Lecture 7/15: Object-Oriented Programming
July 15, 2020
📂Associated files
- Lecture15.zip
- Lecture15_Slides.pdf
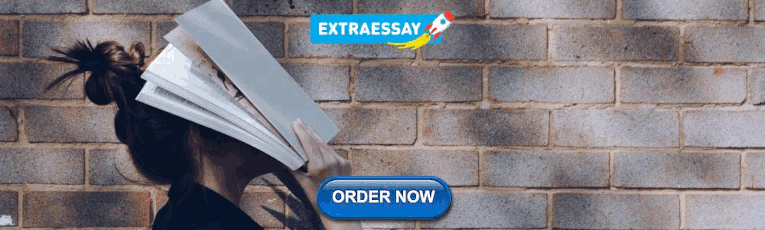
Lecture 15: Object-Oriented Programming
Cs 106b: programming abstractions, summer 2020, stanford university computer science department, lecturers: nick bowman and kylie jue.

For every lecture, we will post the lecture slides and any example code that will be used during lecture, usually in advance of the beginning of the lecture. For today's lecture, you can find the slides below:
- Lecture Slides
- Lecture Code
After the conclusion of each lecture, we will upload the lecture recording to the "Cloud Recordings" tab of the "Zoom" section of Canvas. The lecture recordings will also be linked here for your convenience.
- Lecture Recording
© Stanford 2020 · Website by Julie Zelenski · Page updated 2020-Jul-15
What is object-oriented programming? OOP explained in depth
Object-oriented programming (OOP) is a fundamental programming paradigm used by nearly every developer at some point in their career. OOP is the most popular programming paradigm used for software development and is taught as the standard way to code for most of a programmer’s educational career. Another popular programming paradigm is functional programming, but we won’t get into that right now.
Today we will break down the basics of what makes a program object-oriented so that you can start to utilize this paradigm in your algorithms, projects, and interviews.
Now, let’s dive into these OOP concepts and tutorials!
Here’s what will be covered:
What is Object-Oriented Programming?
Building blocks of oop.
- Four principles of OOP
- What to learn next
Object-Oriented Programming (OOP) is a programming paradigm in computer science that relies on the concept of classes and objects . It is used to structure a software program into simple, reusable pieces of code blueprints (usually called classes), which are used to create individual instances of objects. There are many object-oriented programming languages, including JavaScript, C++ , Java , and Python .
OOP languages are not necessarily restricted to the object-oriented programming paradigm. Some languages, such as JavaScript, Python, and PHP, all allow for both procedural and object-oriented programming styles.
A class is an abstract blueprint that creates more specific, concrete objects. Classes often represent broad categories, like Car or Dog that share attributes . These classes define what attributes an instance of this type will have, like color , but not the value of those attributes for a specific object.
Classes can also contain functions called methods that are available only to objects of that type. These functions are defined within the class and perform some action helpful to that specific object type.
For example, our Car class may have a repaint method that changes the color attribute of our car. This function is only helpful to objects of type Car , so we declare it within the Car class, thus making it a method.
Class templates are used as a blueprint to create individual objects . These represent specific examples of the abstract class, like myCar or goldenRetriever . Each object can have unique values to the properties defined in the class.
For example, say we created a class, Car , to contain all the properties a car must have, color , brand , and model . We then create an instance of a Car type object, myCar to represent my specific car. We could then set the value of the properties defined in the class to describe my car without affecting other objects or the class template. We can then reuse this class to represent any number of cars.
Benefits of OOP for software engineering
- OOP models complex things as reproducible, simple structures
- Reusable, OOP objects can be used across programs
- Polymorphism allows for class-specific behavior
- Easier to debug, classes often contain all applicable information to them
- Securely protects sensitive information through encapsulation
How to structure OOP programs
Let’s take a real-world problem and conceptually design an OOP software program.
Imagine running a dog-sitting camp with hundreds of pets where you keep track of the names, ages, and days attended for each pet.
How would you design simple, reusable software to model the dogs?
With hundreds of dogs, it would be inefficient to write unique entries for each dog because you would be writing a lot of redundant code. Below we see what that might look like with objects rufus and fluffy .
As you can see above, there is a lot of duplicated code between both objects. The age() function appears in each object. Since we want the same information for each dog, we can use objects and classes instead.
Grouping related information together to form a class structure makes the code shorter and easier to maintain.
In the dogsitting example, here’s how a programmer could think about organizing an OOP:
- Create a class for all dogs as a blueprint of information and behaviors (methods) that all dogs will have, regardless of type. This is also known as the parent class .
- Create subclasses to represent different subcategories of dogs under the main blueprint. These are also referred to as child classes .
- Add unique attributes and behaviors to the child classes to represent differences
- Create objects from the child class that represent dogs within that subgroup
The diagram below represents how to design an OOP program by grouping the related data and behaviors together to form a simple template and then creating subgroups for specialized data and behavior.
The Dog class is a generic template containing only the structure of data and behaviors common to all dogs as attributes.
We then create two child classes of Dog , HerdingDog and TrackingDog . These have the inherited behaviors of Dog ( bark() ) but also behavior unique to dogs of that subtype.
Finally, we create objects of the HerdingDog type to represent the individual dogs Fluffy and Maisel .
We can also create objects like Rufus that fit under the broad class of Dog but do not fit under either HerdingDog or TrackingDog .
Next, we’ll take a deeper look at each of the fundamental building blocks of an OOP program used above:
In a nutshell, classes are essentially user-defined data types . Classes are where we create a blueprint for the structure of methods and attributes. Individual objects are instantiated from this blueprint.
Classes contain fields for attributes and methods for behaviors. In our Dog class example, attributes include name & birthday , while methods include bark() and updateAttendance() .
Here’s a code snippet demonstrating how to program a Dog class using the JavaScript language.
Remember, the class is a template for modeling a dog, and an object is instantiated from the class representing an individual real-world item.
Enjoying the article? Scroll down to sign up for our free, bi-monthly newsletter.
Objects are, unsurprisingly, a huge part of OOP! Objects are instances of a class created with specific data. For example, in the code snippet below, Rufus is an instance of the Dog class.
When the new class Dog is called:
- A new object is created named rufus
- The constructor runs name & birthday arguments, and assigns values
Programming vocabulary: In JavaScript, objects are a type of variable. This may cause confusion because objects can be declared without a class template in JavaScript, as shown at the beginning. Objects have states and behaviors. The state of an object is defined by data: things like names, birthdates, and other information you’d want to store about a dog. Behaviors are methods the object can undertake.
N/A | What is it? | Information Contained | Actions | Example |
---|---|---|---|---|
Classes | Blueprint | Attributes | Behaviors defined through methods | Dog Template |
Objects | Instance | State, Data | Methods | Rufus, Fluffy |
Attributes are the information that is stored. Attributes are defined in the Class template. When objects are instantiated, individual objects contain data stored in the Attributes field.
The state of an object is defined by the data in the object’s attributes fields. For example, a puppy and a dog might be treated differently at a pet camp. The birthday could define the state of an object and allow the software to handle dogs of different ages differently.
Methods represent behaviors. Methods perform actions; methods might return information about an object or update an object’s data. The method’s code is defined in the class definition.
When individual objects are instantiated, these objects can call the methods defined in the class. In the code snippet below, the bark method is defined in the Dog class, and the bark() method is called on the Rufus object.
Methods often modify, update or delete data. Methods don’t have to update data though. For example, the bark() method doesn’t update any data because barking doesn’t modify any of the attributes of the Dog class: name or birthday .
The updateAttendance() method adds a day the Dog attended the pet-sitting camp. The attendance attribute is important to keep track of for billing Owners at the end of the month.
Methods are how programmers promote reusability and keep functionality encapsulated inside an object. This reusability is a great benefit when debugging. If there’s an error, there’s only one place to find it and fix it instead of many.
The underscore in _attendance denotes that the variable is protected and shouldn’t be modified directly. The updateAttendance() method changes _attendance .
Four Principles of OOP
The four pillars of object-oriented programming are:
- Inheritance: child classes inherit data and behaviors from the parent class
- Encapsulation: containing information in an object, exposing only selected information
- Abstraction: only exposing high-level public methods for accessing an object
- Polymorphism: many methods can do the same task
Inheritance
Inheritance allows classes to inherit features of other classes. Put another way, parent classes extend attributes and behaviors to child classes. Inheritance supports reusability .
If basic attributes and behaviors are defined in a parent class, child classes can be created, extending the functionality of the parent class and adding additional attributes and behaviors.
For example, herding dogs have the unique ability to herd animals. In other words, all herding dogs are dogs, but not all dogs are herding dogs. We represent this difference by creating a child class HerdingDog from the parent class Dog , and then adding the unique herd() behavior.
The benefits of inheritance are programs can create a generic parent class and then create more specific child classes as needed. This simplifies programming because instead of recreating the structure of the Dog class multiple times, child classes automatically gain access to functionalities within their parent class.
In the following code snippet, child class HerdingDog inherits the method bark from the parent class Dog , and the child class adds an additional method, herd() .
Notice that the HerdingDog class does not have a copy of the bark() method. It inherits the bark() method defined in the parent Dog class.
When the code calls fluffy.bark() method, the bark() method walks up the chain of child to parent classes to find where the bark method is defined.
Note: Parent classes are also known as superclasses or base classes. The child class can also be called a subclass, derived class, or extended class.
In JavaScript, inheritance is also known as prototyping . A prototype object is a template for another object to inherit properties and behaviors. There can be multiple prototype object templates, creating a prototype chain.
This is the same concept as the parent/child inheritance. Inheritance is from parent to child. In our example, all three dogs can bark, but only Maisel and Fluffy can herd.
The herd() method is defined in the child HerdingDog class, so the two objects, Maisel and Fluffy , instantiated from the HerdingDog class have access to the herd() method.
Rufus is an object instantiated from the parent class Dog , so Rufus only has access to the bark() method.
Object | Instantiated from Class | Parent Class | Methods |
---|---|---|---|
Rufus | Dog | N/A | bark() |
Maisel | Herding Dog | Dog | bark(), herd() |
Fluffy | Herding Dog | Dog | bark(), herd() |
Encapsulation
Encapsulation means containing all important information inside an object , and only exposing selected information to the outside world. Attributes and behaviors are defined by code inside the class template.
Then, when an object is instantiated from the class, the data and methods are encapsulated in that object. Encapsulation hides the internal software code implementation inside a class and hides the internal data of inside objects.
Encapsulation requires defining some fields as private and some as public.
- Private/ Internal interface: methods and properties accessible from other methods of the same class.
- Public / External Interface: methods and properties accessible from outside the class.
Let’s use a car as a metaphor for encapsulation. The information the car shares with the outside world, using blinkers to indicate turns, are public interfaces. In contrast, the engine is hidden under the hood.
It’s a private, internal interface. When you’re driving a car down the road, other drivers require information to make decisions, like whether you’re turning left or right. However, exposing internal, private data like the engine temperature would confuse other drivers.
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
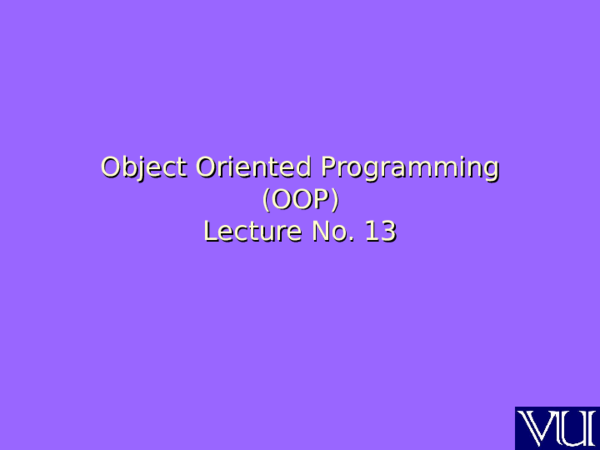
Object Oriented Programming (OOP) - CS304 Power Point Slides Lecture

Related Papers

Mohamed Ahmed
Krishnaprasad Thirunarayan
Riazali Solangi
IRJET Journal
For the growth of software industry in future and the advance of software engineering, use of object-oriented programming (OOP) has increased in the software real world. Some the important features that's know is compulsory and that's features are important to study the depth knowledge of object-oriented programming in this paper, we study the concept of object-oriented programming and its features, advantages, disadvantages, and we also know the constructor and destructors
Collins Korir
Mayank AGARWAL , Asif Hameed
Chintan Varnagar
RELATED PAPERS
asif hameed
Armando Serna Gutiérrez
Nikoleta Razkova
International Journal of Engineering Pedagogy (iJEP)
Merike Saar
XÐIEGO ORTEGA
Paolo scientifico
ctp.di.fct.unl.pt
Fernando Brito e Abreu
Lecture Notes in Computer Science
miguel angel perez
Object Oriented Programming in C++ (BORLAND C++ BUILDER)
Martin Lesage
Geraldine Sajor
ACM SIGCSE Bulletin
Wilf Lalonde
Jürgen Börstler
david daniel
Computing science notes
Pierre America
IJSRD - International Journal for Scientific Research and Development
SHAILESH PANDEY
Proceedings of the ACM Conference on Object-oriented Programming, Systems and Languages, OOPSLA'98, Vancouver, October 1998, ACM ISBN 1-58113-005
Simon Holland
FIE '98. 28th Annual Frontiers in Education Conference. Moving from 'Teacher-Centered' to 'Learner-Centered' Education. Conference Proceedings (Cat. No.98CH36214)
James D Kiper
Hervé Paulino
Mirko Viroli
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Introduction of Object Oriented Programming
As the name suggests, Object-Oriented Programming or OOPs refers to languages that use objects in programming. Object-oriented programming aims to implement real-world entities like inheritance, hiding, polymorphism, etc in programming. The main aim of OOP is to bind together the data and the functions that operate on them so that no other part of the code can access this data except that function.
OOPs Concepts:
- Data Abstraction
- Encapsulation
- Inheritance
- Polymorphism
- Dynamic Binding
- Message Passing
A class is a user-defined data type. It consists of data members and member functions, which can be accessed and used by creating an instance of that class. It represents the set of properties or methods that are common to all objects of one type. A class is like a blueprint for an object.
For Example: Consider the Class of Cars. There may be many cars with different names and brands but all of them will share some common properties like all of them will have 4 wheels, Speed Limit, Mileage range, etc. So here, Car is the class, and wheels, speed limits, mileage are their properties.
2. Object:
It is a basic unit of Object-Oriented Programming and represents the real-life entities. An Object is an instance of a Class. When a class is defined, no memory is allocated but when it is instantiated (i.e. an object is created) memory is allocated. An object has an identity, state, and behavior. Each object contains data and code to manipulate the data. Objects can interact without having to know details of each other’s data or code, it is sufficient to know the type of message accepted and type of response returned by the objects.
For example “Dog” is a real-life Object, which has some characteristics like color, Breed, Bark, Sleep, and Eats.
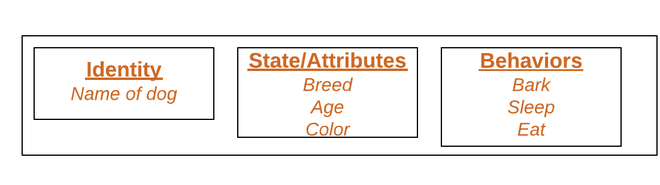
3. Data Abstraction:
Data abstraction is one of the most essential and important features of object-oriented programming. Data abstraction refers to providing only essential information about the data to the outside world, hiding the background details or implementation. Consider a real-life example of a man driving a car. The man only knows that pressing the accelerators will increase the speed of the car or applying brakes will stop the car, but he does not know about how on pressing the accelerator the speed is increasing, he does not know about the inner mechanism of the car or the implementation of the accelerator, brakes, etc in the car. This is what abstraction is.
4. Encapsulation:
Encapsulation is defined as the wrapping up of data under a single unit. It is the mechanism that binds together code and the data it manipulates. In Encapsulation, the variables or data of a class are hidden from any other class and can be accessed only through any member function of their class in which they are declared. As in encapsulation, the data in a class is hidden from other classes, so it is also known as data-hiding .
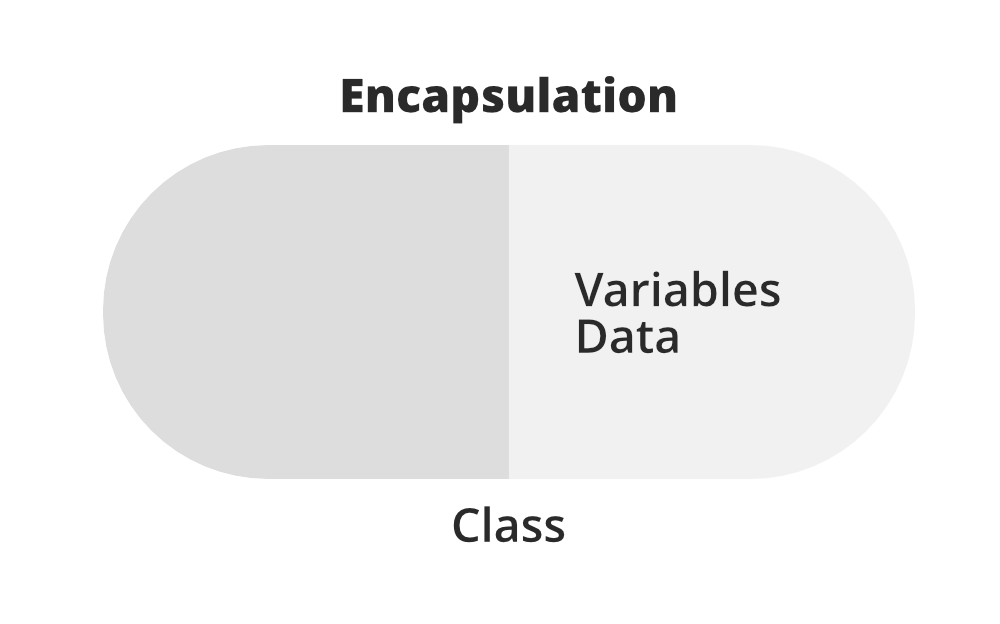
Consider a real-life example of encapsulation, in a company, there are different sections like the accounts section, finance section, sales section, etc. The finance section handles all the financial transactions and keeps records of all the data related to finance. Similarly, the sales section handles all the sales-related activities and keeps records of all the sales. Now there may arise a situation when for some reason an official from the finance section needs all the data about sales in a particular month. In this case, he is not allowed to directly access the data of the sales section. He will first have to contact some other officer in the sales section and then request him to give the particular data. This is what encapsulation is. Here the data of the sales section and the employees that can manipulate them are wrapped under a single name “sales section”.
5. Inheritance:
Inheritance is an important pillar of OOP(Object-Oriented Programming). The capability of a class to derive properties and characteristics from another class is called Inheritance. When we write a class, we inherit properties from other classes. So when we create a class, we do not need to write all the properties and functions again and again, as these can be inherited from another class that possesses it. Inheritance allows the user to reuse the code whenever possible and reduce its redundancy.
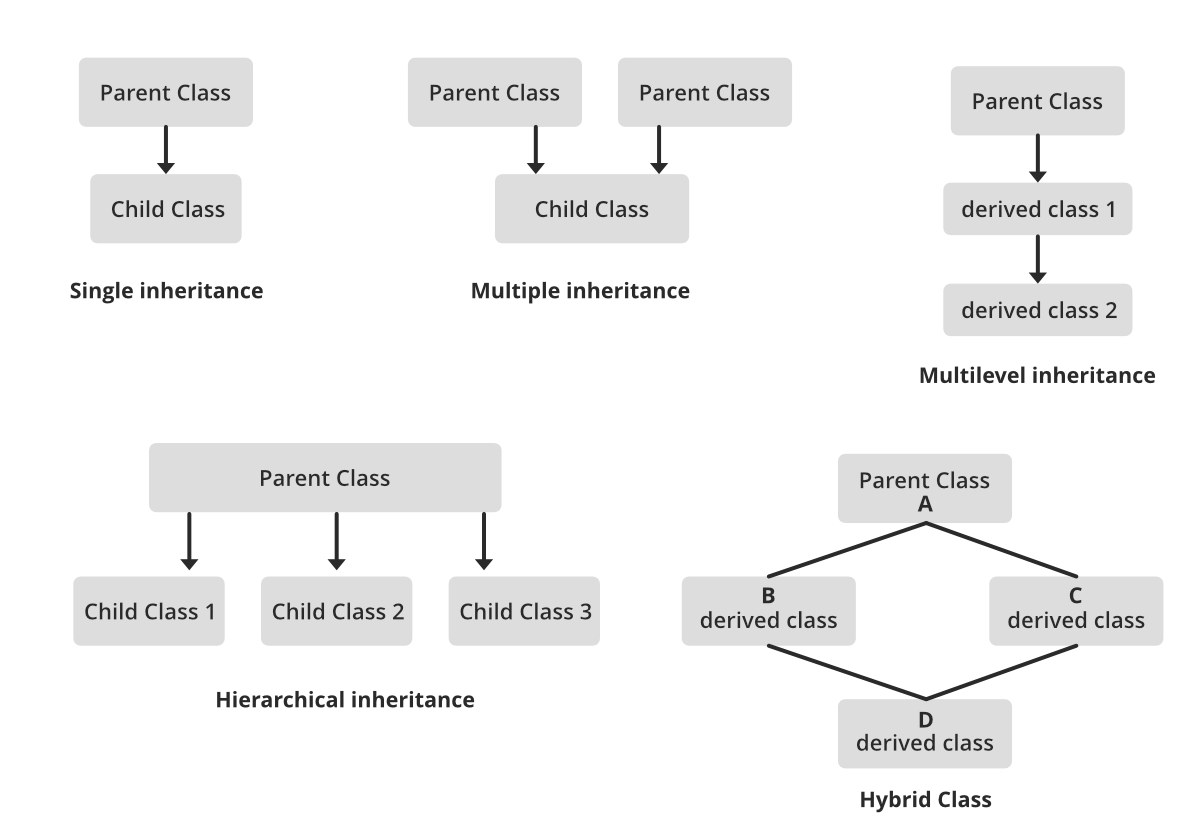
6. Polymorphism:
The word polymorphism means having many forms. In simple words, we can define polymorphism as the ability of a message to be displayed in more than one form. For example, A person at the same time can have different characteristics. Like a man at the same time is a father, a husband, an employee. So the same person posses different behavior in different situations. This is called polymorphism.
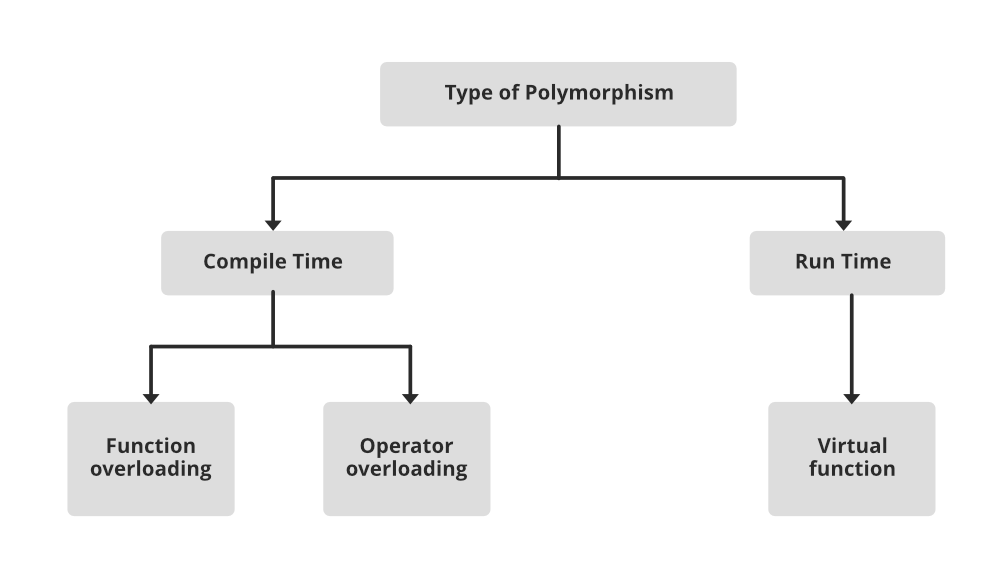
7. Dynamic Binding:
In dynamic binding, the code to be executed in response to the function call is decided at runtime. Dynamic binding means that the code associated with a given procedure call is not known until the time of the call at run time. Dynamic Method Binding One of the main advantages of inheritance is that some derived class D has all the members of its base class B. Once D is not hiding any of the public members of B, then an object of D can represent B in any context where a B could be used. This feature is known as subtype polymorphism.
8. Message Passing:
It is a form of communication used in object-oriented programming as well as parallel programming. Objects communicate with one another by sending and receiving information to each other. A message for an object is a request for execution of a procedure and therefore will invoke a function in the receiving object that generates the desired results. Message passing involves specifying the name of the object, the name of the function, and the information to be sent.
Why do we need object-oriented programming
- To make the development and maintenance of projects more effortless.
- To provide the feature of data hiding that is good for security concerns.
- We can solve real-world problems if we are using object-oriented programming.
- It ensures code reusability.
- It lets us write generic code: which will work with a range of data, so we don’t have to write basic stuff over and over again.
Please Login to comment...
Similar reads.
- Programming Language
- School Programming
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
OOP Meaning – What is Object-Oriented Programming?

In today's technology driven society, computer programming knowledge is in high demand. And as a developer, you'll need to know various programming languages.
Over the past few decades, many programming languages have risen in popularity. You can see how popular languages are ranked in this real time ranking chart here .
While new languages are being created, existing ones are always being updated to make them better.
Although most programming languages have some similarities, each one has specific rules and methods which makes it unique.
One concept that is common among many programming languages is Object Oriented Programming .
When I first came across this term, it was a bit confusing. It took me some time to really understand it's importance in programming. But this also doubled as an opportunity for me to learn its key concepts, and know how important it is for a developer's career and being able to solve challenges.
In this article we will go over Object Oriented Programming (OOP) as a whole, without relying on a particular language. You'll learn what it is, why it's so popular as a programming paradigm, its structure, how it works, its principles, and more.
Let's get started.
What is Object-Oriented Programming?
If you were to conduct a fast internet search on what object-oriented programming is, you'll find that OOP is defined as a programming paradigm that relies on the concept of classes and objects.
Now, for a beginner, that might be a little bit confusing – but no need to worry. I will try to explain it in a simplest way possible, just like the famous phrase "Explain it to me like I'm 5".
Here's a brief overview of what you can achieve with OOP: you can use it to structure a software program into simple, reusable code blocks (in this case usually called classes), which you then use to create individual instances of the objects.
So let's find an easier definition of object-oriented programming and learn more about it.
Explain OOP Like I'm 5
The word object-oriented is a combination of two terms, object and oriented.
The dictionary meaning of an object is "an entity that exists in the real world", and oriented means "interested in a particular kind of thing or entity".
In basic terms, OOP is a programming pattern that is built around objects or entities, so it's called object-oriented programming.
To better understand the concept, let's have a look at commonly used software programs: A good example to explain this would be the use of a printer when you are printing a document.
The first step is initiating the action by clicking on the print command or using keyboard shortcuts. Next you need to select your printer. Afterwards you will wait for a response telling you if the document was printed or not.
Behind what we can't see, the command you clicked interacts with an object (printer) to accomplish the task of printing.
Perhaps you might wonder, how exactly did OOP become so popular?
How OOP Became Popular
The concepts of OOP started to surface back in the 60s with a programming language called Simula . Even though back in the day, developers didn't completely embrace the first advances in OOP languages, the methodologies continued to evolve.
Fast forward to the 80s, and an editorial written by David Robinson was one of the first introductions to OOP, as many developers didn't know it existed.
By now languages like C++ and Eiffel had become more popular and mainstream among computer programmers. The recognition continued to grow during the 90s, and with the arrival of Java, OOP attracted a huge following.
In 2002 in conjunction with the release of the .NET Framework, Microsoft introduced a brand new OOP language called C# – which is often described as the most powerful programming language
It's interesting that, generations later, the concept of organizing your code into meaningful objects that model the parts of your problem continues to puzzle programmers.
Many folks who haven't any idea how a computer works find the thought of object-oriented programming quite natural. In contrast, many folks who have experience with computers initially think there's something strange about object oriented systems.
Structure of OOP
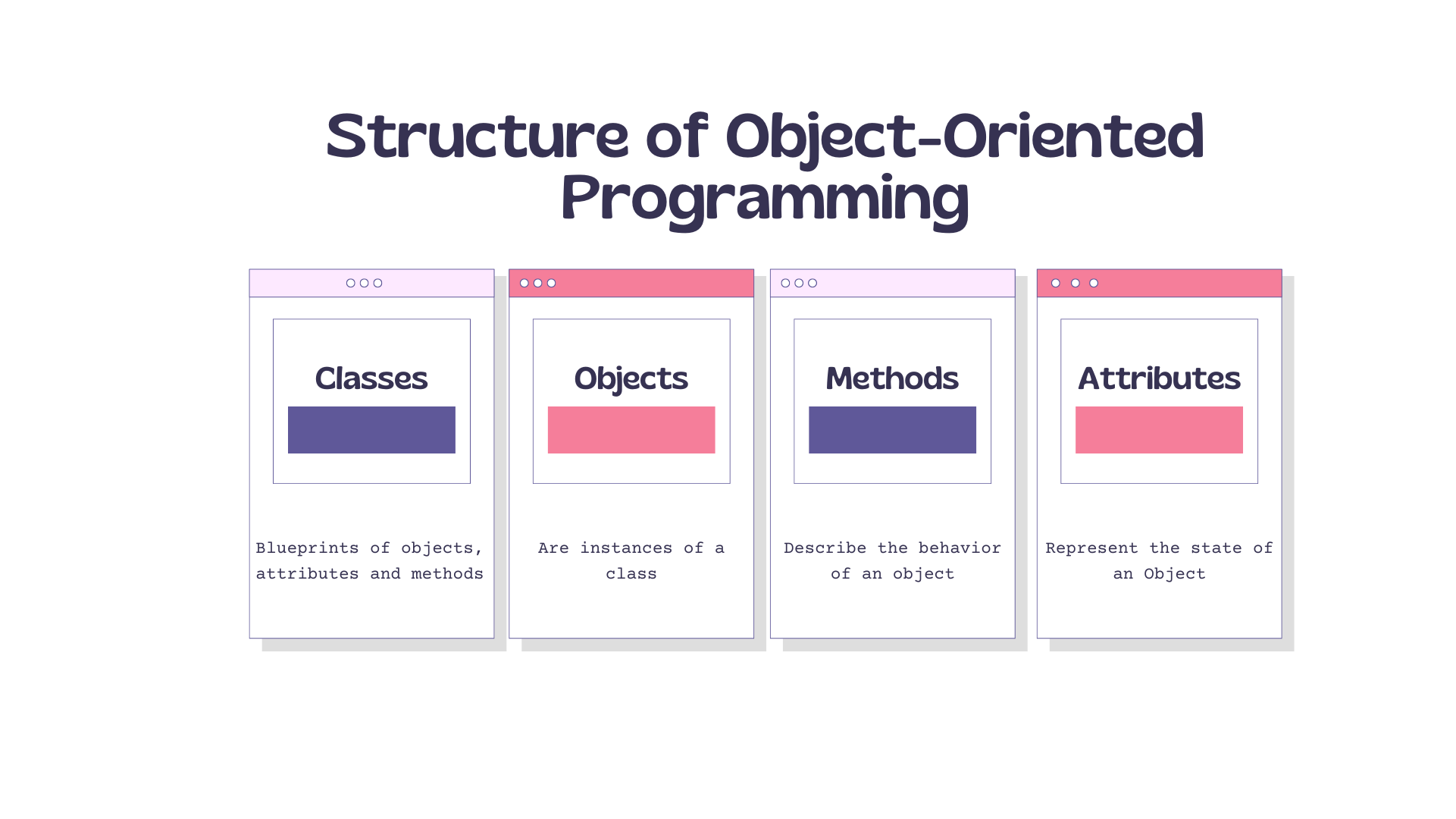
Imagine that you are running a pet shop, with lots of different breeds and you have to keep track of the names, age, days attended to, and other common upkeep details. How would you design reusable software to handle this?
Keep in mind we have many breeds, so writing code for each would be tiresome. But we can group related information together so that we can produce shorter and more reusable code.
That's where the building blocks come in to help us do this by using Classes, Objects, Methods and Attributes .
Let's take a deep dive and understand what exactly these building blocks are:
Classes - these are user-defined data types that act as the blueprint for objects, attributes, and methods.
Objects - These are instances of a class with specifically defined data. When a class is defined initially, the description is the only object that is defined.
Methods - These are functions that are defined inside a class that describe the behavior of an object. They are useful for re-usability or keeping functionality encapsulated inside one object at a time. Code re-usability is a great benefit when debugging.
Attributes - These are defined in the class template and represent the state of an object. Objects contain data stored in the attribute field.
Principles of OOP

In order for us to know how to write good OOP code, we need to understand the 4 pillars of OOP we should adhere to:
Encapsulation
Abstraction, inheritance, polymorphism.
Let's dive in and better understand what exactly each of these mean.
This is the concept that binds data together. Functions manipulate the info and keep it safe. No direct access is granted to the info in case it's hidden. If you wish to gain access to the info, you need to interact with the article in charge of the info.
If you're employed in a company, chances are high that you've had experience with encapsulation.
Think about a human resources department. The human resources staff members encapsulate (hide) the data about employees. They determine how this data will be used and manipulated. Any request for the worker data or request to update the info must be routed through them.
By encapsulating data, you make the information of your system safer and more reliable. You're also able monitor how the information is being accessed and what operations are performed on it. This makes program maintenance easier and simplifies the debugging process.
Abstraction refers to using simple classes to represent complexity. Basically, we use abstraction to handle complexity by allowing the user to only see relevant and useful information.
A good example to explain this is driving an automatic car. When you have an automatic car and want to get from point A to point B, all you need to do is give it the destination and start the car. Then it'll drive you to your destination.
What you don't need to know is how the car is made, how it correctly takes and follows instructions, how the car filters out different options to find the best route, and so on.
The same concept is applied when constructing OOP applications. You do this by hiding details that aren't necessary for the user to see. Abstraction makes it easier and enables you to handle your projects in small, managable parts.
Inheritance allows classes to inherit features of other classes. As an example, you could classify all cats together as having certain common characteristics, like having four legs. Their breeds further classify them into subgroups with common attributes, like size and color.
You use inheritance in OOP to classify the objects in your programs per common characteristics and performance. This makes working with the objects and programming easier, because it enables you to mix general characteristics into a parent object and inherit these characteristics within the child objects.
For example, you'll define an employee object that defines all the overall characteristics of employees in your company.
You'll be able to then define a manager object that inherits the characteristics of the employee object but also adds characteristics unique to managers in your company. The manager object will automatically reflect any changes within the implementation of the employee object.
This is the power of two different objects to reply to one form. The program will determine which usage is critical for every execution of the thing from the parent class which reduces code duplication. It also allows different kinds of objects to interact with the same interface.
Examples of OOP Languages
Technology and programming languages are evolving all the time. We have seen the rise of may langs under the OOP category, but Simula is credited as the first OOP language.
Programming languages that are considered pure OOP treat everything like objects, while the others are designed primarily with some procedural process.
Examples of OOP langs:
- Visual Basic .NET
And many more.
Benefits of OOP
During the 70s and 80s, procedural-oriented programming languages such as C and Pascal were widely used to develop business-oriented software systems. But as the programs performed more complex business functionality and interacted with other systems, the shortcomings of structural programming methodology began to surface.
Because of this, many software developers turned to object-oriented methodologies and programming languages to solve the encountered problems. The benefits of using this languages included the following:
Code Re-usability - through inheritance, you can reuse code. This means a team does not have to write the same code multiple times.
Improved integration with modern operating systems.
Improved Productivity - developers can construct new programs easily and quickly through the use of multiple libraries.
Polymorphism enables a single function to adapt to the class it is placed in.
Easy to upgrade, and programmers can also implement system functionalities independently.
Through Encapsulation objects can be self-contained. It also makes troubleshooting and collaboration on development easier.
By the use of encapsulation and abstraction, complex code is hidden, software maintenance is easier, and internet protocols are protected.
Wrapping Up
Today, most languages allow developers to mix programming paradigms. This is often because they will be used for various programming methods.
For instance, take JavaScript – you can use it for both OOP and functional programming. When you're coding object-oriented JS, you have to think carefully about the structure of the program and plan at the start of coding. You might do this by viewing how you'll be able to break the necessities into simple, reusable classes which will be accustomed blueprint instances of objects.
Developers working with OOP typically agree that in general, using it allows for better data structures and re-usability of code. This saves time in the long term.
I'm a Growing Developer with a keen interest in technology, particularly in the areas of open-source and Python. As a passionate technical writer, I aim to share my knowledge with other developers through informative articles that help them grow and succeed.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
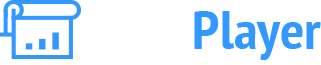
- My presentations
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
OOPS CONCEPT. OOPS Benefits of OOPs OOPs Principles Class Object Objectives.
Published by Justina Todd Modified over 8 years ago
Similar presentations
Presentation on theme: "OOPS CONCEPT. OOPS Benefits of OOPs OOPs Principles Class Object Objectives."— Presentation transcript:

Understand and appreciate Object Oriented Programming (OOP) Objects are self-contained modules or subroutines that contain data as well as the functions.
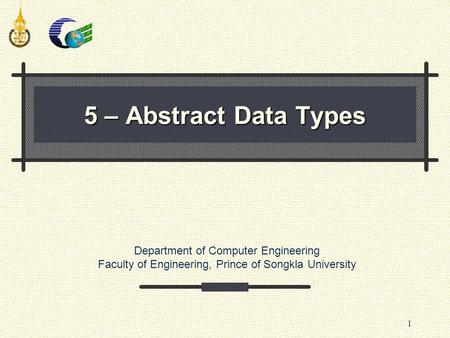
Department of Computer Engineering Faculty of Engineering, Prince of Songkla University 1 5 – Abstract Data Types.
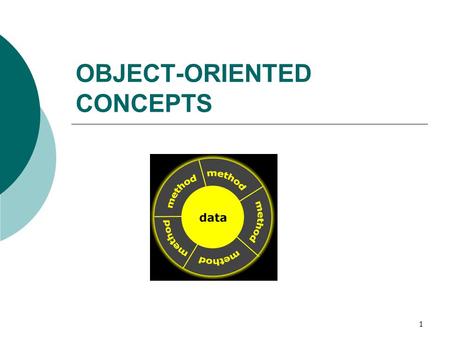
1 OBJECT-ORIENTED CONCEPTS. 2 What is an object? An object is a software entity that mirrors the real world in some way. A software object in OOP.
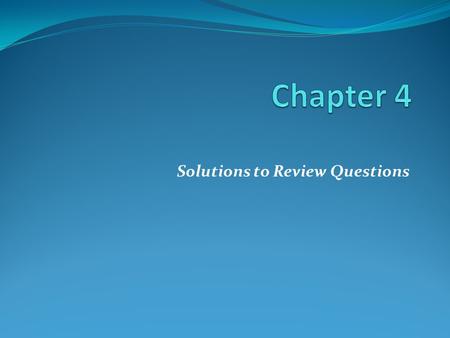
Solutions to Review Questions. 4.1 Define object, class and instance. The UML Glossary gives these definitions: Object: an instance of a class. Class:
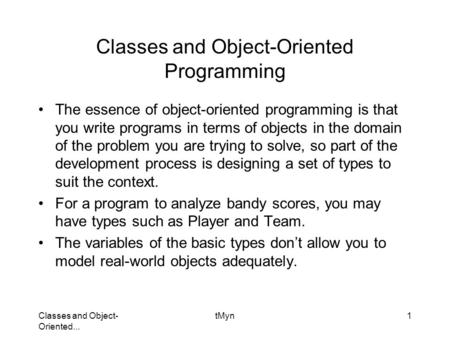
Classes and Object- Oriented... tMyn1 Classes and Object-Oriented Programming The essence of object-oriented programming is that you write programs in.
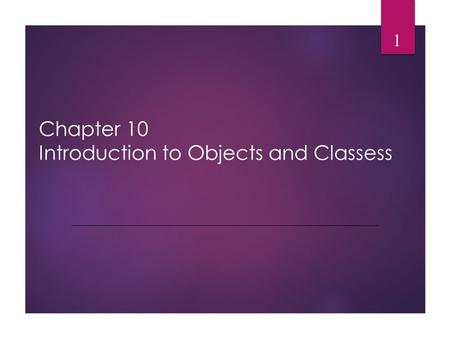
Chapter 10 Introduction to Objects and Classess 1.
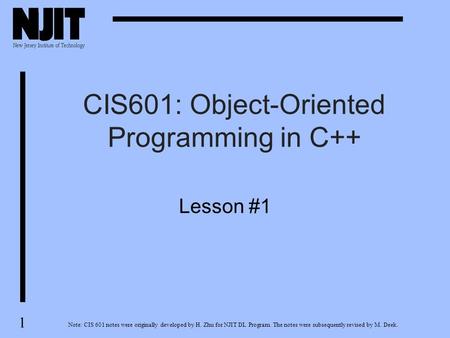
1 CIS601: Object-Oriented Programming in C++ Note: CIS 601 notes were originally developed by H. Zhu for NJIT DL Program. The notes were subsequently revised.
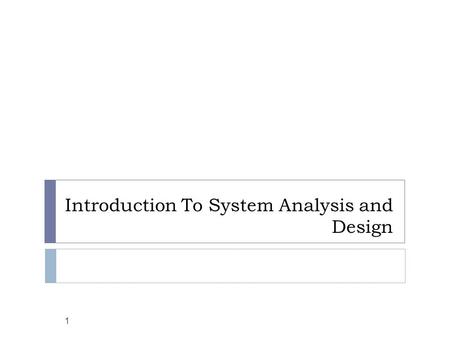
Introduction To System Analysis and Design
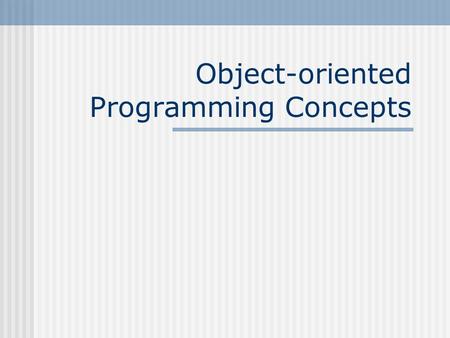
Object-oriented Programming Concepts
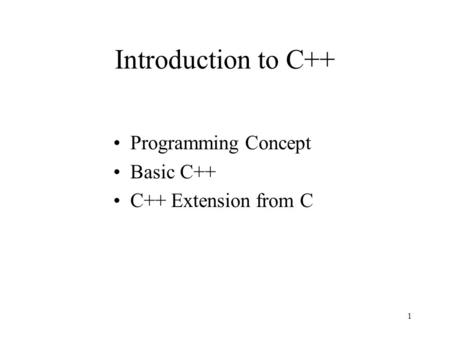
1 Introduction to C++ Programming Concept Basic C++ C++ Extension from C.
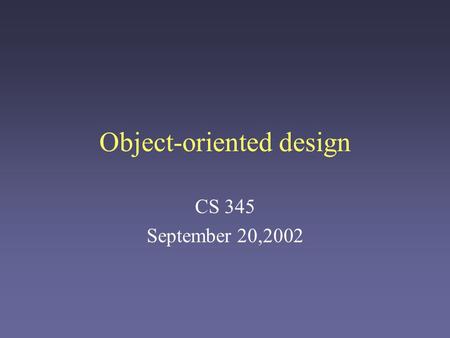
Object-oriented design CS 345 September 20,2002. Unavoidable Complexity Many software systems are very complex: –Many developers –Ongoing lifespan –Large.
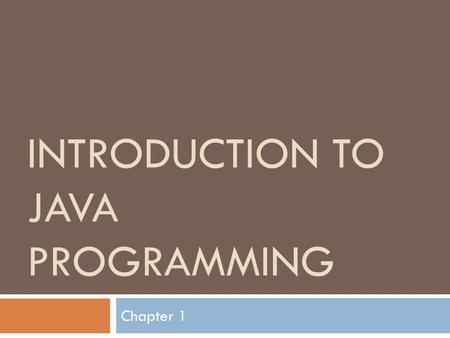
INTRODUCTION TO JAVA PROGRAMMING Chapter 1. What is Computer Programming?
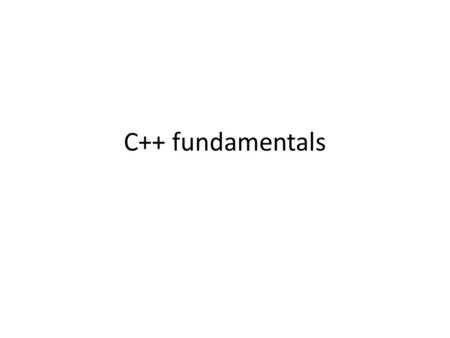
C++ fundamentals.
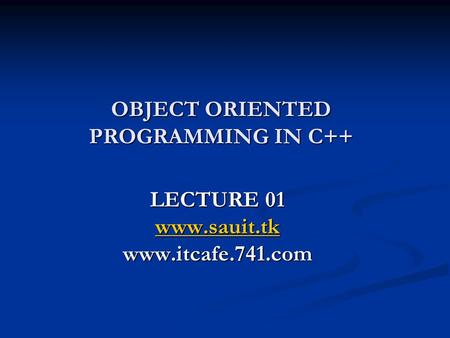
OBJECT ORIENTED PROGRAMMING IN C++ LECTURE
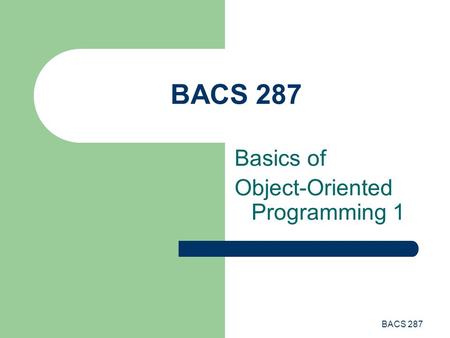
BACS 287 Basics of Object-Oriented Programming 1.
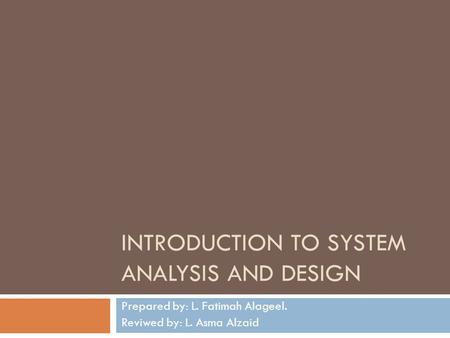
Introduction To System Analysis and design
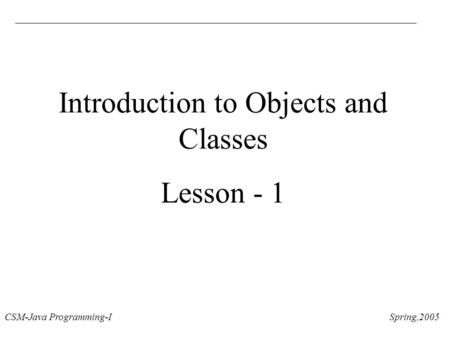
CSM-Java Programming-I Spring,2005 Introduction to Objects and Classes Lesson - 1.
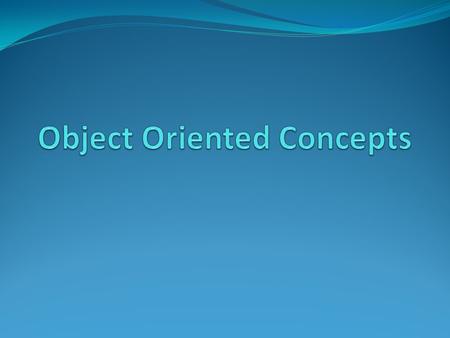
Object Orientation An Object oriented approach views systems and programs as a collection of interacting objects. An object is a thing in a computer system.
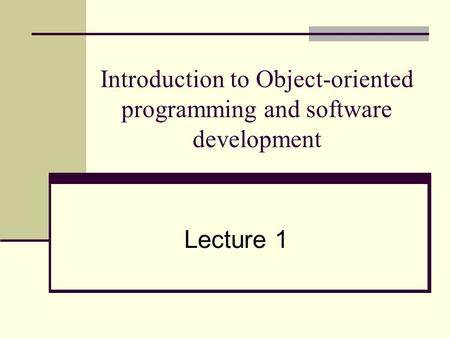
Introduction to Object-oriented programming and software development Lecture 1.
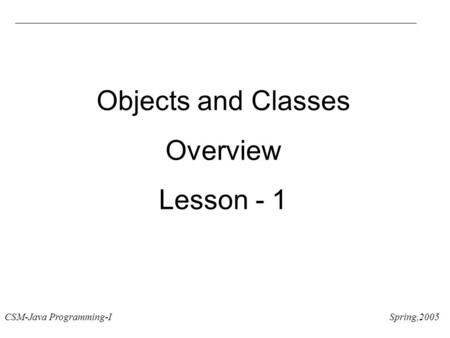
CSM-Java Programming-I Spring,2005 Objects and Classes Overview Lesson - 1.
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
JavaScript seems to be disabled in your browser. For the best experience on our site, be sure to turn on Javascript in your browser.

- My Wish List
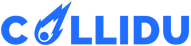
- Compare Products
- Presentations
Object Oriented Programming (OOP)
You must be logged in to download this file*
item details (8 Editable Slides)
(8 Editable Slides)
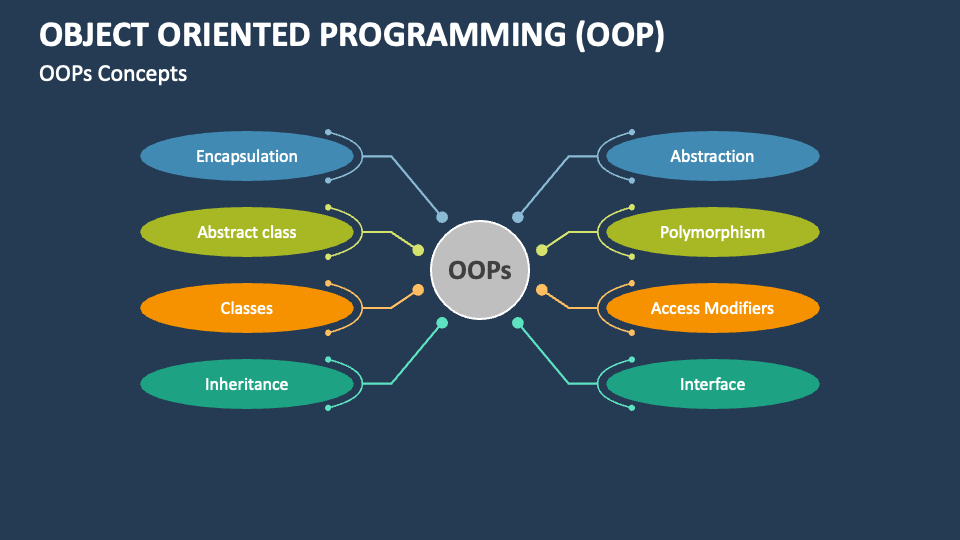
Related Products
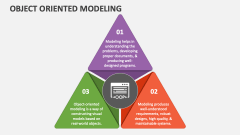
Well-suited for large and complex programs requiring regular maintenance, OOP is a popular programming paradigm based on the concept of objects in the form of data and functions. Describe this programming language's importance, uses, and other key aspects with our Object-Oriented Programming (OOP) presentation template, which is compatible with MS PowerPoint and Google Slides.
Software developers and project heads will find these aesthetically pleasing visuals ideal for demonstrating the benefits and the hierarchy of programming paradigms within the OOP approach. By harnessing this deck, you can discuss the OOP applications (Java and machine learning language) and OOP concepts like Polymorphism and Abstraction.
Sizing Charts
Size | XS | S | S | M | M | L |
---|---|---|---|---|---|---|
EU | 32 | 34 | 36 | 38 | 40 | 42 |
UK | 4 | 6 | 8 | 10 | 12 | 14 |
US | 0 | 2 | 4 | 6 | 8 | 10 |
Bust | 79.5cm / 31" | 82cm / 32" | 84.5cm / 33" | 89.5cm / 35" | 94.5cm / 37" | 99.5cm / 39" |
Waist | 61.5cm / 24" | 64cm / 25" | 66.5cm / 26" | 71.5cm / 28" | 76.5cm / 30" | 81.5cm / 32" |
Hip | 86.5cm / 34" | 89cm / 35" | 91.5cm / 36" | 96.5cm / 38" | 101.5cm / 40" | 106.5cm / 42" |
Size | XS | S | M | L | XL | XXL |
---|---|---|---|---|---|---|
UK/US | 34 | 36 | 38 | 40 | 42 | 44 |
Neck | 37cm / 14.5" | 38cm /15" | 39.5cm / 15.5" | 41cm / 16" | 42cm / 16.5" | 43cm / 17" |
Chest | 86.5cm / 34" | 91.5cm / 36" | 96.5cm / 38" | 101.5cm / 40" | 106.5cm / 42" | 111.5cm / 44" |
Waist | 71.5cm / 28" | 76.5cm / 30" | 81.5cm / 32" | 86.5cm / 34" | 91.5cm / 36" | 96.5cm / 38" |
Seat | 90cm / 35.4" | 95cm / 37.4" | 100cm / 39.4" | 105cm / 41.3" | 110cm / 43.3" | 115cm / 45.3" |
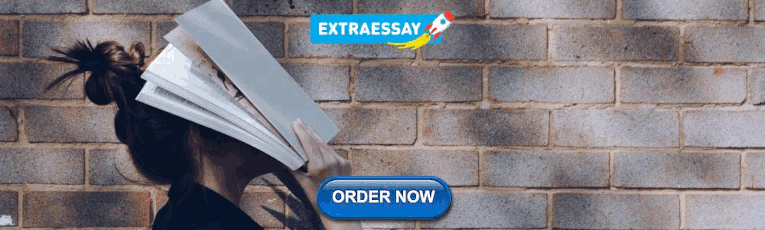
Browse Course Material
Course info, instructors.
- Dr. Ana Bell
- Prof. Eric Grimson
- Prof. John Guttag
Departments
- Electrical Engineering and Computer Science
As Taught In
- Algorithms and Data Structures
- Programming Languages
Learning Resource Types
Introduction to computer science and programming in python, lecture 8: object oriented programming.
Description: In this lecture, Dr. Bell introduces Object Oriented Programming and discusses its representation in Python.
Instructor: Dr. Ana Bell
- Download video
- Download transcript

You are leaving MIT OpenCourseWare
JavaScript seems to be disabled in your browser. For the best experience on our site, be sure to turn on Javascript in your browser.
Exclusive access to over 200,000 completely editable slides.
- Diagram Finder
- Free Templates

- Human Resources
- Project Management
- Timelines & Planning
- Health & Wellness
- Environment
- Cause & Effect
- Executive Summary
- Customer Journey
- 30 60 90 Day Plan
- Social Media
- Escalation Matrix
- Communication
- Go to Market Plan/Strategy
- Recruitment
- Pros and Cons
- Business Plan
- Risk Management
- Roles and Responsibilities
- Mental Health
- ISO Standards
- Process Diagrams
- Puzzle Diagrams
- Organizational Charts
- Arrow Diagrams
- Infographics
- Tree Diagrams
- Matrix Charts
- Stage Diagrams
- Text Boxes & Tables
- Data Driven Charts
- Flow Charts
- Square Puzzle
- Circle Puzzle
- Circular Arrows
- Circle Segments
- Matrix Table
- Pillar Diagrams
- Triangle Puzzle
- Compare Diagrams
- Ladder Diagrams
- Google Slides
- North America Maps
- United States (US) Maps
- Europe Maps
- South America Maps
- Apple Keynote
- People & Objects
- Trending Products
- PowerPoint Templates
Object Oriented Programming (OOP) PowerPoint and Google Slides Template
(8 Editable Slides)
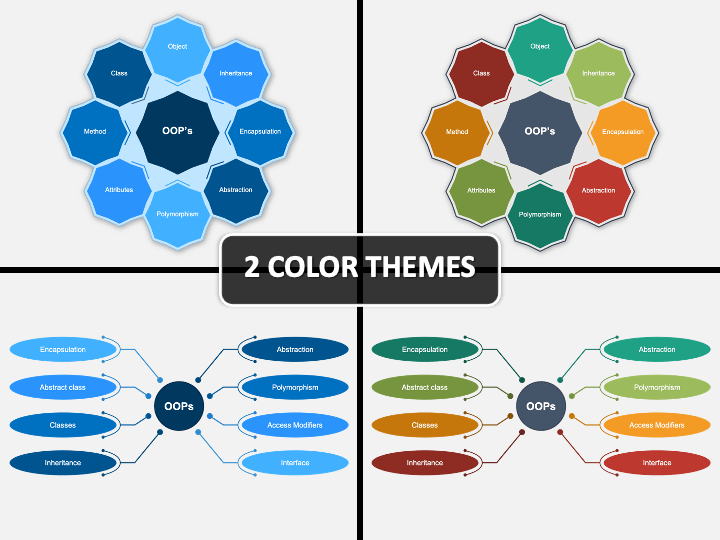
Download Now
This template is part of our Pro Plan.
Gain access to over 200,000 slides with pro plan..
Upgrade Now
Already a Pro customer? Login
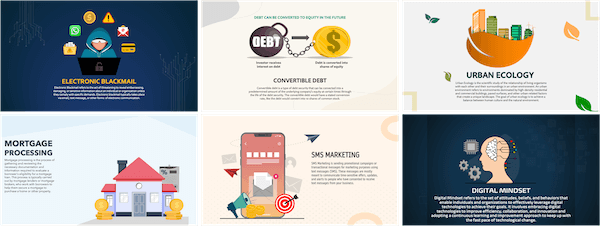
Related Products
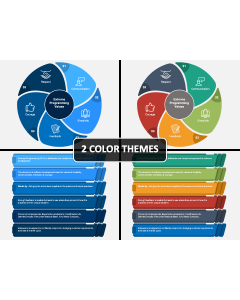
Extreme Programming PowerPoint and Google Slides Template
(7 Editable Slides)
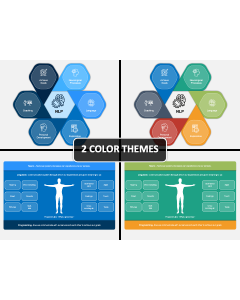
Neuro Linguistic Programming PowerPoint and Google Slides Template
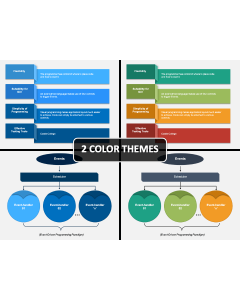
Event Driven Programming PowerPoint and Google Slides Template
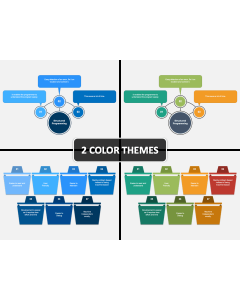
Structured Programming
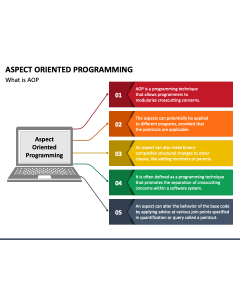
Aspect Oriented Programming
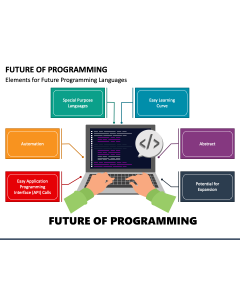
Future of Programming
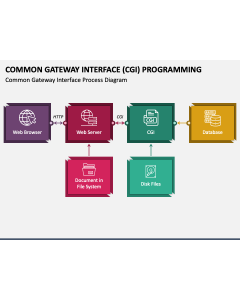
Common Gateway Interface (CGI) Programming
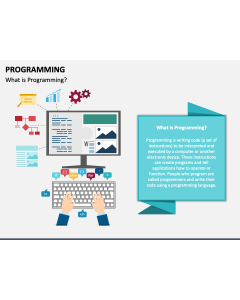
Programming
Leverage our feature-rich Object Oriented Programming PPT template to showcase the reasons why object-oriented programming (OOP) is the most popular programming paradigm and how it is better than procedural programming. Developers can also use this entirely editable deck to exhibit how this programming is used in structuring a complex and large software program.
The set comprises high-quality graphics, cutting-edge designs, and thoroughly researched content. Using it, you can make your presentation stand out and grab your audience’s attention from the beginning to the end of the slideshow.
Key Highlights of the Deck
- The first slide represents a uniquely-designed infographic comprising a circle in the center with several oval-shaped textual boxes attached. It can be used to showcase several OOPs concepts, such as Encapsulation, Abstract Class, Inheritance, etc.
- The hierarchy of programming paradigms is represented through a pyramid-shaped illustration.
- The benefits of OOPs are illustrated through triangular diagrams.
- Access Modifiers are demonstrated through a beautifully-crafted infographic.
- Abstraction is presented in one of the slides.
- A flowchart diagram depicts polymorphism and its sub-components.
- All the concepts of OOPs for Machine Learning are depicted through another well-designed layout.
- OOPs in Java are demonstrated in the last slide.
Key Features of the Template
- You can easily include any visual element in your upcoming or current presentations.
- Easy-to-edit; you can easily modify the size, color, and other elements of the set even if you don’t possess any designing or editing skills.
- You can present the visuals on any screen size without fretting about the resolution.
So, download this power-packed PPT now to accentuate your presentations!
Create compelling presentations in less time
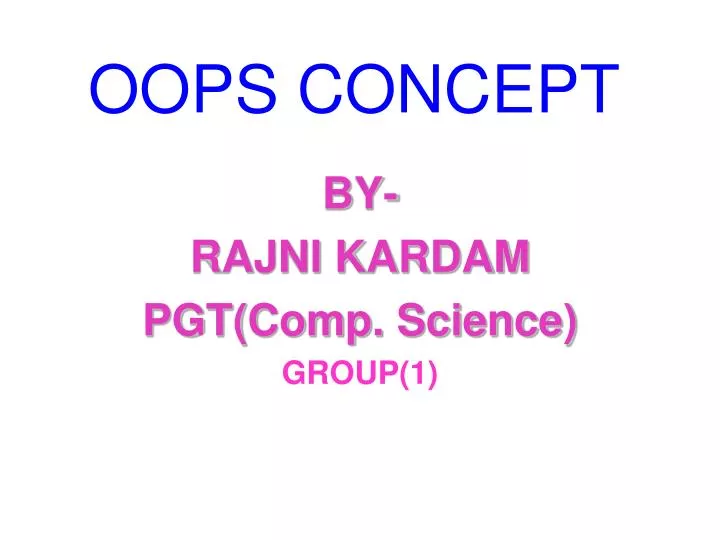
OOPS CONCEPT
Nov 05, 2014
4.28k likes | 7.87k Views
OOPS CONCEPT. BY- RAJNI KARDAM PGT(Comp. Science) GROUP(1). OOPS. Object Oriented Programming Structure. PROCEDURAL Vs OOP PROGRAMMING. OBJECT. Object is an identifiable entity with some characteristics and behaviour. CLASS.
Share Presentation
- data encapsulation
- data abstraction
- class represents
- share common properties
- single unit called class
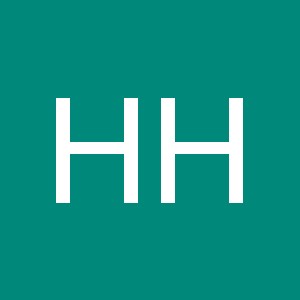
Presentation Transcript
OOPS CONCEPT BY- RAJNI KARDAM PGT(Comp. Science) GROUP(1)
OOPS • Object Oriented Programming Structure
PROCEDURAL Vs OOP PROGRAMMING
OBJECT • Object is an identifiable entity with some characteristics and behaviour.
CLASS • A CLASS represents a group of objects that share common properties and relationships. • Object can be defined as a variable of type class.
Features of OOPs • Data Abstraction • Data Encapsulation & data hiding • Modularity • Inheritance • Polymorphism
Data Abstraction • Abstraction refers to the act of representing essential features without including the background details of explanations.
Data Encapsulation • The wrapping up of data and functions (that operate on data) into a single unit (called class) is known as ENCAPSULATION. DATA Member functions Object
MODULARITY • MODULARITY is a property of a system that has been decomposed into a set of cohesive and loosely coupled modules.
INHERITENCE • INHERITENCE is the capability of one class of things to inherit capabilities or properties from another class.
EXAMPLE OF INHERITENCE
POLYMORPHISM • POLYMORPHISM is the ability for a message or data to be processed in more than one form. Polymorphism is a property by which the same message can be sent to objects of several different classes.
- More by User
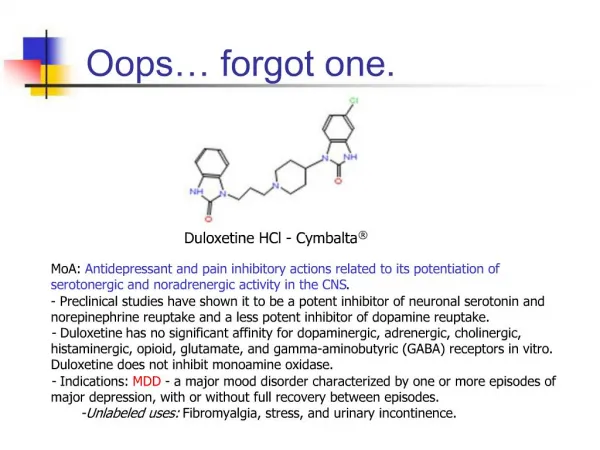
Oops forgot one.
Hypnotic Agents. A dose-related, generalized depression (more than seen with anti-anxiety sedative drugs) of the CNS that produces drowsiness and induces sleepOften an increase in the dosage of a sedative agent will produce hypnotic activityIdeal hypnotic agent would produce drowsiness and facilit
395 views • 22 slides
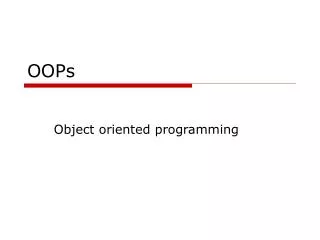
OOPs. Object oriented programming. Abstract data types. Representationof type and operations in a single unit Available for other units to create variables of the type Possibility of hiding data structure of type and implementation of operations. Limitations of ADT and OOP extensions.
549 views • 21 slides
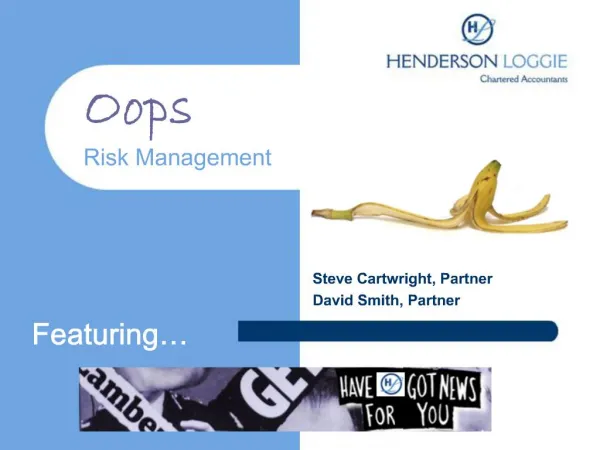
Oops Risk Management
288 views • 15 slides
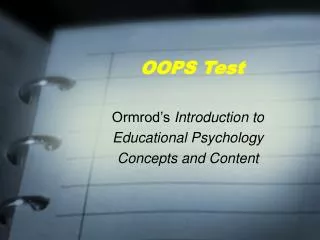
OOPS Test. Ormrod’s Introduction to Educational Psychology Concepts and Content. DIRECTIONS: Read each statement; then decide if each statement is true or false. Mark your response on a sheet of paper. .
472 views • 21 slides
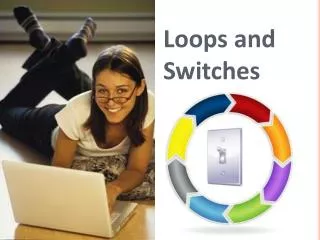
L oops and Switches
L oops and Switches. Loops and Switches Pre-Quiz. 1. What kind of blocks are these ? 2 . Name two kinds of controls that can be specified to determine how long a loop repeats. 3 . Give an example of a program you could write that would use a switch.
416 views • 27 slides
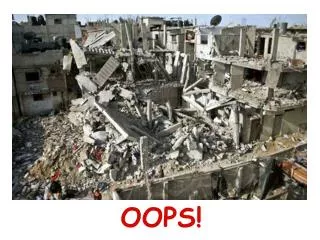
OOPS!. Reconstruction (1865-1877). Key Questions. 1. How do we bring the South back into the Union?. 4. To what extend Was reconstruction successful?. 2. How do we rebuild the South after its destruction during the war?.
255 views • 6 slides
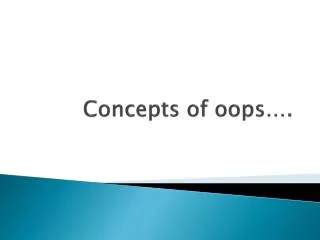
Concepts of oops….
Concepts of oops…. Objects. Object is the basic unit of object-oriented programming. Objects are identified by its unique name. An object represents a particular instance of a class. There can be more than one instance of a class. Each instance of a class can hold its own relevant data.
664 views • 10 slides
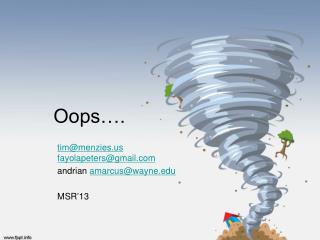
Oops…. [email protected] [email protected] andrian [email protected] MSR ’ 13. Inevitable, due to the complexity &novelty of our work. (But rarely reported, which is…. suspicious) What can we learn from those mistakes?.
744 views • 14 slides
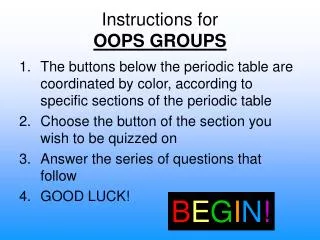
Instructions for OOPS GROUPS
Instructions for OOPS GROUPS. The buttons below the periodic table are coordinated by color, according to specific sections of the periodic table Choose the button of the section you wish to be quizzed on Answer the series of questions that follow GOOD LUCK!. B E G I N !.
773 views • 65 slides
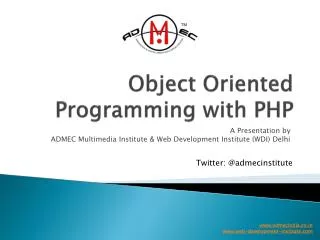
Object Oriented PHP | OOPs
This presentation will gives you comprehensive description about object oriented php
1.35k views • 29 slides
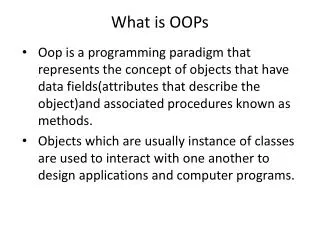
What is OOPs
What is OOPs. Oop is a programming paradigm that represents the concept of objects that have data fields(attributes that describe the object)and associated procedures known as methods.
2.56k views • 169 slides
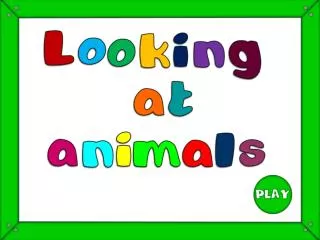
What animal is it?. snake. oops. tortoise. great. rabbit. oops. next. What animal is it?. ant. oops. fish. oops. lizard. great. next. What animal is it?. canary. oops. stick insect. great. snail. oops. next. What animal is it?. guinea pig. oops. ant. great. lizard.
305 views • 9 slides
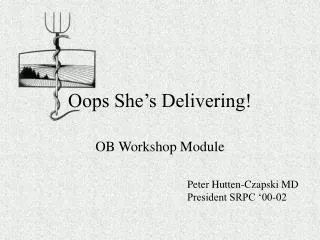
Oops She’s Delivering!
Oops She’s Delivering!. OB Workshop Module. Peter Hutten-Czapski MD President SRPC ‘00-02. Oops She’s Delivering.
705 views • 46 slides
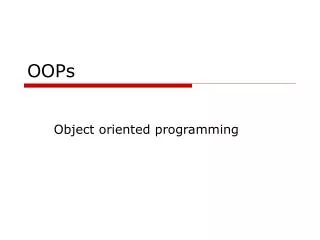
OOPs. Object oriented programming. Based on ADT principles. Representation of type and operations in a single unit Available for other units to create variables of the type Possibility of hiding data structure of type and implementation of operations. Limitations of ADT --> OOP extensions.
471 views • 25 slides
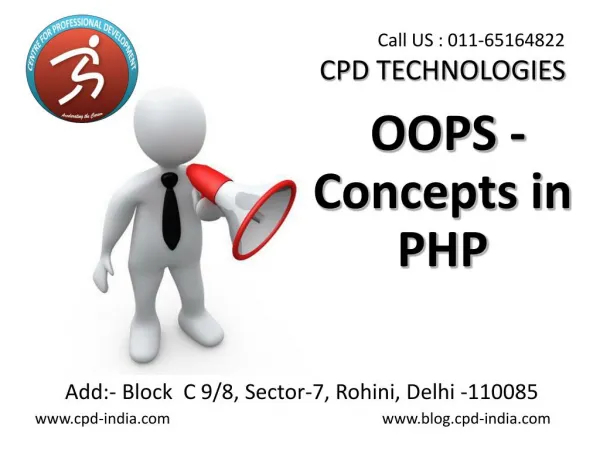
OOPS - concepts in php
Object Oriented Programming (OOP) is the programming method that involves the use of the data , structure and organize classes of an application. The data structure becomes an objects that includes both functions and data.
655 views • 44 slides
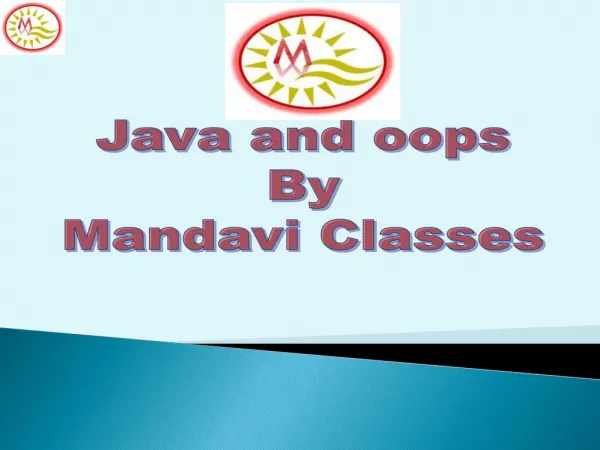
Java and oops
Java is a general-purpose computer programming language that is concurrent, class-based, object-oriented,and specifically designed to have as few implementation dependencies as possible. It is intended to let application developers "write once, run anywhere" (WORA)
392 views • 18 slides
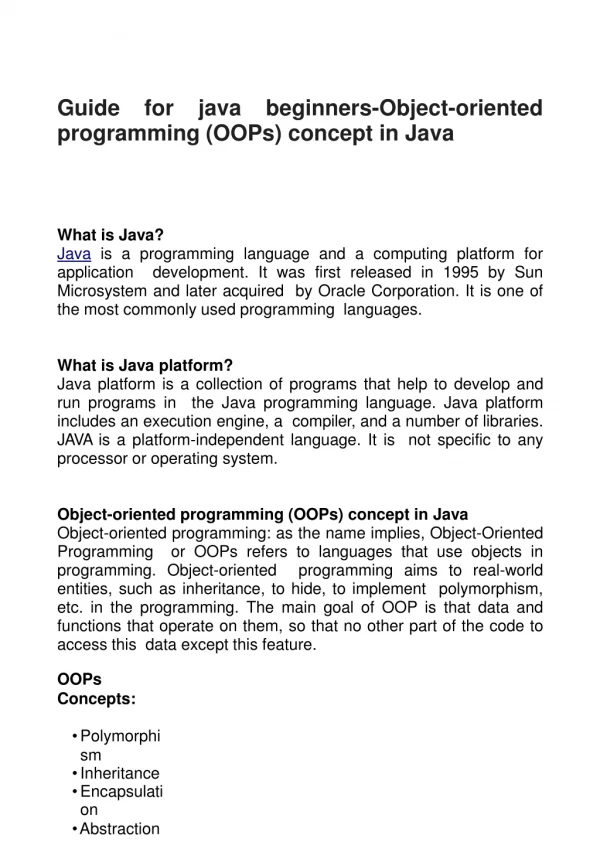
Object-oriented programming (OOPs) concept in Java
Object-oriented programming (OOPs) concept in Java Object-oriented programming: as the name implies, Object-Oriented Programming or OOPs refers to languages that use objects in programming. Object-oriented programming aims to real-world entities, such as inheritance, to hide, to implement polymorphism, etc. in the programming. The main goal of OOP is that data and functions that operate on them, so that no other part of the code to access this data except this feature.
75 views • 3 slides
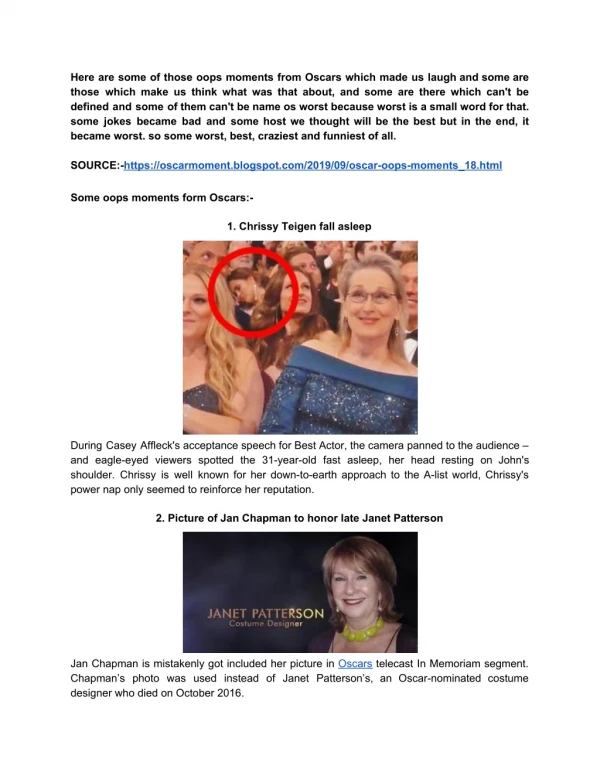
Oscar oops moments
Here are all those moments where the thing that happens was not supposed to happen at that time.
56 views • 4 slides
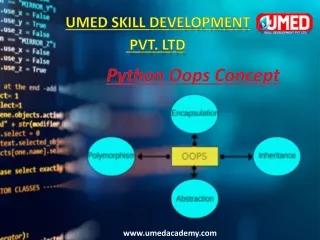
Python Oops Concept
Learn each important topic - classes, object, inheritance, abstraction and more with proper examples of the Python Oops Concept at UMED Academy and start your object oriented journey step-by-step. for more informationu2019s you can visit our website http://www.umedacademy.com/, mail [email protected] & call 91 8338000667.
342 views • 9 slides
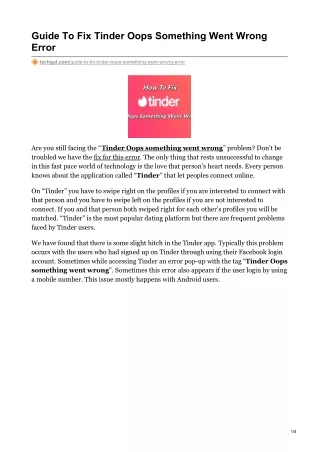
tinder oops
Learn how to fix Tinder's error u201cOops something went wrongu201c. It is one of the most popular platforms on the internet. Itu2019s quite a long time from now that people are experiencing little issues with login. Especially those who signed up for Tinder with their Facebook profile or ID. But most users having the same issue even when they trying logging in with their phone numbers.
113 views • 4 slides
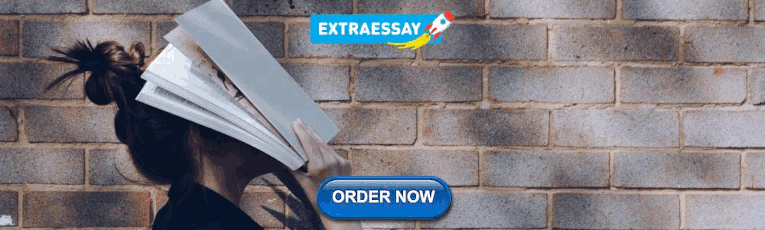
IMAGES
COMMENTS
Introduction to OOP. Building Blocks of OOP: Objects & Classes. Object: models a. Real world object (ex. computer, book, box) Concept (ex. meeting, interview) Process (ex. sorting a stack of papers or comparing two computers to measure their performance) . Class: prototype or blueprint from which objects are created. Introduction to OOP.
OOP provides an easy way to add new data and function. Data Access. In POP, Most function uses Global data for sharing that can be accessed freely from function to function in the system. In OOP, data can not move easily from function to function, it can be kept public or private so we can control the access of data.
Lecture 15: Object-Oriented Programming. CS 106B: Programming Abstractions. Summer 2020, Stanford University Computer Science Department. Lecturers: Nick Bowman and Kylie Jue. For every lecture, we will post the lecture slides and any example code that will be used during lecture, usually in advance of the beginning of the lecture. For today's ...
OBJECT ORIENTED PROGRAMMING (OOP) EVERYTHING IN PYTHON IS AN OBJECT. can create new objects of some type (and has a type) can manipulate objects. can destroy objects. explicitly using del or just "forget" about them. python system will reclaim destroyed or inaccessible objects - called "garbage collection".
Object-oriented programming (OOP) is a fundamental programming paradigm used by nearly every developer at some point in their career. OOP is the most popular programming paradigm used for software development and is taught as the standard way to code for most of a programmer's educational career. Another popular programming paradigm is ...
Lecture presentation on programming in Java. Topics include: object oriented programming, defining classes, using classes, constructors, methods, accessing fields, primitives versus references, references versus values, and static types and methods.
People think of the world in terms of interacting objects: we'd talk about interactions between the steering wheel, the pedals, the wheels, etc. OOP allows programmers to pack away details into neat, self-contained boxes (objects) so that they can think of the objects more abstractly and focus on the interactions between them.
1.5 Basic Concepts of OOP 1.5.1 Objects : Objects are the basic runtime entities in an object oriented system.Theymayrepresentaperson,aplace,abankaccount,a table of data or any item that the program has to handle
Download Free PPT. Download Free PDF ... (OOP) - CS304 Power Point Slides Lecture. Object Oriented Programming (OOP) - CS304 Power Point Slides Lecture. Tayyaba Naz. See Full PDF Download PDF. See Full PDF Download PDF. Related Papers. CS6456 OBJECT ORIENTED PROGRAMMING LECTURE NOTES 2014-2015(EVEN SEM) Prepared by.
Last Updated : 09 Feb, 2023. As the name suggests, Object-Oriented Programming or OOPs refers to languages that use objects in programming. Object-oriented programming aims to implement real-world entities like inheritance, hiding, polymorphism, etc in programming. The main aim of OOP is to bind together the data and the functions that operate ...
The word object-oriented is a combination of two terms, object and oriented. The dictionary meaning of an object is "an entity that exists in the real world", and oriented means "interested in a particular kind of thing or entity". In basic terms, OOP is a programming pattern that is built around objects or entities, so it's called object ...
Presentation on theme: "OOPS CONCEPT. OOPS Benefits of OOPs OOPs Principles Class Object Objectives."— Presentation transcript: 1 OOPS CONCEPT 2 OOPS Benefits of OOPs OOPs Principles Class Object Objectives 3 Top-down structured design: uses algorithmic decomposition where each module denotes a major step in some overall process Object ...
Well-suited for large and complex programs requiring regular maintenance, OOP is a popular programming paradigm based on the concept of objects in the form of data and functions. Describe this programming language's importance, uses, and other key aspects with our Object-Oriented Programming (OOP) presentation template, which is compatible with ...
Description: In this lecture, Dr. Bell introduces Object Oriented Programming and discusses its representation in Python. Instructor: Dr. Ana Bell
Create compelling presentations in less time. Exclusive access to over 200,000 completely editable slides. The Object Oriented Programming PowerPoint and Google Slides template will make your ideas stand out with high-quality illustrations and spellbinding features. Download it right now!
Object Oriented Programming. Chapter 2 introduces Object Oriented Programming. OOP is a relatively new approach to programming which supports the creation of new data types and operations to manipulate those types. This presentation introduces OOP. Data Structures and Other Objects. 587 views • 39 slides
Presentation Transcript. OOPS CONCEPT BY- RAJNI KARDAM PGT (Comp. Science) GROUP (1) OOPS • Object Oriented Programming Structure. PROCEDURAL Vs OOP PROGRAMMING. OBJECT • Object is an identifiable entity with some characteristics and behaviour. CLASS • A CLASS represents a group of objects that share common properties and relationships.