id=”presentation-area”> <!– <section class=”slides”><-></section> –> <section class=”counter”> 1 of 6 </section> </div>
Later on, we’ll use JavaScript to update the text content as the user navigates through the slides.
Finally, we’ll add the slide navigator just below the counter:
<div id=”presentation-area”> <!– <section class=”slides”><-></section> –> <!– <section class=”counter”><-></section> –> <section class=”navigation”> <button id=”full-screen” class=”btn-screen show”> <i class=”fas fa-expand”></i> </button>
<button id=”small-screen” class=”btn-screen”> <i class=”fas fa-compress”></i> </button>
<button id=”left-btn” class=”btn”> <i class=”fas fa-solid fa-caret-left”></i> </button>
<button id=”right-btn” class=”btn”> <i class=”fa-solid fa-caret-right”></i> </button> </section> </div>
This section consists of four buttons responsible for navigating left and right and switching between full-screen mode and small-screen mode. Again, we’ll use the class .show to regulate which button appears at a time.
That’ll be all for the HTML part, let’s move over to styling.
3. Make It Pretty
Our next step takes place within our stylesheet. We’ll be focusing on both aesthetics as well as functionality here. To make each slide translate from left to right, we’ll need to target the class .show with a stylesheet to show the element.
Here’s the complete stylesheet for our project:
* { margin: 0; padding: 0; box-sizing: border-box; font-family: sans-serif; transition: all 0.5s ease; }
body { width: 100vw; height: 100vh; display: flex; align-items: center; justify-content: center; }
ul { margin-left: 2rem; }
ul li, a { font-size: 1.2em; }
.container { background: #212121; width: 100%; height: 100%; position: relative; display: flex; align-items: center; justify-content: center; }
#presentation-area { width: 1000px; height: 500px; position: relative; background: purple; }
/* Styling all three sections */ #presentation-area .presentation { width: 100%; height: 100%; overflow: hidden; background: #ffffff; position: relative; }
#presentation-area .counter { position: absolute; bottom: -30px; left: 0; color: #b6b6b6; }
#presentation-area .navigation { position: absolute; bottom: -45px; right: 0; }
/* On full screen mode */ #presentation-area.full-screen { width: 100%; height: 100%; overflow: hidden; }
#presentation-area.full-screen .counter { bottom: 15px; left: 15px; }
#presentation-area.full-screen .navigation { bottom: 15px; right: 15px; }
#presentation-area.full-screen .navigation .btn:hover { background: #201e1e; color: #ffffff; }
#presentation-area.full-screen .navigation .btn-screen:hover { background: #201e1e; } /* End full screen mode */
/* Buttons */ .navigation button { width: 30px; height: 30px; border: none; outline: none; margin-left: 0.5rem; font-size: 1.5rem; line-height: 30px; text-align: center; cursor: pointer; }
.navigation .btn { background: #464646; color: #ffffff; border-radius: 0.25rem; opacity: 0; transform: scale(0); }
.navigation .btn.show { opacity: 1; transform: scale(1); visibility: visible; }
.navigation .btn-screen { background: transparent; color: #b6b6b6; visibility: hidden; }
.btn-screen.show { opacity: 1; transform: scale(1); visibility: visible; }
.btn-screen.hover { color: #ffffff; box-shadow: 0px 10px 30px rgba(0, 0, 0, 0.1); } /* End Buttons */
/* content */ .presentation .content { padding: 2em; width: 100%; height: calc(100% – 100px); z-index: 11; }
.presentation .content.grid { display: grid; }
.presentation .content.grid.center { justify-content: center; align-items: center; text-align: center; }
.content .title { font-size: 3em; color: purple; }
.content .sub-title { font-size: 2.5em; color: purple; }
.content p { font-size: 1.25em; margin-bottom: 1rem; } /* End Content Stylesheet */
/* Slide */ .presentation .slide { position: absolute; top: 0; left: 0; width: 100%; height: 100%; background: #ffffff; opacity: 0; transform: scale(0); visibility: none; }
.slide.show { opacity: 1; transform: scale(1); visibility: visible; }
.slide .heading { padding: 2rem; background: purple; font-size: 2em; font-weight: bold; color: #ffffff; }
4. Enable Slide Navigation
Whenever we click on the left or right icon, we want the next slide or previous slide to appear. We also want to be able to toggle between full-screen mode and small-screen mode.
Furthermore, we want the slide’s counter to display the accurate slide number on every slide. All these features will be enabled with JavaScript.
Inside js/index.js , we’ll begin by storing references to the presentation wrapper, the slides, and the active slide:
let slidesParentDiv = document.querySelector(‘.slides’); let slides = document.querySelectorAll(‘.slide’); let currentSlide = document.querySelector(‘.slide.show’);
Next, we’ll store references to the slide counter and both of the slide navigators (left and right icons):
var slideCounter = document.querySelector(‘.counter’); var leftBtn = document.querySelector(‘#left-btn’); var rightBtn = document.querySelector(‘#right-btn’);
Then store references to the whole presentation container and both button icons for going into full screen and small screen mode:
let presentationArea = document.querySelector(‘#presentation-area’); var fullScreenBtn = document.querySelector(‘#full-screen’); var smallScreenBtn = document.querySelector(‘#small-screen’);
Now that we’re done with the references, we’ll initialize some variables with default values:
var screenStatus = 0; var currentSlideNo = 1 var totalSides = 0;
screenStatus represents the screen orientation. 0 represents a full screen mode and 1 represents a small screen mode.
currentSlideNo represents the current slide number, which as expected is the first slide. totalSlides is initialized with 0, but this will be replaced by the actual number of our slides.
Moving the Presentation to the Next and Previous Slides
Next, we’ll add click event listeners to the left button, right button, full screen button and small screen button:
leftBtn.addEventListener(‘click’, moveToLeftSlide); rightBtn.addEventListener(‘click’, moveToRightSlide);
fullScreenBtn.addEventListener(‘click’, fullScreenMode); smallScreenBtn.addEventListener(‘click’, smallScreenMode);
We bind corresponding functions that will run when the click event is triggered on the corresponding element.
Here are the two functions responsible for changing the slide:
function moveToLeftSlide() { var tempSlide = currentSlide; currentSlide = currentSlide.previousElementSibling; tempSlide.classList.remove(‘show’); currentSlide.classList.add(‘show’); }
function moveToRightSlide() { var tempSlide = currentSlide; currentSlide = currentSlide.nextElementSibling; tempSlide.classList.remove(‘show’); currentSlide.classList.add(‘show’); }
In the function moveToLeftSlide, we basically access the previous sibling element (ie. the previous slide), remove the .show class on the current slide and add it to that sibling. This will move the presentation to the previous slide.
We do the exact opposite of this in the function moveToRightSlide. Because nextElementSibling is the opposite of previousElementSibling, we’ll be getting the next sibling instead.
Code for Showing the Presentation in Full Screen and Small Screen
Recall that we also added click event listeners to the full screen and small screen icons.
Here’s the function responsible for toggling full-screen mode:
function fullScreenMode() { presentationArea.classList.add(‘full-screen’); fullScreenBtn.classList.remove(‘show’); smallScreenBtn.classList.add(‘show’);
screenStatus = 1; }
function smallScreenMode() { presentationController.classList.remove(‘full-screen’); fullScreenBtn.classList.add(‘show’); smallScreenBtn.classList.remove(‘show’);
screenStatus = 0; }
Recall that presentationArea refers to the element that wraps the whole presentation. By adding the class full-screen to this element, we trigger the CSS that will expand it to take up the whole screen.
Since we’re now in full-screen mode, we need to show the icon for reverting back to the small screen by adding the class .show to it. Finally, we update the variable screenStatus to 1.
For the smallScreenMode function, the opposite is done – we remove the class full-screen, show the expand button icon, and reupdate screenStatus.
Hidding Left and Right Icons in First and Last Slides
Now, we need to invent a way to hide both the left and right buttons when we’re on the first slide and last slide respectively.
We’ll use the following two functions to achieve this:
function hideLeftButton() { if(currentSlideNo == 1) { toLeftBtn.classList.remove(‘show’); } else { toLeftBtn.classList.add(‘show’); } }
function hideRightButton() { if(currentSlideNo === totalSides) { toRightBtn.classList.remove(‘show’); } else { toRightBtn.classList.add(‘show’); } }
Both these functions perform a very simple task: they check for the current slide number and hide the left and right buttons when the presentation is pointing to the first and last slide respectively.
Updating and Displaying Slide Number
Because we’re making use of the variable currentSlideNo to hide or show the left and right button icons, we need a way to update it as the user navigates through the slides.
We also need to display to the user what slide he or she is currently viewing.
We’ll create a function getCurrentSlideNo to update the current slide number:
function getCurrentSlideNo() { let counter = 0;
slides.forEach((slide, i) => { counter++
if(slide.classList.contains(‘show’)){ currentSlideNo = counter; } });
We start the counter at 0, and for each slide on the page, we increment the counter. We assign the active counter (ie. with the class .show) to the currentSlideNo variable.
With that in place, we create another function that inserts some text into the slide counter:
function setSlideNo() { slideNumber.innerText = `${currentSlideNo} of ${totalSides}` }
So if we were on the second slide for example, the slide’s counter will read as: 2 of 6
Putting Everything Together
To ensure that all of these functions run in harmony, we’ll run them in a newly created init function that we’ll execute at start of the script, just below the references:
function init() {
getCurrentSlideNo(); totalSides = slides.length setSlideNo(); hideLeftButton(); hideRightButton(); }
We must also run init() at the bottom of both the moveToLeftSlide and moveToRightSlide functions:
function moveToLeftSlide() { // other code
function moveToRightSlide() { // other code
This will ensure that the function init runs every time the user navigates left or right in the presentation.
Wrapping Up
I hope this tutorial helped you understand basic web development better. Here we built a presentation slideshow from scratch using HTML, CSS and JavaScript.
With this project, you should have learned some basic HTML, CSS and JavaScript syntax to help you with web development.
Recent Posts
- How Flatlogic Started Their Business
- Gulp is back – did it ever leave?
- Solving Memory Leaks in Node.js has Never Been Easier, Introducing the Latest Version of N|Solid
- Svelte 5 is almost here
- JSR isn’t another tool, it’s a fundamental shift

DEV Community

Posted on Jan 11, 2019
How To Build A Captivating Presentation Using HTML, CSS, & JavaScript
Building beautiful presentations is hard. Often you're stuck with Keynote or PowerPoint, and the templates are extremely limited and generic. Well not anymore.
Today, we're going to learn how to create a stunning and animated presentation using HTML, CSS, and JavaScript.
If you're a beginner to web development, don't fret! This tutorial will be easy enough to keep up with. So let's slide right into it!

We're going to be using an awesome framework called Reveal.js . It provides robust functionality for creating interesting and customizable presentations.
- Head over to the Reveal.js repository and clone the project (you can also fork this to your GitHub namespace).

- Change directories into your newly cloned folder and run npm install to download the package dependencies. Then run npm start to run the project.

The index.html file holds all of the markup for the slides. This is one of the downsides of using Reveal.js; all of the content will be placed inside this HTML file.

Built-In Themes
Reveal includes 11 built-in themes for you to choose from:

Changing The Theme
- Open index.html
- Change the CSS import to reflect the theme you want to use

The theme files are:
- solarized.css
Custom Themes
It's quite easy to create a custom theme. Today, I'll be using my custom theme from a presentation I gave called "How To Build Kick-Ass Website: An Introduction To Front-end Development."
Here is what my custom slides look like:

Creating A Custom Theme
- Open css/theme/src inside your IDE. This holds all of the Sass files ( .scss ) for each theme. These files will be transpiled to CSS using Grunt (a JavaScript task runner). If you prefer to write CSS, go ahead and just create the CSS file inside css/theme.
- Create a new .scss file. I will call mine custom.scss . You may have to stop your localhost and run npm run build to transpile your Sass code to CSS.
- Inside the index.html file, change the CSS theme import in the <head> tag to use the name of the newly created stylesheet. The extension will be .css , not .scss .
- Next, I created variables for all of the different styles I wanted to use. You can find custom fonts on Google Fonts. Once the font is downloaded, be sure to add the font URL's into the index.html file.
Here are the variables I chose to use:
- Title Font: Viga
- Content Font: Open Sans
- Code Font: Courier New
- Cursive Font: Great Vibes
- Yellow Color: #F9DC24
- Add a .reveal class to the custom Sass file. This will wrap all of the styles to ensure our custom theme overrides any defaults. Then, add your custom styling!
Unfortunately, due to time constraints, I'll admit that I used quite a bit of !important overrides in my CSS. This is horrible practice and I don't recommend it. The reveal.css file has extremely specific CSS styles, so I should have, if I had more time, gone back and ensured my class names were more specific so I could remove the !importants .
Mixins & Settings
Reveal.js also comes with mixins and settings you can leverage in your custom theme.
To use the mixins and settings, just import the files into your custom theme:
Mixins You can use the vertical-gradient, horizontal-gradient, or radial-gradient mixins to create a neat visual effect.
All you have to do is pass in the required parameters (color value) and voila, you've got a gradient!
Settings In the settings file, you'll find useful variables like heading sizes, default fonts and colors, and more!

The structure for adding new content is:
.reveal > .slides > section
The <section> element represents one slide. Add as many sections as you need for your content.
Vertical Slides
To create vertical slides, simply nest sections.
Transitions
There are several different slide transitions for you to choose from:
To use them, add a data-transition="{name}" to the <section> which contains your slide data.
Fragments are great for highlighting specific pieces of information on your slide. Here is an example.
To use fragments, add a class="fragment {type-of-fragment}" to your element.
The types of fragments can be:
- fade-in-then-out
- fade-in-then-semi-out
- highlight-current-blue
- highlight-red
- highlight-green
- highlight-blue
You can additionally add indices to your elements to indicate in which order they should be highlighted or displayed. You can denote this using the data-fragment-index={index} attribute.
There are way more features to reveal.js which you can leverage to build a beautiful presentation, but these are the main things which got me started.
To learn more about how to format your slides, check out the reveal.js tutorial . All of the code for my presentation can be viewed on GitHub. Feel free to steal my theme!
Top comments (18)
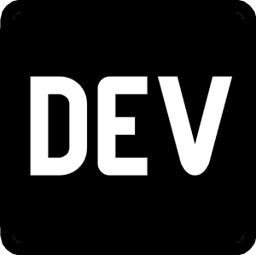
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Oct 2, 2018
I really love reveal.js. I haven't spoken in a while so I haven't used it. I've always used their themes and never thought about making my own. This is probably super useful for company presentations, too. I'm SO over google slides. Trying to format code in those is a nightmare LOL

- Location Stockholm
- Education Siena College
- Work Software Engineer at Spotify
- Joined Dec 21, 2018
Yeah it is time consuming, but the result is much better

- Location Antibes, France
- Work Senior Software Engineer at Spotify
- Joined Oct 16, 2017
The best thing in this - and now I'm not being ironic - is that while you work on a not so much technical task - creating a presentation - you still have to code. And the result is nice.
On the other hand, I know what my presentation skills teachers would say. Well, because they said it... :) If you really want to deliver a captivating presentation, don't use slides at all. Use the time to prepare what you want to say.
I'm not that good - yet, but taking their advice, if must I use few slides, with little information on them and with minimal graphical distractions. My goal is to impress them by what I say, not is what behind my head.
I'm going to a new training soon, where the first day we have to deliver a presentation supported by slides at a big auditorium and the next day we have to go back and forget about the slides and just get on stage and speak. I can't wait for it.

- Location Lake Villa, IL
- Education Bachelor in Electronics Engineering
- Work Computer & Technology Enthusiast
- Joined Oct 8, 2017
How about github.com/team-fluxion/slide-gazer ?
It's my fourth attempt at creating a simple presentation tool to help one present ideas quickly without having to spend time within a presentation editor like Microsoft PowerPoint. It directly converts markdown documents into elegant presentations with a few features and is still under development.

- Location Singapore
- Work Web Developer at FirstCom Solutions
- Joined Jan 15, 2019
Yup, RevealJS is awesome !
Previously I either used PPT or Google Slides. One is a paid license and the other requires an internet connection.
The cool thing about it is that since it's just HTML files behind the scenes, the only software you need to view it with is a web browser. Has amazing syntax-highlighting support via PrismJS. And as a web developer, it makes it simple to integrate other npm packages if need be...
I actually just used it to present a talk this week!

- Email [email protected]
- Location Indianapolis, IN
- Education Purdue University
- Pronouns he/him
- Work Senior Frontend Engineer at Whatnot
- Joined Aug 3, 2017
Great article, Emma! I love Reveal and this is a great write up for using it!

- Location New Delhi, India 🇮🇳
- Joined Dec 5, 2018
I think its a coincidence 😅 I was just starting to think to use reveal.js and suddenly you see this post 🤩

- Location Saratoga Springs,NY
- Education BA, University of Michigan
- Work Documentarian
- Joined Sep 7, 2018
Check out slides.com If you want to skip the heavy lifting and/or use a presentation platform based on reveal.js.
Everything is still easy to customize. The platform provides a UI to work from and an easy way to share your stuff.
BTW - I have no affiliation with slides.com, or even a current account. I used the service a few years back when I regularly presented and wanted to get over PowerPoint, Google Slides, Prezi, etc.
- Location Toronto, ON
- Education MFA in Art Video Syracuse University 2013 😂
- Work Rivalry
- Joined May 31, 2017
Well I guess you get to look ultra pro by skipping the moment where you have to adjust for display detection and make sure your notes don’t show because you plugged your display connector in 😩 But If the conference has no wifi then we’re screwed I guess

- Location Palm Bay, FL
- Education FullSail University
- Work Developer Relations Manager at MetaMask
- Joined Sep 16, 2018
I like Reveal, but I still have not moved past using Google docs slides because every presentation I do has to be done yesterday. Hoping that I can use Reveal more often this year as I get more time to work on each presentation.
- Email [email protected]
- Location Abuja Nigeria
- Work Project Manager Techibytes Media
- Joined Feb 19, 2019
Well this is nice and I haven't tried it maybe because I haven't spoken much in meet ups but I think PowerPoint is still much better than going all these steps and what if I have network connection issues that day then I'm scrolled right?

- Email [email protected]
- Joined Apr 16, 2018
Using Node and Soket.io remote control (meant to be used on phones) for my school's computer science club, it also features some more goodies which are helpful when having multiple presentations. It can be modded to use these styling techniques effortlessly. Feel free to fork!
SBCompSciClub / prez-software
A synchronized role based presentation software using node, prez-software.
TODO: Make system to easily manage multiple presentations Add Hash endocing and decoding for "sudo" key values TODO: Document Code
Run on Dev Server
npm i nodemon app.js Nodemon? - A life saving NPM module that is ran on a system level which automatically runs "node (file.js)" when files are modified. Download nodemon by running npm i -g nodemon
Making a Presentation
- Copy an existing presentation folder
- Change the folder name (which should be located at public/slides) with the name day[num of day] ex(day2)
Making a Slide
Making a slide is pretty simple. Just add a HTML section. <section> <!--slide content--> </section> inside the span with the class of "prez-root". Also keep in mind that you will need to copy and pate the markup inside the prez root to the other pages (viewer & controller).
Adding Text
You may add text however you desire, but for titles use the…
Awesome post! I’m glad I’m not the only one who likes libraries. 😎

- Location Los Angeles
- Education Engineering, Physics, and Math
- Joined Sep 6, 2018
Fantastic post. I just loved it.

- Location France
- Work Co-Founder of Depot
- Joined Sep 2, 2017
Awesome introduction! I feel like I need to give this a try the next time I create a presentation.
Some comments may only be visible to logged-in visitors. Sign in to view all comments.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
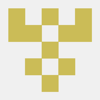
Introduction to Programming: A Beginner's Journey into the World of Code
Captain Iminza - Aug 23

Why I Like Golang and Why It's the Best Language for Me.
Ali - Sep 1
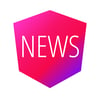
Ng-News 24/36: Incremental Hydration, Largest Angular App & more
ng-news - Sep 10

Mastering Event-Driven Programming with the EventEmitter in Node.js
Sushant Gaurav - Sep 10
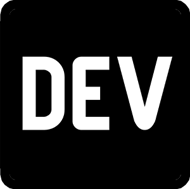
We're a place where coders share, stay up-to-date and grow their careers.
- Powerpoint Tutorials
How to Combine Multiple PowerPoints into One: A Complete Guide
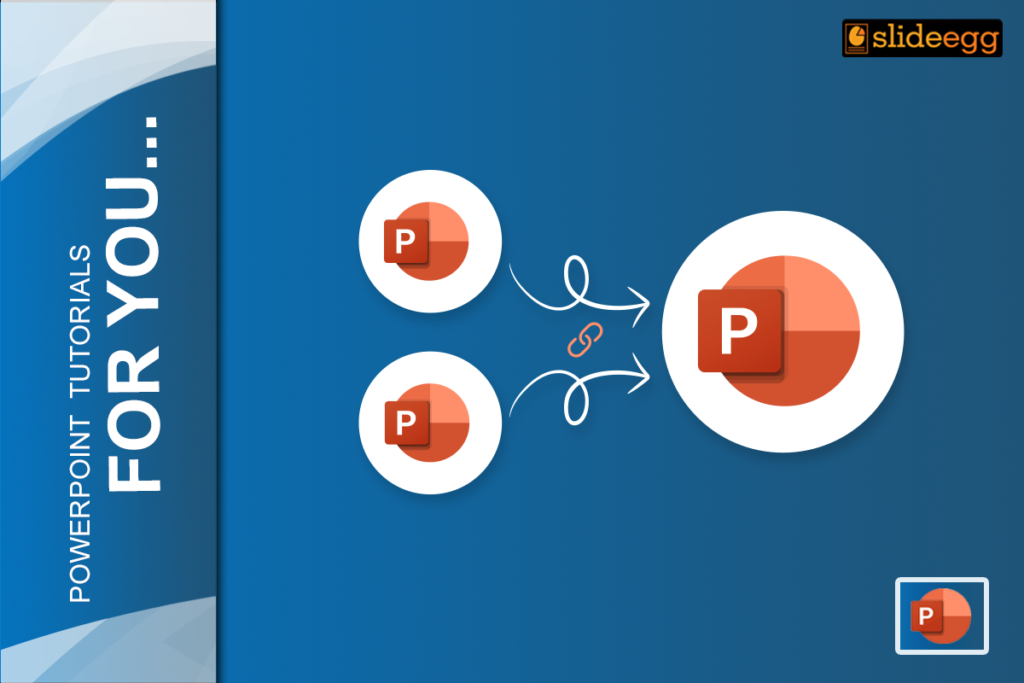
Combining multiple PowerPoint presentations into a single file can be a daunting task, especially when dealing with numerous slides and different formatting styles. But with the right approach, you can streamline this process and create a cohesive presentation effortlessly. In this comprehensive guide, we’ll walk you through several methods to merge your PowerPoints, from basic copy-paste to advanced techniques.
Method 1: The Classic Copy and Paste
The most simple and easy way to combine PowerPoints is by using the good old copy and paste method. Here’s how:
- Open both presentations: Start by opening the main PowerPoint where you want to incorporate the slides from another presentation.
- Select and copy slides: In the presentation you want to merge, select the slides you need. Right-click and choose “Copy” or use the keyboard shortcut Ctrl+C.
- Paste into the main presentation: Go back to your main PowerPoint, click where you want to insert the copied slides, right-click, and select “Paste.”
Pro Tip: To maintain consistent formatting, try using the “Paste Special” option and choose “Keep Source Formatting.”
Method 2: Reuse Slides Feature
PowerPoint offers a built-in feature called “Reuse Slides” that simplifies the merging process. Follow these steps:
- Open the main presentation: Begin with the PowerPoint where you want to merge the slides.
- Access Reuse Slides: Click on the “Home” tab, then “New Slide,” and choose “Reuse Slides.”
- Select the presentation: Browse and select the PowerPoint file containing the slides you want to add.
- Insert slides: Choose the specific slides or insert all slides at once.
Method 3: Insert Object
For more complex scenarios, the “Insert Object” method can be helpful. Here’s how it works:
- Open the main presentation: Open the PowerPoint presentation where you’ll combine the slides.
- Insert Object: Go to the “Insert” tab, click on “Object,” and choose “Create from File.”
- Select the presentation: Browse and select the PowerPoint file you want to embed.
- Choose options: Decide whether to link the object to the original file or create a copy.
Method 4: Drag and Drop (For Similar File Locations)
If your PowerPoints are stored in the same folder, you can simplify the process by dragging and dropping slides.
- Open both presentations: Have both PowerPoints open side by side.
- Select and drag: In the source presentation, select the slides you want to move. Drag and drop them into the desired location in the main presentation.
Method 5: Compare and Merge (Advanced)
PowerPoint’s “Compare” feature offers a more advanced way to combine presentations while highlighting differences. This method is particularly useful when merging multiple versions of the same presentation.
- Open the main presentation: Start with the PowerPoint you want to use as a base.
- Access Compare: Go to the “Review” tab and click on “Compare.”
- Select presentations: Choose the PowerPoint files you want to compare and merge.
- Review and merge: PowerPoint will display the differences between the presentations, allowing you to select the slides you want to keep.
Tips for a Seamless Merge
- Maintain consistency: Ensure a uniform look and feel by applying the same theme and formatting styles to all slides.
- Check for duplicates: Avoid redundant information by reviewing the content before merging.
- Proofread carefully: After combining the presentations, thoroughly proofread for errors and inconsistencies.
By following these methods and tips, you can effectively combine multiple PowerPoints into a single, well-organized presentation. Choose the approach that best suits your needs and enjoy the benefits of a streamlined workflow.
Visit our tips & tricks page to discover additional presentation hacks that can elevate your skills. If you are working on the Google Slides platform, we hope our blog on “ How to Connect Multiple Google Slides into One ” will help you achieve this.
By mastering the art of combining PowerPoints, you’ll save time, improve efficiency, and create impactful presentations that leave a lasting impression on your audience.
Spread Love
Related blogs.
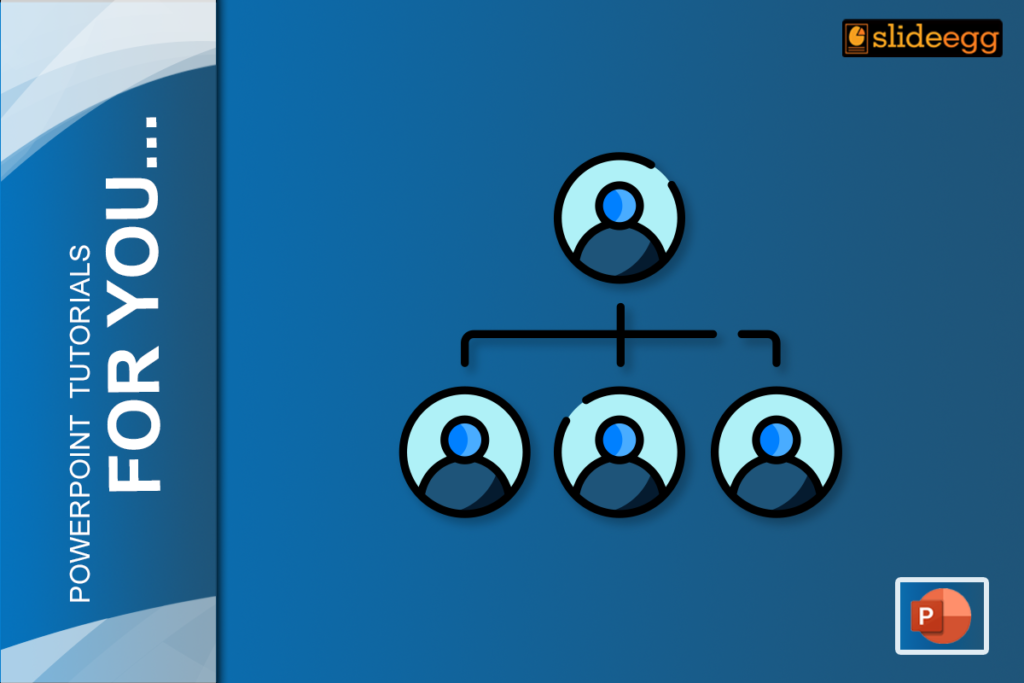
Arockia Mary Amutha
Arockia Mary Amutha is a seasoned senior content writer at SlideEgg, bringing over four years of dedicated experience to the field. Her expertise in presentation tools like PowerPoint, Google Slides, and Canva shines through in her clear, concise, and professional writing style. With a passion for crafting engaging and insightful content, she specializes in creating detailed how-to guides, tutorials, and tips on presentation design that resonate with and empower readers.
Recent Blogs
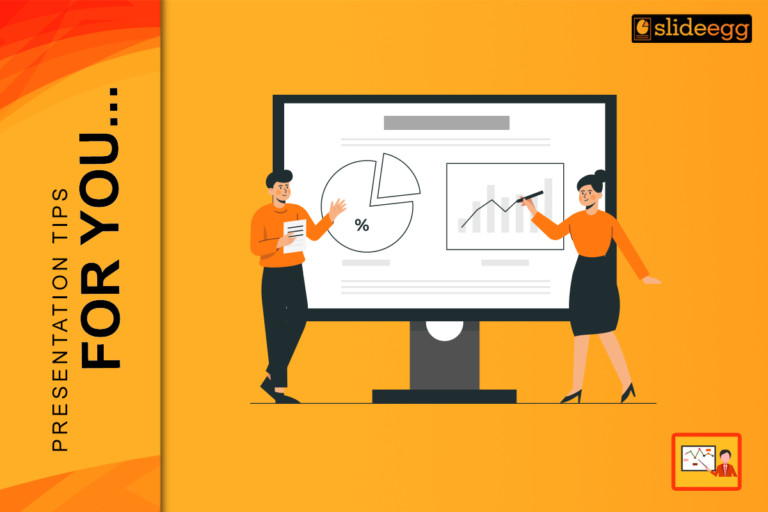
What is a PPT and How to Make Impactful Presentations with PowerPoint?
PowerPoint Presentations, commonly known as PPTs, have become an essential tool in various fields such as business, education, and even...
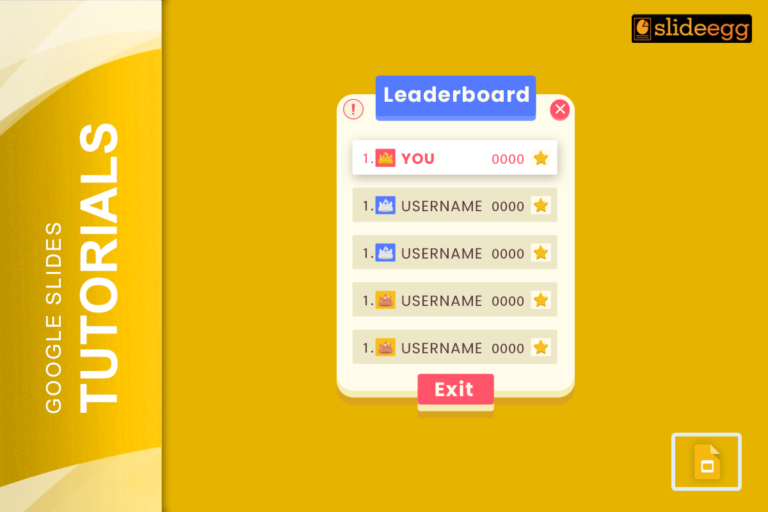
How to Create a Jeopardy Game in Google Slides: A Step-by-Step Guide
Jeopardy is a popular quiz game that challenges participants with answers to which they must respond with the correct questions....
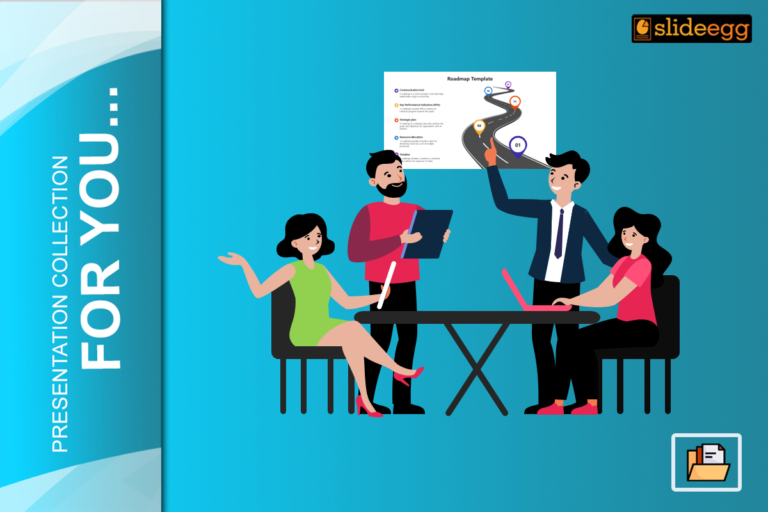
Why Roadmap PowerPoint Template Best For Project Management?
Project management, at this time, will be like walking in a maze, where one becomes lost in the middle of...
SIGNUP FOR NEWSLETTER
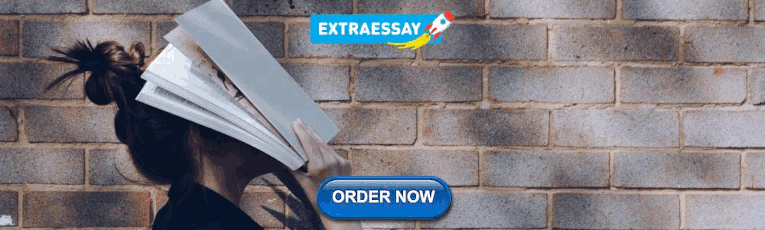
IMAGES
VIDEO
COMMENTS
HTML is used as the graphical user interface in client-side programs written in JavaScript. Server-side languages like PHP and Java also receive data from web pages and use HTML as the output mechanism. The emerging Ajax technologies likewise use HTML and XHTML as their visual engine.
Free Google Slides theme, PowerPoint template, and Canva presentation template. Unleash the power of web design in your classroom with our Geometric Abstract PPT template, ideal for teachers introducing HTML. Dominated by a cool blue hue, this PowerPoint and Google Slides template incorporates a modern, geometric style that will engage your ...
Presentation on theme: "Introduction to HTML- Basics"— Presentation transcript: Objectives In this chapter, you will learn: To understand important components of HTML documents. To use HTML to create Web pages. To be able to add images to Web pages. To understand how to create and use hyperlinks to navigate Web pages.
In the function moveToLeftSlide, we basically access the previous sibling element (i.e. the previous slide), remove the .show class on the current slide, and add it to that sibling. This will move the presentation to the previous slide. We do the exact opposite of this in the function moveToRightSlide.Because nextElementSibling is the opposite of previousElementSibling, we'll be getting the ...
5 How can I test my code Just open the index.html from the template in your text editor and in your browser. When you do any change to the code, check it in the browser by pressing F5 (refresh site) To open the developer tools press: Windows: Control + Shift + I or OSX: Command + Opt + I Other tools are online editors like scratchpad or htmledit
Download presentation. Presentation on theme: "Basics of HTML."—. Presentation transcript: 1 Basics of HTML. 2 Lesson 1 Building Your First Web Page. Before we begin our journey to learn how to build websites with HTML and CSS, it is important to understand the differences between the two languages, the syntax of each language, and some ...
HTML 5 Course. HTML Training (12 Slides) 5059 Views. Unlock a Vast Repository of HTML Training PPT Slides, Meticulously Curated by Our Expert Tutors and Institutes. Download Free and Enhance Your Learning!
Times New Roman Arial Wingdings Garamond Capsules Adobe Photoshop Image HTML (Hypertext MarkUP Language) HTML (Hypertext Markup Language) HTML (Hypertext Markup Language) HTML (Hypertext Markup Language) HTML (Hypertext Markup Language) CSS (Cascading Style Sheet) CSS (Cascading Style Sheet) CSS (Cascading Style Sheet) CSS (Cascading Style ...
Description: HTML stands for Hyper Text Markup Language, a language with set of markup tags to describe web pages. An HTML file must have an .htm or .html file extension. HTML is comprised of "elements" and "tags" that begins with and ends with . - PowerPoint PPT presentation. Number of Views: 7349. Slides: 34. Provided by ...
Presentation on theme: "Introduction to HTML and CSS"— Presentation transcript: 1 Introduction to HTML and CSS. 2 The Web & HTML: Historical Context. Review: HTML consists of lines of text with embedded markup tags that specify Web-page formatting and links to other pages Invented by Tim Berners-Lee at CERN in 1989 In 1993, students, faculty ...
Description: HTML5 Tutorial For Beginners - Learning HTML 5 in simple and easy steps with examples covering 2D Canvas, Audio, Video, New Semantic Elements, Geolocation, Persistent Local Storage, Web Storage, Forms Elements,Application Cache,Inline SVG,Document - PowerPoint PPT presentation. Number of Views: 26196.
WebSlides is the easiest way to make HTML presentations. Just choose a demo and customize it in minutes. 120+ slides ready to use. ... HTML and CSS as narrative elements. Work better, faster. Designers, marketers, and journalists can now focus on the content. ... here's just some tutorials. Just a basic knowledge of HTML is required: Components ...
HTML Basics PPT. HTML (abbreviated as HyperText Markup Language) is a computer language used to develop web pages with the help of various tags and attributes. The major advantage is that it is easy to learn and code even for a newbie. Almost every web browsers and websites use HTML for creating web contents. So, it is necessary for everyone to ...
Getting Started with WebSlides. To get started, first download WebSlides. Then, in the root folder, create a new folder and call it presentation. Inside the newly created presentation folder ...
In this tutorial we provide all the details of all 40 above HTML tags with examples. HTML5 is just a new version of HTML and with it you get some new features which make it easier. these features are layout clearer, header, footer and all. so just visit here to get full tutorial now. - A free PowerPoint PPT presentation (displayed as an HTML5 slide show) on PowerShow.com - id: 8ed816-ZjkwZ
Create the Starter Markup. 3. Make It Pretty. 4. Enable Slide Navigation. Moving the Presentation to the Next and Previous Slides. Code for Showing the Presentation in Full Screen and Small Screen. Hidding Left and Right Icons in First and Last Slides. Updating and Displaying Slide Number.
Making a Presentation. Copy an existing presentation folder; Change the folder name (which should be located at public/slides) with the name day[num of day] ex(day2) Making a Slide. Making a slide is pretty simple. Just add a HTML section. <section> <!--slide content--> </section> inside the span with the class of "prez-root". Also keep in mind ...
Select and copy slides: In the presentation you want to merge, select the slides you need. Right-click and choose "Copy" or use the keyboard shortcut Ctrl+C. Paste into the main presentation: Go back to your main PowerPoint, click where you want to insert the copied slides, right-click, and select "Paste."