Optional sequence of specifiers.The following specifiers are allowed: : allows body to modify the parameters captured by copy, and to call their non-const member functions : explicitly specifies that the function call operator is a function. When this specifier is not present, the function call operator will be anyway, if it happens to satisfy all constexpr function requirements | (since C++17) | : specifies that the function call operator is an . and cannot be used at the same time. | (since C++20) | The lambda expression is a prvalue expression of unique unnamed non-union non-aggregate class type, known as closure type , which is declared (for the purposes of ADL ) in the smallest block scope, class scope, or namespace scope that contains the lambda expression. The closure type has the following members: ClosureType:: operator()( params ) )(params) const { body } | | (the keyword mutable was not used) | )(params) { body } | | (the keyword mutable was used) | <template-params> ret operator()(params) const { body } | | (since C++14) (generic lambda) | <template-params> ret operator()(params) { body } | | (since C++14) (generic lambda, the keyword mutable was used) | | | | Executes the body of the lambda-expression, when invoked. When accessing a variable, accesses its captured copy (for the entities captured by copy), or the original object (for the entities captured by reference). Unless the keyword mutable was used in the lambda-expression, the function-call operator is const-qualified and the objects that were captured by copy are non-modifiable from inside this operator ( ) . The function-call operator is never volatile-qualified and never virtual. The function-call operator is always if it satisfies the requirements of a . It is also constexpr if the keyword constexpr was used in the lambda declaration. | (since C++17) | The function-call operator is an if the keyword was used in the lambda expression. | (since C++20) | For every parameter in params whose type is specified as , an invented template parameter is added to template-params, in order of appearance. The invented template parameter may be a if the corresponding function member of params is a function parameter pack. auto glambda = [](auto a, auto&& b) { return a < b; }; bool b = glambda(3, 3.14); // ok // generic lambda, operator() is a template with one parameter auto vglambda = [](auto printer) { return [=](auto&&... ts) // generic lambda, ts is a parameter pack { printer( <decltype(ts)>(ts)...); return [=] { printer(ts...); }; // nullary lambda (takes no parameters) }; }; auto p = vglambda([](auto v1, auto v2, auto v3) { << v1 << v2 << v3; }); auto q = p(1, 'a', 3.14); // outputs 1a3.14 q(); // outputs 1a3.14 's cannot be explicitly instantiated or explicitly specialized. | (since C++14) | If the lambda definition uses an explicit template parameter list, that template parameter list is used with . For every parameter in params whose type is specified as , an additional invented template parameter is appended to the end of that template parameter list: auto glambda = []<class T>(T a, auto&& b) { return a < b; }; // generic lambda, operator() is a template with one parameter pack auto f = []<typename ...Ts>(Ts&& ...ts) { return foo( <Ts>(ts)...); }; | (since C++20) | the exception specification exception on the lambda-expression applies to the function-call operator or operator template. For the purpose of name lookup , determining the type and value of the this pointer and for accessing non-static class members, the body of the closure type's function call operator is considered in the context of the lambda-expression. ClosureType 's operator() cannot be named in a friend declaration. Dangling referencesIf a non-reference entity is captured by reference, implicitly or explicitly, and the function call operator of the closure object is invoked after the entity's lifetime has ended, undefined behavior occurs. The C++ closures do not extend the lifetimes of the captured references. Same applies to the lifetime of the object pointed to by the captured this pointer. ClosureType:: operator ret (*)( params )() | | | F = ret(*)(params); operator F() const; | | (until C++17) | F = ret(*)(params); constexpr operator F() const; | | (since C++17) | | | | <template-params> using fptr_t = /*see below*/; template<template-params> operator fptr_t<template-params>() const; | | (since C++14) (until C++17) | <template-params> using fptr_t = /*see below*/; template<template-params> constexpr operator fptr_t<template-params>() const; | | (since C++17) | | | | This user-defined conversion function is only defined if the capture list of the lambda-expression is empty. It is a public, constexpr, (since C++17) non-virtual, non-explicit, const noexcept (since C++14) member function of the closure object. It is an immediate function if the function call operator of the lambda is an immediate function. (since C++20) A generic captureless lambda has a user-defined conversion function template with the same invented template parameter list as the function-call operator template. If the return type is empty or auto, it is obtained by return type deduction on the function template specialization, which, in turn, is obtained by for conversion function templates. f1(int (*)(int)) {} void f2(char (*)(int)) {} void h(int (*)(int)) {} // #1 void h(char (*)(int)) {} // #2 auto glambda = [](auto a) { return a; }; f1(glambda); // ok f2(glambda); // error: not convertible h(glambda); // ok: calls #1 since #2 is not convertible int& (*fpi)(int*) = [](auto* a)->auto& { return *a; }; // ok | (since C++14) | The value returned by this conversion function is a pointer to a function with C++ language linkage that, when invoked, has the same effect as invoking the closure object's function call operator directly. This function is constexpr if the function call operator (or specialization, for generic lambdas) is constexpr. Fwd= [](int(*fp)(int), auto a){return fp(a);}; auto C=[](auto a){return a;}; static_assert(Fwd(C,3)==3);//OK auto NC=[](auto a){static int s; return a;}; static_assert(Fwd(NC,3)==3); // error: no specialization can be constexpr because of s If the closure object's has a non-throwing exception specification, then the pointer returned by this function has the type pointer to noexcept function. | (since C++17) | This function is an if the function call operator (or specialization, for generic lambdas) is an immediate function. | (since C++20) | ClosureType:: ClosureType() ) = delete; | | (until C++14) | ) = default; | | (since C++20)(only if no captures are specified) | const ClosureType& ) = default; | | (since C++14) | ClosureType&& ) = default; | | (since C++14) | | | | Closure types are not . Closure types have a deleted (until C++14)no (since C++14) default constructor. | (until C++20) | If no captures are specified, the closure type has a defaulted default constructor. Otherwise, it has no default constructor (this includes the case when there is a capture-default, even if it does not actually capture anything). | (since C++20) | The copy constructor and the move constructor are implicitly-declared (until C++14) declared as defaulted (since C++14) and may be implicitly-defined according to the usual rules for copy constructors and move constructors . ClosureType:: operator=(const ClosureType&) operator=(const ClosureType&) = delete; | | (until C++20) | operator=(const ClosureType&) = default; ClosureType& operator=(ClosureType&&) = default; | | (since C++20) (only if no captures are specified) | operator=(const ClosureType&) = delete; | | (since C++20) (otherwise) | | | | The copy assignment operator is defined as deleted (and the move assignment operator is not declared). Closure types are not . | (until C++20) | If no captures are specified, the closure type has a defaulted copy assignment operator and a defaulted move assignment operator. Otherwise, it has a deleted copy assignment operator (this includes the case when there is a capture-default, even if it does not actually capture anything). | (since C++20) | ClosureType:: ~ClosureType()The destructor is implicitly-declared. ClosureType:: CapturesIf the lambda-expression captures anything by copy (either implicitly with capture clause [=] or explicitly with a capture that does not include the character &, e.g. [a, b, c] ), the closure type includes unnamed non-static data members, declared in unspecified order, that hold copies of all entities that were so captured. Those data members that correspond to captures without initializers are direct-initialized when the lambda-expression is evaluated. Those that correspond to captures with initializers are initialized as the initializer requires (could be copy- or direct-initialization). If an array is captured, array elements are direct-initialized in increasing index order. The order in which the data members are initialized is the order in which they are declared (which is unspecified). The type of each data member is the type of the corresponding captured entity, except if the entity has reference type (in that case, references to functions are captured as lvalue references to the referenced functions, and references to objects are captured as copies of the referenced objects). For the entities that are captured by reference (with the default capture [&] or when using the character &, e.g. [&a, &b, &c] ), it is unspecified if additional data members are declared in the closure type , but any such additional members must satisfy LiteralType (since C++17) . Lambda-expressions are not allowed in , , , , and anywhere in a function (or function template) declaration except the function body and the function's . | (until C++20) | Lambda captureThe captures is a comma-separated list of zero or more captures , optionally beginning with the capture-default . The only capture defaults are - & (implicitly capture the used automatic variables by reference) and
- = (implicitly capture the used automatic variables by copy).
The current object ( *this ) can be implicitly captured if either capture default is present. If implicitly captured, it is always captured by reference, even if the capture default is = . The implicit capture of *this when the capture default is = is deprecated. (since C++20) The syntax of an individual capture in captures is | identifier | (1) | | | identifier | (2) | | | identifier initializer | (3) | (since C++14) | | identifier | (4) | | | identifier | (5) | | | identifier initializer | (6) | (since C++14) | | | (7) | | | | (8) | (since C++17) | | If the capture-default is & , subsequent simple captures must not begin with & . If the capture-default is = , subsequent simple captures must begin with & or be *this (since C++17) or this (since C++20) . Any capture may appear only once: Only lambda-expressions defined at block scope or in a default member initializer may have a capture-default or captures without initializers. For such lambda-expression, the reaching scope is defined as the set of enclosing scopes up to and including the innermost enclosing function (and its parameters). This includes nested block scopes and the scopes of enclosing lambdas if this lambda is nested. The identifier in any capture without an initializer (other than the this -capture) is looked up using usual unqualified name lookup in the reaching scope of the lambda. The result of the lookup must be a variable with automatic storage duration declared in the reaching scope. The variable (or this ) is explicitly captured . A capture with an initializer acts as if it declares and explicitly captures a variable declared with type , whose declarative region is the body of the lambda expression (that is, it is not in scope within its initializer), except that: This is used to capture move-only types with a capture such as x = std::move(x). This also makes it possible to capture by const reference, with &cr = (x) or similar. x = 4; auto y = [&r = x, x = x + 1]()->int { r += 2; return x * x; }(); // updates ::x to 6 and initializes y to 25. | (since C++14) | If a capture list has a capture-default and does not explicitly capture the enclosing object (as this or *this ) or an automatic variable, it captures it implicitly if - the body of the lambda odr-uses the variable or the this pointer
pointer is named in a potentially-evaluated expression within an expression that depends on a generic lambda parameter (until C++17) (including when the implicit is added before a use of non-static class member). For this purpose, the operand of is always considered potentially-evaluated. Entities might be implicitly captured even if they are only named within a . (since C++17) f(int, const int (&)[2] = {}) {} // #1 void f(const int&, const int (&)[1]) {} // #2 void test() { const int x = 17; auto g0 = [](auto a) { f(x); }; // ok: calls #1, does not capture x auto g1 = [=](auto a) { f(x); }; // does not capture x in C++14, captures x in C++17 // the capture can be optimized away auto g2 = [=](auto a) { int selector[sizeof(a) == 1 ? 1 : 2] = {}; f(x, selector); // ok: is a dependent expression, so captures x }; auto g3 = [=](auto a) { typeid(a + x); // captures x regardless of whether a + x is an unevaluated operand }; } | (since C++14) | If the body of a lambda odr-uses an entity captured by copy, the member of the closure type is accessed. If it is not odr-using the entity, the access is to the original object: Within the body of a lambda, any use of decltype on any variable with automatic storage duration is as if it were captured and odr-used, even though decltype itself isn't an odr-use and no actual capture takes place: Any entity captured by a lambda (implicitly or explicitly) is odr-used by the lambda-expression (therefore, implicit capture by a nested lambda triggers implicit capture in the enclosing lambda). All implicitly-captured variables must be declared within the reaching scope of the lambda. If a lambda captures the enclosing object (as this or *this ), either the nearest enclosing function must be a non-static member function or the lambda must be in a default member initializer : If a lambda expression (or an instantiation of a generic lambda's function call operator) ODR-uses this or any variable with automatic storage duration, it must be captured by the lambda expression. Class members cannot be captured explicitly by a capture without initializer (as mentioned above, only variables are permitted in the capture list): When a lambda captures a member using implicit by-copy capture, it does not make a copy of that member variable: the use of a member variable m is treated as an expression ( * this ) . m , and * this is always implicitly captured by reference: If a lambda-expression appears in a default argument , it cannot explicitly or implicitly capture anything. Members of anonymous unions cannot be captured. If a nested lambda m2 captures something that is also captured by the immediately enclosing lambda m1 , then m2 's capture is transformed as follows: - if the enclosing lambda m1 captures by copy, m2 is capturing the non-static member of m1 's closure type, not the original variable or this .
- if the enclosing lambda m1 captures by reference, m2 is capturing the original variable or this .
This example shows how to pass a lambda to a generic algorithm and how objects resulting from a lambda declaration can be stored in std::function objects. Defect reportsThe following behavior-changing defect reports were applied retroactively to previously published C++ standards. DR | Applied to | Behavior as published | Correct behavior | | C++11 | the return type of closure's operator() was only deduced if lambda body contains a single return | deduced as if for C++14 auto-returning function | | C++14 | closure had a deleted default ctor and implicit copy/move ctors | no default and defaulted copy/move | | C++14 | the conversion function for captureless lambdas had unspecified exception specification | conversion function is noexcept | | specifies a type defined by an expression (C++11) | | wraps callable object of any type with specified function call signature (class template) | Stay up-to-date with Modern C++ 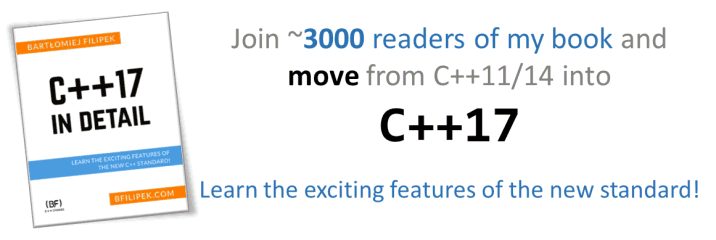 Last Update: 23 November 2020 Under the Covers of C++ Lambdas: Captures, Captures, Captures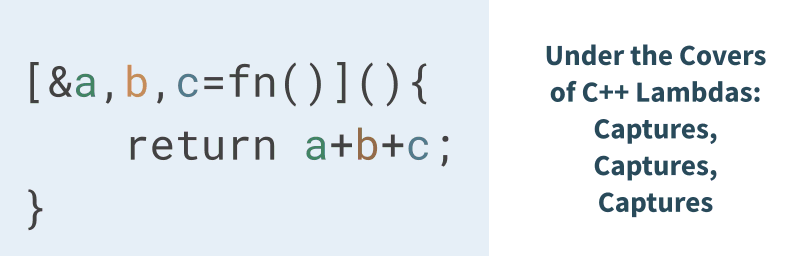 Table of Contents Lambda Capturing syntax allows us to quickly “wrap” a variable from the outside scope and then use it in the lambda body. We also know that under the hood the compiler translates lambda into a closure type… but what happens to those captured variables? Are they translated to public data members or private? See the newest guest post from Andreas to understand this tricky problem. This is a guest post by Andreas Fertig : Andreas is a trainer and consultant for C++ specializing in embedded systems. He has a passion for teaching people how C++ works, which is why he created C++ Insights ( cppinsights.io ). You can find Andres online at AndreasFertig.info and on Twitter , GitHub , or LinkedIn . Originally published at Andreas blog Capturing variables or objects is the probably most compelling thing about lambdas. A few weeks ago, Bartłomiej Filipek approached me with the example below, which also led to a C++ Insights issue (see issue #347 ). It was initially raised to Bartek by Dawid Pilarski during the review of Bartek’s C++ Lambda Story book. The code C++ Insights created for it was the following (yes, the past tense is intentional here): Bartek’s observation was that the way C++ Insights shows the transformation, we get more copies than we should and want. Look at the constructor of __lambda_5_12 . It takes an std::string object by copy. Then in the class-initializer list, _str is copied into str . That makes two copies. As a mental model, once again, think str being an expensive type. Bartek also checked what compilers do with a hand-crafted struct that leaves a bread-crumb for each special-member function called. I assume you are not surprised, but with real lambdas, there is no additional copy. So how does the compiler do this? First, let’s see what the Standard says. N4861 [expr.prim.lambda.closure] p1 says the closure type is a class type. Then in p2 The closure type is not an aggregate type. Now, one thing that (I think is key) is the definition of aggregate [dcl.init.aggr] p1.2 no private or protected direct non-static data members This is to my reading some kind of double negation. As the closure type is a class but not an aggregate, the data members must be private. All the other restrictions for aggregates are met anyway. Then back in [expr.prim.lambda.closure], p3 The closure type for a lambda-expression has a public inline function call operator… Here public is explicitly mentioned. I read it that we use class rather than struct to define the closure type. What does the Standard say about captures? The relevant part for this discussion is [expr.prim.lambda.capture] p15: When the lambda-expression is evaluated, the entities that are captured by copy are used to direct-initialize each corresponding non-static data member of the resulting closure object The data members are direct-initialized ! Remember, we have a class , and the data members are private . Captures Fact Check The AST C++ Insights uses from Clang says that the closure type is defined with class . It also says that the data members are private. So far, the interpretation of the Standard seems fine. I don’t tweak or interfere at this point. But, Clang doesn’t provide a constructor for the closure type! This is the part that C++ Insights makes up. This is why it can be wrong. And this is why the C++ Insights transformation was wrong for Bartek’s initial example. But wait, the data members are private , and there is no constructor. How are they initialized? Especially with direct-init? Do capturing lambdas have a constructor?I discussed this with Jason about this; I think at last year’s code::dive. He also pointed out that C++ Insights shows a constructor while it is unclear whether there really is one. [expr.prim.lambda.closure] p13 says the following: The closure type associated with a lambda-expression has no default constructor if the lambda-expression has a lambda-capture and a defaulted default constructor otherwise. It has a defaulted copy constructor and a defaulted move constructor (11.4.4.2). It has a deleted copy assignment operator if the lambda-expression has a lambda-capture and defaulted copy and move assignment operators otherwise… There is no explicit mention of a constructor to initialize the data members. But even with a constructor, we cannot get direct-init. How does it work efficiently? Suppose we have a class with a private data member. In that case, we can get direct-init behavior by using in-class member initialization (or default member initializer as it is called in the Standard). Here we define a variable in an outer scope #A and use it later #B to initialize a private member of Closure . That works, but note that inside Closure , it is _x now. We cannot use the same name for the data member as the one from the outer scope. The data member would shadow the outer definition and initialize it with itself. For C++ Insights, I cannot show it that way if I don’t replace all captures in the call operator with a prefixed or suffixed version. Once again, we are in compiler-land. Here is my view. All the restrictions like private and a constructor are just firewalls between C++ developers and the compiler. It is an API if you like. Whatever the compiler internally does is up to the compiler, as long as it is as specified by the Standard. Roughly Clang does exactly what we as users are not allowed to do, it to some extend, uses in-class member initialization. In the case of a lambda, the compiler creates the closure type for us. Variables names are only important to the compiler while parsing our code. After that, the compiler thinks and works with the AST. Names are less important in that representation. What the compiler has to do, is to remember that the closure type’s x was initialized with an outer scope x . Believe me, that is a power the compiler has. C++ Insights and lambda’s constructorsThanks to Bartek’s idea, the constructors of lambdas take their arguments by const reference now. This helps in most cases to make the code behave close to what the compiler does. However, when a variable is moved into a lambda, the C++ Insights version is still slightly less efficient than what the compiler generates. Here is an example: If you run this on your command-line or in Compiler Explorer , you get the following output: This is the transformed version from C++ Insights: Here is the output which you can see on Compiler Explorer : Notice the second move-ctor ? This is because it is still no direct-init. I need a second move in the lambda’s constructor to keep the move’ness. The compiler still beats me (or C++ Insights). What’s next On his blog Andreas also has other posts where he goes under the hood and explains how the compiler translates lambda expressions. See here: Andreas Fertig Blog Support C++ Insights Have fun with C++ Insights. You can support the project by becoming a Patreon or, of course, with code contributions . I've prepared a valuable bonus if you're interested in Modern C++! Learn all major features of recent C++ Standards! Check it out here: Similar Articles:- How to Pass a Variadic Pack as the First Argument of a Function in C++
- Lambda Week: Tricks
- Lambda Week: Going Generic
- Lambda Week: Capturing Things
- Lambda Week: Syntax changes, C++11 to C++20
Lucentbeing.com » Nested Lambdas and Move Capture in C++14Nested lambdas and move capture in c++14. Anonymous functions, or lambdas , were introduced in C++11 as a convenient, lightweight syntax for creating one-off functions. What excited me most about this development was that I could now compile a functional language into C++ by constructing a more faithful embedding of higher-order functions, without requiring me to deal with issues like closures and lifting. It wasn’t perfect though, and there were a number of warts that made lambdas unusable for anything other than the simplest cases. In this post I’m going to motivate C++14’s initialized lambda capture as a solution to one of the numerous problems hitting one of my most common use-cases: nested lambdas. Take #1: Pass by Value, Capture by ValueConsider a simple haskell-esque function: This translates into a correspondingly simple C++11 lambda as follows: On the face of it, this doesn’t look so bad – an outer lambda which takes an argument and returns an inner lambda which takes its own argument and while capturing the outer argument. This function can be called as add(5)(2) , in the usual curried form. On closer inspection, things can get less than pretty. Specifically, see if you can spot the number of times the value x is actually copied before the addition is performed. Since add takes x by value, that’s potentially where the first copy happens. x is then copied again, when it is captured in the inner lambda. That’s two copies, when at worst we should only have one (the outer one), and at best none at all. There are several methods to eliminate some or all of these copies, methods that are available in C++11. They all have some drawbacks, making you forfeit either convenience, semantics or safety. Let’s take a look at a few of these. Take #2: Pass by Value, Capture by ReferenceThe obvious way to eliminate the capture copy is to capture by reference, instead of by value. After all, that’s what it’s there for. This leads to issues however, because we’re allowing a reference to a stack-allocated value escape its scope. Here’s the same example, capturing x by reference: While x is no longer copied at the capture point, this opens us up to a whole host of unforeseen behaviors. Since x is stack allocated, it technically doesn’t exist after add returns. This means that any piece of code that can conceivably call the inner lambda is already dealing with a reference to a memory location that doesn’t necessarily contain the value it did when the lambda was created. At best you’ll get the wrong answer immediately; at worst you’ll get it sometime later where you can no longer correlate the error and the cause. Take #3: Pass by Reference, Capture by ReferenceWhat if x were guaranteed to exist after add returned? This would solve the inner lambda’s problems, but the only way to accomplish this to force add to accept its own argument by reference. Combined with reference capture, this eliminates both copies. This is not however without its own problems. The semantic issue from the previous solution still exists, since we are still capturing in the inner lambda by reference. A more obvious problem is that we can no longer call add with r-value arguments, such as temporaries and literals. For example, we can’t call add(5)(2) , as 5 isn’t an l-value, and cannot therefore be passed by reference. We’re forced to do one of two things: declare the argument ahead of time ( int x = 5; add(x)(2) ), or use constant references. Take #4: Pass by Constant Reference, Capture by ReferenceAccepting an argument by constant reference allows us to pass in both l-values and r-values – the compiler extends the lifetime of an r-value by just long enough that things work out. Our add function now looks like: Which is great – no copies anywhere, and we can call the function however we’d like. Semantic issues still abound, but apart from the fact that the program might not do what we want it to do, we’re fine. But we’re not done yet. Suppose we wanted to change the definition of add just a little bit – increment x before adding it. This is a contrived example, but it’s not that difficult to imagine a function where you’d like to modify your arguments. But this can’t work! We’ve already declared x to be passed in by constant reference, which means we can’t change it. What we really want is for x to be passed in by value. At this point, we realize that we’re precisely back at square one, and throw up our hands. At least that’s how it was before initialized lambda capture in C++14. Take #5: Pass by Value, Capture by MoveInitialized lambda capture gives us access to the [id = expression] syntax in the capture specifier list, allowing us to initialize the captured variables however we want. The utility in our recurrent example is in the realization that x isn’t used in the outer function after the return statement (for obvious reasons), so it can technically be moved into the returned lambda. By moving x into the lambda, we prevent the capture copy, while permitting x to be modified in the outer function (and indeed, in the inner function as well). Additionally, by accepting x by value in the outer function, we permit the compiler to infer moves there as well. This will result in exactly as many copies as are necessary in order to get the desired behaviour. This technique exhibits varying degrees of success for different data-types; primitive types such as int and float arguably don’t benefit that much, since a move is about as expensive as a copy. More complex types with heap-allocated resources such as std::vector might take arbitrarily long to copy, making a move quite appealing. Lastly, for types which can only be moved and not copied – such as std::unique_ptr , this is the only way to capture them. Shahar Mike's Web SpotYet another geek's blog Under the hood of lambdas and std::functionTable of Contents In this post we’ll explore how lambdas behave in different aspects. Then we’ll look into std::function and how it works. What’s a lambda?Here’s a quick recap if you have yet to use one of the most powerful features of C++11 – lambdas: Lambdas are a fancy name for anonymous functions. Essentially they are an easy way to write functions (such as callbacks) in the logical place they should be in the code. My favorite expression in C++ is [](){}(); , which declares an empty lambda and immediately executes it. It is of course completely useless. Better examples are with STL, like: This has the following advantages over C++98 alternatives: it is where the code would logically be (as opposed to defining a class/function somewhere outside this scope), and it does not pollute any namespace (although this could be easily be bypassed even in C++98). Lambdas have 3 parts: - Capture list – these are variables that are copied inside the lambda to be used in the code;
- Argument list – these are the arguments that are passed to the lambda at execution time;
- Code – well.. code.
Here’s a simple example: - First line is simple - create 2 int s named i and j .
- Captures i by value, j by reference,
- Accepts 2 parameters: bool b and float f ,
- Prints b and f when invoked
- Third line calls this lambda with true and 1.0f
I find it useful to think of lambdas as classes : - The data members for f above are i and j ;
- The lambda can access these members inside it’s code scope.
- When a lambda is created, a constructor copies the captured variables to the data members;
- It has an operator()(...) (for f the ... is bool, float );
- It has a scope-lifetime and a destructor which frees members.
One last thing syntax-wise: you can also specify a default capture: - [&](){ i = 0; j = 0; } is a lambda that captures i and j by reference. [&] means ‘capture by-reference all variables that are in use in the function’
- [=](){ cout << k; } is a lambda that captures k by value. Similarly, [=] means ‘capture by-value all variables that are in use in the function’
- You can also mix and match: [&, i, j](){} captures all variables by reference except for i and j which are captures by value. And of-course the opposite is also possible: [=, &i, &j](){} .
Capture by value vs by referenceAbove we mentioned capturing a lambda by value vs by reference. What’s the difference? Here’s a simple code that will illustrate: Lambda’s typeOne important thing to note is that a lambda is not a std::function . It is true that a lambda can be assigned to a std::function , but that is not its native type. We’ll talk about what that means soon. As a matter of fact, there is no standard type for lambdas. A lambda’s type is implementation defined, and the only way to capture a lambda with no conversion is by using auto : However, if your capture list is empty you may convert your lambda to a C-style function pointer: Lambda’s scopeAll captured variables have the scope of the lambda: mutable lambdaslambda’s operator() is const by-default, meaning it can’t modify the variables it captured by-value (which are analogous to class members). To change this default add mutable : This gets even more interesting when talking about copying lambdas. Key thing to remember - they behave like classes: Lambda’s sizeBecause lambdas have captures, there’s no single size for all lambdas. Example: Output (64-bit build): PerformanceLambdas are also awesome when it comes to performance. Because they are objects rather than pointers they can be inlined very easily by the compiler, much like functors. This means that calling a lambda many times (such as with std::sort or std::copy_if ) is much better than using a global function. This is one example of where C++ is actually faster than C. std::functionstd::function is a templated object that is used to store and call any callable type, such as functions, objects, lambdas and the result of std::bind . Simple exampleStd::function ’s size. On clang++ the size of all std::function s (regardless of return value or parameters) is always 32 bytes. It uses what is called small size optimization , much like std::string does on many implementations. This basically means that for small objects std::function can keep them as part of its memory, but for bigger objects it defers to dynamic memory allocation. Here’s an example on a 64-bit machine: 17. That’s the threshold beyond which std::function reverts to dynamic allocation (on clang). Note that the allocation is for the size of 17 bytes as the lambda object needs to be contiguous in memory. That’s it for my first post. I hope you enjoyed reading it as much as I enjoyed writing it. Please let me know if you have any suggestions, questions or comments!  More Lambda Features with C++20Lambdas in C++20 can be default-constructed and support copy-assignment when they have no state. Lambdas can be used in unevaluated contexts. Additionally, they detect when you implicitly copy the this pointer. This means a significant cause of undefined behavior with lambdas is gone. 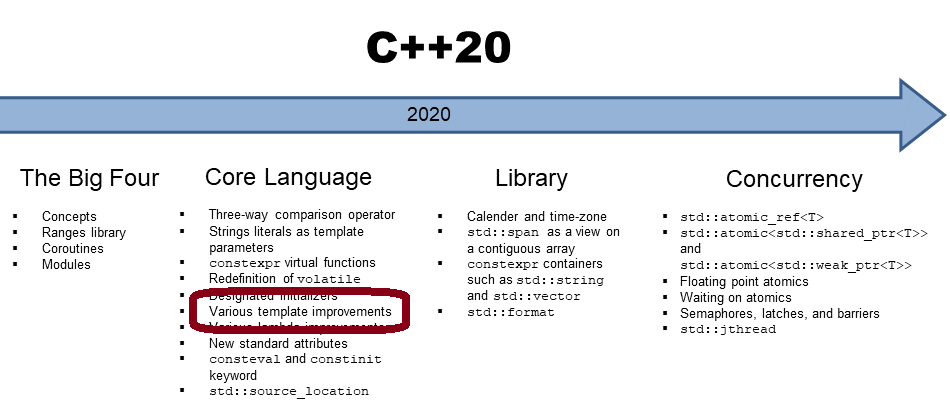 I want to start with the last feature of the enumeration. The compiler detects the undefined behavior when you implicitly copy the this pointer. Okay, what does undefined behavior mean? With undefined behavior, there are no restrictions on the behavior of the program, and you have, therefore, no guarantee of what can happen. I like to say in my seminars: When you have undefined behavior, your program has catch-fire semantics. This means your computer can even catch fire. In former days undefined behavior was described more rigorously: with undefined behavior, you can launch a cruise missile. Anyway, when you have undefined behavior, there is only one action left: fix the undefined behavior. In the next section, I intentionally cause undefined behavior. Implicitly Copy of the this PointerThe following program implicitly captures the this pointer by copy. The compilation of the program works as expected, but this does not hold for the execution of the program. Modernes C++ Mentoring- " Fundamentals for C++ Professionals " (open)
- " Design Patterns and Architectural Patterns with C++ " (open)
- " C++20: Get the Details " (open)
- " Concurrency with Modern C++ " (open)
- "Generic Programming (Templates) with C++": October 2024
- "Embedded Programming with Modern C++": October 2024
- "Clean Code: Best Practices for Modern C++": March 2025
Do you want to stay informed: Subscribe. 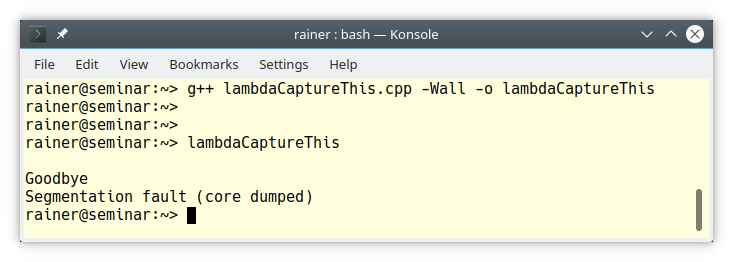 Do you spot the issue in th e program lambdaCaptureThis.cpp ? The member function foo (1) returns the lambda [=] { std::cout << s << std::endl; } having an implicit copy of the this pointer. This implicit copy is no issue in (2) but becomes an issue at the end of the scope. The end of the scope means the end of the lifetime of the local lambda (3). Consequently, the call lam() (4) triggers undefined behavior. A C++20 compiler must, in this case, write a warning. Here is the output with the Compiler Explorer and GCC.  The two missing lambdas features of C++20 sound not so thrilling: Lambdas in C++20 can be default-constructed and support copy-assignment when they have no state. Lambdas can be used in unevaluated contexts. Before I present both features together, I have to make a detour: What does unevaluated context mean? Unevaluated ContextThe following code snippet has a function declaration and a function definition. add1 declares a function, but add2 defines it. This means if you use add1 in an evaluated context, such as invoking it, you get a link-time error. The critical observation is that you can use add1 in unevaluated contexts such as typeid , or decltype . Both operators accept unevaluated operands. typeid(add1).name() (1) returns a string representation of the type, and decltype (2) deduces the type of its argument. 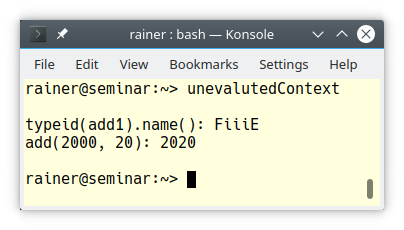 Stateless Lambdas can be default-constructed and copy-assignedLambdas can be used in unevaluated contexts. Admittedly, this is quite a long title. Maybe the term stateless lambda is new to you. A stateless lambda is a lambda that captures nothing from its environment. Or, to put it the other way around. A stateless lambda is a lambda where the initial brackets [] in the lambda definition are empty. For example, the lambda expression auto add = [ ](int a, int b) { return a + b; }; is stateless. When you combine the features, you get lambdas, which are pretty handy. Before I show you the example, I must add a few remarks. std::set such as all other ordered associative containers from the standard template library ( std::map, std::multiset , and std::multimap ) use per-default std::less to sort the keys. std::less guarantees that all keys are ordered lexicographically in ascending order. The declaration of std::set on cppreference.com shows you this ordering behavior. Now, let me play with the ordering in the following example. set1 and set4 sort their keys in ascending order. set2 , set3 , and set5 uniquely use a lambda in an unevaluated context. The using keyword (1) declares a type alias, which is used in the following line (2) to define the sets. Creating the set causes the call of the default constructor of the stateless lambda. Thanks to the Compiler Explorer and GCC, here is the program’s output. 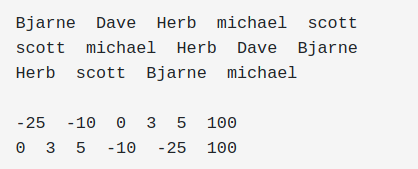 When you study the output of the program, you may be surprised. The special set3, which uses the lambda [](const auto& l, const auto& r){ return l.size() < r.size(); } as a predicate, ignores the name “ Dave”. The reason is simple. “ Dave ” has the same size as “ Herb “, which was added first. std::set supports unique keys, and the keys are identical using the special predicate. If I had used std::multiset, this wouldn’t have happened. Standard Seminars (English/German)Here is a compilation of my standard seminars. These seminars are only meant to give you a first orientation. - C++ – The Core Language
- C++ – The Standard Library
- C++ – Compact
- C++11 and C++14
- Concurrency with Modern C++
- Design Pattern and Architectural Pattern with C++
- Embedded Programming with Modern C++
- Generic Programming (Templates) with C++
- Clean Code with Modern C++
Online Seminars (German)- Embedded Programmierung mit modernem C++ (24. Sep. 2024 bis 26. Sep. 2024)
- Mobil: +49 176 5506 5086
- Mail: [email protected]
- German Seminar Page: www.ModernesCpp.de
- Mentoring Page: www.ModernesCpp.org
Modernes C++ Mentoring, 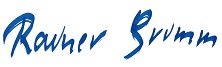 You might also like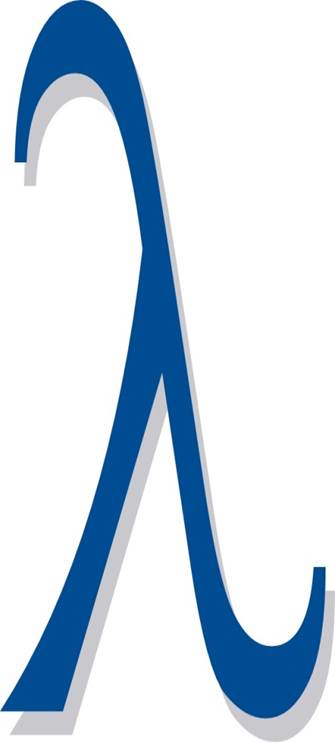 Leave a ReplyLeave a reply cancel reply. Your email address will not be published. Required fields are marked * 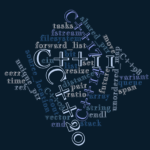 - Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. Capturing lambda and move assignableI am confused by why a capturing lambda is not move assignable, but its manual definition (as struct with operator()) is. Consider the following simplified code: The class LambdaCPPInsights is the translated (and then renamed) lambda struct as given by cpp insights, should therefore be the same thing as the lambda defined by [env = env]() {} . However, the output of the previous code under gcc 11.2 and c++20 is: The example on godbolt is found here: https://godbolt.org/z/Yx3n9eGj5 What am I missing? - variable-assignment
- move-semantics
 A lambda with a capture has a deleted copy assignment operator, which also implies that it has no implicit move assignment operator. See [expr.prim.lambda.closure]/13 . Your LambdaCPPInsights is not correctly reproducing the closure type. It should explicitly default the copy and move constructors, and explicitly delete the copy assignment operator. Your AnswerReminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more Sign up or log inPost as a guest. Required, but never shown By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy . Not the answer you're looking for? Browse other questions tagged c++ lambda variable-assignment c++20 move-semantics or ask your own question .- The Overflow Blog
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions- How much time would an astronaut experience accelerating to .9999x the speed of light at an acceleration of 1G from the astronauts perspective?
- Disable terminal switch focus shortcut (Cmd+Left/Right Arrow)
- How is an inverting opamp adder circuit able to regulate its feedback?
- If you switch to a non-orthogonal basis, are vectors that were previously orthogonal still orthogonal?
- Coding exercise to represent an integer as words using python
- Explain how π – 1 + 50 + ⅔ × 1000 is PLONK
- What does ציר"ו stand for?
- Why does Jenny hug Cindy at the end of "Commando" (1985)?
- Which version of Netscape, on which OS, appears in the movie “Cut” (2000)?
- Does an airplane fly less or more efficiently after an mid-flight engine failure?
- Should you refactor when there are no tests?
- How many ways can you make change?
- How to frame certain cells with tabular?
- Doesn't counting hole and electron current lead to double-counting of actual current?
- Does it pay to put effort in fixing this problem or better reinstall Ubuntu 24.04 from scratch?
- Is a company liable for "potential" harms?
- Who owns code contributed to a license-free repository?
- Lore reasons for being faithless
- Integration by parts for twice differentiable functions
- What happens to entropy during compression?
- How to prepare a superposed state?
- Does measuring a quantum object collapse the wave function even if the particle is not found in the position where it was measured?
- Is it illegal to use a fake state ID to enter a private establishment even when a legitimate ID would've been fine?
- Functor composition rule necessary?
 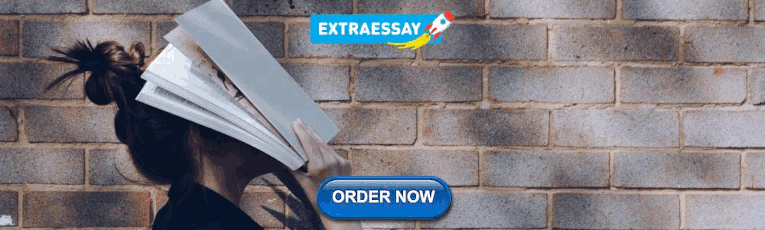 |
IMAGES
VIDEO
COMMENTS
In C++14, we have generalized lambda capture that solves not only that problem, but allows you to define arbitrary new local variables in the lambda object. For example: auto u = make_unique<some_type>( some, parameters ); // a unique_ptr is move-only. go.run( [ u=move(u) ] { do_something_with( u ); } ); //move the unique_ptr into the lambda ...
The capture clause. The capture clause is used to (indirectly) give a lambda access to variables available in the surrounding scope that it normally would not have access to. All we need to do is list the entities we want to access from within the lambda as part of the capture clause.
captures - A comma-separated list of zero or more captures, optionally beginning with a capture-default.. See below for the detailed description of captures.. A lambda expression can use a variable without capturing it if the variable is a non-local variable or has static or thread local storage duration (in which case the variable cannot be captured), or
The generalized lambda capture is much more general in the sense that captured variables can be initialized with anything like so: auto lambda = [value = 0] mutable { return ++value; }; In C++11 this is not possible yet, but with some tricks that involve helper types. Fortunately, the Clang 3.4 compiler already implements this awesome feature.
Here, capture clauses are defined inside the square brackets []. Capture Clauses in lambda expressions determine how the external variables are accessed from the lambda expression. The capture clauses allow the lambda function to capture external variables and use them inside the lambda body. There are three ways to capture external variables ...
Following is the syntax for lambda expressions with capture clauses: Syntax [capture-list] (parameters) -> return-type {// lambda body} There are mainly two types of capture clauses in lambda expressions : Capturing a Variable by Value : A copy of a variable is captured at the time when the lambda is being created. Any particular changes made ...
It has a defaulted copy constructor and a defaulted move constructor (11.4.4.2). It has a deleted copy assignment operator if the lambda-expression has a lambda-capture and defaulted copy and move assignment operators otherwise... There is no explicit mention of a constructor to initialize the data members.
capture clause (Also known as the lambda-introducer in the C++ specification.). parameter list Optional. (Also known as the lambda declarator). mutable specification Optional.. exception-specification Optional.. trailing-return-type Optional.. lambda body.. Capture clause. A lambda can introduce new variables in its body (in C++14), and it can also access, or capture, variables from the ...
The basic syntax of a Lambda Expression in C++ is; 1. 2. 3. Datatype Lambda Expression = [Capture Clause] (Parameter List) -> Return Type { Body } Generalized lambdas can be defined with the auto keyword that comes with C++11. We can define a generic lambda with the auto keyword as below.
This syntax effectively removes the const from the call operator declaration in the closure type. If you have a simple lambda expression with a mutable: int x = 1; auto foo = [x]() mutable { ++x; }; It will be "expanded" into the following functor: struct __lambda_x1 { void operator()() { ++x; } int x; }; On the other hand, if you capture ...
If the lambda-expression captures anything by copy (either implicitly with capture clause [=] or explicitly with a capture that does not include the character &, e.g. [a, b, c]), the closure type includes unnamed non-static data members, declared in unspecified order, that hold copies of all entities that were so captured.. The type of each data member is the type of the corresponding captured ...
The capture clause of a lambda expression can't contain a variable that has a managed type. However, you can pass an argument that has a managed type to the parameter list of a lambda expression. The following example contains a lambda expression that captures the local unmanaged variable ch by value and takes a System.String object as its ...
Only lambda-expressions defined at block scope or in a default member initializer may have a capture-default or captures without initializers. For such lambda-expression, the reaching scope is defined as the set of enclosing scopes up to and including the innermost enclosing function (and its parameters). This includes nested block scopes and the scopes of enclosing lambdas if this lambda is ...
The relevant part for this discussion is [expr.prim.lambda.capture] p15: When the lambda-expression is evaluated, the entities that are captured by copy are used to direct-initialize each corresponding non-static data member of the resulting closure object ... It has a deleted copy assignment operator if the lambda-expression has a lambda ...
return [x = std::move(x)] (int y) {. return x + y; } } By moving x into the lambda, we prevent the capture copy, while permitting x to be modified in the outer function (and indeed, in the inner function as well). Additionally, by accepting x by value in the outer function, we permit the compiler to infer moves there as well.
A lambda's type is implementation defined, and the only way to capture a lambda with no conversion is by using auto: auto f2 = [](){}; However, if your capture list is empty you may convert your lambda to a C-style function pointer: void (*foo)(bool, int); foo = [](bool, int){}; Lambda's scope. All captured variables have the scope of the ...
int (*fp)(void*) = lambda_ptr<int>(b); fp(&b); UPDATE. Improved version. It was a while since first post about C++ lambda with captures as a function pointer was posted. As It was usable for me and other people I made some improvement. Standard function C pointer api uses void fn (void* data) convention.
The assignment expression operator := added in Python 3.8 supports assignment inside of lambda expressions. This operator can only appear within a parenthesized (...), bracketed [...], or braced {...} expression for syntactic reasons. For example, we will be able to write the following: import sys. say_hello = lambda: (.
August 7, 2020 / 0 Comments / in C++20 / by Rainer Grimm. Lambdas in C++20 can be default-constructed and support copy-assignment when they have no state. Lambdas can be used in unevaluated contexts. Additionally, they detect when you implicitly copy the this pointer. This means a significant cause of undefined behavior with lambdas is gone.
2. A lambda with a capture has a deleted copy assignment operator, which also implies that it has no implicit move assignment operator. See [expr.prim.lambda.closure]/13. Your LambdaCPPInsights is not correctly reproducing the closure type. It should explicitly default the copy and move constructors, and explicitly delete the copy assignment ...