- Science, Tech, Math ›
- Computer Science ›
- Java Programming ›
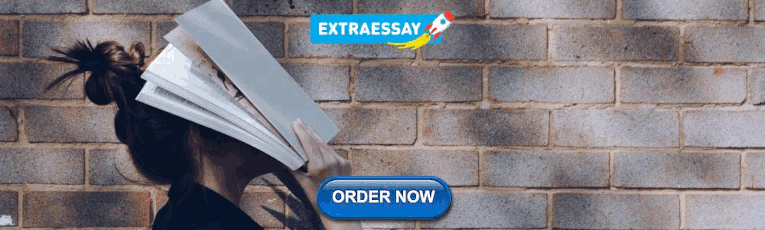
Compound-Assignment Operators
- Java Programming
- PHP Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- M.A., Advanced Information Systems, University of Glasgow
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound-Assignment Operators in Java
Java supports 11 compound-assignment operators:
Example Usage
To assign the result of an addition operation to a variable using the standard syntax:
But use a compound-assignment operator to effect the same outcome with the simpler syntax:
- Java Expressions Introduced
- Understanding the Concatenation of Strings in Java
- Conditional Operators
- Conditional Statements in Java
- Working With Arrays in Java
- Declaring Variables in Java
- If-Then and If-Then-Else Conditional Statements in Java
- How to Use a Constant in Java
- Common Java Runtime Errors
- What Is Java?
- What Is Java Overloading?
- Definition of a Java Method Signature
- What a Java Package Is In Programming
- Java: Inheritance, Superclass, and Subclass
- Understanding Java's Cannot Find Symbol Error Message
- Using Java Naming Conventions

- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
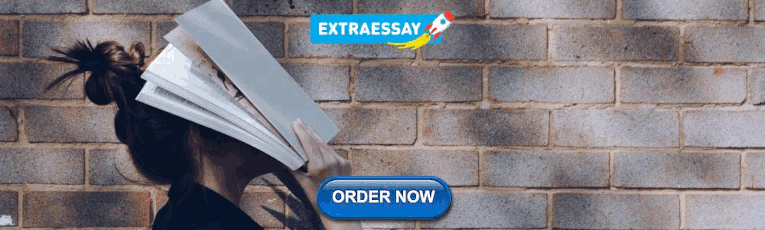
Compound Assignment Operators in C++
The compound assignment operators are specified in the form e1 op= e2, where e1 is a modifiable l-value not of const type and e2 is one of the following −
- An arithmetic type
- A pointer, if op is + or –
The e1 op= e2 form behaves as e1 = e1 op e2, but e1 is evaluated only once.
The following are the compound assignment operators in C++ −
Let's have a look at an example using some of these operators −
This will give the output −
Note that Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type or it must be a constant expression that evaluates to 0. If the left operand is of an integral type, the right operand must not be of a pointer type.

- Related Articles
- Compound assignment operators in C#
- Compound assignment operators in Java\n
- Perl Assignment Operators
- Assignment operators in Dart Programming
- Compound operators in Arduino
- What is the difference between = and: = assignment operators?
- Passing the Assignment in C++
- Airplane Seat Assignment Probability in C++
- Copy constructor vs assignment operator in C++
- Unary operators in C/C++
- Ternary Operators in C/C++
- # and ## Operators in C ?
- Operators Precedence in C++
- Unary operators in C++
- Conversion Operators in C++
Kickstart Your Career
Get certified by completing the course
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Compound Assignment
- 6 contributors
The compound-assignment operators combine the simple-assignment operator with another binary operator. Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment expression such as
expression1 += expression2
can be understood as
expression1 = expression1 + expression2
However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation.
The operands of a compound-assignment operator must be of integral or floating type. Each compound-assignment operator performs the conversions that the corresponding binary operator performs and restricts the types of its operands accordingly. The addition-assignment ( += ) and subtraction-assignment ( -= ) operators can also have a left operand of pointer type, in which case the right-hand operand must be of integral type. The result of a compound-assignment operation has the value and type of the left operand.
In this example, a bitwise-inclusive-AND operation is performed on n and MASK , and the result is assigned to n . The manifest constant MASK is defined with a #define preprocessor directive.
C Assignment Operators
Was this page helpful?
Additional resources
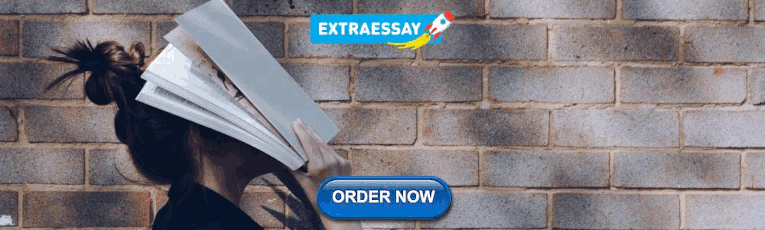
IMAGES
VIDEO
COMMENTS
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
The basic arithmetic compound assignment operators include += (addition and assignment), -= (subtraction and assignment), *= (multiplication and assignment), /= (division and assignment), …
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These …
The following are the compound assignment operators in C++ −. Example. Let's have a look at an example using some of these operators −. #include<iostream> using …
Built-in compound assignment operator. The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the …
Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment …
Compound Assignment Operators are a shorter way to apply an arithmetic or bitwise operation and to assign the value of the operation to the variable on the left-hand side. For example, the following two multiplication …
Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator. Let’s look at each of the assignment operators …