Computersciencementor | Hardware, Software, Networking and programming
Algorithm and flowchart explained with examples, what is algorithm and flowchart.
Algorithm and flowchart are programming tools. A Programmer uses various programming languages to create programs. But before actually writing a program in a programming language, a programmer first needs to find a procedure for solving the problem which is known as planning the program. The program written without proper pre-planning have higher chances of errors. The tools that are used to plan or design the problem are known as programming tools. Algorithm and flowchart are widely used programming tools.
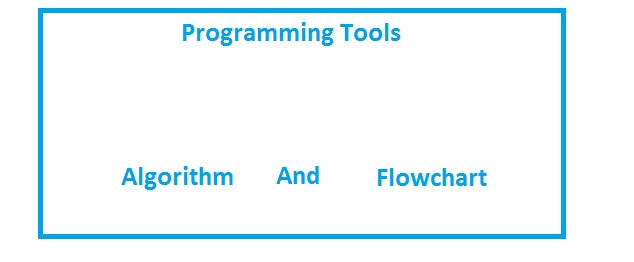
The word “algorithm” relates to the name of the mathematician Al- khowarizmi , which means a procedure or a technique. Programmer commonly uses an algorithm for planning and solving the problems.
An algorithm is a specific set of meaningful instructions written in a specific order for carrying out or solving a specific problem.
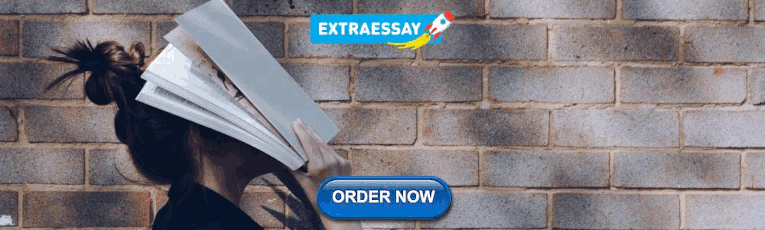
Types of Algorithm:
The algorithm and flowchart are classified into three types of control structures.
- Branching(Selection)
- Loop(Repetition)
According to the condition and requirement, these three control structures can be used.
In the sequence structure, statements are placed one after the other and the execution takes place starting from up to down.
Whereas in branch control, there is a condition and according to a condition, a decision of either TRUE or FALSE is achieved. In the case of TRUE, one of the two branches is explored; but in the case of FALSE condition, the other alternative is taken. Generally, the ‘IF-THEN’ is used to represent branch control.
Write an algorithm to find the smallest number between two numbers
Write an algorithm to check odd or even number.
The Loop or Repetition allows a statements or block of statements to be executed repeatedly based on certain loop condition. ‘While’ and ‘for’ construct are used to represent the loop structure in most programming languages. Loops are of two types: Bounded and Unbounded loop. In bounded loop, the number of iterations is fixed while in unbounded loops the condition has to satisfy to end the loop.
An algorithm to calculate even numbers between 20 and 40
Write an algorithm to input a natural number, n, and calculate the odd numbers equal or less than n.
Characteristics of a good algorithm.
- The Finite number of steps:
After starting an algorithm for any problem, it has to terminate at some point.
- Easy Modification.
There can be numbers of steps in an algorithm depending on the type of problem. It supports easy modification of Steps.
- Easy and simple to understand
A Simple English language is used while writing an algorithm. It is not dependent on any particular programming language. People without the knowledge of programming can read and understand the steps in the algorithm.
An algorithm is just a design of a program. Every program needs to display certain output after processing the input data. So one always expects the result as an output from an algorithm. It can give output at different stages. The result obtained at the end of an algorithm is known as an end result and if the result is obtained at an intermediate stage of process or operation then the result is known as an intermediate result. Also, the output has to be as expected having some relation to the inputs.
The first design of flowchart goes back to 1945 which was designed by John Von Neumann . Unlike an algorithm, Flowchart uses different symbols to design a solution to a problem. It is another commonly used programming tool.
In general, a flowchart is a diagram that uses different symbols to visually present the flow of data. By looking at a flow chart one can understand the operations and sequence of operations performed in a system. This is why flowchart is often considered as a blueprint of a design used for solving a specific problem.
A flowchart is defined as a symbolic or a graphical representation of an algorithm that uses different standard symbols.
Flowchart Symbols:
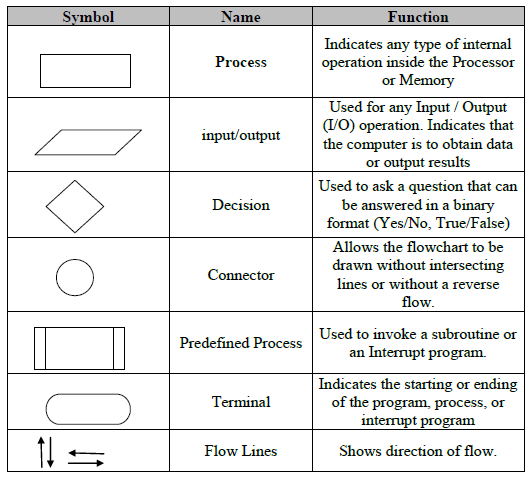
Guidelines for drawing a flowchart.
- The Title for every flowchart is compulsory.
- There must be START and END point for every flowchart.
- The symbols used in flowchart should have only one entry point on the top. The exit point for symbols (except for decision/diamond symbol) is on the button.
- There should be two exit points for decision symbol; exit points can be on the bottom and one side or on the sides.
- The flow of flowchart is generally from top to bottom. But in some cases, it can also flow to upward direction
- The direction of the flow of control should be indicated by arrowheads.
- The operations for every step should be written inside the symbol.
- The language used in flowchart should be simple so that it can be easily understood.
- The flowlines that show the direction of flow of flowchart must not cross each other.
- While connecting different pages of the same flowchart, Connectors must be used.
Some examples of algorithm and flowchart.
Example1: To calculate the area of a circle
Step1: Start
Step2: Input radius of the circle say r
Step3: Use the formula πr 2 and store result in a variable AREA
Step4: Print AREA
Step5: Stop Flowchart:
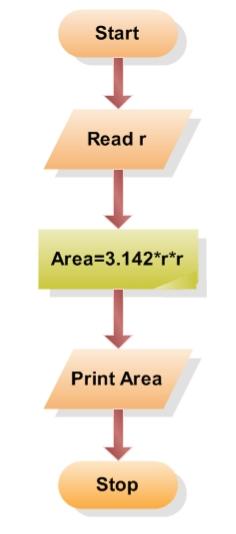
Related Posts
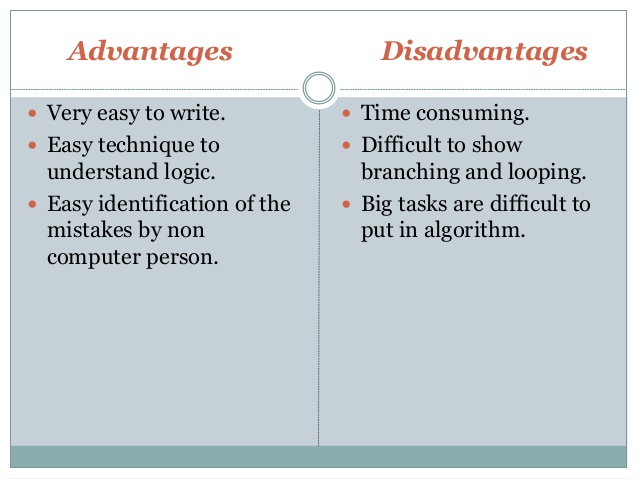
Sampurna shrestha
Save my name, email, and website in this browser for the next time I comment.
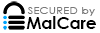
Algorithms, flowcharts, and pseudocode. ¶
Overview, objectives, and key terms ¶.
In this lesson, we’ll dive right into the basic logic needed to plan one’s program, significantly extending the process identified in Lesson 2 . We’ll examine algorithms for several applications and illustrate solutions using flowcharts and pseudocode . Along the way, we’ll see for the first time the three principal structures in programming logic: sequence , selection , and iteration . Throughout, specialized syntax (Python or otherwise) is avoided (with the exception of the handy slicing syntax introduced in Lesson 3 ), but variables and simple operators are used.
Objectives ¶
By the end of this lesson, you should be able to
- define what an algorithm is
- decompose problems into a sequence of simple steps
- illustrate those steps graphically using flowcharts
- describe those steps in words using pseudocode
Key Terms ¶
- counter variable
- index variable
- conditional statement
Algorithms ¶
An algorithm is procedure by which a problem (computational or otherwise) is solved following a certain set of rules. In many computer science departments, entire courses are dedicated to the study of algorithms important for solving a wide variety of problems. Some of the most important algorithms (including a few that we’ll be studying in part throughout the semester) are
- Gaussian elimination (for solving \(\mathbf{Ax}=\mathbf{b}\) )
- Bubble sort (for sorting an array of values)
- Quicksort (a better way to sort those same values)
- Sieve of Eratosthenes (for finding prime numbers)
- Babylonian method (for finding square roots)
- Linear search (for finding a value in an array)
- Binary search (a better way for finding that value)
- Dijkstra’s algorithm (for finding, e.g., the shortest path between two cities)
- RSA algorithm (for encrypting and decrypting messages)
Many more such algorithms are listed elsewhere .
It turns out, however, that we apply algorithms all the time in every-day life, often without realizing it. Such occasions might be as boring as choosing one’s outfit for the day based on planned activities, the weather, the general status of laundry (and how many times you think you can wear that pair of jeans), etc. Other occasions of somewhat more importance might include choosing a baby’s name (which might mean making a list, whittling it down, and then convincing your wife that Linus is the one not just because that’s the name of the Finnish-born inventor of Linux). The list goes on and on: life presents problems, and we apply logic to solve those problems.
Sequence by Example: Computing Grades ¶
Let’s consider something with which you are all familiar: a course grading scheme. As a concrete example, suppose the course in question has graded tasks in four categories, each with its own weight:
- homework - 20%
- laboratories - 10%
- quizzes - 10%
- examinations - 60%
Such a scheme is probably familiar, and you’ve probably used your intuition, knowledge of your grades, and knowledge of this sort of weighting scheme to compute a final percentage. Here, we’ll formalize that process using flowcharts and pseudocode.
To simplify matters somewhat (for now), assume that there are a fixed number of tasks in each category, and each task within a category is worth the same amount. For example, the course might have 5 homework assignments, and each of these assignments might be assessed out of ten points. Take those scores to be 10, 7, 9, 10, and 10.
You might immediately see how the computation of a homework percentage can be broken into several independent steps. For instance, we can first compute the homework score. How? Think carefully. If you were using a calculator, what would you enter? On mine, I can enter 10 followed by a + and then 7 and then another + . After every addition + , it prints out the running total. In other words, it’s automatically storing the running total.
We can be more explicit, though. Before we add any of the scores at all, we can set the total score to zero, and thereafter, we can add each of the homework scores to that total in sequence . When that’s done, we need to compute the total possible score, which is the number of tasks multiplied by the point value per task. Finally, we need to compute the percentage earned on these homework assignments, which is equal to the total homework score divided by the total number of points possible (and then multiplied by 100).
This entire process can be described using pseudocode , which is any informal, high-level (few details) language used to describe a program, algorithm, procedure, or anything else with well-defined steps. There is no official syntax for pseudocode, but usually it is much closer to regular English than computer programming languages like Python. Here is one possible pseudocode representation of the procedure just outlined for computing the homework score:
Of course, this might seem long-winded, but it is explicit . As long as the input and output processes are specified (e.g., providing a printout of grades and asking for a final value to be written on that printout), this procedure could be given to most reasonable people and executed without difficulty. For reference, the total homework score (in percent) that one should get from the individual scores above is 92.
Exercise : For each line of the pseudocode above, write the value of total_score , total_possible , and hw_percent . If a variable does not have a value at a particular line, then say it is undefined .
A flowchart of the same procedure is provided below.
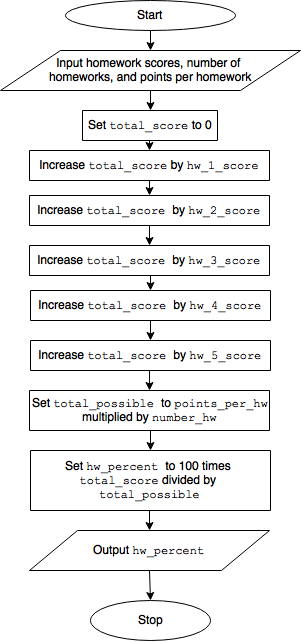
Flowchart for Computing Homework Percentage
Now, revisit the bolded word above: sequence . This particular process for computing the homework percentage is a sequence , which is the simplest of the logical structures used in programming. A sequence is nothing more than a set of instructions executed one after another. The number of these instructions and the order in which they are executed is known from the start, just like our example here (and the example seen in Lesson 2 ).
Selection ¶
The same sort of procedure can be used for all of the grading categories, and hence, let us assume now that we have available the final percentages for homework, laboratories, quizzes, and homeworks. The question now is how to assign a final letter grade based, for example, on the traditional 90/80/70/60 scheme for A/B/C/D. For this grading scheme, anything 90% or higher earns an A, anything less than 90% but greater than or equal to 80% earns a B, and so forth.
Of course, this requires that we first compute the final grade, for which the final percentages and the corresponding category weights are required. In pseudocode, that process can be done via
What we need next is to define the grade, and that’s where selection enters the game. Selection refers to a structure that allows certain statements to be executed preferentially based on some condition . Hence, selection is often implemented using conditional statements . In English, a basic conditional statement takes the form If some condition is satisfied, then do something . More complicated statements can be formed, but all conditional statements can be rewritten using one or more of these simple statements.
A basic conditional statement can be represented graphically as shown below.
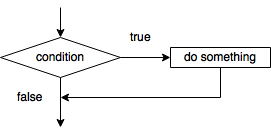
Flowchart fragment for a simple conditional statement
Armed with conditional statements for selection, we can tackle the final grade. Given the final percentage, we check whether that value is greater than 90. If so, the grade assigned is an A. If not, we can further check whether that percentage is greater than or equal to 80 and less than 90. If so, a B. If not, we check against 70 and then 60 for a C and D, respectively. If the percentage has not earned a D by the time we’ve checked, our poor student’s grade, of course, is an F.
This procedure, much like those described previously, can be described compactly in pseudocode:
As a flowchart, this same procedure is shown below.
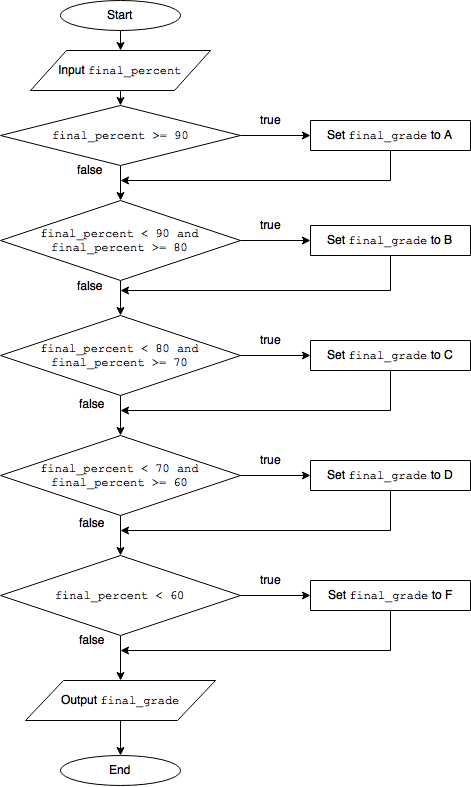
Flowchart for computing the final grade
Iteration ¶
Suppose that the homework grades analyzed above are subjected to a slight policy change: the lowest score is dropped. Such a policy is pretty common. How do we tackle this problem? It requires that we make a decision when adding a particular homework score to the total. In particularly, we skip adding the lowest score.
Exercise : Think back to the scores (i.e., 10, 7, 9, 10, and 10). Now, pick out the lowest score and, importantly , write down how you came to that conclusion. Seriously—think about what you needed to do to choose the lowest number and get it down in words.
When I first glance at the numbers, I see that there are some 10’s and other numbers, and scanning left to right, I pick out 7 as being the lowest of them. Then, keeping track of 7 (subconsciously, in this case), I scan the list and confirm that 7 is, in fact, the lowest score. I’ll bet you do something similar, but computers don’t have eyes (or a subconscience), and we therefore need to define this a bit more carefully.
The Basic Idea ¶
To start, let’s assume that the individual homework grades are stored in an array (e.g., a NumPy ndarray ) rather than as separate values. Call this array hw_scores so that we use, e.g., hw_scores[0] and hw_scores[1] instead of hw_1_score and hw_2_score . From this array, we need to pick out which element represents the score to be dropped. Therefore, we need language to describe the process of iterating through the array elements, i.e., iteration . In English, we might say something like “for each score in the array, do something.” More generically, iteration consists of doing that something until some condition is no longer satisfied, as illustrated in the flowchart fragment below:
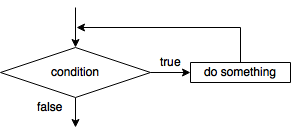
Flowchart fragment for a simple loop
Notice that this flowchart bears a striking similarity to the one shown above for the conditional statement. The difference, however, is that the flow of the program is redirected to before the condition block. In other words, a repeating loop is produced that is broken only if the condition is no longer because of changes made inside the “do something” block. The pseudocode fragment for this same loop is
Let’s apply this to a simple but illustrative problem before moving on to our homework application. Suppose we want to print every value in an array a of length n . A canonical approach to this sort of problem starts by defining an index or counter variable (often named i ) that is used to access the i th element of the array during the i th time through the loop. After the i th element has been used, the counter is increased by one, and the loop begins again. Once the counter is equal to the number of elements in the array, the loop is terminated. Here’s the complete algorithm in pseudocode
and as a flowchart
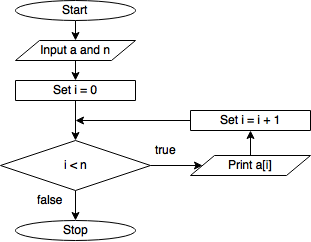
Flowchart for printing the elements of an array
Back to Homework ¶
For our homework example, the condition is “have all the scores been considered?”, while the “something” to be done is checking whether or not a given score is lower than the others. The counter-based approach to iteration developed above can be used to go through each element of the homework score array, thereby ensuring that all the elements are considered. We could compare the i th grade to every other grade (implying an iteration within an iteration), but there is a simpler way. First, we can set the lowest score equal to the first grade (i.e., hw_grades[0] ). Then, we can go through all the other scores and compare each to the lowest score. If a score is lower than the current value of lowest score, that score becomes the lowest score. Along the way, we can also keep track of the index (location) of the lowest score within the array. Here’s the idea in pseudocode:
Here it is also as a flow chart:
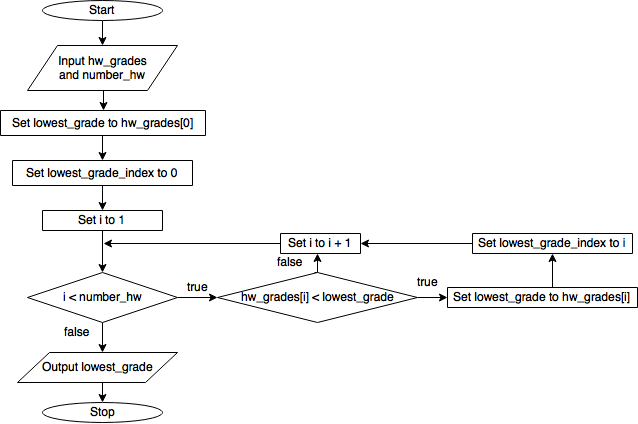
Flowchart for finding the lowest grade
Putting It All Together ¶
By now, we have all the pieces needed to compute the total homework percentage when the lowest score is dropped. Here it is, all together, in pseudocode:
Practice Problems ¶
Planning out the logic needed to solve problems can be tricky, and most students require lots of practice. Each of the topics discussed above will be the focus of the next several lessons as we dive into their implementation in Python. However, now is the time to start applying the sequence, selection, and iteration constructs, and listed below are several great practice problems to help get you started.
Develop an algorithm for each of the following tasks using both pseudocode and a flowchart . Note, many of these tasks represent great practice problems for Python programs, too. Of course, if you plan out the logic first using the tools employed in this lesson, the implementation of your solution in Python later on should be much easier!
- Prepare a pot of coffee.
- Prepare a cup of coffee for your friend. (Cream or sugar?)
- String a guitar.
- Choose your outfit (making sure to account for the day of the week and the weather).
- Determine the largest number in an array.
- Determine the second largest number in an array.
- Determine whether an integer is even.
- Determine whether an integer is prime .
- Determine whether a given year is a leap year .
- Determine the next leap year after a given year.
- Divide one number by another number using long division
- Determine the sum of an array of numbers.
- Determine the sum of the elements of an array of numbers that are divisible by 3 and 5.
- Given any two integers n and d , determine whether the quotient n/d leads to a finite decimal number (e.g., \(5/4 = 1.25\) ) or an infinite decimal number (e.g., \(1/3 = 0.3333\ldots = 0.\bar{3}\) ).
- Extend the homework grade algorithm to drop the two lowest scores.
- Determine a final grade in ME 400 following the course grading policies (including drops).
Further Reading ¶
Here is a nice example of flow chart construction for the game of hangman.
Table Of Contents
- Sequence by Example: Computing Grades
- The Basic Idea
- Back to Homework
- Putting It All Together
- Practice Problems
- Further Reading
Related Topics
- Previous: More on NumPy Arrays: Slicing and np.linalg
- Next: Lecture 6 - Conditional Statements and the Structure of Python Code
- Show Source
Quick search
Pseudocode and Flowcharts
Software development is complex and usually involves many parties working together. Therefore, planning out a project before beginning to program is essential for success.
In this article, we will take a real-world problem and attempt to design an algorithm step by step to best solve it using pseudocode and flowcharts.
Password validator
The problem.
Passwords are everywhere, and we create them all the time to access a great array of services. However, it can sometimes be helpful to guide users to make stronger passwords. This can be done by imposing some restrictions on what passwords are considered valid.
If we want to set a couple of restrictions, such as that the password must be at least 8 characters and contain a number, then the following passwords would be valid:
- supers3cure
- meandmy2dogs
But these would not:
We have all seen plenty of passwords like these, so let’s come up with a simple algorithm to validate passwords like this!
The solution
First, let’s take this problem and brainstorm some steps to validate passwords that are at least 8 characters long and also contain a number:
Input the password that we plan to validate.
To keep track of the password length, establish a pass_length variable and set it to 0 .
To keep track of whether the password contains a number, establish a contains_number variable and initially set it to False .
Has the entire password been searched?
If not, continue to step 5.
If so, skip to step 8.
Iterate, or move to, to the next character in password .
Increase the value of pass_length by 1 .
Is the current character a number?
If not, go straight back to step 4 and continue to iterate over the entire password .
If so, set the contains_number variable to True and then go back to step 4.
Is the pass_length greater than 8 and is contain_number equal to True ?
If not, then the password is invalid.
If so, then the password is valid!
Doodling a flowchart
Now that we have a framework for the task that needs to be completed, we can get to formalizing the solution. As a picture is worth a thousand words, a nice doodle can be a helpful way to communicate a complex idea — and in software development, the professional form of doodling is the flowchart !
Common flowchart symbols
Flowcharts have some standard symbols that allow them to be read and understood by a wider group of people. These are some of the most commonly-used symbols:
The terminal is an oval that indicates the beginning and end of a program. It usually contains the words Start or End .
The flowline is a line from one symbol pointing towards another to show the process’s order of operation. This displays the flow of execution in a program.
Input/Output
Input/output is represented by a rhomboid and indicates the input or output of data. This is similar to setting a value to a variable.
A process, represented by a rectangle, is an operation that manipulates data. Think of this as changing the value of a number-based variable using an operator such as + .
Decisions are represented by a rhombus and show a conditional operation that will determine which path a program should take. This is similar to conditional statements which control the flow of execution in a program.
Converting steps into symbols
Ok! Now that we have all of the steps for the algorithm figured out, let’s pair them with the relevant flowchart symbol:
INPUT/OUTPUT : Input the password that we plan to validate.
PROCESS : To keep track of the password length, establish a pass_length variable and initially set it to 0 .
PROCESS : To keep track of whether the password contains a number, establish a contains_number variable and initially set it to False .
DECISION : Has the entire password been searched?
FLOWLINE : If not, continue to step 5.
FLOWLINE : If so, skip to step 8.
PROCESS : Iterate to the next character in password .
PROCESS : Increment pass_length .
DECISION : Is the current character a number?
FLOWLINE : If not, go straight back to step 4 and continue to iterate over the entire password .
PROCESS/FLOWLINE : If so, set the contains_number variable to True and then go back to step 4.
DECISION : Is the pass_length greater than 8 and is contain_number equal to True ?
TERMINAL : If not, then the password is invalid.
TERMINAL : If so, then the password is valid!
Drawing the flowchart
Whew. Now that every step is associated with a symbol, we can connect them all together to put the flow into the chart!
Luckily, most steps just happen one after another, so the final product is relatively straightforward. However, do note how the iteration of the password requires the flowlines to physically loop in the flowchart:
Progressing with pseudocode
Now that we have the entire algorithm thought out and in visual form, we can take steps to turn it into code. Some people may be able to jump right into a development environment and start hacking away, but let’s take it slow and create some pseudocode first.
- Pseudocode is a description of an algorithm using everyday wording, but molded to appear similar to a simplified programming language.
To create pseudocode from what we have so far we can use the flowchart’s flowlines to guide the structure of our code as we simplify the steps we outlined earlier:
The final code
Now the closing moments! With pseudocode in hand, the algorithm can be programmed in any language. Here it is in Python :
Even if this code seems foreign, the power of flowcharts and pseudocode shines through. It allows people, regardless of technical expertise, to communicate algorithms and other technical solutions. These ideas can then be implemented in whatever technologies work best, and the notes can be kept around in case the algorithm needs to be reimplemented in different technologies in the future.
Wrapping up
Awesome job on making it to the end of this article! While this was mainly a practical article, here is what we learned:
- In code-based flowcharts, common ANSI shapes are ovals for terminals, arrows for flowlines, rhomboids for inputs and outputs, rhombuses for decisions, and rectangles for processes.
The Codecademy Team, composed of experienced educators and tech experts, is dedicated to making tech skills accessible to all. We empower learners worldwide with expert-reviewed content that develops and enhances the technical skills needed to advance and succeed in their careers.
Related articles
Password attacks, setting up postman, learn more on codecademy, introduction to it, code foundations.
Popular Tutorials
Popular examples, learn python interactively, related articles.
- self in Python, Demystified
- Increment ++ and Decrement -- Operator as Prefix and Postfix
- Interpreter Vs Compiler : Differences Between Interpreter and Compiler
- Algorithm in Programming
Flowchart In Programming
A flowchart is a diagrammatic representation of an algorithm. A flowchart can be helpful for both writing programs and explaining the program to others.
Symbols Used In Flowchart
Symbol | Purpose | Description |
---|---|---|
Flow line | Indicates the flow of logic by connecting symbols. | |
Terminal(Stop/Start) | Represents the start and the end of a flowchart. | |
Input/Output | Used for input and output operation. | |
Processing | Used for arithmetic operations and data-manipulations. | |
Decision | Used for decision making between two or more alternatives. | |
On-page Connector | Used to join different flowline | |
Off-page Connector | Used to connect the flowchart portion on a different page. | |
Predefined Process/Function | Represents a group of statements performing one processing task. |
Examples of flowcharts in programming
1. Add two numbers entered by the user.
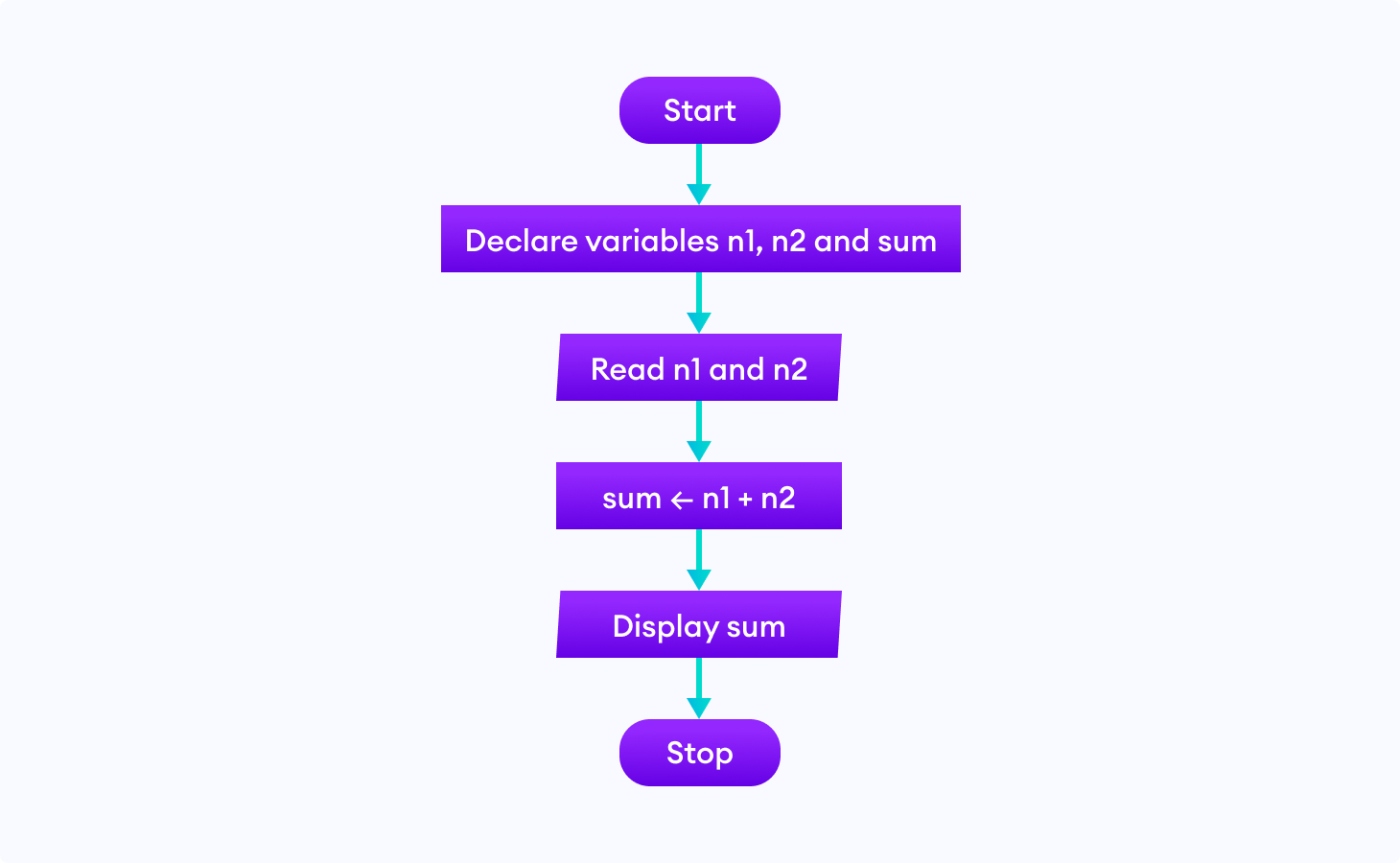
2. Find the largest among three different numbers entered by the user.
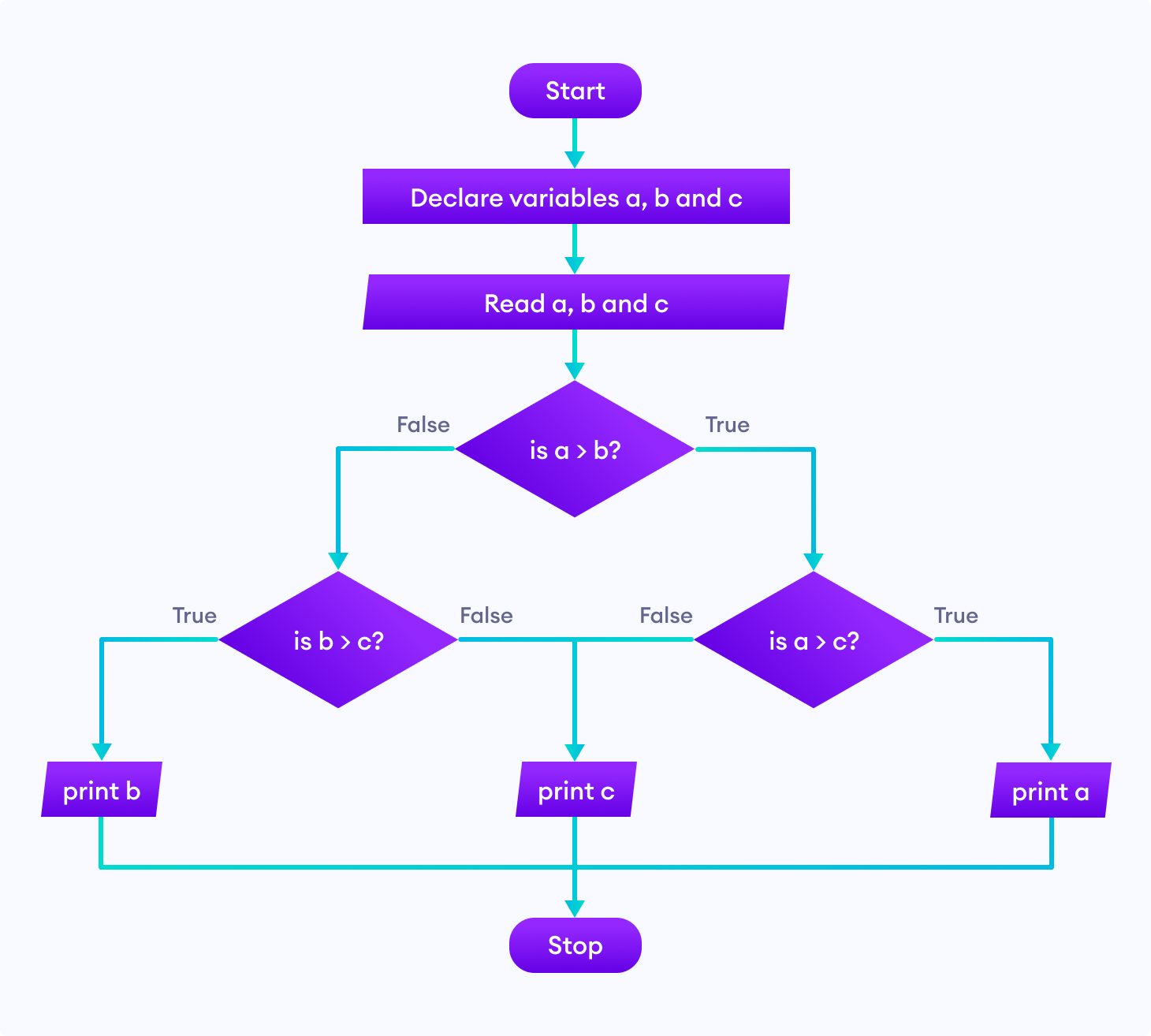
3. Find all the roots of a quadratic equation ax 2 +bx+c=0
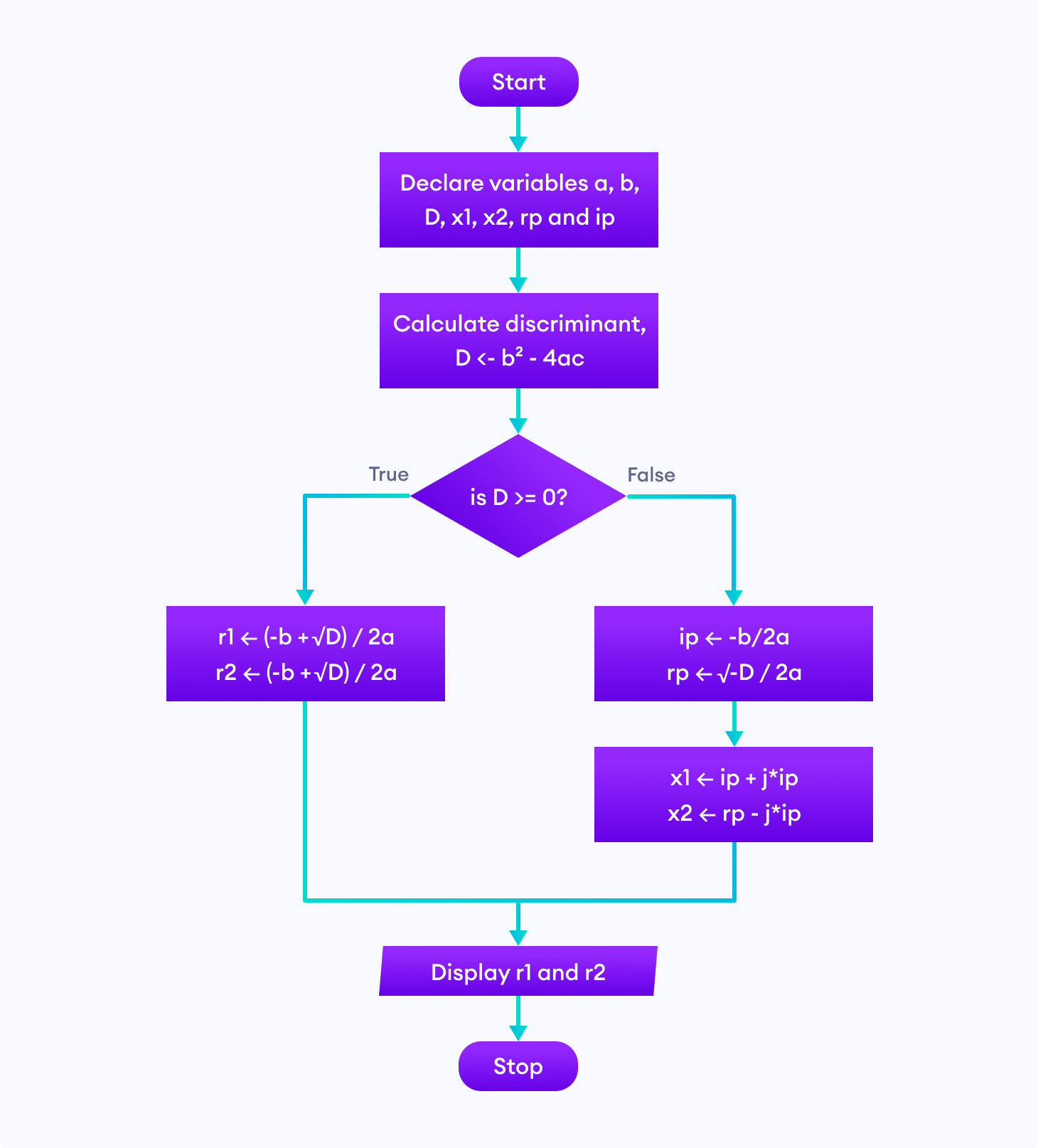
4. Find the Fibonacci series till term≤1000.
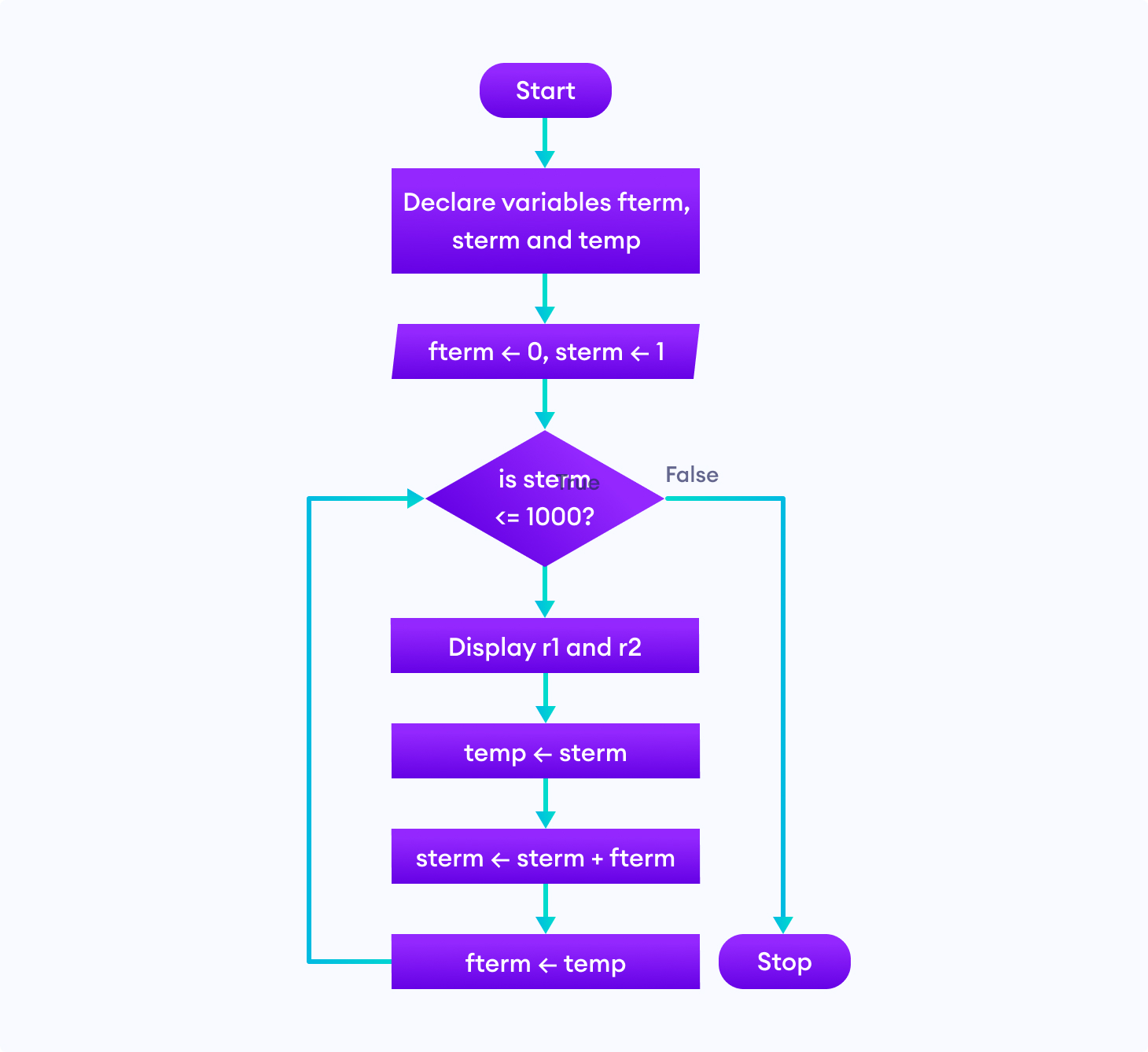
Note: Though flowcharts can be useful for writing and analyzing a program, drawing a flowchart for complex programs can be more complicated than writing the program itself. Hence, creating flowcharts for complex programs is often ignored.
Sorry about that.
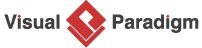
- Demo Videos
- Interactive Product Tours
- Request Demo
Flowchart Tutorial (with Symbols, Guide and Examples)
A flowchart is simply a graphical representation of steps. It shows steps in sequential order and is widely used in presenting the flow of algorithms, workflow or processes. Typically, a flowchart shows the steps as boxes of various kinds, and their order by connecting them with arrows.
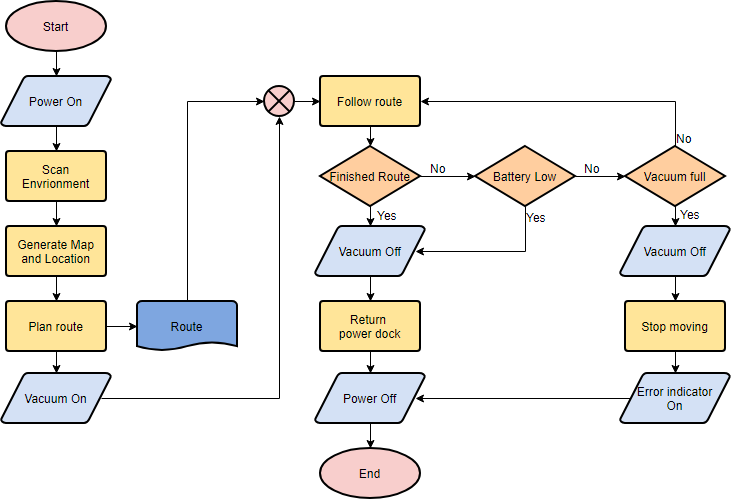
What is a Flowchart?
A flowchart is a graphical representations of steps. It was originated from computer science as a tool for representing algorithms and programming logic but had extended to use in all other kinds of processes. Nowadays, flowcharts play an extremely important role in displaying information and assisting reasoning. They help us visualize complex processes, or make explicit the structure of problems and tasks. A flowchart can also be used to define a process or project to be implemented.
Flowchart Symbols
Different flowchart shapes have different conventional meanings. The meanings of some of the more common shapes are as follows:
The terminator symbol represents the starting or ending point of the system.
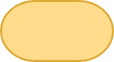
A box indicates some particular operation.
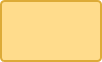
This represents a printout, such as a document or a report.
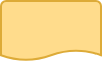
A diamond represents a decision or branching point. Lines coming out from the diamond indicates different possible situations, leading to different sub-processes.

It represents information entering or leaving the system. An input might be an order from a customer. Output can be a product to be delivered.
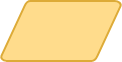
On-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on the same page.

Off-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on a different page.

Delay or Bottleneck
Identifies a delay or a bottleneck.
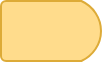
Lines represent the flow of the sequence and direction of a process.

When to Draw Flowchart?
Using a flowchart has a variety of benefits:
- It helps to clarify complex processes.
- It identifies steps that do not add value to the internal or external customer, including delays; needless storage and transportation; unnecessary work, duplication, and added expense; breakdowns in communication.
- It helps team members gain a shared understanding of the process and use this knowledge to collect data, identify problems, focus discussions, and identify resources.
- It serves as a basis for designing new processes.
Flowchart examples
Here are several flowchart examples. See how you can apply a flowchart practically.
Flowchart Example – Medical Service
This is a hospital flowchart example that shows how clinical cases shall be processed. This flowchart uses decision shapes intensively in representing alternative flows.
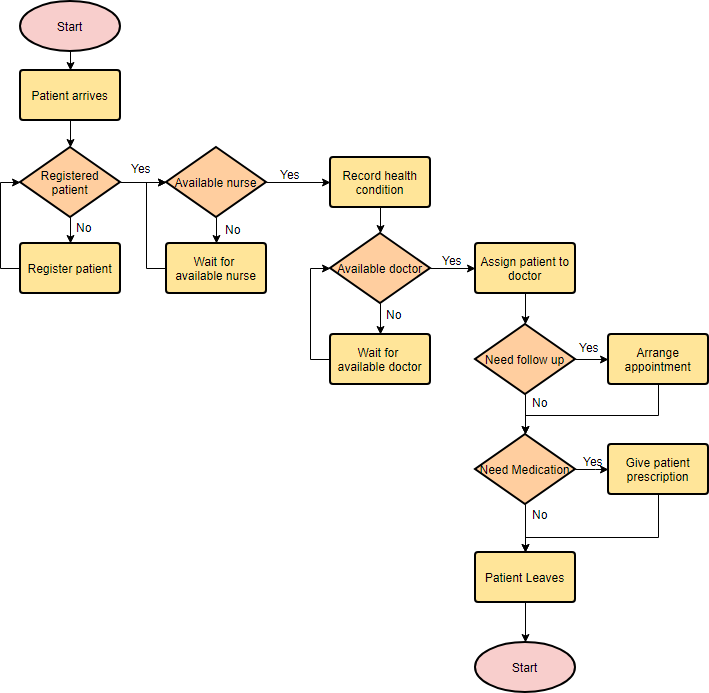
Flowchart Example – Simple Algorithms
A flowchart can also be used in visualizing algorithms, regardless of its complexity. Here is an example that shows how flowchart can be used in showing a simple summation process.
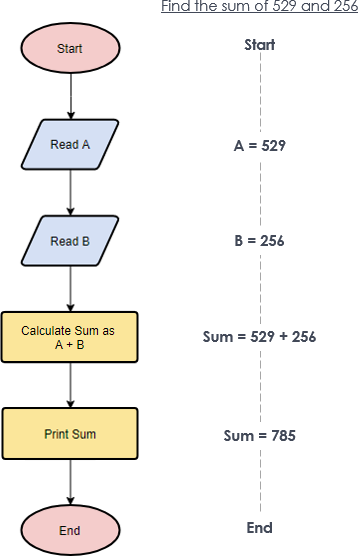
Flowchart Example – Calculate Profit and Loss
The flowchart example below shows how profit and loss can be calculated.
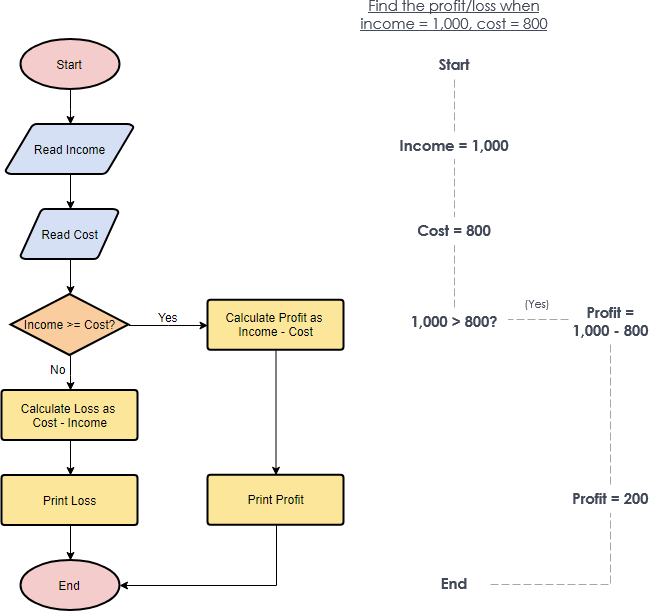
Creating a Flowchart in Visual Paradigm
Let’s see how to draw a flowchart in Visual Paradigm. We will use a very simple flowchart example here. You may expand the example when finished this tutorial.
- Select Diagram > New from the main menu.
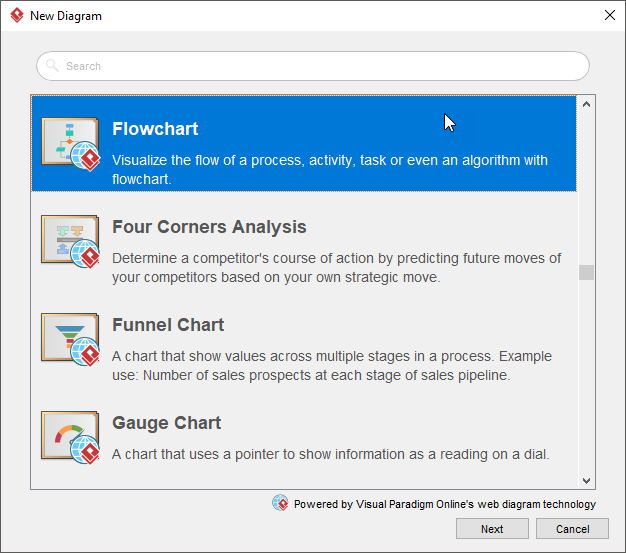
- Enter the name of the flowchart and click OK .
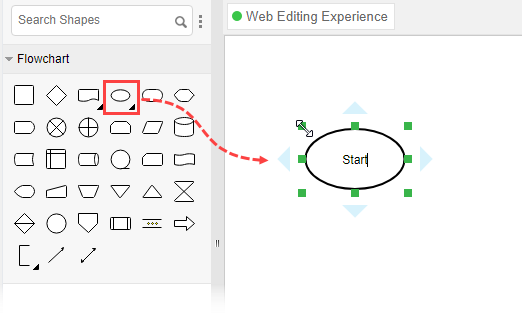
- Enter Add items to Cart as the name of the process.

Turn every software project into a successful one.
We use cookies to offer you a better experience. By visiting our website, you agree to the use of cookies as described in our Cookie Policy .
© 2024 by Visual Paradigm. All rights reserved.
- Privacy statement

Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
Kenneth Leroy Busbee
A flowchart is a type of diagram that represents an algorithm, workflow or process. The flowchart shows the steps as boxes of various kinds, and their order by connecting the boxes with arrows. This diagrammatic representation illustrates a solution model to a given problem. Flowcharts are used in analyzing, designing, documenting or managing a process or program in various fields. [1]
Common flowcharting symbols and examples follow. When first reading this section, focus on the simple symbols and examples. Return to this section in later chapters to review the advanced symbols and examples.
Simple Flowcharting Symbols
The rounded rectangles, or terminal points, indicate the flowchart’s starting and ending points.

Note: The default flow is left to right and top to bottom (the same way you read English). To save time arrowheads are often only drawn when the flow lines go contrary the normal.

Input/Output
The parallelograms designate input or output operations.

The rectangle depicts a process such as a mathematical computation, or a variable assignment.

The diamond is used to represent the true/false statement being tested in a decision symbol.

Advanced Flowcharting Symbols
Module call.
A program module is represented in a flowchart by rectangle with some lines to distinguish it from process symbol. Often programmers will make a distinction between program control and specific task modules as shown below.
Local module: usually a program control function.

Library module: usually a specific task function.

Sometimes a flowchart is broken into two or more smaller flowcharts. This is usually done when a flowchart does not fit on a single page, or must be divided into sections. A connector symbol, which is a small circle with a letter or number inside it, allows you to connect two flowcharts on the same page. A connector symbol that looks like a pocket on a shirt, allows you to connect to a flowchart on a different page.
On-Page Connector

Off-Page Connector

Simple Examples
We will demonstrate various flowcharting items by showing the flowchart for some pseudocode.
pseudocode: Function with no parameter passing

pseudocode: Function main calling the clear monitor function

Sequence Control Structures
The next item is pseudocode for a simple temperature conversion program. This demonstrates the use of both the on-page and off-page connectors. It also illustrates the sequence control structure where nothing unusual happens. Just do one instruction after another in the sequence listed.
pseudocode: Sequence control structure

Advanced Examples
Selection control structures.
pseudocode: If then Else

pseudocode: Case

Iteration (Repetition) Control Structures
pseudocode: While

pseudocode: For
The for loop does not have a standard flowcharting method and you will find it done in different ways. The for loop as a counting loop can be flowcharted similar to the while loop as a counting loop.

pseudocode: Do While

pseudocode: Repeat Until

- cnx.org: Programming Fundamentals – A Modular Structured Approach using C++
- Wikipedia: Flowchart ↵
Programming Fundamentals Copyright © 2018 by Kenneth Leroy Busbee is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License , except where otherwise noted.
Share This Book
Algorithms and Flowcharts: Mapping Process
Last updated: March 18, 2024
1. Introduction
In this tutorial, we’ll study how to represent relevant programming structures into a flowchart, thus exploring how to map an algorithm to a flowchart and vice-versa.
First, we’ll understand why using pseudocode and flowcharts to design an algorithm before actually implementing it with a specific programming language. Thus, we’ll explore the equivalence between pseudocode structures and flowchart elements. So, we’ll investigate some case studies. Finally, we’ll see a comparison between pseudocode and flowcharts in a systematic summary.
2. Designing Algorithms
As time passes, computer programs become more and more complex. In this way, many programming paradigms, structures, languages, and libraries appeared to deal with this increasing programming complexity.
With complex programs and multiple choices of resources to develop them, the decision-making of which means to use to solve a problem became a critical part of any programming project.
In such a scenario, textual and graphical designing tools were proposed to create software projects. Software projects abstract implementation details of production-intended programming languages. However, we still have logical compliance between these projects and any programming language.
Specific examples of algorithm designing tools are abstracted languages (textual) and flowcharts (graphical). Let’s see relevant concepts about them in the following subsections.
2.1. Textual Design Tools
The idea behind textual design tools is to simplify the rigorous programming languages tokens and structure, getting close to natural language. However, it does not mean that textual design tools are not free from ambiguity or can not precisely define the routines of an algorithm.
A very well-known example of a textual design tool is pseudocode. Pseudocode abstracts programming structures by redefining them with a simpler lexicon/syntax, which is typically closer to the natural and native language of the programmers.
In this way, we can find pseudocode in several different natural languages. Moreover, it is relevant to highlight that pseudocode may consider the structures of a programming language in special.
Another example is the Program Design Language (PDL). Actually, PDL is very similar to pseudocode since it aims to design an algorithm unambiguously. But, unlike pseudocode, PDL is just a structured English language, not considering any specific programming language structures.
2.2. Graphical Design Tools
Graphical tools aim to precisely and unambiguously define algorithms, as text design tools do. However, instead of using text, these tools adopt standard graphic elements representing different operations and resources of an algorithm.
A widely accepted graphic representation of algorithms in software projects is called flowcharts.
With flowcharts, we can depict resources, operations, conditionals, loopings, and data flows. So, we naturally map flowcharts to PDL, pseudocode, and programming languages (and vice-versa).
However, we need standard illustrations indicating programming operations and resources to draw flowcharts in a universally recognized way. In such a manner, the ISO 5807 was published defining element illustrations for flowcharts.
Besides the illustrations, the ISO 5807 indicates the correct form to name and use the elements to develop a complete and accurate flowchart.
Next, we’ll understand the equivalence of pseudocode structures to flowchart elements, besides the mapping process between them.
3. Flowchart Elements and Peseudocode Structures
As previously stated, programming structures of pseudocode have, in most cases, a direct mapping to flowchart elements. Even when there is no direct mapping, the flowchart elements relate to some operational aspects of pseudocode. Let’s see how it happens.
3.1. Terminator
According to ISO 5807, every flowchart has a starting and ending element, which we call a terminator. The illustration of the terminator element follows:
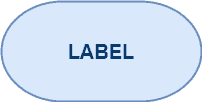
Terminators occur only twice in a flowchart : to indicate the beginning of the logical flows or determine the ending of the same logical flows. Both occurrences of this element are mandatory.
Making an analogy with pseudocode, terminators indicate the bounds of a program, subroutine, or function.
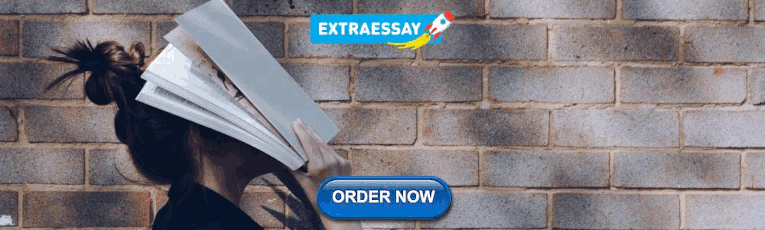
3.2. Manual Input
Elements that indicate data provided to a software program by manual means. Manual inputs are optional elements and can occur several times in the same flowchart.
The following image depicts the symbol of manual inputs:
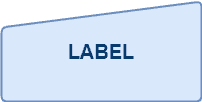
In practice, we can understand manual inputs as data provided through, for example, push buttons, barcode readers, and (most commonly) keyboards. In pseudocode, reading operations that involve the user translates to manual inputs.
3.3. Process
Processes represent the execution of operations that manipulates data. Thus, a process execution usually changes data values, form, or location. This element is optional and may happen many times in flowcharts.
The image below depicts the symbol used for process elements:
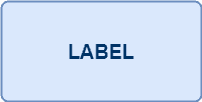
Any data manipulation in pseudocode maps to processes in flowcharts. So, arithmetic operations and assignment operations, for instance, are candidates to define a processing element.
3.4. Display
The display element indicates data exhibited in any medium that makes it accessible to the users. This element is optional and employable several times in the same flowchart.
Next, we have the image that depicts the display element:
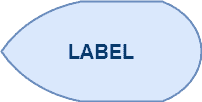
The most representative equipment of a display element is the computer screen. Thus, we can map printing operations defined in pseudocode to flowcharts’ display elements.
3.5. Decision
Decision elements are conditional constructs in a flowchart. In this way, decisions have a single entry point but many possible exits. So, choosing one of these exits undergoes evaluations of conditions defined within the element.
This element is optional, and we can have multiple occurrences in a flowchart.
The following image shows the symbol of a decision element:
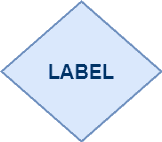
Conditional constructs of pseudocode can be directly mapped to decision elements in flowcharts. Examples are the if , if-else , and switch-case logical blocks.
3.6. Predefined Process
Predefined processes represent a process defined elsewhere. In typical cases, predefined processes express routines described as other flowcharts. This element is optional, and we can use it many times in a flowchart.
The image that illustrates a predefined process element in a flowchart follows:
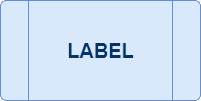
In pseudocode, subroutines and functions frequently produce a flowchart by themselves. So, other routines that call these functions and subroutines can employ predefined process elements to refer to these other flowcharts.
3.7. Connector
Connector elements make it possible to split a flowchart into multiple segments. Thus, connectors link these different flowchart segments, presenting the logical order between them.
Furthermore, connectors are often used to merge flow lines (see more about them in the following subsection) into a single line. It may occur, for example, after the definition of conditionals.
Connectors are optional elements, having from zero to several occurrences in flowcharts.
The following image depicts the symbol of connectors:
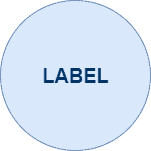
A practical example of a connector usage scenario is defining an if-else logical block in pseudocode. The execution flow returns to a common point after executing an if or else block. So, we can merge the different execution flows from conditionals into a single one with connectors.
Lines indicate the relations between elements, creating the possible execution flows in a flowchart. Moreover, lines have an arrowed side specifying the execution flow direction.
Lines are mandatory elements, with at least one occurrence in a flowchart.
We have the available symbols to define a line element depicted next:
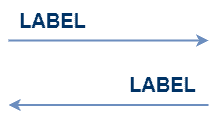
We do not have specific pseudocode constructs to exemplify a line. Actually, the manner in that we write pseudocode already defines its execution flows. So, it also determines how lines will be placed in a flowchart.
3.9. Other Elements
The flowchart elements presented in the previous subsections are the most popular ones. However, we have many other flowchart elements tailored for other specific operations and resources.
For instance, we have elements of preparation, delay, storage, and comment.
All those elements are presented and defined in the ISO 5807 specification document.
4. Pseudocode/Flowchart Mapping Example
Let’s consider the implementation of a simplified craps game. In this game, we throw two dice and check the sum of the values: if the sum is 2, 3, 4, 10, 11, or 11, we won; in any other case, we lost.
The following pseudocode shows a simplified craps game algorithm:
Our objective is to map the simplified craps game algorithm to a flowchart. Thus, we’ll analyze the lines of the algorithm and identify the proper flowchart element to represent them.
First, we have lines 1, 2, and 3. These lines have arithmetic expressions and attribution operations. So, we are executing internal processes of the game to determine the dice values and the summing of them. In this way, we can map these lines to a process block.
Lines 4 and 5, in turn, execute operations to show the dice values for the user. Thus, since we have output operations, we can use a display block to represent them in the flowchart.
Lines 6 and 8, however, form a conditional if-else logical block. In such a manner, we can employ a decision element to depict it in the flowchart.
Finally, lines 7 and 9 are exhibition operations. So, we can also use display elements to represent them.
Of course, we delineate the algorithm bounds with the mandatory terminator elements and define the workflows with lines.
The following image shows the corresponding flowchart for the previously presented algorithm:
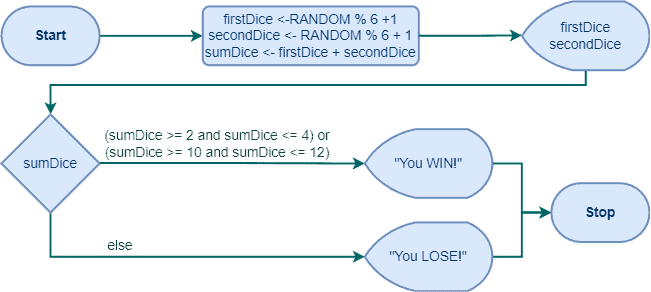
5. Systematic Summary
As software systems become more and more complex, new design tools emerged to support their projects in a standard and efficient way.
Some of these tools stood out. Examples are the textual tools of pseudocode and PDL and the graphical tools of flowcharts.
In particular, ISO 5807 proposed several elements to build flowcharts, providing their graphical representation and role standardization.
It is relevant to highlight that there are many design systems to create flowcharts. An example of a generic system in which we can do it is LaTeX .
The following table summarizes the most relevant flowchart elements and describes their meaning in a flowchart:
Brief Definition | Symbol | Pseudocode Structures | |
---|---|---|---|
Terminator | Mandatory element; Indicates the beginning and the end of a flowchart | Ellipse | The boundaries of a function or program |
Manual Input | Optional element; Indicates data input by manual means | Quadrilateral | Reading operations (e.g., input, scanf, cin, …) |
Process | Optional element; Means operation that manipulate data | Rectangle | Assignments, arithmetic operations |
Display | Optional element; Represents data output operations | Kite | operations… |
Decision | Optional element; Consists of conditional constructs that can split the workflow | Rhombus | Conditional constructs (e.g., if-else, switch-case) |
Predefined Process | Optional Element; Indicates Processes and routines defined elsewhere | Overlapping Rectangles | Sub-routines and functions |
Connector | Optional Element; Represents a split point in the flowchart | Circle | — |
Line | Mandatory Element; Shows the execution flows of a flowchart | Arrows | The execution order of pseudocode operations |
6. Conclusion
In this tutorial, we studied flowcharts. First, we reviewed concepts of algorithm design tools, including both the textual and graphical ones. Thus, we explored the flowchart elements (according to the ISO 5807) and their relation with pseudocode structures. So, we saw the mapping process of an example pseudocode to a flowchart. Finally, we compiled relevant information on each studied flowchart element in a systematic summary.
We can conclude that flowcharts are great tools for describing algorithms. With them, we can create designs logically compliant with implementations employing production programming languages.
EdrawMax – Easy Diagram App
Make a diagram in 3 steps.
- Create more than 280 types of diagrams
- Supply more than 1500 built-in templates and 26,000 symbols
- Share your work and collaborate with your team in any file format
- What is a Flowchart
- How to Draw a FlowChart
- Flowchart Symbol and Their Usage
- Flowchart Examples & Templates
- Free Flowchart Maker
- What is a Floor Plan
- How to Draw Your Own Floor Plan
- Floor Plan Symbols
- Floor Plan Examples & Templates
- Floor Plan Maker
- What is a UML Diagram
- How to Create A UML Class Diagram
- UML Diagram Symbol
- UML Diagram Examples & Templates
- UML Diagram Tool
- Wiring Diagram
- Circuit Diagram
- How to Create a Basic Electrical Diagram
- Basic Electrical Symbols and Their Usage
- Electrical Drawing Software
- What is a Mind Map
- How to Create A Mind Map on Microsoft Word
- Mind Map Examples & Templates
- Free Mind Map Software
- What is an Organizational Chart
- How to Create an Org Chart
- Basic Organizational Chart Symbols
- Free Org Chart Templates
- Organizational Chart Software
- What is a P&ID
- How to Read P&ID
- How to Create a P&ID Drawing
- P&ID Symbol Legend
- P&ID Software
- What is a Gantt Chart
- What is a Milestone in a Gantt Chart
- How to Make a Gantt Chart
- Gantt Chart Examples
- Gantt Chart Symbols
- Gantt Chart Maker
- What is an Infographic
- 50+ Editable Infographic Templates
- How to Make an Infographic
- 7 Easy Tips to Make Your Infographics Stand Out
- Infographic Maker
- Diagram Center
Explain Algorithm and Flowchart with Examples
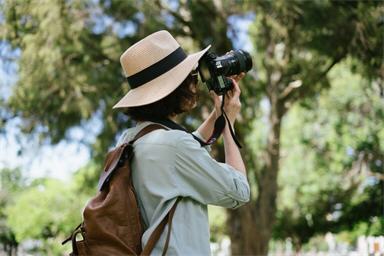
The algorithm and flowchart are two types of tools to explain the process of a program. In this page, we discuss the differences between an algorithm and a flowchart and how to create a flowchart to illustrate the algorithm visually. Algorithms and flowcharts are two different tools that are helpful for creating new programs, especially in computer programming. An algorithm is a step-by-step analysis of the process, while a flowchart explains the steps of a program in a graphical way.
In this article
Definition of algorithm, definition of flowchart, difference between algorithm and flowchart.
- Recursive Algorithm
- Divide and Conquer Algorithm
- Dynamic Programming Algorithm
- Greedy Algorithm
- Brute Force Algorithm
- Backtracking Algorithm
Use Flowcharts to Represent Algorithms
Writing a logical step-by-step method to solve the problem is called the algorithm . In other words, an algorithm is a procedure for solving problems. In order to solve a mathematical or computer problem, this is the first step in the process.
An algorithm includes calculations, reasoning, and data processing. Algorithms can be presented by natural languages, pseudocode, and flowcharts, etc.
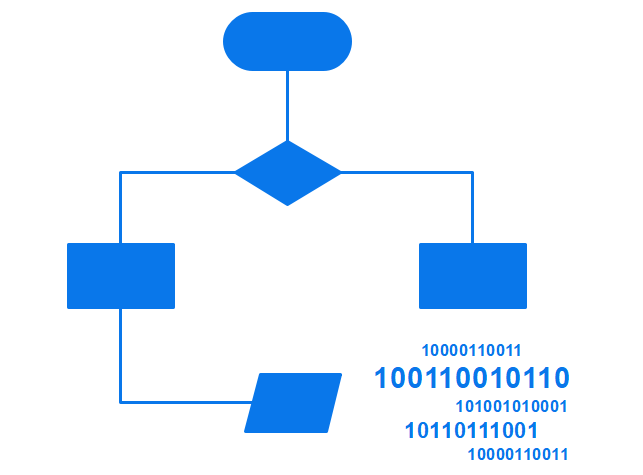
A flowchart is the graphical or pictorial representation of an algorithm with the help of different symbols, shapes, and arrows to demonstrate a process or a program. With algorithms, we can easily understand a program. The main purpose of using a flowchart is to analyze different methods. Several standard symbols are applied in a flowchart:
Terminal Box - Start / End | |
Input / Output | |
Process / Instruction | |
Decision | |
Connector / Arrow |
The symbols above represent different parts of a flowchart. The process in a flowchart can be expressed through boxes and arrows with different sizes and colors. In a flowchart, we can easily highlight certain elements and the relationships between each part.
During the reading of this article, if you find an icon type picture that you are interested in, you can download EdrawMax products to discover more or experience creating a free diagram of your own. All drawings are from EdrawMax .

If you compare a flowchart to a movie, then an algorithm is the story of that movie. In other words, an algorithm is the core of a flowchart . Actually, in the field of computer programming, there are many differences between algorithm and flowchart regarding various aspects, such as the accuracy, the way they display, and the way people feel about them. Below is a table illustrating the differences between them in detail.
- It is a procedure for solving problems.
- The process is shown in step-by-step instruction.
- It is complex and difficult to understand.
- It is convenient to debug errors.
- The solution is showcased in natural language.
- It is somewhat easier to solve complex problem.
- It costs more time to create an algorithm.
- It is a graphic representation of a process.
- The process is shown in block-by-block information diagram.
- It is intuitive and easy to understand.
- It is hard to debug errors.
- The solution is showcased in pictorial format.
- It is hard to solve complex problem.
- It costs less time to create a flowchart.

EdrawMax provides beginners and pros the cutting-edge functionalities to build professional-looking diagrams easier, faster, and cheaper! It allows you to create more than 280 types of diagrams and should be an excellent Visio alternative.
Types of Algorithm
It is not surprising that algorithms are widely used in computer programming. However, it can be applied to solving mathematical problems and even in everyday life. Here comes a question: how many types of algorithms? According to Dr. Christoph Koutschan, a computer scientist working at the Research Institute for Symbolic Computation (RISC) in Austria, he has surveyed voting for the important types of algorithms. As a result, he has listed 32 crucial algorithms in computer science. Despite the complexity of algorithms, we can generally divide algorithms into six fundamental types based on their function.
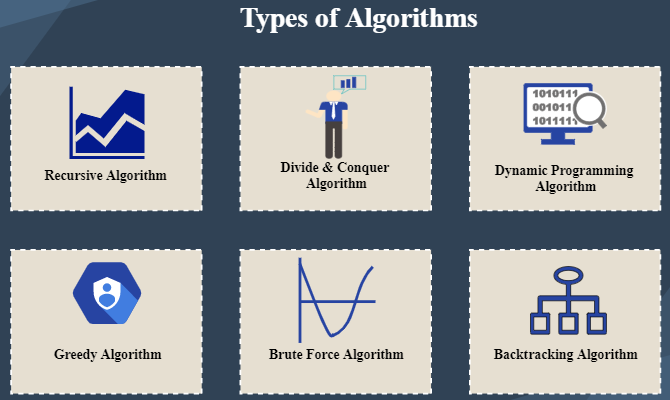
#1 Recursive Algorithm
It refers to a way to solve problems by repeatedly breaking down the problem into sub-problems of the same kind. The classic example of using a recursive algorithm to solve problems is the Tower of Hanoi.
#2 Divide and Conquer Algorithm
Traditionally, the divide and conquer algorithm consists of two parts: 1. breaking down a problem into some smaller independent sub-problems of the same type; 2. finding the final solution of the original issues after solving these more minor problems separately. The key points of the divide and conquer algorithm are:
- If you can find the repeated sub-problems and the loop substructure of the original problem, you may quickly turn the original problem into a small, simple issue.
- Try to break down the whole solution into various steps (different steps need different solutions) to make the process easier.
- Are sub-problems easy to solve? If not, the original problem may cost lots of time.
#3 Dynamic Programming Algorithm
Developed by Richard Bellman in the 1950s, the dynamic programming algorithm is generally used for optimization problems. In this type of algorithm, past results are collected for future use. Like the divide and conquer algorithm, a dynamic programming algorithm simplifies a complex problem by breaking it down into some simple sub-problems. However, the most significant difference between them is that the latter requires overlapping sub-problems, while the former doesn’t need to.
#4 Greedy Algorithm
This is another way of solving optimization problems – greedy algorithm. It refers to always finding the best solution in every step instead of considering the overall optimality. That is to say, what he has done is just at a local optimum. Due to the limitations of the greedy algorithm, it has to be noted that the key to choosing a greedy algorithm is whether to consider any consequences in the future.
#5 Brute Force Algorithm
The brute force algorithm is a simple and straightforward solution to the problem, generally based on the description of the problem and the definition of the concept involved. You can also use "just do it!" to describe the strategy of brute force. In short, a brute force algorithm is considered as one of the simplest algorithms, which iterates all possibilities and ends up with a satisfactory solution.
#6 Backtracking Algorithm
Based on a depth-first recursive search, the backtracking algorithm focusing on finding the solution to the problem during the enumeration-like searching process. When it cannot satisfy the condition, it will return "backtracking" and tries another path. It is suitable for solving large and complicated problems, which gains the reputation of the "general solution method". One of the most famous backtracking algorithm example it the eight queens puzzle.
Now that we have learned the definitions of algorithm and flowchart, how can we use a flowchart to represent an algorithm? To create an algorithm flowchart, we need to use a handy diagramming tool like EdrawMax to finish the work.
Algorithms are mainly used for mathematical and computer programs, whilst flowcharts can be used to describe all sorts of processes: business, educational, personal, and algorithms. So flowcharts are often used as a program planning tool to organize the program's step-by-step process visually. Here are some examples:
Example 1: Print 1 to 20:
- Step 1: Initialize X as 0,
- Step 2: Increment X by 1,
- Step 3: Print X,
- Step 4: If X is less than 20 then go back to step 2.
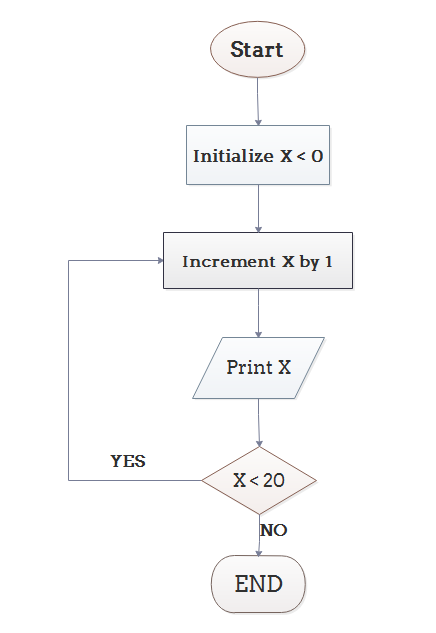
Example 2: Convert Temperature from Fahrenheit (℉) to Celsius (℃)
- Step 1: Read temperature in Fahrenheit,
- Step 2: Calculate temperature with formula C=5/9*(F-32),
- Step 3: Print C.
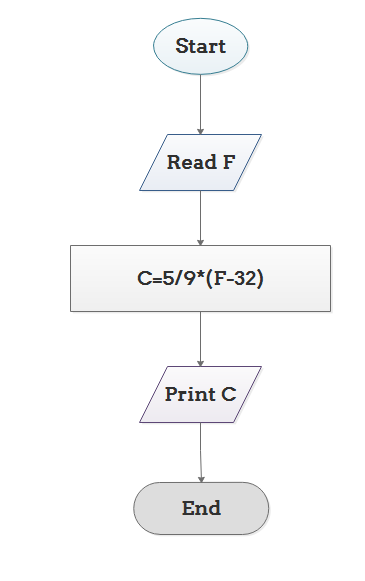
Example 3: Determine Whether A Student Passed the Exam or Not:
- Step 1: Input grades of 4 courses M1, M2, M3 and M4,
- Step 2: Calculate the average grade with formula "Grade=(M1+M2+M3+M4)/4"
- Step 3: If the average grade is less than 60, print "FAIL", else print "PASS".

From the above, we can come to the conclusion that a flowchart is a pictorial representation of an algorithm, an algorithm can be expressed and analyzed through a flowchart. An algorithm shows you every step of reaching the final solution, while a flowchart shows you how to carry out the process by connecting each step. An algorithm uses mainly words to describe the steps while you can create a flowchart with flowchart symbols to make the process more logical.
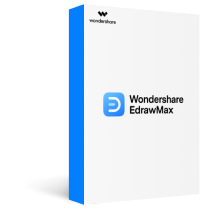
You May Also Like
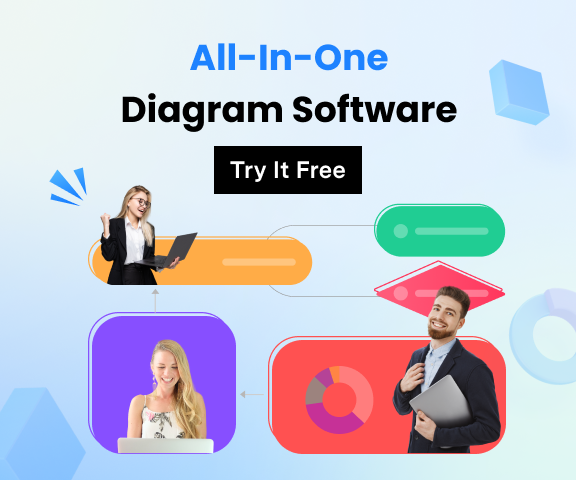
Related articles
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
AP®︎/College Computer Science Principles
Course: ap®︎/college computer science principles > unit 4, the building blocks of algorithms.
- Expressing an algorithm

Want to join the conversation?
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

- What is System Design
- Design Patterns
- Creational Design Patterns
- Structural Design Patterns
- Behavioral Design Patterns
- Design Patterns Cheat Sheet
- Design Patterns Interview Questions
- Design Patterns in Java
- Design Patterns in JavaScript
- Design Patterns in C++
- Design Patterns in Python
An introduction to Flowcharts
What is a Flowchart? Flowchart is a graphical representation of an algorithm. Programmers often use it as a program-planning tool to solve a problem. It makes use of symbols which are connected among them to indicate the flow of information and processing. The process of drawing a flowchart for an algorithm is known as “flowcharting”.
Basic Symbols used in Flowchart Designs
- Terminal: The oval symbol indicates Start, Stop and Halt in a program’s logic flow. A pause/halt is generally used in a program logic under some error conditions. Terminal is the first and last symbols in the flowchart.
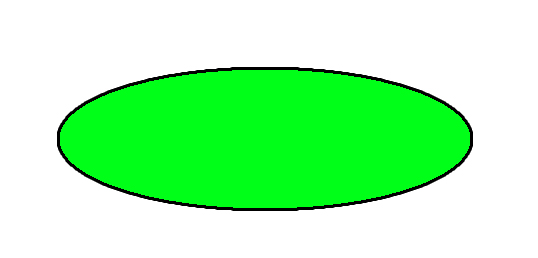
- Input/Output: A parallelogram denotes any function of input/output type. Program instructions that take input from input devices and display output on output devices are indicated with parallelogram in a flowchart.
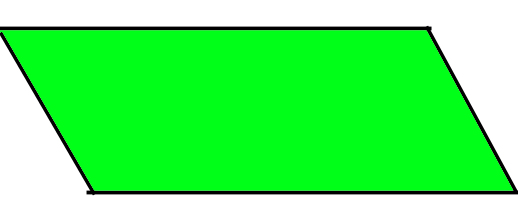
- Processing: A box represents arithmetic instructions. All arithmetic processes such as adding, subtracting, multiplication and division are indicated by action or process symbol.
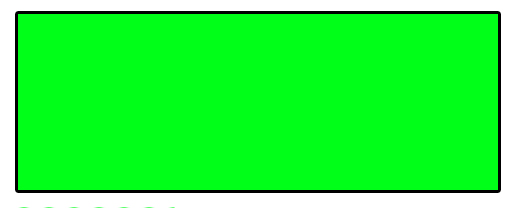
- Decision Diamond symbol represents a decision point. Decision based operations such as yes/no question or true/false are indicated by diamond in flowchart.
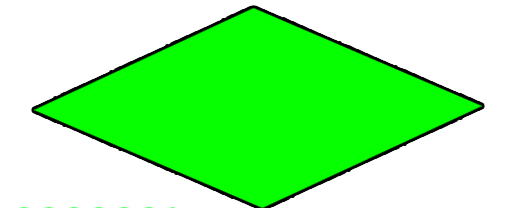
- Connectors: Whenever flowchart becomes complex or it spreads over more than one page, it is useful to use connectors to avoid any confusions. It is represented by a circle.
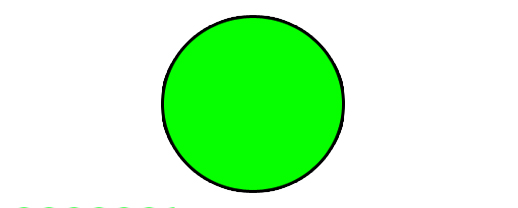
- Flow lines: Flow lines indicate the exact sequence in which instructions are executed. Arrows represent the direction of flow of control and relationship among different symbols of flowchart.
Rules For Creating Flowchart :
A flowchart is a graphical representation of an algorithm.it should follow some rules while creating a flowchart Rule 1: Flowchart opening statement must be ‘start’ keyword. Rule 2: Flowchart ending statement must be ‘end’ keyword. Rule 3: All symbols in the flowchart must be connected with an arrow line. Rule 4: The decision symbol in the flowchart is associated with the arrow line.
Advantages of Flowchart:
- Flowcharts are a better way of communicating the logic of the system.
- Flowcharts act as a guide for blueprint during program designed.
- Flowcharts help in debugging process.
- With the help of flowcharts programs can be easily analyzed.
- It provides better documentation.
- Flowcharts serve as a good proper documentation.
- Easy to trace errors in the software.
- Easy to understand.
- The flowchart can be reused for inconvenience in the future.
- It helps to provide correct logic.
Disadvantages of Flowchart:
- It is difficult to draw flowcharts for large and complex programs.
- There is no standard to determine the amount of detail.
- Difficult to reproduce the flowcharts.
- It is very difficult to modify the Flowchart.
- Making a flowchart is costly.
- Some developer thinks that it is waste of time.
- It makes software processes low.
- If changes are done in software, then the flowchart must be redrawn
Example : Draw a flowchart to input two numbers from the user and display the largest of two numbers
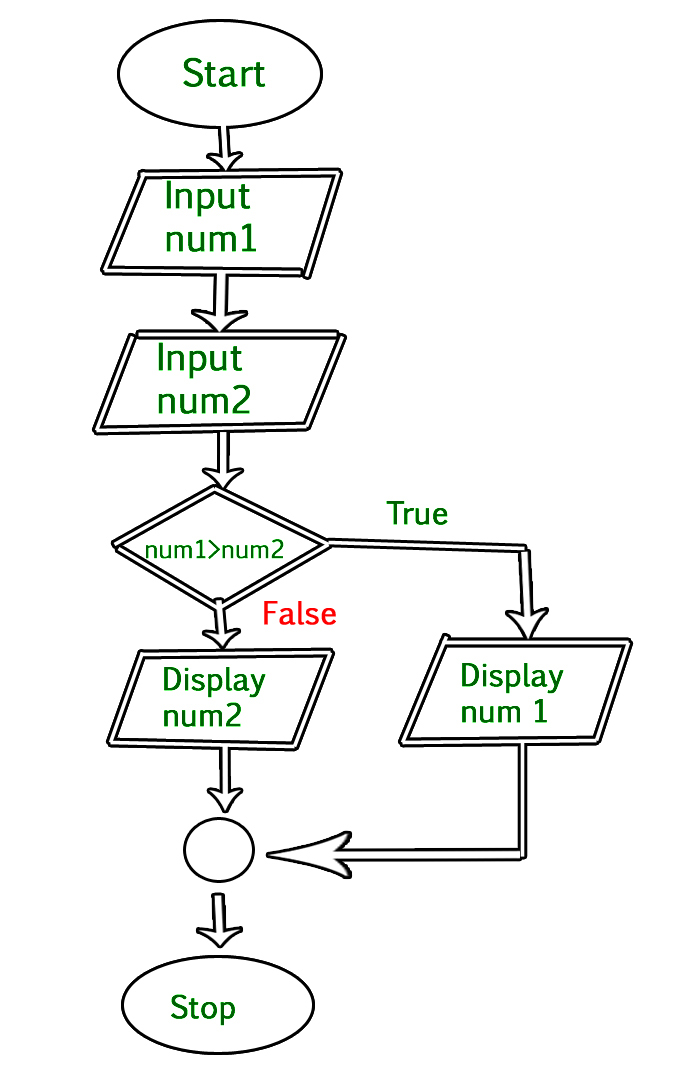
References: Computer Fundamentals by Pradeep K. Sinha and Priti Sinha
Please Login to comment...
Similar reads.
- Design Pattern
- School Programming
- System Design
- Technical Scripter
- CBSE - Class 11
- school-programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Algorithms and Flowcharts
Class 8 - logix kips icse computer with bluej, fill in the blanks.
The step-by-step procedure to solve any problem is called Algorithm .
Flowcharts are the pictorial representation of a program.
The process of drawing a flowchart for an algorithm is known as Flowcharting .
An algorithm should involve Finite number of steps to reach a solution.
The Decision box is used for checking or applying any condition in the program.
State True or False
A flowchart is not a pictorial representation of steps to get the solution of a problem. False
Algorithm means a set of rules which specify how to solve a specific problem. True
Flowcharts are helpful in analyzing the logic of problems. True
Connectors are used to connect the boxes. False
The general direction of flow in any flowchart is from bottom to top or from right to left. True
Multiple Choice Questions
In a flowchart, .......... indicate the sequence of steps and the direction of flow.
- Flow lines ✓
- Decision box
- Processing box
.......... is used to accept input and give output of a program.
- Input/Output box ✓
The .......... represents the starting or ending point of a program.
- Start /Stop box ✓
.......... connectors are used to join the parts of a flowchart contained within the same page.
- None of these
Draw the flowchart symbols for the following
Input a, b:, answer the following.
What is an algorithm? Write any three characteristics of an algorithm.
The step-by-step procedure to solve any logical and mathematical problem is called an Algorithm. Three characteristics of an algorithm are:
- Input — An algorithm accepts an input.
- Generality — An algorithm works in a set of inputs.
- Definiteness — Each instruction should be written in a simple and precise manner so that everyone can understand it.
Define the term flowchart. Write any two advantages of a flowchart.
A flowchart is a pictorial representation of the steps or an algorithm used to solve a particular problem. Two advantages of a flowchart are:
- Communication — The pictorial representation of the flowchart provides better communication. It is easier for the programmer to explain the logic of a program.
- Effective Analysis — It is a very useful technique, as flowchart is a pictorial representation that helps the programmer to analyze the problem in detail.
What is the use of input/output box?
It is used for accepting inputs or giving output of the program.
When do we use the Process box?
It is used for writing the processing instructions and doing calculations.
What is the use of Decision box?
It is used for checking or applying any condition in the program.
Write algorithms for the following problems
To polish your shoes.
Step 1: Start Step 2: Open the shoe polish Step 3: Put shoe polish on brush Step 4: Polish one shoe Step 5: Put shoe polish on brush Step 6: Polish the other shoe Step 7: Close the shoe polish Step 8: Stop
To input three sides of a triangle and print if it is scalene, isosceles or equilateral.
Step 1: Start Step 2: Read three sides of triangle and store them in a, b, c. Step 3: Check if a == b and b == c Step 4: If true, print "Equilateral Triangle" and goto step 8 Step 5: Check if a == b or b == c or c == a Step 6: If true, print "Isosceles Triangle" and goto step 8 Step 7: Print "Scalene Triangle" Step 8: Stop
Draw the following flowcharts
Draw a flowchart in Microsoft Word for buying stationery from the market.
Draw a flowchart in Microsoft Word for the steps required to play music stored in a CD.
Draw a flowchart in Microsoft Word to accept two numbers, if the first number is greater than the second number, print their sum, otherwise print their product.
Draw a flowchart in Microsoft Word for the steps required to find the cost of 24 pens when the cost of one pen is Rs. 12.
Write an algorithm and draw the flowchart for the following
Finding the cube of a given number.
Step 1: Start Step 2: Read the number and store it in a. Step 3: Calculate cube of number as a * a * a Step 4: Print cube of number Step 5: Stop
Print the smallest of two numbers.
Step 1: Start Step 2: Read the two numbers and store them in a and b. Step 3: Check if a < b Step 4: If true then print a and goto step 6 Step 5: If false then print b Step 6: Stop
Correct the Flowchart symbols
To wash the face
Corrected Flowchart
To multiply the two numbers
Make flowcharts for the following statements
Accept the age of a person. Display the message Eligible For Role if the age is equal to or greater than 18, otherwise display the message Not Eligible.
Input a name. If name is Kabir, then accept marks. If marks are >= 85, then display Good Performance else display Pass.
Accept one character. Display the message Vowel if the entered character is a vowel, otherwise display the message Not a Vowel.
Input a year. Display the message Leap year if the entered year has 366 days, otherwise display the message Not a leap year.
HAC-based adaptive combined pick-up path optimization strategy for intelligent warehouse
- Original Research Paper
- Published: 18 August 2024
Cite this article
- Shuhui Bi ORCID: orcid.org/0000-0002-2832-4985 1 ,
- Ronghao Shang 1 ,
- Haofeng Luo 1 ,
- Yuan Xu 1 ,
- Zhihao Li 1 &
- Yudong Zhang 2
Smart warehousing has been widely used due to its efficient storage and applications. However, the efficiency of transporting high-demand goods is still limited, because the existing methods lack path optimization strategies applicable to multiple scenarios and are unable to adapt conflict strategies to different warehouses. For solving these problems, this paper considers a multi-robot path planning method from three aspects: conflict-free scheduling, order picking and collision avoidance, which is adaptive to the picking needs of different warehouses by hierarchical agglomerative clustering algorithm, improved Reservation Table, and Dynamic Weighted Table. Firstly, the traditional A* algorithm is improved to better fit the actual warehouse operation mode. Secondly, the reservation table method is applied to solve the head-on collision problem of robots, and this paper improves the efficiency of the reservation table by changing the form of the reservation table. And the dynamic weighted table is added to solve the multi-robot problem about intersection conflict. Then, the HAC algorithm is applied to analyse the goods demand degree in current orders based on historical order data and rearrange the goods order in descending order, so that goods with a high-demand degree can be discharged from the warehouse in the first batch. Moreover, a complete outbound process is presented, which integrates HAC algorithm, improved reservation table and dynamic weighting table. Finally, the simulation is done to verify the validity of the proposed algorithm, which shows that the overall transit time of high-demand goods is reduced by 21.84% on average compared to the “A* + reservation table” algorithm, and the effectiveness of the solution is fully verified.
This is a preview of subscription content, log in via an institution to check access.
Access this article
Subscribe and save.
- Get 10 units per month
- Download Article/Chapter or eBook
- 1 Unit = 1 Article or 1 Chapter
- Cancel anytime
Price includes VAT (Russian Federation)
Instant access to the full article PDF.
Rent this article via DeepDyve
Institutional subscriptions
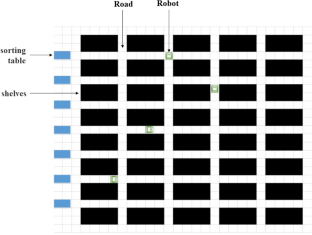
Explore related subjects
- Artificial Intelligence
Code Availability
All research data supporting this paper are directly available within this paper.
Li Z, Wang X, Bi S, Zhao Q (2022) Collaborative path optimization method for flood control material storage. In: IoT and big data technologies for health care: second EAI international conference, IoTCare 2021, Part II, pp. 440–450
Garnier S, Gautrais J, Theraulaz G (2007) The biological principles of swarm intelligence. Swarm Intell 1:3–31
Article Google Scholar
Agrawal A, Sudheer A, Ashok S (2015) Ant colony based path planning for swarm robots. In: Proceedings of the 2015 conference on advances in robotics, pp. 1–5
Li S, Weihua S, Guo P, Zhang S, Xie P (2020) Research on SAR drone global path planning based on improved A* algorithm. Chin. Med. Equip. J 41(12):16–20
Hidalgo-Paniagua A, Vega-Rodríguez MA, Ferruz J, Pavón N (2017) Solving the multi-objective path planning problem in mobile robotics with a firefly-based approach. Soft Comput 21:949–964
Liu Y, Chen Z, Li Y, Lu M, Chen C, Zhang X (2022) Robot search path planning method based on prioritized deep reinforcement learning. Int J Control Autom Syst 20(8):2669–2680
Lv X, Li W, Wang J (2022) Safety-field-based path planning algorithm of lane changing for autonomous vehicles. Int J Control Autom Syst 20(2):564–576
Bi S, Wang Q, Xu Y, Zhang Y (2023) Multiple factors collaborative optimisation of intelligent storage system. Int J Adv Mech Syst 10(4):165–173
Google Scholar
Boysen N, Briskorn D, Emde S (2017) Parts-to-picker based order processing in a rack-moving mobile robots environment. Eur J Oper Res 262(2):550–562
Xie L, Thieme N, Krenzler R, Li H (2021) Introducing split orders and optimizing operational policies in robotic mobile fulfillment systems. Eur J Oper Res 288(1):80–97
Article MathSciNet Google Scholar
Zou B (2018) Study on operational policies optimization in vehicle-based storage and retrieval systems. PhD thesis, Huazhong University of Science and Technology
Bolu A, Korçak Ö (2021) Adaptive task planning for multi-robot smart warehouse. IEEE Access 9:27346–27358
Zhang J, Yang F, Weng X (2019) A building-block-based genetic algorithm for solving the robots allocation problem in a robotic mobile fulfilment system. Math Probl Eng 25:1–15
Fan LWHLY (2019) The collaborative scheduling of multi-mobile robots in intelligentwarehouse based on the lmproved genetic algorithm. J Wuhan Univ Technol (Inf Manag Eng) 41(3):293–298
Vivaldini K, Rocha LF, Martarelli NJ, Becker M, Moreira AP (2016) Integrated tasks assignment and routing for the estimation of the optimal number of AGVs. Int J Adv Manuf Technol 82:719–736
Li F (2020) Big data-based method and system for warehouse storage space allocation. CN111476413A
Zou X (2018) A study on the efficiency optimization of AVS/RS and picking in B2C e-commerce distribution center. PhD thesis, Shandong University
Li X, Liang Y, Young Y (2019) Apriori algorithm-based analysis of storage space optimization for split-zero shelves. China Mark 27:181–182
Xue F, Dong T (2018) Research on the logistics robot task allocation method based on improved ant colony algorithm. Int J Wirel Mob Comput 14(4):307–311
Merschformann M, Lamballais T, De Koster M, Suhl L (2019) Decision rules for robotic mobile fulfillment systems. Oper Res Perspect 6:100128
Bi S, Li Z, Wang L, Xu Y (2023) Dynamic weighted and heat-map integrated scalable information path-planning algorithm. EAI Endorsed Trans Scalable Inf Syst 10(2):e5
Pagnuco I, Pastore J, Abras G, Brun M, Ballarin V (2017) Analysis of genetic association using hierarchical clustering and cluster validation indices. Genomics 109(5–6):438–445
Hu R, Dou W, Liu J (2014) A clustering-based collaborative filtering approach for big data application. IEEE Trans Emerg Top Comput 2(3):302–313
Tan P-N, Steinbach M, Kumar V (2016) Introduction to data mining. Pearson India
Lung C-H, Zhou C (2010) Using hierarchical agglomerative clustering in wireless sensor networks: an energy-efficient and flexible approach. Ad Hoc Netw 8(3):328–344
Chim H, Deng X (2008) Efficient phrase-based document similarity for clustering. IEEE Trans Knowl Data Eng 20(9):1217–1229
Han X, Zhu Y, Ting KM, Li G (2023) The impact of isolation kernel on agglomerative hierarchical clustering algorithms. Pattern Recogn 139:109517
Xia D, Wu Y, Wang Y, Zou X (2019) Order sequence optimization for parts-to-picker intelligent robot system. J Shenzhen Univ Sci Eng 36(06):696–701
Ward JH Jr (1963) Hierarchical grouping to optimize an objective function. J Am Stat Assoc 58(301):236–244
Download references
This work was supported by the Natural Science Foundation of Shandong Province (ZR2020KF027, ZR2023MF024, ZR2023MF121).
Author information
Authors and affiliations.
School of Electrical Engineering, University of Jinan, Jinan, 250022, Shandong, China
Shuhui Bi, Ronghao Shang, Haofeng Luo, Yuan Xu & Zhihao Li
School of Computing and Mathematical Sciences, University of Leicester, Leicester, East Midlands, LE1 7RH, UK
Yudong Zhang
You can also search for this author in PubMed Google Scholar
Contributions
All authors contributed to the study conception and design. Material preparation, data collection and analysis were performed by [SB], [ZL], [HL] and [YX]. The first draft of the manuscript was written by [SB], [ZL]. The second draft of the manuscript was finished by [SB] and [RS]. [YZ] participates partly the numerical simulation. All authors commented on previous versions of the manuscript. All authors read and approved the final manuscript.
Corresponding authors
Correspondence to Yuan Xu or Zhihao Li .
Ethics declarations
Conflict of interest.
The authors declare that there is no competing financial interest or personal relationship that could have appeared to influence the work reported in this paper.
Consent for publication
All authors consent that this article is publicated in Intelligent Service Robotics.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Supplementary Information
Below is the link to the electronic supplementary material.
Supplementary file 1 (xlsx 87005 KB)
Rights and permissions.
Springer Nature or its licensor (e.g. a society or other partner) holds exclusive rights to this article under a publishing agreement with the author(s) or other rightsholder(s); author self-archiving of the accepted manuscript version of this article is solely governed by the terms of such publishing agreement and applicable law.
Reprints and permissions
About this article
Bi, S., Shang, R., Luo, H. et al. HAC-based adaptive combined pick-up path optimization strategy for intelligent warehouse. Intel Serv Robotics (2024). https://doi.org/10.1007/s11370-024-00556-z
Download citation
Received : 17 June 2023
Accepted : 26 June 2024
Published : 18 August 2024
DOI : https://doi.org/10.1007/s11370-024-00556-z
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Hierarchical agglomerative clustering (HAC) algorithm
- Multi-robot path planning
- Dynamic weighted table
- Improved A* algorithm
- Find a journal
- Publish with us
- Track your research
Information
- Author Services
Initiatives
You are accessing a machine-readable page. In order to be human-readable, please install an RSS reader.
All articles published by MDPI are made immediately available worldwide under an open access license. No special permission is required to reuse all or part of the article published by MDPI, including figures and tables. For articles published under an open access Creative Common CC BY license, any part of the article may be reused without permission provided that the original article is clearly cited. For more information, please refer to https://www.mdpi.com/openaccess .
Feature papers represent the most advanced research with significant potential for high impact in the field. A Feature Paper should be a substantial original Article that involves several techniques or approaches, provides an outlook for future research directions and describes possible research applications.
Feature papers are submitted upon individual invitation or recommendation by the scientific editors and must receive positive feedback from the reviewers.
Editor’s Choice articles are based on recommendations by the scientific editors of MDPI journals from around the world. Editors select a small number of articles recently published in the journal that they believe will be particularly interesting to readers, or important in the respective research area. The aim is to provide a snapshot of some of the most exciting work published in the various research areas of the journal.
Original Submission Date Received: .
- Active Journals
- Find a Journal
- Proceedings Series
- For Authors
- For Reviewers
- For Editors
- For Librarians
- For Publishers
- For Societies
- For Conference Organizers
- Open Access Policy
- Institutional Open Access Program
- Special Issues Guidelines
- Editorial Process
- Research and Publication Ethics
- Article Processing Charges
- Testimonials
- Preprints.org
- SciProfiles
- Encyclopedia
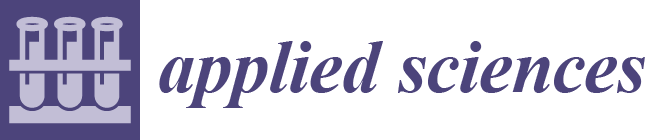
Article Menu

- Subscribe SciFeed
- Recommended Articles
- Google Scholar
- on Google Scholar
- Table of Contents
Find support for a specific problem in the support section of our website.
Please let us know what you think of our products and services.
Visit our dedicated information section to learn more about MDPI.
JSmol Viewer
Surveillance unmanned ground vehicle path planning with path smoothing and vehicle breakdown recovery.
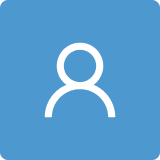
1. Introduction
2. route generation methodology.
Total Algorithmic Flow: Lower-Level and Higher-Level Path Generation |
Sub-area graphs with candidate start locations, total area graph, current vehicle locations, and vehicle route progress |
## Run lower-level path generation on all sub-areas to create several routes for each area |
sub_area sub_areas |
start_point sub_area |
lower-level path generation algorithm route |
infeasible turns route |
## Run higher-level path generation to create the total route for each vehicle |
## The higher-level path generation waits for vehicles to finish the assigned route, or for a vehicle to breakdown so it can re-generate the routes |
True |
higher-level path generation algorithm total paths |
all_vehicles_finsihed vehicle_breakdown |
all_vehicles_finsihed |
False |
vehicle_breakdown |
current_vehicle_locations vehicle_route_progress |
coverage paths for each vehicle |
2.1. Graph Formulation
2.2. lower-level path generation: ant colony optimization (aco), 2.2.1. foundation and basic principles, 2.2.2. modifications for the coverage salesman planning problem (csp), 2.3. higher-level path generation: genetic algorithm (ga), 2.3.1. foundation and basic principles, 2.3.2. chromosome structure, 2.3.3. crossover operation, 2.3.4. mutation operation, 2.3.5. objective function, 2.4. feasible path generation: turn radius consideration, 2.4.1. corner-cutting method, 2.4.2. reeds–shepp method, 2.5. real-time scheduling for breakdown scenarios.
Real-Time Scheduling for Breakdown Scenarios |
Paths assigned to each vehicle, vehicle locations, broken vehicle(s) |
## Calculate the remaining portion of each vehicle route |
## This portion updates the paths for the serviceable areas at the point of a breakdown |
vehicle vehicles |
current_location servicing |
current_location servicing_path |
start_points servicing_path |
Run the higher-level path generation to redistribute the remaining serviceable areas |
## The broken vehicle is not considered for this operation |
higher_level_path_generation broken_vehicle(s) |
coverage paths for each vehicle without the broken vehicle |
3. Study Parameters
3.1. vehicle specifications, 3.2. area specifications, 4. results and discussion, 5. conclusions, author contributions, institutional review board statement, informed consent statement, data availability statement, conflicts of interest.
- Ayub, M.F.; Ghawash, F.; Shabbir, M.A.; Kamran, M.; Butt, F.A. Next generation security and surveillance system using autonomous vehicles. In Proceedings of the Ubiquitous Positioning Indoor Navigation and Location Based Service (UPINLBS), Wuhan, China, 22–23 March 2018. [ Google Scholar ]
- Wu, Y.; Wu, S.; Hu, X. Cooperative path planning of UAVs & UGVs for a persistent surveillance task in urban environments. IEEE Internet Things J. 2021 , 8 , 4906–4919. [ Google Scholar ]
- Wang, T.; Huang, P.; Dong, G. Modeling and path planning for persistent surveillance by unmanned ground vehicle. IEEE Trans. Autom. Sci. Eng. 2021 , 18 , 1615–1625. [ Google Scholar ] [ CrossRef ]
- Brust, M.R.; Danoy, G.; Bouvry, P.; Gashi, D.; Pathak, H.; Goncalves, M.P. Defending against intrusion of malicious UAVs with networked UAV defense swarms. In Proceedings of the IEEE Conference on Local Computer Networks Workshops (LCN Workshops), Singapore, 9 October 2017. [ Google Scholar ]
- Waslander, S.L. Unmanned aerial and ground vehicle teams: Recent work and open problems. In Autonomous Control Systems and Vehicles: Intelligent Unmanned Systems ; Nonami, K., Kartidjo, M., Yoon, K.J., Budiyono, A., Eds.; Springer: Berlin, Germany, 2024; pp. 21–36. [ Google Scholar ]
- Yan, R.J.; Pang, S.; Sun, H.B.; Pang, Y.J. Development and missions of unmanned surface vehicle. J. Mar. Sci. Appl. 2010 , 9 , 451–457. [ Google Scholar ] [ CrossRef ]
- Chriki, A.; Touati, H.; Snoussi, H.; Kamoun, F. UAV-based surveillance system: An anomaly detection approach. In Proceedings of the IEEE Symposium on Computers and Communications (ISCC), Rennes, France, 7–10 July 2020. [ Google Scholar ]
- Xia, Y.; Batta, R.; Nagi, R. Controlling a fleet of unmanned aerial vehicles to collect uncertain information in a threat environment. Oper. Res. 2017 , 65 , 674–692. [ Google Scholar ] [ CrossRef ]
- Maheswaran, S.; Murugesan, G.; Duraisamy, P.; Vivek, B.; Selvapriya, S.; Vinith, S.; Vasantharajan, V. Unmanned ground vehicle for surveillance. In Proceedings of the 2020 11th International Conference on Computing, Communication and Networking Technologies (ICCCNT), Kharagpur, India, 1–3 July 2020. [ Google Scholar ]
- Kulkarni, P.P.; Krute, S.R.; Muchandi, S.S.; Patil, P.; Patil, S. Unmanned ground vehicle for security and surveillance. In Proceedings of the 2020 IEEE International Conference for Innovation in Technology (INOCON), Bangluru, India, 6–8 November 2020. [ Google Scholar ]
- Gadekar, A.; Fulsundar, S.; Deshmukh, P.; Aher, J.; Kataria, K.; Patel, V.; Barve, S. Rakshak: A modular unmanned ground vehicle for surveillance and logistics operations. Cogn. Rob. 2023 , 3 , 23–33. [ Google Scholar ] [ CrossRef ]
- Almoaili, E.; Kurdi, H. Path planning algorithm for unmanned ground vehicles (UGVs) in known static environments. Proc. Comp. Sci. 2020 , 177 , 57–63. [ Google Scholar ] [ CrossRef ]
- Ahmadzadeh, A.; Keller, J.; Pappas, G.; Jadbabaie, A.; Kumar, V. An optimization-based approach to time-critical cooperative surveillance and coverage with UAVs. In Experimental Robotics: Proceedings of the 10th International Symposium on Experimental Robotics, Rio de Janeiro, Brazil, 6–10 July 2008 ; Khatib, O., Kumar, V., Rus, D., Eds.; Springer: Berlin/Heidelberg, Germany, 2008; pp. 491–500. [ Google Scholar ]
- Maza, I.; Ollero, A. Multiple UAV cooperative searching operation using polygon area decomposition and efficient coverage algorithms. In Distributed Autonomous Robotic Systems ; Alami, R., Chatila, R., Asama, H., Eds.; Springer: Tokyo, Japan, 2007; pp. 221–230. [ Google Scholar ]
- Zhao, Z.; Zhang, Y.; Shi, J.; Long, L.; Lu, Z. Robust lidar-inertial odometry with ground condition perception and optimization algorithm for UGV. Sensors 2022 , 22 , 7424. [ Google Scholar ] [ CrossRef ]
- Current, J.R.; Schilling, D.A. The covering salesman problem. Transp. Sci. 1989 , 23 , 208–213. [ Google Scholar ] [ CrossRef ]
- Liu, Y.; Lin, X.; Zhu, S. Combined coverage path planning for autonomous cleaning robots in unstructured environments. In Proceedings of the World Congress on Intelligent Control and Automation (WCICA), Chongqing, China, 25–27 June 2008. [ Google Scholar ]
- Hameed, I.A. Coverage path planning software for autonomous robotic lawn mower using Dubins’ curve. In Proceedings of the IEEE International Conference on Real-Time Computing and Robotics (RCAR), Okinawa, Japan, 14–18 July 2017. [ Google Scholar ]
- Wang, Z.; Bo, Z. Coverage path planning for mobile robot based on genetic algorithm. In Proceedings of the IEEE Workshop on Electronics, Computer and Applications (IWECA), Ottawa, ON, Canada, 8–9 May 2014. [ Google Scholar ]
- Hameed, I.A.; Bochtis, D.D.; Sorensen, C.J. Driving angle and track sequence optimization for operational path planning using genetic algorithms. Appl. Eng. Agric. 2011 , 27 , 1077–1086. [ Google Scholar ] [ CrossRef ]
- Holland, J.H. Erratum: Genetic algorithms and the optimal allocation of trials. SIAM J. Comput. 1974 , 3 , 88–102. [ Google Scholar ] [ CrossRef ]
- Skinderowicz, R. Improving ant colony optimization efficiency for solving large TSP instances. Appl. Soft Comput. 2022 , 120 , 108653. [ Google Scholar ] [ CrossRef ]
- Gao, W. New ant colony optimization algorithm for the traveling salesman problem. Int. J. Comput. Intell. Syst. 2020 , 13 , 44–55. [ Google Scholar ] [ CrossRef ]
- Yao, X.S.; Ou, Y.; Zhou, K.Q. TSP solving utilizing improved ant colony algorithm. J. Phys. Conf. Ser. 2021 , 2129 , 012026. [ Google Scholar ] [ CrossRef ]
- Dutta, P.; Khan, I.; Basuli, K.; Maiti, M.K. A modified ACO with K-Opt for restricted covering salesman problems in different environments. Soft Comput. 2022 , 26 , 5773–5803. [ Google Scholar ] [ CrossRef ]
- Dorigo, M.; Stützle, T. Ant Colony Optimization ; MIT Press: Cambridge, MA, USA, 2004. [ Google Scholar ]
- Parsons, T.; Seo, J. FS-ACO: An algorithm for unsafe u-turn detours in service vehicle route optimization applications. In Proceedings of the 2023 23rd International Conference on Control, Automation and Systems (ICCAS), Yeosu, Republic of Korea, 17–20 October 2023. [ Google Scholar ]
- Li, G.; Liu, C.; Wu, L.; Xiao, W. A mixing algorithm of ACO and ABC for solving path planning of mobile robot. Appl. Soft Comput. 2023 , 148 , 110868. [ Google Scholar ] [ CrossRef ]
- Elcock, J.; Edward, N. An efficient ACO-based algorithm for task scheduling in heterogeneous multiprocessing environments. Array 2023 , 17 , 100280. [ Google Scholar ] [ CrossRef ]
- Almadhoun, R.; Taha, T.; Seneviratne, L.; Zweiri, Y. A survey on multi-robot coverage path planning for model reconstruction and mapping. SN Appl. Sci. 2019 , 1 , 847. [ Google Scholar ] [ CrossRef ]
- Xidias, E.; Zacharia, P.; Nearchou, A. Path planning and scheduling for a fleet of autonomous vehicles. Robotica 2016 , 34 , 2257–2273. [ Google Scholar ] [ CrossRef ]
- Parsons, T.; Baghyari, F.; Seo, J.; Kim, W.; Lee, M. Advanced path planning for autonomous street-sweeper fleets under complex operational conditions. Robotics 2024 , 13 , 37. [ Google Scholar ] [ CrossRef ]
- Niroumandrad, N.; Lahrichi, N.; Lodi, A. Learning tabu search algorithms: A scheduling application. Comp. Op. Res. 2024 , 170 , 106751. [ Google Scholar ] [ CrossRef ]
- Suanpang, P.; Jamjuntr, P.; Jermsittiparsert, K.; Kaewyong, P. Tourism service scheduling in smart city based on hybrid genetic algorithm simulated annealing algorithm. Sustainability 2022 , 14 , 16293. [ Google Scholar ] [ CrossRef ]
- Hu, Y.; Li, D.; He, Y.; Han, J. Path planning of UGV based on Bézier curves. Robotica 2019 , 37 , 969–997. [ Google Scholar ] [ CrossRef ]
- Hemmat, M.A.A.; Liu, Z.; Zhang, Y. Real-time path planning and following for nonholonomic unmanned ground vehicles. In Proceedings of the International Conference on Advanced Mechatronic Systems (ICAMechS), Xiamen, China, 6–9 December 2017. [ Google Scholar ]
- Parsons, T.; Hanafi Sheikhha, F.; Ahmadi Khiyavi, O.; Seo, J.; Kim, W.; Lee, S. Optimal path generation with obstacle avoidance and subfield connection for an autonomous tractor. Agriculture 2023 , 13 , 56. [ Google Scholar ] [ CrossRef ]
- Song, J.; Gupta, S.; Hare, J. Game-theoretic cooperative coverage using autonomous vehicles. In Proceedings of the Oceans, St. John’s, NL, Canada, 14–19 September 2014. [ Google Scholar ]
- Song, J.; Gupta, S. CARE: Cooperative autonomy for resilience and efficiency of robot teams for complete coverage of unknown environments under robot failures. Auton. Robot. 2020 , 44 , 647–671. [ Google Scholar ] [ CrossRef ]
- Reeds, J.A.; Shepp, L.A. Optimal paths for a car that goes both forwards and backwards. Pac. J. Math. 1990 , 145 , 367–393. [ Google Scholar ] [ CrossRef ]
- Sakai, A. Reeds Shepp Planning. Available online: https://atsushisakai.github.io/PythonRobotics/modules/path_planning/reeds_shepp_path/reeds_shepp_path.html (accessed on 28 February 2024).
Click here to enlarge figure
Parameter | Value |
---|---|
Travel Speed | 15 km/h |
Minimum Turning Radius | 5.60 m |
Scanning Range | 25.0 m |
Algorithm | Parameter | Value |
---|---|---|
Lower-Level Path Generation (ACO) | α (Pheromone Influence) | 0.1 |
β (Edge Distance Influence) | 0.3 | |
ρ (Pheromone Evaporation Rate) | 0.4 | |
Number of Ants * | 24 | |
Iterations | 250 | |
Higher-Level Path Generation (GA) | Generations | 60 |
Population Size | 20 | |
Crossover Probability | 0.9 | |
Mutation Probability | 0.05 | |
CPUs * | 5 |
Scenario | Number of Vehicles | Operating Conditions | Starting Locations |
---|---|---|---|
1 | 3 | Normal | Different Starting Locations |
2 | 3 | Normal | Same Starting Location |
3 | 3 | Normal | Same Starting Location |
4 | 5 | Normal | Same Starting Location |
5 | 3 | 1 Vehicle Breakdown After 700 m | Same Starting Location |
6 | 5 | 2 Vehicles Breakdown After 500 m | Same Starting Location |
Scenario | Vehicle | Distance (m) | Time (min) | Total Distance (m) | Overall Time (min) | Computation Time (s) |
---|---|---|---|---|---|---|
1 | 1 | 1364 | 5.40 | 5962 | 9.44 | 80 |
2 | 2239 | 8.96 | ||||
3 | 2359 | 9.44 | ||||
2 | 1 | 2718 | 10.87 | 6239 | 10.87 | 81 |
2 | 1348 | 5.39 | ||||
3 | 2173 | 8.69 | ||||
3 | 1 | 2293 | 9.17 | 5246 | 9.17 | 85 |
2 | 1157 | 4.63 | ||||
3 | 1796 | 7.18 | ||||
4 | 1 | 1396 | 5.58 | 6943 | 6.60 | 82 |
2 | 1649 | 6.60 | ||||
3 | 1621 | 6.48 | ||||
4 | 1120 | 4.48 | ||||
5 | 1157 | 4.63 | ||||
5 | 1 | 2479 | 9.92 | 5769 | 10.10 | 83 |
2 * | 765 | 3.06 | ||||
3 | 2525 | 10.10 | ||||
6 | 1 | 1429 | 5.71 | 7014 | 12.64 | 81 |
2 * | 549 | 2.2 | ||||
3 | 3160 | 12.64 | ||||
4 * | 563 | 2.25 | ||||
5 | 1313 | 5.25 |
The statements, opinions and data contained in all publications are solely those of the individual author(s) and contributor(s) and not of MDPI and/or the editor(s). MDPI and/or the editor(s) disclaim responsibility for any injury to people or property resulting from any ideas, methods, instructions or products referred to in the content. |
Share and Cite
Parsons, T.; Baghyari, F.; Seo, J.; Kim, B.; Kim, M.; Lee, H. Surveillance Unmanned Ground Vehicle Path Planning with Path Smoothing and Vehicle Breakdown Recovery. Appl. Sci. 2024 , 14 , 7266. https://doi.org/10.3390/app14167266
Parsons T, Baghyari F, Seo J, Kim B, Kim M, Lee H. Surveillance Unmanned Ground Vehicle Path Planning with Path Smoothing and Vehicle Breakdown Recovery. Applied Sciences . 2024; 14(16):7266. https://doi.org/10.3390/app14167266
Parsons, Tyler, Farhad Baghyari, Jaho Seo, Byeongjin Kim, Mingeuk Kim, and Hanmin Lee. 2024. "Surveillance Unmanned Ground Vehicle Path Planning with Path Smoothing and Vehicle Breakdown Recovery" Applied Sciences 14, no. 16: 7266. https://doi.org/10.3390/app14167266
Article Metrics
Further information, mdpi initiatives, follow mdpi.
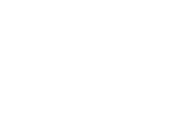
Subscribe to receive issue release notifications and newsletters from MDPI journals
- Machine Learning Decision Tree – Solved Problem (ID3 algorithm)
- Poisson Distribution | Probability and Stochastic Process
- Conditional Probability | Joint Probability
- Solved assignment problems in communicaion |online Request
- while Loop in C++
EngineersTutor
Solved assignment problems in c++ (with algorithm and flowchart).
Q1 . Create a program to compute the volume of a sphere. Use the formula: V = (4/3) *pi*r 3 where pi is equal to 3.1416 approximately. The r is the radius of sphere. Display the result.
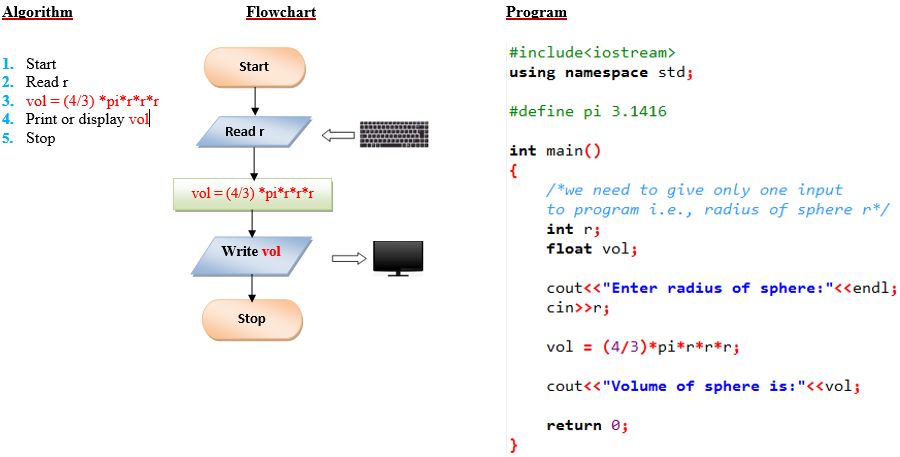
Q2 . Write a program the converts the input Celsius degree into its equivalent Fahrenheit degree. Use the formula: F = (9/5) *C+32.
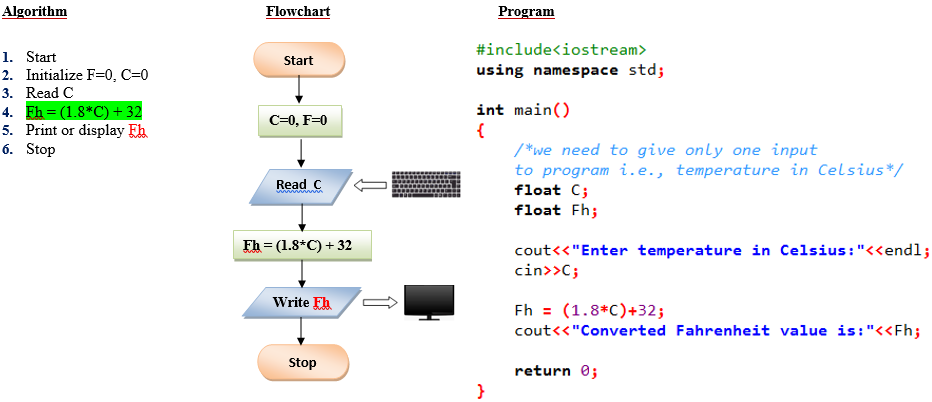
Q3 . Write a program that converts the input dollar to its peso exchange rate equivalent. Assume that the present exchange rate is 51.50 pesos against the dollar. Then display the peso equivalent exchange rate.
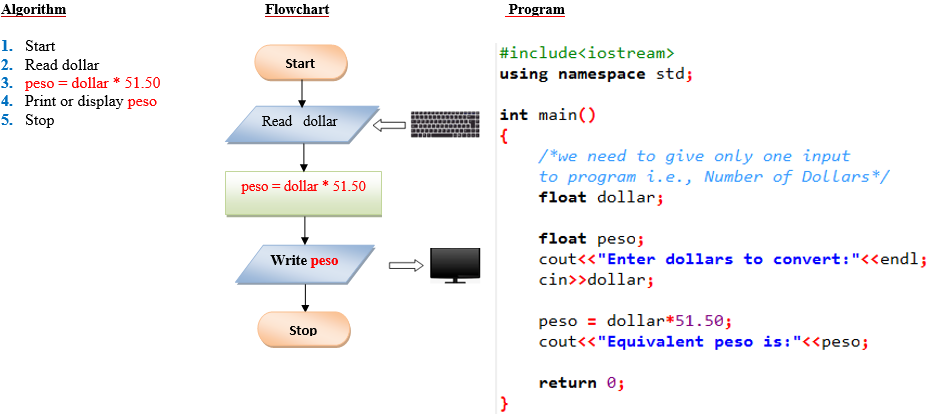
Q4 . Write a program that converts an input inch(es) into its equivalent centimeters. Take note that one inch is equivalent to 2.54cms.
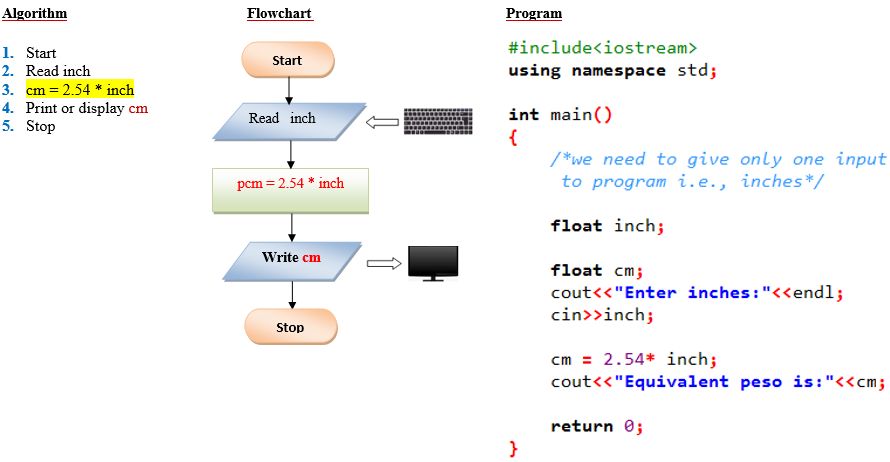
Q5 . Write a program that exchanges the value of two variables: x and y. The output must be: the value of variable y will become the value of variable x, and vice versa.
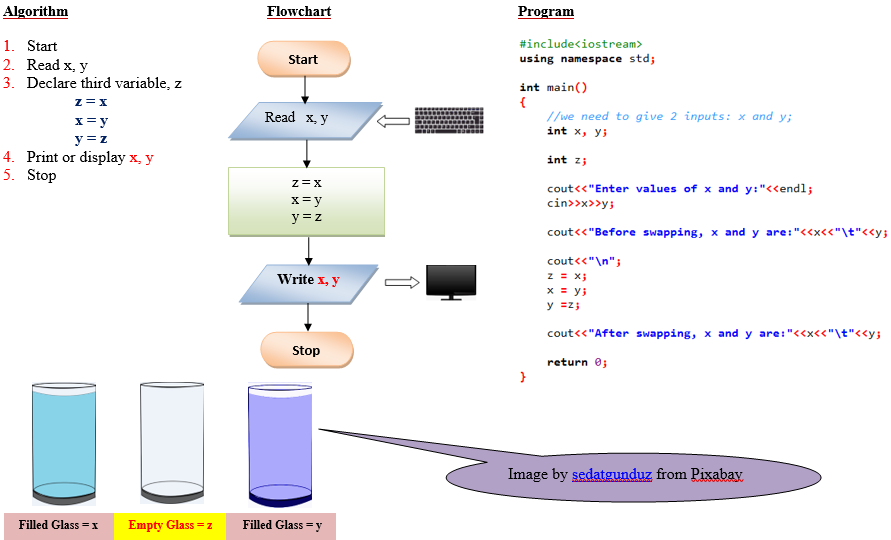
Q6 . Design a program to find the circumference of a circle. Use the formula: C=2πr, where π is approximately equivalent 3.1416.
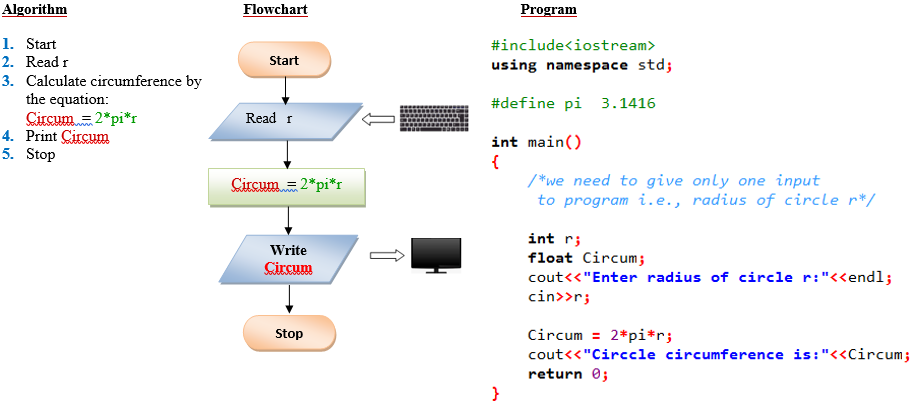
Q7 . Write a program that takes as input the purchase price of an item (P), its expected number of years of service (Y) and its expected salvage value (S). Then outputs the yearly depreciation for the item (D). Use the formula: D = (P – S) Y
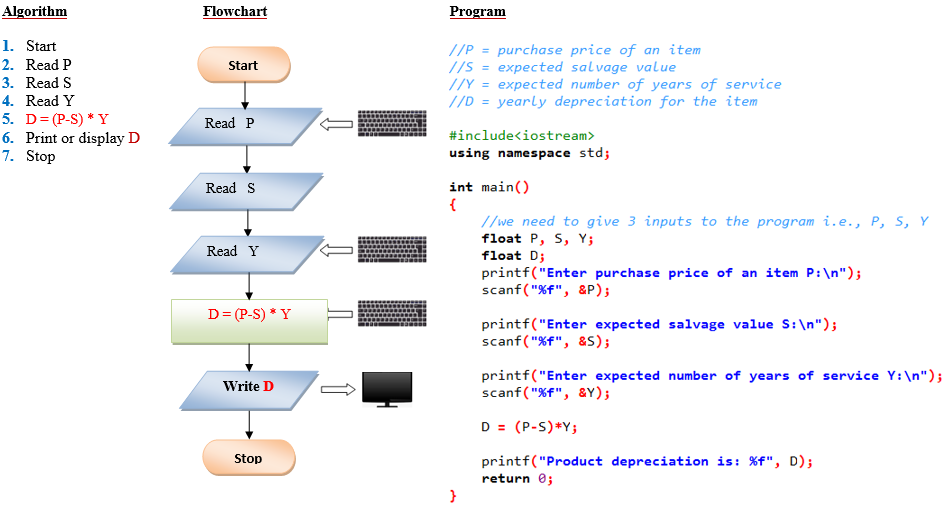
Q8 . Swapping of 2 variables without using temporary (or 3 rd variable).
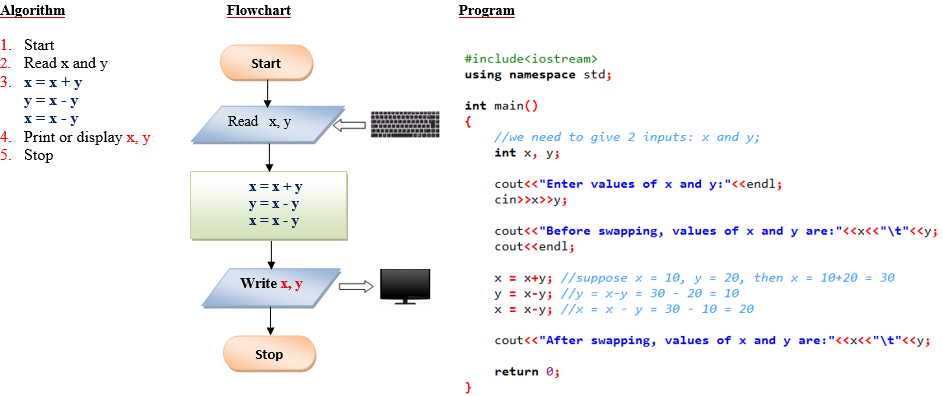
Q9 . Determine the most economical quantity to be stocked for each product that a manufacturing company has in its inventory: This quantity, called economic order quantity (EOQ) is calculated as follows: EOQ=2rs/1 where: R= total yearly production requirement S=set up cost per order I=inventory carrying cost per unit.
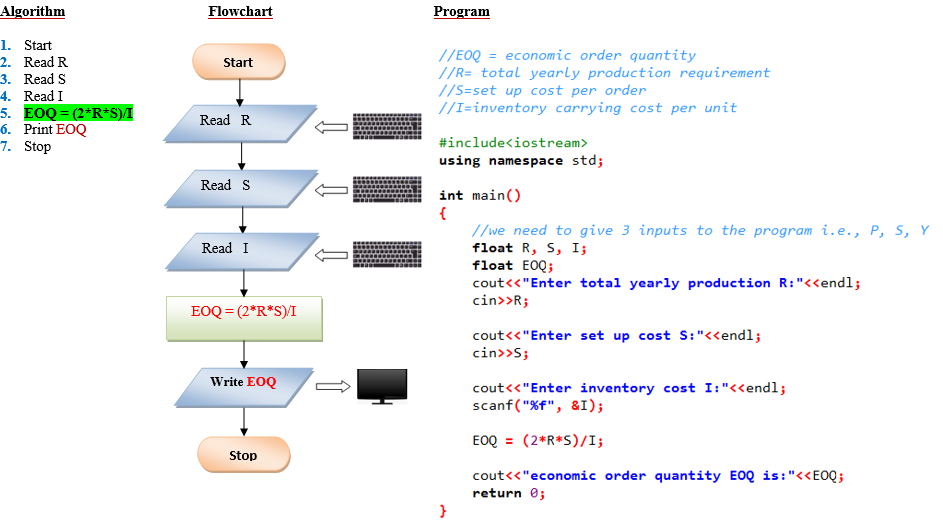
Q10 . Write a program to compute the radius of a circle. Derive your formula from the given equation: A=πr², then display the output.
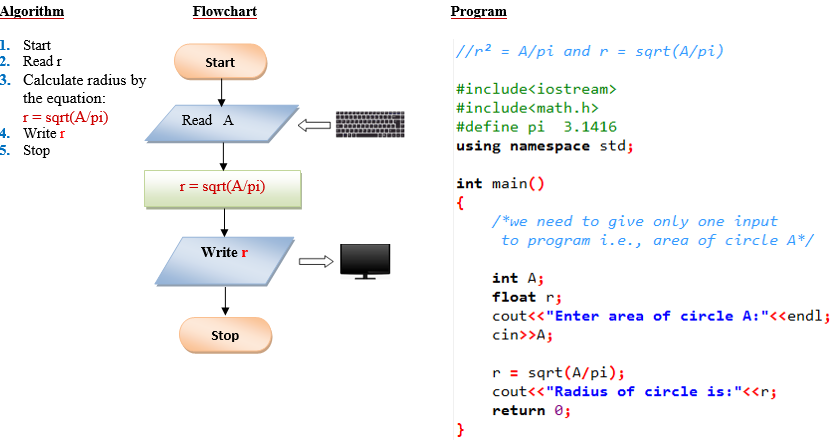
- ← Solved Assignment Problems in C (with Algorithm and Flowchart)
- Solved Assignment Problems in Java (with Algorithm and Flowchart) →
Gopal Krishna
Hey Engineers, welcome to the award-winning blog,Engineers Tutor. I'm Gopal Krishna. a professional engineer & blogger from Andhra Pradesh, India. Notes and Video Materials for Engineering in Electronics, Communications and Computer Science subjects are added. "A blog to support Electronics, Electrical communication and computer students".
You May Also Like
Arrays in c++, hierarchy of operators in c++, solved assignment problems in c++ – part2, leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
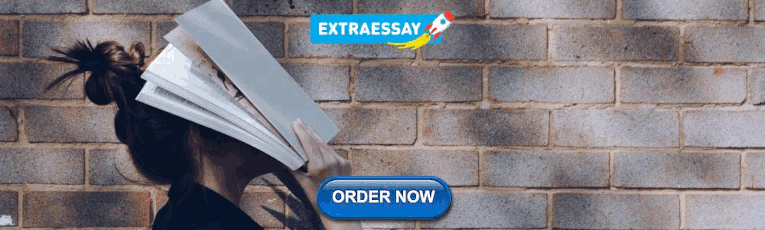
IMAGES
COMMENTS
Program. An algorithm is defined as sequence of steps to solve a problem (task). A flowchart is pictorial (graphical) representation of an algorithm. Set of instructions. Instruction is a command to the computer to do some task. Algorithm can also be defined as a plan to solve a problem and represents its logic. A picture is worth of 1000 words.
Some examples of algorithm and flowchart. Example1: To calculate the area of a circle. Algorithm: Step1: Start. Step2: Input radius of the circle say r. Step3: Use the formula πr 2 and store result in a variable AREA. Step4: Print AREA. Step5: Stop Flowchart: Example 2: Design an algorithm and flowchart to input fifty numbers and calculate ...
Explain the role of each. 1.3: Activity 3 - Using pseudo-codes and flowcharts to represent algorithms is shared under a license and was authored, remixed, and/or curated by LibreTexts. The student will learn how to design an algorithm using either a pseudo code or flowchart. Pseudo code is a mixture of English like statements, some mathematical ...
Draw a Flowchart for the Adding Two Numbers Algorithm. Step 1: Start Step 2: Declare a variable N1. Step 3: Declare a variable N2. Step 4: Declare a variable S to store the sum. Step 5: Get the value of N1 from the user. Step 6: Get the value of N2 from the user. Step 7: Add N1 and N2 and assign the result to S.
Algorithms, flowcharts, ... For example, the course might have 5 homework assignments, and each of these assignments might be assessed out of ten points. Take those scores to be 10, 7, 9, 10, and 10. ... Here is a nice example of flow chart construction for the game of hangman. Table Of Contents. Algorithms, flowcharts, and pseudocode. ...
Solved Assignment Problems in C (with Algorithm and Flowchart) Q1. Create a program to compute the volume of a sphere. Use the formula: V = (4/3) *pi*r 3 where pi is equal to 3.1416 approximately. The r is the radius of sphere. Display the result. Q2.
Pseudocode is a description of an algorithm using everyday wording, but molded to appear similar to a simplified programming language. In code-based flowcharts, common ANSI shapes are ovals for terminals, arrows for flowlines, rhomboids for inputs and outputs, rhombuses for decisions, and rectangles for processes.
2. Algorithms, flow charts and pseudocode 3. Procedural programming in Python 4. Data types and control structures 5. Fundamental algorithms 6. Binary encodings 7. Basics of computability and complexity 8. Basics of Recursion 9. Subject to time availability: Basics of Data File management 6 Liaqat Ali, Summer 2018. 5/13/2018 6
Examples of flowcharts in programming. 1. Add two numbers entered by the user. Flowchart to add two numbers. 2. Find the largest among three different numbers entered by the user. Flowchart to find the largest among three numbers. 3. Find all the roots of a quadratic equation ax2+bx+c=0.
Flowchart Tutorial (with Symbols, Guide and Examples) A flowchart is simply a graphical representation of steps. It shows steps in sequential order and is widely used in presenting the flow of algorithms, workflow or processes. Typically, a flowchart shows the steps as boxes of various kinds, and their order by connecting them with arrows.
Flowcharts Kenneth Leroy Busbee. Overview. A flowchart is a type of diagram that represents an algorithm, workflow or process. The flowchart shows the steps as boxes of various kinds, and their order by connecting the boxes with arrows. ... The rectangle depicts a process such as a mathematical computation, or a variable assignment.
Algorithms. 1. Introduction. In this tutorial, we'll study how to represent relevant programming structures into a flowchart, thus exploring how to map an algorithm to a flowchart and vice-versa. First, we'll understand why using pseudocode and flowcharts to design an algorithm before actually implementing it with a specific programming ...
An algorithm is defined as sequence of steps to solve a problem (task). The steps must be finite, well defined and unambiguous. Writing algorithm requires some thinking. Algorithm can also be defined as a plan to solve a problem and represents its logic. Note that an algorithm is of no use if it does not help us arrive at the desired solution.
Difference between Algorithm and Flowchart. If you compare a flowchart to a movie, then an algorithm is the story of that movie. In other words, an algorithm is the core of a flowchart.Actually, in the field of computer programming, there are many differences between algorithm and flowchart regarding various aspects, such as the accuracy, the way they display, and the way people feel about them.
Examples of pseudocode assignment statements: Cost ← 10 Cost has the value 10 Price ← Cost * 2 Price has the value 20 ... Algorithms and Flowcharts Faisal Chughtai (+92) 03008460713 www.faisalchughtai.com Using the example above, a better way of writing the algorithm would be. INPUT number1, number2
There are three building blocks of algorithms: sequencing, selection, and iteration. Sequencing is the sequential execution of operations, selection is the decision to execute one operation versus another operation (like a fork in the road), and iteration is repeating the same operations a certain number of times or until something is true.
A flowchart is a graphical representation of an algorithm.it should follow some rules while creating a flowchart. Rule 1: Flowchart opening statement must be 'start' keyword. Rule 2: Flowchart ending statement must be 'end' keyword. Rule 3: All symbols in the flowchart must be connected with an arrow line.
A flowchart is not a pictorial representation of steps to get the solution of a problem. False. Question 2. Algorithm means a set of rules which specify how to solve a specific problem. True. Question 3. Flowcharts are helpful in analyzing the logic of problems. True. Question 4. Connectors are used to connect the boxes. False. Question 5
Solved Assignment Problems in C (with Algorithm and Flowchart) Q1. Create a program to compute the volume of a sphere. Use the formula: V = (4/3) *pi*r3 where pi is equal to 3.1416 approximately. The r is the radius of sphere. Display the result. Algorithm Flowchart Program 1. Start 2. Read r 3. vol = (4/3) *pi*r*r*r 4.
ding on the value of the input variables.Step 4 Output the results of your algorithm's operations: In case of area of rectangle output will be the value stored in variable AREA. if the input variables described a rectangle with a HEIGHT of 2 and a WIDTH of 3, would output the value of 6.FLOWCHART: The first design of flowchart goes back to 19.
Place students into small groups and ask them to improve the algorithm for getting up and going to school in the morning (in the form of a flowchart). Tip: You can get the students to write down any algorithm (e.g. making the tea, tying a shoelace, making a jam sandwich etc.) however, I find, the more complex the algorithm, the easier it is to ...
Smart warehousing has been widely used due to its efficient storage and applications. However, the efficiency of transporting high-demand goods is still limited, because the existing methods lack path optimization strategies applicable to multiple scenarios and are unable to adapt conflict strategies to different warehouses. For solving these problems, this paper considers a multi-robot path ...
Solved Assignment Problems - Algorithms and Flowcharts Algorithm An algorithm is defined as sequence of steps to solve a problem (task). The steps must be finite, well defined and unambiguous. Writing algorithm requires some thinking. Algorithm can also be defined as a plan to solve a problem and represents its logic. Note that an algorithm is ...
As unmanned ground vehicles (UGV) continue to be adapted to new applications, an emerging area lacks proper guidance for global route optimization methodology. This area is surveillance. In autonomous surveillance applications, a UGV is equipped with a sensor that receives data within a specific range from the vehicle while it traverses the environment. In this paper, the ant colony ...
Solved Assignment Problems in C++ (with Algorithm and Flowchart) Q1. Create a program to compute the volume of a sphere. Use the formula: V = (4/3) *pi*r 3 where pi is equal to 3.1416 approximately. The r is the radius of sphere. Display the result. Q2.